How to Build a Web Crawler in Python: A Comprehensive Guide
By Rahul Lath on Aug 08, 2023
Updated Jan 30, 2025

Building a web crawler in Python can be a fun project that opens up many ways to collect and analyze data. A web crawler, also known as a spider or bot, is a program that systematically browses the web to gather information from websites. In the era of big data, web crawling using Python has become a crucial skill for data scientists, marketers, and researchers.
This guide will walk you through the process of creating a web crawler, covering everything from the prerequisites to best practices and legal considerations. Whether you’re a beginner looking to understand the basics or a seasoned programmer aiming to develop an advanced crawler, this tutorial has something for you.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Prerequisites to Building a Web Crawler
Basic Knowledge of Python
- Understanding of Python’s syntax and structure
- Familiarity with functions, loops, and conditional statements
Understanding of HTML and CSS
- Ability to read and interpret HTML tags
- Knowledge of CSS selectors to target specific elements
Familiarity with HTTP Requests
- Understanding of GET and POST requests
- Knowledge of how to handle cookies and sessions
Setting Up Your Environment
Installing Python
- Download the latest version of Python from the official website
- Follow the installation instructions for your operating system
Setting Up a Virtual Environment
- Create a virtual environment to manage dependencies
- Activate the environment using the appropriate command for your system
Essential Python Libraries for Web Crawling
- Requests: For making HTTP requests
- BeautifulSoup: For parsing HTML content
- Scrapy: A powerful framework for large-scale web crawling
Understanding the Basics of Web Crawling
What is Web Scraping and How is it Different from Web Crawling?
- Web Scraping: Extracting specific information from a web page
- Web Crawling: Navigating through websites to collect data, potentially leading to web scraping
Types of Web Crawlers
Different types of web crawlers are explained in detail on Datahut’s blog post. These include:
- Generic Crawlers: They browse the entire web without specific targets
- Focused Crawlers: They target specific websites or topics
- Incremental Crawlers: They only collect new or updated information
The Anatomy of a Web Page
Understanding the structure of a web page is essential in crawling. This includes:
- HTML Tags: The building blocks of a web page
- CSS Selectors: Used to style specific HTML elements
- JavaScript: May be used to load content dynamically
Understanding Robots.txt and Ethical Web Crawling
- Robots.txt: A file that tells web crawlers what pages they can or cannot request
- Ethical Crawling: Respecting the rules defined in robots.txt and not overloading servers
Diving into Python Libraries for Web Crawling
Introduction to BeautifulSoup
BeautifulSoup is a popular Python library for web scraping. It provides methods to search, navigate, and modify the parse tree.
Parsing HTML with BeautifulSoup
Here’s an example of how to parse HTML content:
1from bs4 import BeautifulSoup
2
3html_content = "<html><head><title>Web Crawling</title></head><body><p>This is an example.</p></body></html>"
4soup = BeautifulSoup(html_content, 'html.parser')
5print(soup.title.text) # Outputs: Web Crawling
Navigating the Parse Tree
BeautifulSoup allows you to navigate the HTML structure easily:
1paragraph = soup.p
2print(paragraph.text) # Outputs: This is an example.
Introduction to Scrapy
Scrapy is an open-source framework for extracting data from websites. It’s more powerful than BeautifulSoup and suitable for building web crawler in python for large-scale projects.
Creating a Scrapy Project
Start by creating a Scrapy project:
1scrapy startproject my_crawler
Understanding Spiders in Scrapy
Spiders are classes that Scrapy uses to scrape information from a website (or a group of websites).
Building Your First Web Crawler with BeautifulSoup
Planning Your Web Crawler
- Define the target website
- Determine the data you want to scrape
Writing the Code
Here’s a basic example to scrape titles from a website:
1import requests
2from bs4 import BeautifulSoup
3
4url = 'https://example.com'
5response = requests.get(url)
6soup = BeautifulSoup(response.content, 'html.parser')
7title = soup.title.text
8print(f"Title: {title}")
Testing and Debugging Your Web Crawler
- Test: Check the code by running it on different web pages.
- Common Errors:
- HTTP Error: Occurs when the request to the website fails.
- AttributeError: Happens if you try to access a tag that doesn’t exist in the HTML.
- ConnectionError: If there’s an issue with your internet connection.
Building a More Advanced Web Crawler with Scrapy
Planning Your Scrapy Web Crawler
- Define your target websites and data
- Understand the structure of the websites
Writing the Spider
Spiders in Scrapy are Python classes that define how a certain site or a group of sites will be scraped.
1import scrapy
2
3class MySpider(scrapy.Spider):
4 name = 'example'
5 start_urls = ['http://example.com']
6
7 def parse(self, response):
8 self.log('Visited %s' % response.url)
9 # Extracting data here
Storing the Data
You can store the scraped data in various formats like JSON, CSV, or XML.
Testing and Debugging Your Scrapy Web Crawler
- Testing: Scrapy provides a command-line tool to test the spiders.
- Common Errors:
- Spider Error: Issues within the spider itself.
- Middleware Error: Occurs when there’s an issue in the middlewares.
Overcoming Common Challenges in Web Crawling
Dealing with JavaScript-Loaded Content
Some websites load content dynamically using JavaScript. Standard HTTP requests may not fetch this content. A solution is to use tools like Selenium to interact with JavaScript.
Handling Captchas and Login Forms
Captchas are specifically designed to prevent bots like web crawlers. Some solutions:
- Avoiding sites with Captchas when possible.
- Using third-party services that solve Captchas (within legal bounds).
For login forms, credentials can be submitted using HTTP POST requests or tools like Selenium.
Managing Crawl Depth and Crawl Rate
- Crawl Depth: Refers to how deep the crawler should go into the site’s structure. This can be controlled in Scrapy using the DEPTH_LIMIT setting.
- Crawl Rate: Refers to how fast the crawler makes requests. It’s ethical to respect the server’s resources by controlling the crawl rate.
Best Practices and Legal Considerations in Web Crawling
Respecting Website Policies and Terms of Service
It’s vital to read and understand the target website’s terms of service and robots.txt file, which may contain rules specifically related to web crawling.
Ensuring Your Web Crawler is Polite
A polite web crawler in python considers the website’s robots.txt, doesn’t overwhelm the server, and identifies itself by providing contact information in the user agent string.
Understanding Legal Risks and How to Mitigate Them
The legal landscape around web crawling can be complex. Always consult with legal professionals to ensure that your web crawling activities comply with all relevant laws.
Conclusion
Building a web crawler in python is an exciting and valuable skill, with applications ranging from data mining to competitive analysis. By understanding the principles, utilizing powerful libraries like BeautifulSoup and Scrapy, and adhering to best practices and legal considerations, you can develop efficient and responsible web crawlers.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
Is Web Crawling Legal?
Generally, yes, but it depends on how you do it and the site’s terms of service.
How Can I Respect the Privacy of Website Users While Crawling?
Follow ethical guidelines and legal requirements regarding personal data. Read robots.txt
How Can I Improve the Speed of My Web Crawler?
Parallelize requests, manage the crawl depth and rate, and utilize efficient code.
How Can I Crawl a Website That Blocks Bots?
Follow the rules of the site. If crawling is allowed, you might have to do things like change the user agents.
What Are Some Common Uses of Web Crawlers?
Data analysis, market research, search engine indexing, etc.
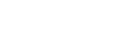
Jan 30, 2025
Was this helpful?