Python Tutorials
What is Memoization in Python?
Memorization is a programming and computer science technique that shortens the time it takes for a function to execute by caching its previously computed results. Memorization is helpful in Python for functions that carry out time-consuming or repetitive tasks, such as computing Fibonacci sequences or resolving difficult mathematical equations. Memorization speeds up the performance of the program by caching the output of these functions to cut down on computation time.
Memoization is a fundamental idea in Python programming that can increase a program’s effectiveness and speed. Python supports a number of implementation methods for memorization, including decorators. With the help of decorators, it is possible to improve or change the behavior of a function without altering its code in Python.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
What is Memoization?
Memory storage allows a program to access cached results rather than having to recalculate them each time the same inputs are passed to a function, which is how memory management works. The program checks to see if the result for a specific set of inputs has already been computed and stored in memory before calling a function with those inputs. The program retrieves the outcome and returns it as the function output if it is located in memory. The program executes the function and saves the output in memory for later use if the result cannot be found in memory.
Memorization has one major advantage over conventional computation techniques: it can drastically cut down on the time and resources needed to compute a function’s output. This is especially helpful when dealing with functions that perform repetitive tasks or have a high computational cost. The program can execute more quickly by avoiding the overhead of repeatedly computing the same result by caching the output of these functions.
Memoization is useful in various Python programming applications, such as:
- Recursive functions that call themselves with the same input values
- Computationally intensive functions, such as mathematical functions
- Functions that retrieve data from a remote source or database
How to Implement Memoization Using Decorators in Python
It is simple to implement memoization in Python using decorators. A function known as a decorator alters another function’s behavior without altering the source code of the target function. Here is a step-by-step tutorial for making a Python memoization decorator:
- Define a decorator function that takes a function as an argument.
- Define a dictionary variable to store the cached results.
- Inside the decorator function, define an inner function that takes the arguments of the decorated function.
- Check inside the inner function to see if the result for the supplied arguments is already in the results dictionary that has been cached. Return the cached outcome if it is. Execute the decorated function using the supplied arguments if it isn’t, and then save the outcome in the dictionary.
- Return the result from the inner function.
Here is an example of how to use the memoization decorator to cache the result of a Fibonacci sequence function:
def memoize(func):
cache = {}
def inner(n):
if n not in cache:
cache[n] = func(n)
return cache[n]
return inner
@memoize
def fib(n):
if n < 2:
return n
return fib(n-1) + fib(n-2)
print(fib(10))
The result of the fib function is cached in this example using the memoize decorator. The decorator determines whether the outcome for a particular value of n is already in the cache dictionary. If so, the function returns the result from the cache. If not, the function uses recursion to determine the Fibonacci sequence and saves the result in the cache dictionary for later use.
In Python programming, memory management is a potent technique that can shorten a program’s computation time and enhance performance. You can quickly cache the output of your functions and avoid the overhead of repeatedly computing the same output by implementing memoization using decorators in Python. This can be especially useful in applications that deal with computationally expensive or repetitive tasks. Understanding memoization and how to implement it using decorators is a valuable skill for any Python programmer to have.
Variations of Memoization Decorators
Depending on your particular use case, you can use a variety of memoization decorators:
Simple memoization decorator for functions without arguments: This decorator can be used for functions that do not take any arguments. It simply caches the function result based on its name.
def memoize(func):
cache = {}
def inner():
if func.__name__ not in cache:
cache[func.__name__] = func()
return cache[func.__name__]
return inner
Memoization decorator for functions with arguments: This decorator can be used for functions that take one or more arguments. It caches the result based on the arguments passed to the function.
def memoize(func):
cache = {}
def inner(*args):
if args not in cache:
cache[args] = func(*args)
return cache[args]
return inner
Memoization decorator with expiration time: This decorator can be used to have cached results automatically expire after a predetermined period of time. It accepts a second-long optional argument that specifies the expiration time.
import time
def memoize(expiration_time=60):
cache = {}
def decorator(func):
def inner(*args):
if args not in cache or time.time() - cache[args][1] > expiration_time:
cache[args] = (func(*args), time.time())
return cache[args][0]
return inner
return decorator
Memorization decorator with cache size restriction: This decorator can be used to set a limit on the number of results that can be stored in the cache. The least recently used result is removed from the cache when the cache size limit is reached to make room for fresh results.
import collections
def memoize(cache_size=100):
cache = collections.OrderedDict()
def decorator(func):
def inner(*args):
if args not in cache:
if len(cache) >= cache_size:
cache.popitem(last=False)
cache[args] = func(*args)
return cache[args]
return inner
return decorator
Pros and Cons of Memoization
Performance and speed benefits of memorization include the following:
- Reduced computation time: Memorization, especially for computationally expensive tasks, can significantly reduce the time needed to compute a function’s result.
- Increased program performance: By reducing the overhead of time-consuming or expensive computations, memorization can increase a program’s overall performance.
However, there may be some disadvantages to memoizing, such as:
- Memory issues may arise for large or complex programs because memorization increases the memory usage of a program by caching the outcomes of functions.
- Potential bugs: If memory management is improperly implemented, it can result in bugs in a program by caching results for mutable data types that can change between function calls.
Memorization may not be appropriate in some situations, such as when working with functions that have non-deterministic outputs or when the function’s inputs change frequently.
Best Practices for Using Memoization
To make sure that the memoization decorators are straightforward, readable, and dependable when using memoization in Python, it is crucial to adhere to best practices. Some best practices include:
- Maintaining memoization decorators’ simplicity and readability: Memoization decorators shouldn’t be overly complicated or challenging to modify. Instead, they should be simple to read and understand.
- Memoization decorators should have appropriate documentation and adhere to understandable naming conventions so that other programmers can understand their function and application.
- Testing and debugging memoization decorators: To ensure that they are working properly, memoization decorators should be thoroughly tested. Any bugs or problems should be fixed right away to avoid program errors.
- Using memoization sparingly and only for functions that are computationally expensive or perform repetitive tasks will prevent overuse. Memorization overuse can result in increased memory usage and potential program bugs.
By following these best practices, you can ensure that your memoization decorators are effective and reliable, and that they improve the performance of your Python programs.
Real World Examples
Numerous real-world scenarios can benefit from the use of decorators in memorization, including:
- Timing functions that use memoization: You can monitor how long it takes to compute a function for a specific input by caching the result of a function that uses memoization.
- Memorization can be used to cache API responses when an API request is made, which will cut down on the number of requests made and speed up the program’s response time.
- Memoizing complex functions for faster computation: Memoization can be particularly useful for complex functions that take a long time to compute, such as machine learning models or simulations.
Conclusion
Python’s decorator-based memory management is a potent method for enhancing the speed and effectiveness of programs. Memorization can significantly decrease computation time and enhance program performance by caching function results. However, in order to prevent potential bugs and memory problems, it is crucial to use memoization sparingly and in accordance with best practices. Overall, memoization is a useful tool that every Python programmer should possess.
With the help of decorators, which are a potent feature of Python, it is possible to improve or alter the behavior of functions without altering their source code. You can give a function extra functionality, like memoization or error handling, by enclosing it in a decorator. Decorators are a crucial tool for any Python programmer to have because they can be used to increase the effectiveness and performance of programs.
Finally, knowing how to use decorators effectively in Python programming can help you create programs that are more reliable and effective. In order to prevent problems, it is crucial to use decorators sparingly and in accordance with best practices, whether you are using them for error handling, memoization, or other functionality. You can advance your Python programming abilities by mastering decorators.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is memoization in Python?
Python uses a technique called memory to cache previously computed results, which speeds up the execution of a function. This contributes to the program’s performance improvement and decrease in computation time.
What is memoization in Python recursion?
Memoization in Python recursion involves caching the results of a function that calls itself with the same input values. This helps to avoid redundant computation and improve the performance of the recursive function.
What is the difference between memoize and cache in Python?
To store and retrieve previously computed results of a function, two techniques are used: memorize and cache. The primary distinction between the two is that while cache is a more general technique for storing and retrieving data in a program, memoize is used to cache the results of a single function.
Is memoization same as caching?
Memoization and caching are similar techniques used to store and retrieve previously computed results of a function. The main difference is that memoization is typically used for functions that take a long time to compute or perform repetitive tasks, while caching is used to store and retrieve any kind of data in a program.
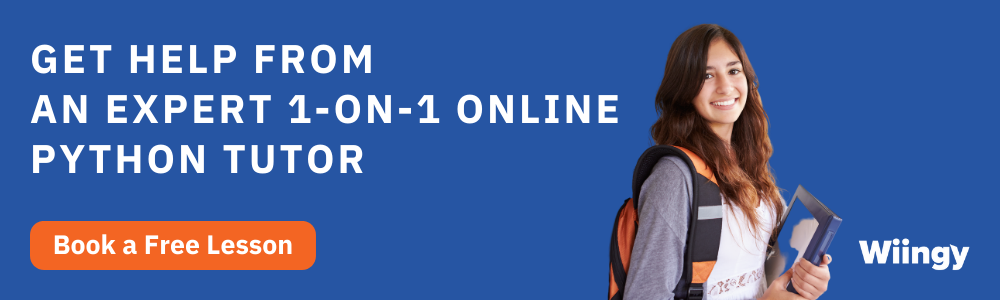
Written by by
Rahul LathReviewed by by
Arpit Rankwar