Printing output in python allows programmers to display text, variables, and computation results in the console or command-line interface. On the other hand, there are situations when we must print text or variables without beginning a new line.
Think about a situation where we need to print a line of numbers or characters. If we use the standard print() function, each output will start on a new line, so our output will be vertical. We can utilize Python’s “print without newline” capability to prevent this.
Python has a number of methods for printing text or variables without beginning a new line. In this post, we’ll look at one of the most popular techniques: using the print() function’s “end” option.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Using the end parameter
The end parameter in the print() function allows us to specify what character or string to use at the end of the printed output instead of the default newline character (\n). By default, the print() function uses the newline character to move to the next line after each print statement.
To print without a newline in Python, we can use the end parameter and set it to an empty string (”). Here is an example code demonstrating how to use the end parameter to print without a newline:
1# Example 1: Print without newline
2print("Hello ", end="")
3print("World!") # Output: Hello World!
In the above code, we first print the string “Hello ” with the end parameter set to an empty string. This tells Python not to start a new line after the “Hello ” string. Then, we print the “World!” string, which gets printed immediately after “Hello ” on the same line, resulting in the output “Hello World!” without a newline character.
We can also use the end parameter to print with a custom character or string at the end. For example:
1# Example 2: Print with a custom end character
2print("1", end="-")
3print("2", end="-")
4print("3") # Output: 1-2-3
In the above code, we print the numbers 1, 2, and 3 using the end parameter set to a hyphen (-). This results in the output “1-2-3” with hyphens between each number.
Using the sys.stdout.write() function
Another way to print without a newline in Python is by using the sys.stdout.write() function. This function writes a string to the standard output stream (stdout) without adding a newline character at the end.
Here is an example code demonstrating how to use the sys.stdout.write() function to print without a newline:
1import sys
2
3# Example 1: Print without newline using sys.stdout.write()
4sys.stdout.write("Hello, Welcome to Output in Python ")
5sys.stdout.write("World!") # Output: Hello World!
In the above code, we first import the sys module, which provides access to some variables used or maintained by the interpreter and to functions that interact strongly with the interpreter.
Then, we use the sys.stdout.write() function to print the strings “Hello ” and “World!” without a newline character. The output will be “Hello World!” printed on the same line.
We can also use the sys.stdout.write() function to print with a custom end character or string. For example:
1import sys
2
3# Example 2: Print with a custom end character using sys.stdout.write()
4sys.stdout.write("1")
5sys.stdout.write("-")
6sys.stdout.write("2")
7sys.stdout.write("-")
8sys.stdout.write("3") # Output: 1-2-3
In the above code, we print the numbers 1, 2, and 3 using the sys.stdout.write() function and a hyphen (-) as a custom end character. This results in the output “1-2-3” with hyphens between each number.
Comparison of the two methods
Both the end parameter in the print() function and the sys.stdout.write() function can be used to print without a newline in Python. However, there are some advantages and disadvantages of each method.
The end parameter method is simpler and easier to read, especially for beginners. It is also the recommended way of printing without a newline in most cases. The syntax is straightforward, and it can handle most use cases where printing without a newline is needed.
On the other hand, the sys.stdout.write() function is more low-level and advanced, and it may not be suitable for beginners. It also requires importing the sys module, which can be an additional step. However, it can be more flexible in certain scenarios, especially when dealing with non-string data types.
In general, the end parameter method is preferred for most use cases, unless there is a specific reason to use the sys.stdout.write() function. The end parameter method is more readable and less error-prone, and it can handle most scenarios where printing without a newline is needed.
Best Practices and Tips
When printing without a newline in Python, it is important to follow some best practices to avoid common errors and ensure that the output is correct.
Here are some best practices for printing without a newline in Python:
- Use the end parameter method in the print() function for most use cases. It is simpler and easier to read.
- Use the sys.stdout.write() function only when necessary, such as when dealing with non-string data types or when more advanced formatting is required.
- Use clear and descriptive strings for the output to make it more readable and understandable.
- Use proper indentation and spacing to make the code more readable and maintainable.
Here are some tips for avoiding common printing-related errors:
- Make sure to include the necessary parentheses, quotes, and other syntax elements when printing.
- Check for spelling and syntax errors in the strings being printed.
- Avoid using special characters or escape sequences in the strings being printed, as they can cause unexpected behavior.
- Test the code thoroughly to ensure that the output is correct and meets the requirements of the task at hand.
Conclusion
Printing without a newline is an important feature in Python that can be used to display output on a single line or to format output in a specific way. In this article, we have discussed two methods for printing without a newline in Python: using the end parameter in the print() function and using the sys.stdout.write() function.
The end parameter method is simpler and easier to read, while the sys.stdout.write() function is more low-level and advanced. Both methods have their advantages and disadvantages, and the choice of which method to use depends on the specific requirements of the task at hand.
To print without a newline in Python, it is important to follow best practices and avoid common errors. By doing so, we can ensure that the output is correct and meets the requirements of the task at hand.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is printing output in Python?
Printing output in Python is the process of displaying information to the user or saving it to a file or other output destination. In Python, the most common way to print output is by using the print() function. This function takes one or more arguments, which can be strings, numbers, or other types of data. The output is then displayed on the scree
What will be the output of print () statement?
The output of a print() statement in Python will depend on the arguments that are passed to the function. If a string is passed as an argument, it will be printed to the screen followed by a newline character. If a number or other data type is passed as an argument, it will be converted to a string and then printed to the screen. If multiple arguments are passed, they will be printed to the screen separated by spaces and followed by a newline character. If no arguments are passed, a blank line will be printed to the screen.
How do you print text output in Python?
To print text output in Python, you can use the print() function. The print() function takes one or more arguments, which can be strings or other data types. To print a single line of text, you can pass a string as an argument to the print() function. To print multiple lines of text, you can use the escape character ‘\n’ to create line breaks within a string and then pass it as an argument to the print() function.
How do you get output in Python?
To get output in Python, you can use the print() function to display information on the screen or send it to a file or other output destination. You can also use other methods to output information, such as writing to a file, using the logging module, or using the sys module to redirect output to a different destination.
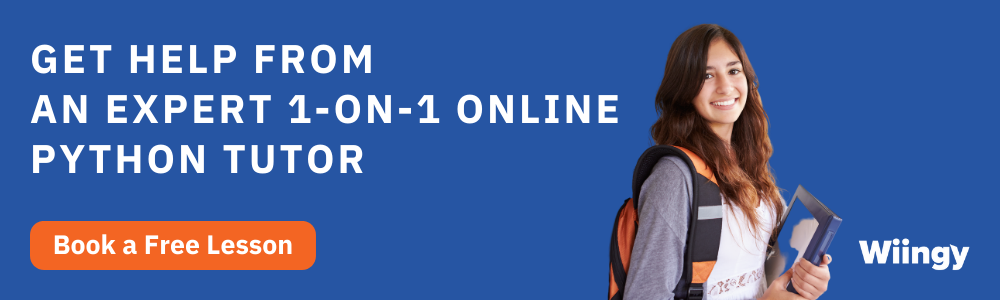
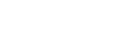
Jan 30, 2025
Was this helpful?