Python Tutorials
What is File Handling in Python?
Python programming requires the use of file handling, which enables users to read and write data to and from files. In plain English, file handling describes how Python communicates with files stored in a computer’s file system. Working with data stored in files is made simpler by Python’s built-in modules and functions for handling files.
This article’s goal is to offer a thorough introduction to file handling in Python, covering the fundamentals of opening and closing files, reading and writing files, and using various file handling modes.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Opening and Closing Files
The initial step in file handling is opening and closing files. A file must first be opened before any operation can be performed on it, and it must also be closed after the operation is finished. To open and close files in Python, use the built-in open() and close() methods, respectively.
There are different modes for opening files, which determine the operation that can be performed on a file. The modes include:
'r' - read mode (default)
'w' - write mode
'a' - append mode
'x' - exclusive creation mode
'b' - binary mode
't' - text mode (default)
To open a file in read mode, the ‘r’ mode is used. For write mode, the ‘w’ mode is used, which overwrites the file’s contents. In append mode, ‘a’ is used, which appends the data to the end of the file. The ‘x’ mode creates a new file and raises an error if the file already exists. For binary mode, ‘b’ is used, and for text mode, ‘t’ is used.
Here’s an example of how to open and close a file in Python:
# Open a file in read mode
file = open('example.txt', 'r')
# Perform operations on the file
# ...
# Close the file
file.close()
Reading and Writing Files
The next step after opening a file is to read or write data to or from it. Depending on the mode used to open the file, there are several ways to read and write files in Python.
The read() method is used to read a file, and it reads the whole thing. As an alternative, you can use the readline() method to read a line at a time or the readlines() method to read every line into a list.
To write to a file, the write() method is used, which writes the data to the file. In write mode, the file’s contents are overwritten, so care should be taken to avoid losing any data. In append mode, the write() method adds data to the end of the file.
Here’s an example of how to read and write files in Python:
# Open a file in write mode
file = open('example.txt', 'w')
# Write data to the file
file.write('Hello, World!')
# Close the file
file.close()
# Open the file in read mode
file = open('example.txt', 'r')
# Read the contents of the file
data = file.read()
# Print the data
print(data)
# Close the file
file.close()
File Objects
In Python, files opened in the program are represented by objects called file objects. These objects have methods that let users open and close files and read and write data, among other operations. The file object methods read(), readline(), write(), and seek() are a few of the frequently used ones.
While the readline() method reads one line at a time, the read() method reads the entire file or a specified number of bytes from the file. The seek() method moves the current file position to a predetermined position, while the write() method adds data to the file.
Here’s an example of how to use file object methods in Python:
# Open a file in read mode
file = open('example.txt', 'r')
# Read the first 10 bytes of the file
data = file.read(10)
# Print the data
print(data)
# Change the file position to byte 20
file.seek(20)
# Read the next 10 bytes of the file
data = file.read(10)
# Print the data
print(data)
# Close the file
file.close()
File Handling in Python – Best Practices
It’s crucial to adhere to best practices when handling files in Python in order to make sure the program runs smoothly and without errors. To always handle errors when opening and closing files is one of the best practices. An error occurs, for instance, if you attempt to open a file that doesn’t exist. Users can use a try-except block to handle the error and give the user a message in order to prevent this.
Always closing the file after performing operations on it is another best practice. If the file is not properly closed, it may result in program errors and data loss. When opening files, it’s also a good idea to use with statements because they automatically close the file once the operations are finished.
Creating folders and giving files descriptive names are examples of good file handling techniques. Absolute file paths should also be avoided by users because they can result in issues when running the program on various computers.
Reading and Writing to CSV Files
Data in tabular format is frequently stored in CSV files, and Python comes with a built-in csv module to read and write to these files. CSV files can be handled using the csv module’s functions, such as csv.reader() and csv.writer().
The csv.reader() function, which reads the contents of the file and returns it as a list of lists, is used to read data from a CSV file. Each list corresponds to a row and each list element to a column in the CSV file.
The csv.writer() function is used to write data to a CSV file, and it writes the data to the file in CSV format.
Here’s an example of how to read and write to CSV files in Python:
import csv
# Open a CSV file in read mode
with open('example.csv', 'r') as file:
reader = csv.reader(file)
# Print each row in the CSV file
for row in reader:
print(row)
# Open a CSV file in write mode
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
# Write data to the CSV file
writer.writerow(['Name', 'Age', 'City'])
writer.writerow(['John', '25', 'New York'])
writer.writerow(['Jane', '30', 'Los Angeles'])
Reading and Writing to JSON Files
The lightweight data interchange format JSON (JavaScript Object Notation) is frequently used for storing and exchanging data between web applications. To read and write data in JSON format, Python includes a built-in json module. To read and write to JSON files, the json module includes functions like json.dump() and json.load().
The json.dump() function is used to write data to a JSON file, and it writes the data to the file in JSON format. Using the json.load() function, which reads a JSON file’s contents and returns them as a Python object, you can read data from a JSON file.
Here’s an example of how to read and write to JSON files in Python:
import json
# Write data to a JSON file
data = {'name': 'John', 'age': 25, 'city': 'New York'}
with open('example.json', 'w') as file:
json.dump(data, file)
# Read data from a JSON file
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
Conclusion
The ability to work with data stored in files is made possible by file handling, which is a crucial component of Python programming. Python programmers need to be adept at opening and closing files, reading from and writing to files, and comprehending various file formats. The program will run more smoothly if best practices are followed, such as handling errors when opening and closing files and organizing files into folders.
Overall, for Python programmers who want to manipulate data stored in files, understanding file handling and knowing how to handle files effectively are essential.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is Python file handling?
Python’s interaction with files on a computer’s file system is referred to as file handling. This includes opening and closing files, reading from and writing to files, and comprehending various file formats.
What is file handling?
File handling is how a computer program opens and closes files, reads and writes data to files, and understands different file formats.
What are the types of file in file handling in Python?
Text, binary, CSV, JSON, and other types of files can all be handled by Python’s file handling system
What is the csv module in Python used for?
Data can be read from and written to CSV files using the Python csv module
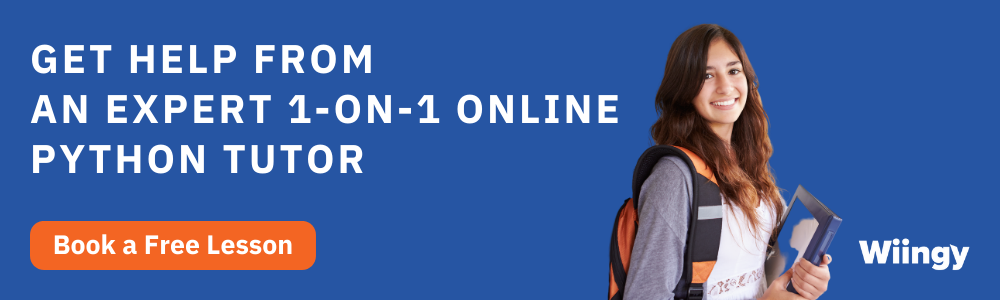
Written by by
Rahul LathReviewed by by
Arpit Rankwar