What are Python Sets?
Python sets are a grouping of distinct objects. It is a group of elements that is neither ordered nor immutable. The set() function or curly braces can be used to create sets. A set’s components can be of any data type, including strings, integers, and even other sets.
The fact that a set forbids duplicate elements is one of its primary characteristics. A set won’t change if an element is already there; adding it again won’t. Additionally, sets do not keep the elements they contain in any particular order. This means that the order in which the elements are added to the set does not matter.
B. Difference between sets and other data structures
Although sets resemble other data structures like lists and tuples, there are some significant differences between them. The biggest distinction is that lists and tuples support duplicate elements, whereas sets do not. Lists and tuples are ordered, whereas sets are unordered. Tuples are immutable, whereas sets are also mutable, meaning they can be changed after creation.
C. How sets are used in Python
In Python, sets are used to carry out set operations such as union, intersection, and difference. Algorithms and calculations in mathematics frequently use set operations. Duplicates can also be eliminated from a list or tuple using sets.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Creating Sets in Python
A. Literal notation
Sets can be created using curly braces {} with elements separated by commas. Here is an example of creating a set with integers:
1my_set = {1, 2, 3, 4, 5}
B. Using the set() constructor
Another way to create a set is by using the set() constructor function. The constructor function can take any iterable as an argument and returns a set containing all the unique elements in the iterable. Here is an example of creating a set using the constructor function:
1my_set = set([1, 2, 3, 4, 5])
C. Creating an empty set
To create an empty set, you cannot use empty curly braces as they will be interpreted as an empty dictionary. Instead, you can use the set() constructor function to create an empty set:
1my_set = set()
Set Size and Membership
You can determine the size of a set by using the len() function. The len() function returns the number of elements in a set. Here is an example of using the len() function to determine the size of a set:
1my_set = {1, 2, 3, 4, 5} print(len(my_set)) # Output: 5
Checking if an element is in a set
You can check if an element is in a set by using the in keyword. The in keyword returns True if the element is in the set and False otherwise. Here is an example of checking if an element is in a set:
1my_set = {1, 2, 3, 4, 5} print(3 in my_set) # Output: True print(6 in my_set) # Output: False
Operating on a Set
A. Operators vs. Methods
Sets support both operators and methods for performing operations on them. Operators are symbols that perform an operation on two or more sets, while methods are functions that are called on a single set to modify it or perform an operation on it.
B. Available Operators and Methods
The following are the available operators and methods for sets in Python:
- Union Operator (|) and union() method: The union operator and the union() method return a set containing all the elements from both sets.
- Intersection Operator (&) and intersection() method: The intersection operator and the intersection() method return a set containing only the elements that are common in both sets.
- Difference Operator (-) and difference() method: The difference operator and the difference() method return a set containing the elements that are in the first set but not in the second set.
- Symmetric Difference Operator (^) and symmetric_difference() method: The symmetric difference operator and the symmetric_difference() method return a set containing the elements that are in either of the sets but not in both.
- Subset Operator (<) and issubset() method: The subset operator and the issubset() method return True if the first set is a subset of the second set.
- Superset Operator (>) and issuperset() method: The superset operator and the issuperset() method return True if the first set is a superset of the second set.
- Disjoint Operator and isdisjoint() method: The disjoint operator and the isdisjoint() method return True if the two sets have no common elements.
C. Examples of using operators and methods
Here are some examples of using operators and methods with sets:
1set1 = {1, 2, 3, 4} set2 = {3, 4, 5, 6} # Using union operator union_set = set1 | set2 print(union_set) # Output: {1, 2, 3, 4, 5, 6}
2# Using intersection method
3intersection_set = set1.intersection(set2) print(intersection_set)
4# Output: {3, 4}
5# Using difference operator
6difference_set = set1 - set2 print(difference_set)
7# Output: {1, 2}
8# Using symmetric difference
9method symmetric_difference_set = set1.symmetric_difference(set2) print(symmetric_difference_set)
10# Output: {1, 2, 5, 6}
11# Using subset operator
12print(set1 < set2)
13# Output: False
14# Using issubset method
15print(set1.issubset(set2))
16# Output: False
17# Using disjoint operator
18print(set1.isdisjoint(set2))
19# Output: False
Modifying a Set
A. Adding an element to a set
To add an element to a set, you can use the add() method. The add() method adds an element to the set if it is not already present in the set.
1my_set = {1, 2, 3} my_set.add(4) print(my_set)
2# Output: {1, 2, 3, 4}
B. Removing an element from a set
To remove an element from a set, you can use the remove() method. The remove() method removes the element from the set if it is present in the set, and raises a KeyError if the element is not in the set.
1my_set = {1, 2, 3} my_set.remove(2) print(my_set)
2 # Output: {1, 3}
C. Updating a set with another set
To update a set with another set, you can use the update() method. The update() method adds all the elements from the given set(s) to the original set.
1my_set = {1, 2, 3} my_set.update({3, 4, 5}) print(my_set) # Output: {1, 2, 3, 4, 5}
D. Clearing a set
To remove all the elements from a set, you can use the clear() method. The clear() method removes all the elements from the set and makes it an empty set.
1my_set = {1, 2, 3} my_set.clear() print(my_set)
2 # Output: set()
Augmented Assignment Operators and Methods
Examples of using augmented assignment operators
Augmented assignment operators are shorthand notations for performing an operation and assigning the result back to the original variable. For example, the following statement is equivalent to x = x + 1:
1x += 1
The following are the available augmented assignment operators for sets in Python:
- Union assignment operator (|=): The union assignment operator adds all the elements from the second set to the first set.
1set1 = {1, 2, 3} set2 = {3, 4, 5} set1 |= set2 print(set1) # Output: {1, 2, 3, 4, 5}
- Intersection assignment operator (&=): The intersection assignment operator keeps only the elements that are common in both sets.
1set1 = {1, 2, 3} set2 = {3, 4, 5} set1 &= set2 print(set1) # Output: {3}
- Difference assignment operator (-=): The difference assignment operator removes the elements from the first set that are present in the second set.
1set1 = {1, 2, 3} set2 = {3, 4, 5} set1 -= set2 print(set1)
2# Output: {1, 2}
- Symmetric difference assignment operator (^=): The symmetric difference assignment operator keeps only the elements that are in either of the sets but not in both.
1set1 = {1, 2, 3} set2 = {3, 4, 5} set1 ^= set2 print(set1) # Output: {1, 2, 4, 5}
B. Examples of using methods for augmented assignment
The following are the available methods for performing augmented assignment on sets in Python:
- update() method: The update() method adds all the elements from the given set(s) to the original set.
1set1 = {1, 2, 3} set2 = {3, 4, 5} set1.update(set2) print(set1)
2 # Output: {1, 2, 3, 4, 5}
- intersection_update() method: The intersection_update() method keeps only the elements that are common in both sets.
1set1 = {1, 2, 3} set2 = {3, 4, 5} set1.intersection_update(set2) print(set1) # Output: {3}
- difference_update() method: The difference_update() method removes the elements from the first set that are present in the second set.
1set1 = {1, 2, 3} set
Other Methods for Modifying Sets
A. Intersection, Union, and Difference
Sets in Python have three methods for performing the basic set operations – union, intersection, and difference.
- The union() method returns a set containing all the distinct elements from both sets.
1set1 = {1, 2, 3} set2 = {3, 4, 5} set3 = set1.union(set2) print(set3) # Output: {1, 2, 3, 4, 5}
- The intersection() method returns a set containing only the common elements from both sets.
1set1 = {1, 2, 3} set2 = {3, 4, 5} set3 = set1.intersection(set2) print(set3) # Output: {3}
- The difference() method returns a set containing the elements that are present in the first set but not in the second set.
1set1 = {1, 2, 3} set2 = {3, 4, 5} set3 = set1.difference(set2) print(set3) # Output: {1, 2}
B. Symmetric Difference and Disjoint
Sets in Python have two methods for performing symmetric difference and disjoint operations.
- The symmetric_difference() method returns a set containing the elements that are in either of the sets but not in both.
1set1 = {1, 2, 3} set2 = {3, 4, 5} set3 = set1.symmetric_difference(set2) print(set3) # Output: {1, 2, 4, 5}
- The isdisjoint() method returns True if two sets have no common elements.
1set1 = {1, 2, 3} set2 = {4, 5, 6} print(set1.isdisjoint(set2)) # Output: True
C. Subsets, Supersets, and Proper Subsets
Sets in Python have three methods for checking if a set is a subset, superset, or proper subset of another set.
- The issubset() method returns True if all the elements of a set are present in another set.
1set1 = {1, 2, 3} set2 = {1, 2} print(set2.issubset(set1)) # Output: True
- The issuperset() method returns True if a set contains all the elements of another set.
1set1 = {1, 2, 3} set2 = {1, 2} print(set1.issuperset(set2)) # Output: True
- The ispropersubset() method returns True if a set is a proper subset of another set, i.e., it is a subset but not equal.
1set1 = {1, 2, 3} set2 = {1, 2} print(set2.ispropersubset(set1)) # Output: True
D. Copying a Set
To create a copy of a set, you can use the copy() method or the set() constructor.
1set1 = {1, 2, 3} set2 = set1.copy() set3 = set(set1) print(set2) # Output: {1, 2, 3} print(set3) # Output: {1, 2, 3}
Frozen Sets
A. Definition of frozen sets
Frozen sets are immutable collections of unique elements that are hashable, which means they can be used as elements of a set, but they cannot be modified once they are created. Frozen sets are created using the frozenset() constructor.
1fset = frozenset([1, 2, 3]) print(fset) # Output: frozenset({1, 2, 3})
B. Creating frozen sets
Frozen sets can be created from other sets or any iterable using the frozenset() constructor.
1set1 = {1, 2, 3} fset = frozenset(set1) print(fset) # Output: frozenset({1, 2, 3})
C. Differences between frozen sets and sets
Frozen sets differ from sets in that they are immutable and hashable, which means they can be used as keys in dictionaries and elements of other sets.
1fset = frozenset([1, 2, 3]) d = {fset: 'hello'} print(d)
2 # Output: {frozenset({1, 2, 3}): 'hello'}
Since frozen sets cannot be modified, they do not have any methods for adding, removing, or updating elements like sets do.
Set Comprehensions
A. Basic syntax
Set comprehensions provide a concise way to create sets in Python. The basic syntax of a set comprehension is similar to that of a list comprehension, except that it is enclosed in curly braces instead of square brackets.
1set1 = {x for x in range(10)} print(set1)
2 # Output: {0, 1, 2, 3, 4, 5, 6, 7, 8, 9}
B. Creating sets with set comprehensions
Set comprehensions can be used to create sets from any iterable, including other sets.
1set1 = {x**2 for x in range(10)} print(set1)
2# Output: {0, 1, 4, 9, 16, 25, 36, 49, 64, 81}
3set2 = {x for x in set1 if x % 2 == 0} print(set2)
4# Output: {0, 64, 4, 36, 16, 8}
C. Using set comprehensions with conditionals
Set comprehensions can also be used with conditionals to filter elements.
1set1 = {x for x in range(10) if x % 2 == 0} print(set1)
2# Output: {0, 2, 4, 6, 8}
Applications of Sets in Python
A. Removing duplicates from a list
One common application of sets in Python is to remove duplicates from a list. We can easily convert a list to a set to remove duplicates, and then convert it back to a list if necessary.
1list1 = [1, 2, 3, 2, 1, 4, 5] set1 = set(list1) list2 = list(set1) print(list2) # Output: [1, 2, 3, 4, 5]
B. Finding common elements in multiple lists
Sets can also be used to find common elements in multiple lists. We can convert each list to a set and then take the intersection of the sets to find the common elements.
1list1 = [1, 2, 3, 4, 5] list2 = [4, 5, 6, 7, 8] set1 = set(list1) set2 = set(list2) common = set1.intersection(set2) print(common) # Output: {4, 5}
C. Checking for membership in a set
Sets are also useful for checking if an element is in a collection. Since sets use hash tables for fast membership testing, they are very efficient for large collections.
1set1 = {1, 2, 3, 4, 5} if 3 in set1: print('3 is in the set') # Output: 3 is in the set
D. Performing set operations on large datasets
Sets are also useful for performing set operations like union, intersection, and difference on large datasets. Since sets are implemented using hash tables, they are very efficient for these types of operations.
1set1 = {1, 2, 3, 4, 5} set2 = {4, 5, 6, 7, 8} union = set1.union(set2) intersection = set1.intersection(set2) difference = set1.difference(set2) print(union) # Output: {1, 2, 3, 4, 5, 6, 7,8}
Conclusion
- A set is an unordered collection of unique elements in Python.
- Sets are defined using the set() constructor or with literal notation {}.
- Sets are mutable, and their contents can be modified using a variety of methods.
- Sets have built-in methods for performing operations like union, intersection, difference, and more.
- Set comprehensions provide a concise way to create sets in Python.
Python sets provide a powerful and efficient way to work with collections of unique elements. They are useful in a variety of programming tasks, from removing duplicates to performing set operations on large datasets. Understanding how to create, modify, and operate on sets is an important part of becoming a proficient Python programmer.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs:
Q: What are sets in Python?
A: Sets in Python are an unordered collection of unique elements. They are defined using the set() constructor or with literal notation {}.
Q: What are the 2 types of sets in Python?
A: There are two types of sets in Python: mutable sets and immutable sets (frozen sets). Mutable sets can be modified, while frozen sets cannot.
Q: What are Python sets good for?
A: Python sets are good for a variety of programming tasks, such as removing duplicates from a list, finding common elements in multiple lists, checking for membership in a set, and performing set operations on large datasets.
Q: Is {} a set in Python?
A: No, {} is not a set in Python. It is the literal notation for an empty dictionary. To create an empty set, you should use set() instead.
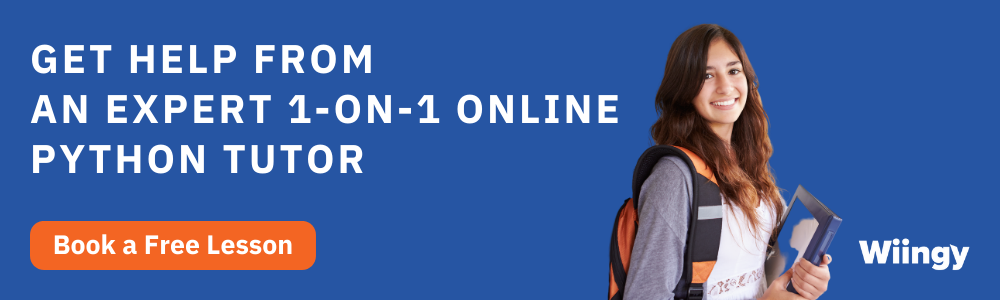
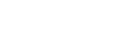
Jan 30, 2025
Was this helpful?