Python Tutorials
What are Equality (==) and Identity (is) Operators in Python
In Python, the “==” (Equality operators) and “is” (Identify operators) are used to compare objects. The “==” operator compares the values of two objects, whereas the “is” operator compares the identity of two objects.
Understanding the difference between these operators is important because they behave differently, and using the wrong operator can lead to unexpected behavior in your code.
This blog post will explain the difference between the “==” and “is” operators in Python, provide example code snippets, and offer best practices for using each operator.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Explanation of the == Operator
A. Explanation of how the == operator works in Python
The “==” operator checks if the values of two objects are equal. It returns “True” if the values are the same and “False” otherwise.
B. Use cases for the == operator
The “==” operator is commonly used to compare strings, numbers, and other data types for equality.
C. Comparison of variables with the == operator
When using the “==” operator, the values of two variables are compared. For example, if x == y, Python will check if the values of x and y are equal.
D. Example code snippets to illustrate the use of the == operator
x = 10
y = 10
if x == y:
print("x and y are equal")
else:
print("x and y are not equal")
Explanation of the is Operator
A. Explanation of how the is operator works in Python
The “is” operator checks if two variables refer to the same object in memory. It returns “True” if the objects are the same and “False” otherwise.
B. Use cases for the is operator
The “is” operator is commonly used to check if a variable is None or if two variables point to the same object in memory.
C. Comparison of variables with the is operator
When using the “is” operator, the memory address of two variables is compared. For example, if x is y, Python will check if x and y point to the same object in memory.
D. Example code snippets to illustrate the use of the is operator
x = [1, 2, 3]
y = [1, 2, 3]
if x is y:
print("x and y point to the same object")
else:
print("x and y do not point to the same object")
Comparison of == and is Operators
A. Explanation of the differences between the == and is operators
The main difference between the “==” and “is” operators is that “==” compares the values of two objects, while “is” compares their identities.
B. Comparison of how each operator handles different data types
The “==” operator can be used to compare all data types in Python, while the “is” operator is mainly used to compare objects and check if a variable is None.
C. Comparison of performance between the two operators
The “is” operator is generally faster than the “==” operator because it does not need to compare the values of two objects.
D. Explanation of which operator to use in specific use cases
In general, the “==” operator should be used to compare values, and the “is” operator should be used to check if two variables point to the same object in memory or if a variable is None.
Best Practices for Using the == and is Operators
When using the “==” operator, it is important to consider the type of data being compared. For example, comparing a string to a number using the “==” operator may not give the expected results. In general, it is best to use the “==” operator for comparing simple data types, such as numbers and strings.
When using the “is” operator, it is important to consider the type of data being compared as well. The “is” operator should only be used to compare objects and check if a variable is None. It should not be used to compare simple data types, such as numbers and strings.
Potential pitfalls when using the == and is operators
One potential pitfall of using the “==” operator is that it can lead to unexpected behavior if the data types being compared are not compatible. For example, comparing a string to a number using the “==” operator may not give the expected results.
Another potential pitfall of using the “is” operator is that it can lead to unexpected behavior if you are not careful. For example, if you use the “is” operator to compare two lists with the same values, Python may return “False” because the two lists have different identities in memory.
Tips for debugging issues with the == and is operators
When debugging issues with the “==” operator, it can be helpful to print the values of the variables being compared to make sure they are the expected data types. If the data types are not compatible, you may need to convert one or both of the variables to a compatible data type.
When debugging issues with the “is” operator, it can be helpful to print the memory addresses of the variables being compared to make sure they are the same. If the memory addresses are different, it means the variables do not point to the same object in memory.
Conclusion
A. Recap of the differences between the == and is operators
The “==” and “is” operators are both used to compare objects in Python, but they behave differently. The “==” operator compares the values of two objects, while the “is” operator compares their identities.
B. Final thoughts on the best use cases for each operator
In general, the “==” operator should be used to compare values, and the “is” operator should be used to check if two variables point to the same object in memory or if a variable is None.
C. Importance of understanding the differences between the two operators
Understanding the differences between the “==” and “is” operators is important for writing reliable and bug-free code in Python. By following the best practices outlined in this post, you can avoid common pitfalls and write code that behaves as expected.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is the difference between ‘/’ and ‘/’ in Python?
The ‘/’ and ‘//’ operators both perform division in Python, but they return different results depending on how they are used.
The ‘/’ operator performs normal division and returns a float result. This means that even if the operands are integers, the result will still be a float if the division operation results in a fractional value.
The ‘//’ operator performs integer division, which means it only returns the integer quotient of the division operation. This is useful when you only need the whole number portion of the result, such as when dividing quantities that are always represented in whole numbers (like the number of items in a cart).
What does ‘/’ mean in Python?
In Python, the ‘/’ operator is used to perform division between two numbers. It returns the quotient of the division operation as a float. This means that even if the operands are integers, the result will still be a float if the division operation results in a fractional value.
What is Python and operator?
The ‘and’ operator in Python is a logical operator that checks if both operands are True. If both operands are True, the operator returns True. Otherwise, it returns False. The ‘and’ operator is commonly used in conditional statements and loops to evaluate multiple conditions at once.
What is the difference between ‘/’ and operator?
The ‘/’ operator and ‘and’ operator in Python are completely different operators that serve different purposes. The ‘/’ operator is used for division, while the ‘and’ operator is used for logical operations. The ‘/’ operator returns a numeric result, while the ‘and’ operator returns a boolean result. Additionally, the ‘/’ operator takes two operands and returns one result, while the ‘and’ operator takes two operands and returns one boolean result based on the logical AND operation.
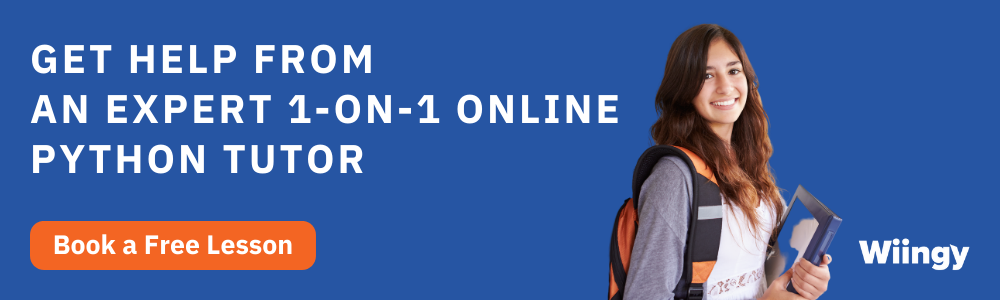
Written by
Rahul LathReviewed by
Arpit Rankwar