Python is a powerful programming language with a lot of operators, working with different kinds of data. Membership and identity operators are two of these operators. These operations are used to compare values and determine whether they are the same object in memory or part of the same group.
In this post, we’ll talk about Python’s membership and identity operators. We will outline the operators, show instances of how they function, and discuss use scenarios.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Membership Operators
Membership operators are used to find out if a value or variable is a part of a sequence. In Python, there are two membership operators: “in” and “not in.”
- The “in” operator: The “in” operator checks if a value or variable is present in a sequence or not. It returns True if the value or variable is found in the sequence, and False otherwise.
Example:
1# Check if 3 is present in the list
2my_list = [1, 2, 3, 4, 5]
3print(3 in my_list) # True
4
5# Check if 'a' is present in the string
6my_string = 'Hello, World!'
7print('a' in my_string) # False
In this example, the first print statement returns True because the value 3 is present in the list “my_list”. The second print statement returns False because the character ‘a’ is not present in the string “my_string”.
- The “not in” operator: The “not in” operator checks if a value or variable is not present in a sequence. It returns True if the value or variable is not found in the sequence, and False otherwise.
Example:
1# Check if 6 is not present in the list
2my_list = [1, 2, 3, 4, 5]
3print(6 not in my_list) # True
4
5# Check if 'z' is not present in the string
6my_string = 'Hello, World!'
7print('z' not in my_string) # True
In this example, the first print statement returns True because the value 6 is not present in the list “my_list”. The second print statement also returns True because the character ‘z’ is not present in the string “my_string”.
Use cases for membership operators: Membership operators are commonly used to check if an element is present in a list or tuple. They are also used to search for characters or substrings in a string. They are particularly useful when you want to check if a certain value or variable is present in a collection before performing an action on it.
Identity Operators
When comparing two objects’ locations in memory, identity operators are employed. In Python, there are two identity operators: “is” and “is not.
- The “is” operator: The “is” operator checks if two objects refer to the same memory location or not. It returns True if both objects refer to the same memory location, and False otherwise.
Example:
1# Compare two strings
2my_string1 = "Hello"
3my_string2 = "Hello"
4print(my_string1 is my_string2) # True
5
6# Compare two lists
7my_list1 = [1, 2, 3]
8my_list2 = [1, 2, 3]
9print(my_list1 is my_list2) # False
In this example, the first print statement returns True because both strings “my_string1” and “my_string2” refer to the same memory location. The second print statement returns False because both lists “my_list1” and “my_list2” refer to different memory locations, even though they contain the same elements.
- The “is not” operator: The “is not” operator checks if two objects do not refer to the same memory location. It returns True if both objects do not refer to the same memory location, and False otherwise.
Example:
1# Compare two strings
2my_string1 = "Hello"
3my_string2 = "World"
4print(my_string1 is not my_string2) # True
5
6# Compare two lists
7my_list1 = [1, 2, 3]
8my_list2 = [1, 2, 3]
9print(my_list1 is not my_list2) # True
In this example, the first print statement returns True because the two strings “my_string1” and “my_string2” refer to different memory locations. The second print statement also returns True because both lists “my_list1” and “my_list2” refer to different memory locations.
Use cases for identity operators: Identity operators are commonly used to compare two objects and check if they refer to the same memory location. They are useful when you want to determine if two objects are actually the same object in memory.
Additional Tips and Best Practices
Common mistakes when using membership and identity operators: One common mistake is using the wrong operator when comparing objects. It is important to understand the difference between equality and identity. The “==” operator is used for checking if two objects have the same value, while the “is” operator is used for checking if two objects are the same object in memory. For example, in the following code snippet:
1a = [1, 2, 3]
2b = [1, 2, 3]
3print(a == b) # Output: True
4print(a is b) # Output: False
The “==” operator returns True because the two lists have the same values. However, the “is” operator returns False because the two lists are different objects in memory.
Another mistake is using membership operators with non-sequence data types like integers or floats. The in and not in operators work with sequences like lists, tuples, and strings, but not with non-sequence data types.
Best practices for using membership and identity operators: It is best to use membership operators with sequence data types like lists, tuples, and strings. When using identity operators, it is important to understand the concept of memory location and how it works in Python.
To improve readability, it is recommended to use parentheses around complex expressions. For example:
1if (value in my_list) and (other_value not in my_tuple):
2 # do something
Additionally, using variable names that are descriptive can also make the code more readable.
Comparison of Membership and Identity Operators
Membership operators check if a value or variable is part of a sequence. Identity operators, on the other hand, compare where two objects are in memory.Identity operators return True or False based on where in memory two objects are located. Membership operators return True or False based on whether or not a value or variable is in a sequence.
When to use identity operators and when to use membership operators: Identity operators are used to compare where in memory two objects are located, while membership operators are used to find out if a value or variable is in a sequence. Membership operators are often used with lists, tuples, and strings, while identity operators are often used with objects and variables.
Use membership operators when checking if a value is in a sequence, and use identity operators when checking if two objects are the same object in memory. For example:
1my_list = [1, 2, 3]
2if 2 in my_list:
3 # do something
4
5a = [1, 2, 3]
6b = a
7if a is b:
8 # do something
In the first example, we use the “in” operator to check if the value 2 is in the list “my_list”. In the second example, we use the “is” operator to check if the variables “a” and “b” are the same object in memory.
Conclusion
In conclusion, membership and identity operators are important tools in Python for comparing objects and checking whether values are in sequences. By understanding the differences between these operators and best practices for using them, you can write more efficient and readable code. So go ahead and use these operators in your Python code!
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is membership and identity operators in Python?
Membership operators in Python are used to check if a particular value or variable is a member of a given sequence or collection. They are used to test the membership of a value in a sequence, such as a list, tuple, or string. On the other hand, identity operators in Python are used to compare the identity of two objects.
Membership operators are used to test if a value is in a sequence, while identity operators are used to test if two variables refer to the same object or not.
What is the difference between identity operator and membership operators?
The identity operators ‘is’ and ‘is not’ are used to check whether two variables refer to the same object or not. The membership operators ‘in’ and ‘not in’ are used to test whether a value is a member of a sequence or collection.
The difference between these two types of operators is in their functionality. Identity operators compare the memory location of two objects, while membership operators test whether a value is present in a sequence or collection. In other words, identity operators compare the identity of objects, while membership operators compare the values contained in objects.
What are the membership operators in Python?
Membership operators are used to test whether a value is a member of a sequence or collection. Python provides two membership operators: ‘in’ and ‘not in’. The ‘in’ operator returns True if a value is present in a sequence, and False otherwise. The ‘not in’ operator returns True if a value is not present in a sequence, and False otherwise.
For example, if we have a list of numbers, we can use the membership operator ‘in’ to check whether a specific number is present in the list or not. Similarly, we can use the ‘not in’ operator to check whether a number is not present in the list.
Which operator is called as membership operator in Python?
The operator that is called the membership operator in Python is the ‘in’ operator. It is used to test whether a value is present in a sequence or collection. It returns True if the value is present in the sequence or collection, and False otherwise.
The ‘in’ operator is often used in conditional statements or loops to check if a value is a member of a sequence. For example, it can be used to check if a certain element exists in a list or a string, or if a key exists in a dictionary.
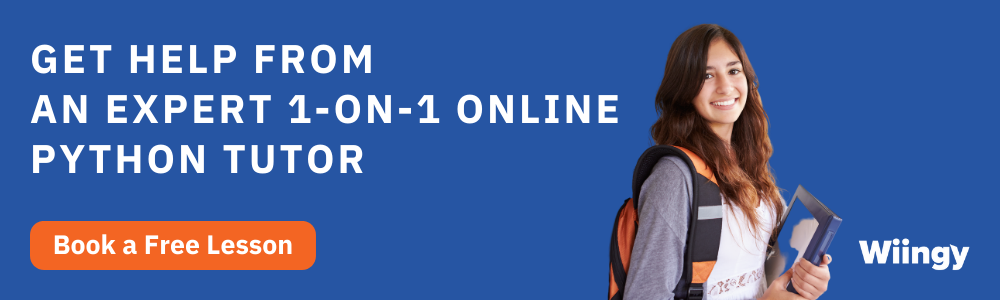
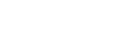
Jan 30, 2025
Was this helpful?