Python Tutorials
What is Output in Python?
The information that a program generates or displays is referred to as “output” in programming. Programming must include outputs because they give users a better understanding of what the program is doing and allow them to comment on the input that has been given.
Python is a popular programming language that is often used for projects like machine learning, building websites, and analyzing data.There are numerous ways to produce and show output in Python. This blog post will give an overview of the various methods available for printing output in Python and discuss some of them.
By the end of this article, students will have a fundamental understanding of how to use Python’s print() function to produce output. Also, some of the many formats and display options for output will be explained to them.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Basic Output in Python
The print() function is used in Python to generate output to the console or screen. It is a built-in function that can be used to output text or variables. To use the print() function, simply type the word print followed by parentheses, like this: print().
To output text using the print() function, you simply need to enclose the text in quotation marks. For example, to output the text “Hello, World!” to the console, you would write:
print("Hello, World!")
To output variables, you simply need to include the variable name inside the parentheses. For example, if you have a variable called name that contains the value “Alice”, you can output the value of the variable like this:
name = "Alice" print("My name is", name)
This will output the value “Alice” to the console.
You can also include multiple variables or text in a single print statement by using commas to separate them. For example:
name = "John"
age = 12
print("My name is", name, "and I am", age, "years old.")
This will output the text “My name is John and I am 12 years old.” to the console.
By default, the print() function adds a newline character at the end of the output, which means that each print statement starts on a new line. If you want to output text without adding a newline character, you can use the end parameter like this:
print("Hello", end="") print(", World!")
This will output the text “Hello, World!” on a single line.
In summary, the print() function is a simple and powerful way to generate output in Python. You can use it to output text or variables, and you can format the output in a variety of ways.
Formatting Output in Python
You can format your output in Python in addition to using the print() function by using string formatting techniques. One of the most popular methods is using the str.format() method. The str.format() method allows you to insert values into a string by using placeholders. Here’s an example:
name = "Alice" age = 12 print("My name is {} and I am {} years old".format(name, age))
This will print “My name is Alice and I am 12 years old” to the screen. The curly brackets {} are placeholders for the values that you want to insert into the string.
You can also specify the position of the values in the string by using numbers inside the curly brackets. For example:
name = "Alice" age = 12 print("My name is {1} and I am {0} years old".format(age, name))
This will print “My name is Alice and I am 12 years old” to the screen, just like the previous example.
You can also specify the alignment of the values in the string by using < for left alignment, ^ for center alignment, and > for right alignment. For example:
name = "Alice" age = 12 print("My name is {:<10} and I am {:>3} years old".format(name, age))
This will print “My name is Alice and I am 12 years old” to the screen.
Finally, you can specify the sign of a number by using + for positive numbers and – for negative numbers. For example:
x = 12 y = -12 print("x = {:+} and y = {:+}".format(x, y))
This will print “x = +12 and y = -12” to the screen.
These are just a few examples of how you can format your output in Python. By using these techniques, you can make your program’s output look neat, organized, and easy to understand.
Advanced Output Control in Python
In Python, you can use control sequences to modify the formatting and appearance of output. Control sequences are special sequences of characters that are interpreted by the console or terminal.
Here are some examples of control sequences and their purposes:
- \n: This control sequence adds a newline character, which starts a new line of output.
- \t: This control sequence adds a tab character, which indents the text by a certain number of spaces.
- \b: This control sequence adds a backspace character, which moves the cursor back one position.
- \r: This control sequence adds a carriage return character, which moves the cursor to the beginning of the current line.
You can use these control sequences in your print statements to format output in a variety of ways. For example:
print("This is a sentence.\nThis is a new line.")
This will output the text “This is a sentence.” on one line and “This is a new line.” on the next line.
In addition to control sequences, you can also use special characters to modify the appearance of output. For example, you can use the ASCII escape character \033[ to add color to your output:
print("\033[1;31;40mRed text on black background!\033[0m")
This will output the text “Red text on black background!” in red text on a black background.
In summary, control sequences and special characters provide powerful tools for formatting and customizing output in Python. By using these techniques, you can make your output more readable and visually appealing.
Output to Files in Python
In addition to printing output to the console, you can also save output to a file in Python. This can be useful for logging data, generating reports, or creating backups.
To write output to a file, you need to use the open() function to open a file in write mode. The open() function takes two arguments: the filename and the mode. There are several modes you can use, including:
- “w”: write mode, which overwrites the file if it already exists or creates a new file if it does not exist
- “a”: append mode, which appends new data to the end of the file
- “x”: exclusive mode, which creates a new file and raises an error if the file already exists
Once you have opened a file, you can use the write() method to write data to the file. For example:
with open("output.txt", "w") as file:
file.write("Hello, world!")
This code will create a new file called output.txt in write mode, write the text “Hello, world!” to the file, and then close the file. Note that we are using a with statement to automatically close the file when we are done with it.
Conclusion
In this blog post, we have covered the different ways to output in Python. We started by discussing the basics of using the print() function to output text and variables to the console. We then moved on to more advanced techniques, such as using control sequences to modify the formatting and appearance of output. Finally, we discussed how to save output to a file using the open() function and the different modes available.
It is important to understand and control output in programming, as it can greatly affect the readability and usability of your code. By using the techniques we have discussed in this blog post, you can make your output more informative and visually appealing.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is output formatting?
Output formatting refers to the rules and conventions used to display the results of a program or process. It involves specifying the size, shape, color, and other properties of text, images, or other visual elements that will be output to a screen, printer, or other device. Proper output formatting can help to improve the usability and readability of information and make it easier to comprehend.
What is an example of output format?
An example of output format is a table that displays data in rows and columns. For instance, in a spreadsheet, the data can be organized into cells, and the cells can be formatted with different fonts, colors, and styles to make the data more visually appealing and readable.
What is meant by output formatting in Python?
Output formatting in Python refers to the way in which data is displayed on the screen or in a file. This can include manipulating the size, shape, color, and other properties of text and visual elements to make the data more visually appealing and easier to understand. Python provides various tools and libraries for output formatting, including built-in functions like print() and more advanced options like string formatting and regular expressions.
What is input and output format?
Input and output format is a term used in computer science and information technology to describe the structure and organization of data that is entered into or extracted from a system. Input format can include various types of data, such as text, numbers, images, and multimedia files, and can be entered through different input devices, such as a keyboard, mouse, or microphone. Output format refers to the way in which data is presented to the user, such as in a text file, spreadsheet, graphic, or audiovisual format. Proper input and output formatting are essential for ensuring that data is accurately processed and communicated between different systems and applications.
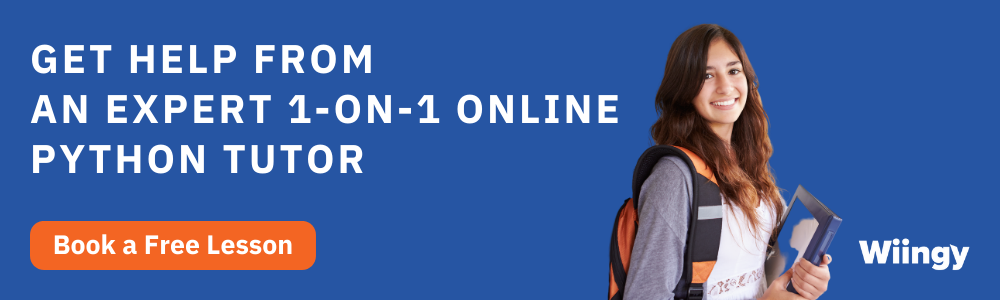
Written by
Rahul LathReviewed by
Arpit Rankwar