What is a Destructor in Python and Why is it Important?
A destructor in Python is a special method that is automatically invoked when an object is no longer required or when the program is about to exit. The destructor is responsible for freeing any allocated resources that the object used during its lifetime.
The destructor method is essential because it helps manage memory and other program resources. It ensures that memory occupied by an object is released when it is no longer required, thereby preventing memory leaks and other issues. In essence, destructors aid in optimizing program performance and preventing problems caused by improper resource management.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
How Destructors in Python work
The Python garbage collector automatically calls an object’s destructor when it is no longer required. Python has a garbage collection mechanism that scans the program’s memory periodically to determine which objects are no longer in use.
A reference counting mechanism is used by the garbage collector to determine when an object is no longer required. Every Python object has a reference count that indicates the number of variables that refer to it. When an object’s reference count reaches zero, the garbage collector invokes its destructor to free any resources it allocated during its lifetime.
Python’s garbage collector is optimized for memory management efficiency and can handle circular references, which occur when two or more objects share a common reference. Using a mark-and-sweep algorithm, the garbage collector identifies and eliminates circular references, ensuring that no memory is wasted.
How to Define a Destructor in Python
In Python, a destructor is defined using a special method called del(). The syntax for defining a destructor is as follows:
1class MyClass:
2 def __init__(self):
3 # constructor code here
4
5 def __del__(self):
6 # destructor code here
In the above example, the constructor is defined using the init() method, while the destructor is defined using the del() method.
Here’s an example of a destructor in Python:
1class File:
2 def __init__(self, name):
3 self.name = name
4 self.file = open(name, 'w')
5
6 def __del__(self):
7 self.file.close()
In the above example, the File class represents a file object. The constructor opens a file with the given name and stores a reference to it in the object’s file attribute. The destructor closes the file when the object is no longer needed.
Python provides the del() method in addition to the del() method for deleting objects. The del() method can be used to delete objects explicitly, which can be useful in situations where greater resource management control is required. The majority of the time, however, Python’s built-in garbage collector and destructor mechanism is adequate for managing resources efficiently.
How to Use Destructors in Python
- By releasing resources when they are no longer required, destructors facilitate memory management and prevent memory leaks.
- When an object is destroyed, they enable the execution of cleanup code, such as closing a file or releasing a network connection.
- Destructors can be utilized to remove temporary files or objects that are no longer required, thereby enhancing the performance of the program.
Example use cases for destructors:
- When file handles or database connections are no longer required, they are closed.
- Eliminating temporary files and directories that were created during program execution.
- Releasing system resources, including network connections and memory allocations.
- Implementing customized resource management for classes requiring it.
Best practices for using destructors in Python:
- Always explicitly release resources in the destructor, such as closing file handles or freeing memory.
- Avoid creating circular references between objects, as this can impede the garbage collector’s ability to properly free up memory.
- If an object is not properly destroyed, relying solely on its destructor to manage its resources can lead to complications.
When to Use Destructors in Python
- Destructors should be used when an object’s destruction requires the cleanup of resources, such as closing file handles or releasing memory.
- They are especially helpful for classes that manage system resources or execute I/O operations, such as file or network connections.
Situations where destructors are useful:
- When a class must manage system resources or perform I/O operations, including file and network connections.
- When a class is used to represent a temporary object that is no longer required after a certain point in the program, the object is said to be disposable.
Alternatives to using destructors:
- Python provides additional resource management mechanisms, such as the with statement, which can be used to clean up resources automatically when they are no longer required.
- Additionally, context managers can be used to manage resources explicitly, without relying on the destructor.
Destructor Pitfalls and Common Mistakes
- Failing to properly release resources in the destructor, such as failing to close a file handle or release memory.
- Circular references between objects can prevent the garbage collector from releasing memory correctly.
- Depending solely on the destructor to manage resources, which can cause problems if the object is not properly destroyed.
Pitfalls to be aware of when using destructors:
- Destructors can be unpredictable, as they are not always called immediately when an object is destroyed.
- They may not be called at all if the program exits abruptly or if an error occurs.
- Circular references between objects can prevent the garbage collector from freeing memory properly, leading to memory leaks and other issues.
How to avoid common mistakes when using destructors:
- Avoid relying solely on the garbage collector to manage resources and release resources explicitly in the destructor.
- Use weak references or redesign the class hierarchy to avoid creating circular references between objects.
- Utilize alternative resource management mechanisms, such as the with statement or context managers, when applicable.
Advantages of Using Destructors in Python
- By releasing resources when they are no longer required, destroyors aid in effective memory management and stop memory leaks.
- When an object is destroyed, they offer a way to run cleanup code, such as closing a file or cutting off a network connection.
- To enhance program performance, destructors can be used to remove temporary files or objects that are no longer required.
- By automating the cleanup procedure, they streamline the management of system resources and make programming simpler.
.
Here’s an example that demonstrates how destructors can make programming easier and more efficient:
1class DatabaseConnection:
2 def __init__(self, host, port):
3 self.host = host
4 self.port = port
5 self.connection = open_connection(host, port)
6
7 def __del__(self):
8 self.connection.close()
9
10 def execute(self, query):
11 # execute database query here
In the above example, the DatabaseConnection class manages a database connection and automatically closes it when the object is destroyed. This simplifies the management of database connections and ensures that resources are released properly, even in the event of an error.
Conclusion
In this article, we’ve discussed destructors in Python and their importance for efficient memory management and resource cleanup. We’ve seen how destructors work in Python and how to define them in our classes. We’ve also discussed the benefits of using destructors, including how they can simplify programming and improve program performance.
It’s important to use destructors correctly in Python and avoid common pitfalls, such as circular references and improper resource management. By following best practices and using alternative resource management mechanisms when appropriate, we can ensure that our programs are efficient and free from memory leaks and other related issues.
In conclusion, destructors are an important tool in Python programming, and their proper use can lead to more efficient and effective programs.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are the destructors in Python?
When an object is no longer required, Python’s destructors are special methods that are automatically invoked. Their function is to release any resources that the object had reserved during its lifetime.
How do you write a destructor in Python?
A destructor is defined in Python using a unique method called del(). The syntax for defining a destructor is as follows:
1class MyClass: def __init__(self): # constructor code here def __del__(self): # destructor code here
What is destructor and its types?
In object-oriented programming languages, a destructor is a unique method that is automatically called when an object is no longer required. There are two types of destructors:
Implicit destructors: These are destructors that are called automatically by the language runtime when an object is no longer needed. In Python, the del() method is an implicit destructor.
Explicit destructors: These are destructors that are called explicitly by the program to release resources or perform cleanup tasks. In Python, the del() method is an example of an explicit destructor.
What is destructor and constructor in Python?
In Python, a constructor is a special method that is called when an object is created, and its purpose is to initialize the object’s attributes. A destructor, on the other hand, is a special method that is called when an object is no longer needed, and its purpose is to release any resources that were allocated by the object during its lifetime.
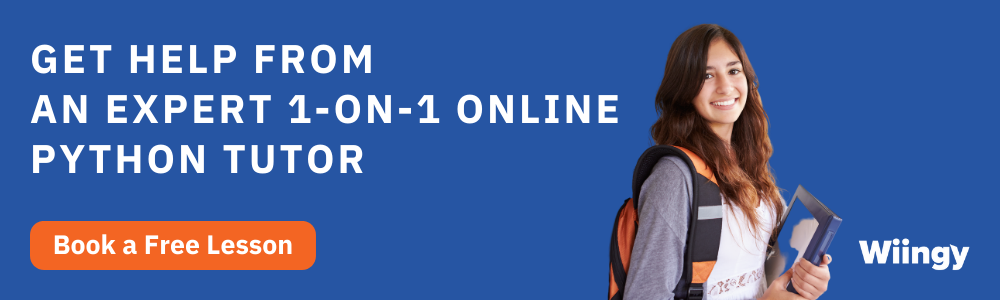
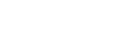
Jan 30, 2025
Was this helpful?