Python Tutorials
What are First Class Functions in Python?
Python’s capability to handle functions as first-class objects is one of its key characteristics. In this article, we’ll talk about Python’s first-class functions and how to use them in programming.
Functions are first-class objects in the Python programming language, which means they can be used in the same ways as other objects. Assigning functions to variables, passing them as arguments to other functions, and returning functions as values all fall under this category. First-class functions are those that can handle functions in this manner.
Understanding first-class functions is crucial for programming in Python. It enables programmers to create more effective, modular, and reusable code. Additionally, Python is a necessity for any developer who wants to work with these tools because so many libraries and frameworks use it.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
First-Class Functions Explained
A. Definition of First-Class Functions
In Python, the term “first-class function” refers to a function’s ability to be treated as an object that can be assigned to a variable, used as an argument for other functions, and returned as a value. As a result, functions in Python are identical to other objects like strings, integers, and lists.
B. Properties of First-Class Functions
Three essential characteristics of Python first-class functions are as follows:
Functions can be assigned to variables: Python allows you to assign functions to variables just like you would any other object. This allows for easy manipulation and reuse of functions.
Example:
def square(x):
return x ** 2
my_func = square
print(my_func(3)) # Output: 9
Functions can be passed as arguments to other functions: Functions can be passed as arguments to other functions. This is helpful for writing more modular, reusable code as well as higher-order functions.
Example:
def apply_operation(func, x):
return func(x)
def square(x):
return x ** 2
print(apply_operation(square, 3)) # Output: 9
Functions can also return values from other functions, which is another way that functions can return values. This is useful for returning functions based on specific criteria or for creating functions on the fly.
Example:
def get_operation(op):
if op == '+':
def add(x, y):
return x + y
return add
elif op == '-':
def subtract(x, y):
return x - y
return subtract
add_func = get_operation('+')
subtract_func = get_operation('-')
print(add_func(3, 4)) # Output: 7
print(subtract_func(10, 5)) # Output: 5
C. Examples of First-Class Functions in Python
Assigning a Function to a Variable:
def cube(x):
return x ** 3
my_func = cube
print(my_func(2)) # Output: 8
Passing a Function as an Argument:
def apply_operation(func, x):
return func(x)
def cube(x):
return x ** 3
print(apply_operation(cube, 3)) # Output: 27
Example of Returning a Function as a Value:
def get_operation(op):
if op == '+':
def add(x, y):
return x + y
return add
elif op == '-':
def subtract(x, y):
return x - y
return subtract
add_func = get_operation('+')
subtract_func = get_operation('-')
print(add_func(3, 4)) # Output: 7
print(subtract_func(10, 5)) # Output: 5
In the above example, get_operation is a function that takes an operator as an argument and returns a function based on the operator. If the operator is +, the function returns an add function that takes two arguments and returns their sum. Similarly, if the operator is -, the function returns a subtract function that takes two arguments and returns their difference.
We then assign the returned function to variables add_func and subtract_func, respectively, and use them to perform addition and subtraction.
Higher-Order Functions
A. Definition of Higher-Order Functions
Higher-order functions are those that accept arguments from other functions or return values that are themselves other functions. The idea of first-class functions in Python makes higher-order functions possible.
B. How Higher-Order Functions Work
Higher-order functions operate by accepting a function as an argument, altering it, and then returning the altered function. More modular and reusable code can be produced as a result.
C. Examples of Higher-Order Functions in Python
A Function That Takes a Function as an Argument:
def apply_func(func, lst):
return [func(x) for x in lst]
def square(x):
return x ** 2
numbers = [1, 2, 3, 4, 5]
squared_numbers = apply_func(square, numbers)
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
In the above example, apply_func is a higher-order function that takes a function (square) and a list of numbers (numbers) as arguments. It then applies the square function to each number in the list and returns a new list with the squared numbers.
A Function That Returns a Function as a Value:
def make_adder(n):
def adder(x):
return x + n
return adder
add_five = make_adder(5)
print(add_five(10)) # Output: 15
In the above example, make_adder is a higher-order function that takes a number n and returns a new function adder that adds n to its argument. We then assign the returned function to a variable add_five, which adds 5 to any number passed to it.
Built-in Higher-Order Functions in Python
A. Map, Filter, and Reduce
Python provides several built-in higher-order functions, including map(), filter(), and reduce(). These functions are commonly used in Python programming and can be used to process data in a more efficient and concise way.
map() applies a function to each element of an iterable and returns an iterator of the results. filter() selects elements from an iterable that satisfy a given condition and returns an iterator of those elements. reduce() applies a function to the first two elements of an iterable, then applies the function to the result and the next element, and so on until all elements have been processed.
B. Examples of Using Built-in Higher-Order Functions
Example of Using map() to Apply a Function to Each Element in a List:
def square(x):
return x ** 2
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(square, numbers))
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
In the above example, we define a function square that squares a number. We then use map() to apply the square function to each number in the list numbers and return a new list with the squared numbers.
Example of Using filter() to Select Elements from a List That Satisfy a Condition:
def is_even(x):
return x % 2 == 0
numbers = [1, 2, 3, 4, 5]
even_numbers = list(filter(is_even, numbers))
print(even_numbers) # Output: [2, 4]
In the above example, we define a function is_even that checks if a number is even. We then use filter() to select all even numbers from the list numbers and return a new list with those numbers.
Example of Using reduce() to Combine All Elements in a List Into a Single Value:
from functools import reduce
def add(x, y):
return x + y
numbers = [1, 2, 3, 4, 5]
sum_of_numbers = reduce(add, numbers)
print(sum_of_numbers) # Output: 15
In the above example, we define a function add that adds two numbers. We then use reduce() to apply the add function to the first two numbers in the list numbers, then apply it to the result and the next number, and so on until all numbers have been processed. The final result is the sum of all numbers in the list.
Advantages of First-Class Functions
A. Code Reusability
First-class functions make it simpler to reuse code. It is simpler to write code that can be reused in various areas of a program because functions can be assigned to variables and passed as arguments to other functions.
B. Modular Programming
First-class functions also allow for modular programming, which divides a program into smaller, easier-to-manage modules. Each module, which can be reused in various parts of the program, can be created to handle a particular task or set of tasks.
C. Code Conciseness
First-class functions can contribute to cleaner, simpler, and easier to read code. They make it possible to express complicated operations in a clearer and more readable way.
Best Practices for using First Class Functions in Python
There are a few best practices to adhere to when using first-class functions in Python to make sure the code is efficient, readable, and maintainable.
A. Proper Usage of First-Class Functions
It is best to use first-class functions in a way that makes sense for the program that is being created.They should not be used improperly or without due consideration; rather, they should be used to increase the modularity, reuse, and conciseness of the code. It is also important to understand the advantages and limitations of first-class functions and to use them in a way that aligns with best practices and coding standards.
B. Maintaining Code Readability
It is vital to maintain code readability when employing first-class functions. This can be accomplished by utilizing descriptive function names, commenting code as needed, and formatting code consistently. In addition, it is essential to use variables and function names that accurately describe their function and to avoid variables with ambiguous names.
C. Pitfalls to Avoid
There are a few pitfalls to watch out for when using first-class functions. One common mistake is using first-class features carelessly or inappropriately. Overly complicated functions that are challenging to read and maintain are another pitfall. Additionally, it’s crucial to stay away from functions with side effects because they can cause unpredictable behavior and make debugging code more challenging.
Overall, developers can use Python’s first-class functions to create more effective, modular, and maintainable code by adhering to best practices and avoiding pitfalls.
Conclusion
In this article, we talked about Python’s first-class functions and how crucial they are to programming. In our explanation, we covered the characteristics of first-class functions, such as their capacity to assign values to variables, accept arguments, and return results. Additionally, we covered higher-order functions and gave examples of Python’s pre-built higher-order functions. Finally, we discussed the benefits of first-class functions in programming and offered best practices for their appropriate application.
For the purpose of creating modular, effective, and reusable code, first-class functions must be used properly. When used improperly or without due consideration, they can produce difficult-to-maintain and -debug code. First-class functions should be used in a way that makes sense for the program being developed, while also being aware of their benefits and limitations.
Python’s first-class functions are a potent tool that can be used to create code that is more effective, modular, and reusable. We can anticipate seeing even more sophisticated applications of first-class functions and higher-order functions as Python continues to develop. It’s crucial to keep up with these advancements as well as to keep discovering and using Python’s capabilities.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is a first-class function in Python?
A Python first-class function is one that can be used to assign values to variables, pass arguments to other functions, and return results.
What are functions as first class?
First-class functions in Python are those that can be treated as objects and modified in the same way as other objects. Assigning functions to variables, passing them as arguments, and returning functions as values all fall under this category.
Why are functions first class Python?
Python’s support for functional programming makes functions first-class objects. Python makes it possible for programmers to write more effective, modular, and reusable code by treating functions as first-class objects.
What are first-class types in Python?
In Python, first-class objects also include integers, strings, and lists in addition to functions. Similar to functions, these objects can also be assigned to variables, passed as arguments, and returned as values.
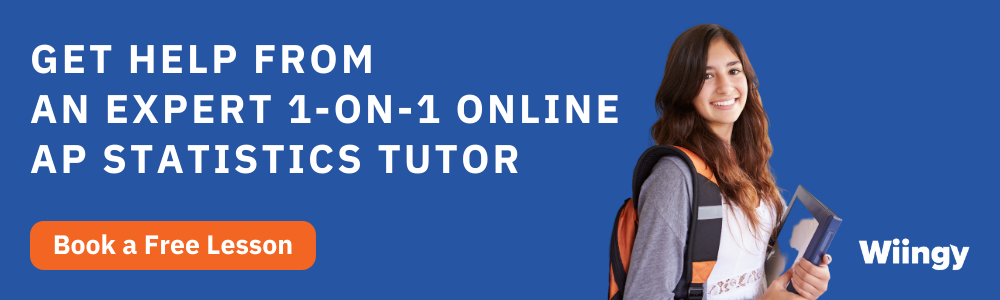
Written by by
Rahul LathReviewed by by
Arpit Rankwar