What are Constructors in Python?
When an object of a class is created in Python, a constructor is a special kind of method that is automatically called. Instance variables of a class are initialized using constructors. They are defined by using the init() method and share the same name as the class.
An important concept in object-oriented programming, constructors are crucial for beginners. They enable programmers to produce objects of a class with their attributes set to initial values. By employing constructors, we can guarantee that the object is created in a usable state and prevent any potential undefined behavior.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Types of Constructors in Python
In Python, there are three different kinds of constructors:
Default Constructor: This is the simplest constructor type and does not accept any parameters. Python automatically creates a default constructor when a class does not have one defined explicitly. All of the class’ instance variables are initialized to their default values by the default constructor.
Non-parameterized Constructor: This kind of constructor is explicitly defined by the programmer but does not accept any parameters. It is also known as the constructor without arguments. The instance variable values are initialized to some predetermined default values in this kind of constructor.
Parameterized Constructor: This type of constructor accepts one or more parameters and initializes the instance variables of the class with the values passed as arguments. It is also called the argument constructor.
Non-Parameterized Constructor
A non-parameterized constructor is one which does not accept parameters. It is used to set some default values for the instance variables of a class and is explicitly defined by the programmer.
Example:
1class Person:
2 def __init__(self):
3 self.name = "John"
4 self.age = 20
5
6p = Person()
7print("Name:", p.name)
8print("Age:", p.age)
9Output:
10Name: John
11Age: 20
In the above example, we have defined a non-parameterized constructor for the Person class. It initializes the name and age attributes of the object to “John” and 20, respectively. When we create an object of the Person class using the constructor, it sets the name and age attributes to their default values.
Benefits of using non-parameterized constructor:
- Easy to use: Non-parameterized constructors are easy to use and do not require any arguments to be passed while creating an object.
- Default values: Non-parameterized constructors provide default values to the instance variables of a class, which can be helpful in situations where we do not have any specific values to initialize the variables with.
- Code optimization: Using non-parameterized constructors can optimize the code by reducing the number of parameters that need to be passed while creating an object.
Default Constructor
A default constructor is a constructor that takes no arguments and is defined implicitly by Python if no other constructor is defined. It initializes all the instance variables of a class to their default values. The default constructor is called automatically when an object of the class is created.
Example:
1class Student:
2 def __init__(self):
3 self.name = "John Doe"
4 self.age = 18
5 self.grade = "A"
6
7s = Student()
8print("Name:", s.name)
9print("Age:", s.age)
10print("Grade:", s.grade)
11Output:
12Name: John Doe
13Age: 18
14Grade: A
In the above example, we have defined a default constructor for the Student class. The default constructor initializes the name, age, and grade attributes of the object to their default values. When we create an object of the Student class using the default constructor, it sets the name, age, and grade attributes to their default values.
Benefits of using default constructor:
- Easy to use: Default constructors are easy to use and do not require any arguments to be passed while creating an object.
- Code optimization: Using default constructors can optimize the code by reducing the number of parameters that need to be passed while creating an object.
- Consistency: Using default constructors can ensure consistency across all objects of a class by initializing them to their default values.
Parameterized Constructor
An instance variable of a class is initialized with the values passed as arguments by a parameterized constructor, which accepts one or more arguments. It enables programmers to create objects of a class with attributes that have unique values set.
Example:
1class Rectangle:
2 def __init__(self, length, breadth):
3 self.length = length
4 self.breadth = breadth
5
6r = Rectangle(10, 5)
7print("Length:", r.length)
8print("Breadth:", r.breadth)
9Output:
10Length: 10
11Breadth: 5
In the above example, we have defined a parameterized constructor for the Rectangle class. The parameterized constructor takes two arguments, length and breadth, and initializes the length and breadth attributes of the object with the values passed as arguments. When we create an object of the Rectangle class using the parameterized constructor, it sets the length and breadth attributes to the values passed as arguments.
Benefits of using parameterized constructor:
- Customization: When creating objects of a class, parameterized constructors let programmers alter the values of instance variables.
- Flexibility: By allowing objects of the class to be created with different values assigned to their attributes, parameterized constructors can increase a class’s flexibility.
- Reusability: By allowing objects of the class to be created with different values assigned to their attributes, parameterized constructors can increase a class’s reusability.
Understanding Self Parameter in Constructors
A reference to the object being created is provided by the self parameter in constructors. It is a unique parameter that can be used to access the class’s instance variables and methods. When an object of the class is created, the self parameter, which is always the first parameter of a constructor, is automatically passed.
Example:
1class Employee:
2 def __init__(self, name, age, salary):
3 self.name = name
4 self.age = age
5 self.salary = salary
6
7e = Employee("John Doe", 25, 50000)
8print("Name:", e.name)
9print("Age:", e.age)
10print("Salary:", e.salary)
11Output:
12Name: John Doe
13Age: 25
14Salary: 50000
In the above example, we have defined a parameterized constructor for the Employee class, which takes three arguments, name, age, and salary, and initializes the instance variables of the class with the values passed as arguments. The self parameter is used to access the instance variables and set their values.
Explanation of how self parameter works in constructors:
When we create an object of a class, Python automatically passes the reference to that object as the first parameter to the constructor. This parameter is named self by convention, but it can be named anything else as well. The self parameter is used to access the instance variables and methods of the class within the constructor.
Example of self parameter in Python constructors:
1class Car:
2 def __init__(self, make, model, year):
3 self.make = make
4 self.model = model
5 self.year = year
6
7 def display_car_details(self):
8 print("Make:", self.make)
9 print("Model:", self.model)
10 print("Year:", self.year)
11
12c = Car("Honda", "Civic", 2020)
13c.display_car_details()
14Output:
15Make: Honda
16Model: Civic
17Year: 2020
In the example above, a Car class has been created with a parameterized constructor that accepts the three arguments make, model, and year and initializes the class’ instance variables with the values supplied. The display_car_details() method, which is invoked using the class object, is used to show the car’s details. In the method, the self parameter is used to access the class’ instance variables.
Initialization and Destructors
Initialization is the process of setting some default values for a class’s instance variables. Python constructors are used for this. Destructors, on the other hand, are procedures that are automatically called when an object is destroyed or garbage-collected. The del() method is used to define the destructor method in Python.
Explanation of how initialization and destructors work in Python:
The constructor of a class is automatically invoked when an object of that class is created in order to initialize the class’ instance variables. Similar to this, the destructor is automatically called when an object is destroyed or garbage collected to release the resources used by the object. When an object is no longer in use, Python automatically calls the destructor rather than the programmer explicitly doing so.
Example of initialization and destructors in Python:
1class Book:
2 def __init__(self, title, author):
3 self.title = title
4 self.author = author
5 print("Book Initialized")
6
7 def __del__(self):
8 print("Book Destroyed")
9
10b = Book("Python for Beginners", "John Doe")
11del b
12Output:
13Book Initialized
14Book Destroyed
In the example above, we defined a class called Book with a constructor that accepts the parameters title and author as well as initializes the class’ instance variables with those values. To release the resources used by the object, the del() method serves as a destructor. The constructor is automatically called when an object of the Book class is created, and the destructor is automatically called when an object is deleted using the del keyword.
Advantages of Using Constructors
The advantages of constructors in Python programming include:
- Code optimization: By lowering the number of parameters that must be passed when creating an object, constructors can improve the code.
- Consistency: By initializing all class objects to their default values using constructors, consistency can be guaranteed across all of the objects in the class.
- Customization: When creating objects of a class, parameterized constructors let programmers alter the values of instance variables.
- Flexibility: By allowing objects of the class to be created with different values assigned to their attributes, constructors can increase a class’s flexibility.
How constructors simplify and speed up programming:
Constructors in Python offer a way to create objects of a class with initial values set to its attributes, which can make programming simpler and more effective. They enable programmers to guarantee that the object is created in a valid state and prevent any undefined behavior that might otherwise happen. By placing the initialization logic in a separate method, constructors also improve the code’s readability and maintainability.
Learning constructors is just one step in your coding journey. For students who want to go beyond tutorials and get hands-on guidance, Wiingy offers personalized tutoring across multiple programming topics. You can connect with online coding tutors for help in Python fundamentals or explore online computer science tutoring for deeper conceptual clarity. If you’re focusing on specific languages, there are dedicated options like java tutoring online and python lessons online to suit your learning goals and projects.
Conclusion
In this article, we covered Python constructors and their significance in programming, particularly for newcomers. Additionally, we discussed the various Python constructor types and described how they operate, including default, non-parameterized, and parameterized constructors.
We also went over the idea of the self parameter in constructors and how it functions. Finally, we talked about the advantages of using constructors in Python programming and how they facilitate and improve programming. Understanding constructors is essential for becoming a proficient Python programmer because they are a fundamental idea in object-oriented programming.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are constructors in Python?
When an object of a class is created in Python, a special method called a constructor is automatically called. They share the same name as the class name and are used to initialize the instance variables of a class.
What is init constructor in Python?
Python has a unique method called init that serves as a class’ constructor. It is used to initialize the class’ instance variables and is called automatically whenever an object of a given class is created.
What is the use of constructor in OOP Python?
In OOP Python, the constructor is used to initialize class instance variables and make sure the object is created in a usable state. It is a fundamental idea in object-oriented programming and enables developers to construct objects that belong to a class with their attributes set to initial values.
What is constructor vs init in Python?
In Python, the words “constructor” and “init” have identical definitions. A class’s constructor, the init method, is automatically called whenever an object belonging to that class is created.
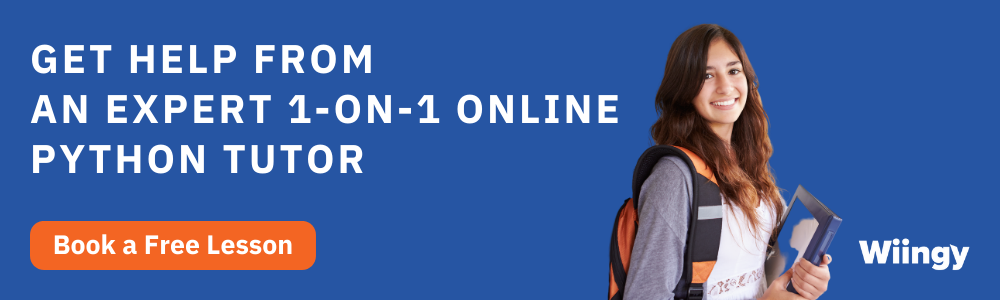
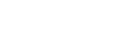
Apr 15, 2025
Was this helpful?