In this article
What are Python Keywords?
List of Python Keywords and Their Functions
Python Keywords in Object-Oriented Programming
Advanced Techniques for Using Python Keywords
Python Keywords vs Identifiers: What’s the Difference?
Best Practices for Optimizing Code with Python Keywords
Common Mistakes with Python Keywords and How to Avoid Them
Future of Python Keywords: New Additions and Changes
Best Practices for Working with Python Keywords in Large Projects
Conclusion
FAQs
What are Python Keywords?
In Python, reserved words with a particular meaning and function are known as “keywords.” As these keywords are already specified by the language, they cannot be used as variable names or identifiers. Keywords in python are case-sensitive, which means that they must be typed with the appropriate case for them to work correctly.
There are 35 keywords in Python 3.10:
1import keyword
2print(keyword.kwlist)
3['False', 'None', 'True', 'and', 'as',
4'assert', 'async', 'await', 'break',
5'class', 'continue', 'def', 'del',
6'elif', 'else', 'except', 'finally',
7'for', 'from', 'global', 'if', 'import',
8'in', 'is', 'lambda', 'nonlocal', 'not',
9'or', 'pass', 'raise', 'return', 'try',
10'while', 'with', 'yield']
Importance of Python Keywords
Python keywords are used to define data types, control flow, and functions. It would be challenging to create effective and efficient Python programs without keywords.
For example, the if and else keywords are used to define conditional statements, allowing the programmer to control the flow of the program based on certain conditions. The def keyword is used to define functions, which are reusable blocks of code that can be called multiple times throughout the program. The while and for keywords are used to create loops, which allow the program to perform repetitive tasks with ease.
Python’s keywords are utilized in almost every element of the language, so knowing them is crucial for any Python programmer. You may build Python code that is more productive and efficient by being an expert keyword user.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
List of Python Keywords and Their Functions
Comprehensive list of all Python keywords
- False – a boolean value representing false
- None – a null value
- True – a boolean value representing true
- and – a logical operator used to combine two conditions, both of which must be true for the overall condition to be true
- as – used to create an alias for a module or object
- assert – used to test a condition and raise an exception if the condition is not met
- async – used to define asynchronous functions and coroutines
- await – used to suspend an asynchronous function until a coroutine has completed
- break – used to exit a loop prematurely
- class – used to define a new class
- continue – used to skip over the rest of a loop iteration and move to the next iteration
- def – used to define a new function
- del – used to delete a variable, list item, or dictionary item
- elif – used to add additional conditions to an if statement
- else – used to define what should happen if a condition is not met in an if statement
- except – used to handle exceptions that occur in a try/except block
- finally – used to define code that should be executed regardless of whether an exception was raised in a try/except block
- for – used to iterate over a sequence
- from – used to import specific attributes or functions from a module
- global – used to modify a variable outside of the current scope
- if – used to test a condition
- import – used to import a module
- in – used to test if a value is in a sequence
- is – used to test if two objects are the same object
- lambda – used to define anonymous functions
- nonlocal – used to modify a variable in the nearest enclosing scope
- not – a logical operator used to negate a condition
- or – a logical operator used to combine two conditions, one of which must be true for the overall condition to be true
- pass – a null operation, used as a placeholder when no action is required
- raise – used to raise an exception
- return – used to return a value from a function
- try – used to define a block of code to be tested for exceptions
- while – used to repeat a block of code while a condition is true
- with – used to define a block of code to be executed in the context of a particular object
- yield – used to return a generator object
Python Keywords in Object-Oriented Programming
Python keywords are an important part of object-oriented programming (OOP). They provide a set of tools and functions that help programmers write code that is complex, modular, and reusable. In this section, we’ll examine some of the fundamental OOP ideas that depend on Python keywords and give real-world examples of their use.
Important OOP Ideas Using Python Keywords
- The “class” keyword in Python lets you create a new class, which acts as a template for building objects.The class’s attributes and methods describe how the objects they represent look and behave.
- The basic idea behind inheritance in OOP is that one class can use the methods and properties of another class.The terms “super” and “subclass” are used to implement inheritance in Python.
- Polymorphism is the ability of objects from different classes to be used in the same way.The “polymorphic” keyword in Python is used to implement polymorphism.
- Encapsulation is the process of hiding the inside details of an object and only letting the outside world know what it needs to know. Encapsulation in Python is accomplished by using the private and public keywords.
Examples of How Python Keywords are Used in OOP
Let’s look at some examples of how Python keywords are used in object-oriented programming.
Example 1: Defining a Class
1class Person:
2 def __init__(self, name, age):
3 self.name = name
4 self.age = age
5 def introduce(self):
6 print("Hi, my name is", self.name, "and I am", self.age, "years old.")
7person1 = Person("Alice", 25)
8person1.introduce()
In this example, we define a class called ‘Person’ using the ‘class’ keyword. The class has two attributes, ‘name’ and ‘age’, and one method, ‘introduce’. We create an instance of the class using the constructor method ‘init‘ and assign it to the variable ‘person1‘. We then call the ‘introduce’ method on the ‘person1’ object, which prints out a greeting message.
Example 2: Inheritance
1class Student(Person):
2 def __init__(self, name, age, major):
3 super().__init__(name, age)
4 self.major = major
5 def introduce(self):
6 super().introduce()
7 print("I am majoring in", self.major)
8student1 = Student("Bob", 20, "Computer Science")
9student1.introduce()
In this example, we define a subclass called ‘Student’ that inherits from the ‘Person’ class using the ‘super’ keyword. The subclass has an additional attribute, ‘major’, and overrides the ‘introduce’ method to add information about the student’s major. We create an instance of the subclass using the constructor method ‘init‘ and assign it to the variable ‘student1’. We then call the ‘introduce’ method on the ‘student1’ object, which prints out a greeting message and information about the student’s major.
Example 3: Polymorphism
1def print_info(person):
2 person.introduce()
3person1 = Person("Alice", 25)
4student1 = Student("Bob", 20, "Computer Science")
5print_info(person1)
6print_info(student1)
In this example, we define a function called ‘print_info’ that takes an object of the ‘Person’ or ‘Student’ class as an argument and calls its ‘introduce’ method. We create two instances of the classes, ‘person1’ and ‘student1’, and pass them as arguments to the ‘print_info’ function.
1class Person:
2 def __init__(self, name, age):
3 self.name = name
4 self.age = age
5 def introduce(self):
6 print("Hi, my name is", self.name, "and I'm", self.age, "years old.")
7class Student(Person):
8 def __init__(self, name, age, major):
9 super().__init__(name, age)
10 self.major = major
11 def introduce(self):
12 super().introduce()
13 print("I'm majoring in", self.major)
14def print_info(obj):
15 obj.introduce()
16person1 = Person("Alice", 25)
17student1 = Student("Bob", 20, "Computer Science")
18print_info(person1)
19# Output - Hi, my name is Alice and I'm 25 years old.
20print_info(student1)
21# Output - Hi, my name is Bob and I'm 20 years old. I'm majoring in Computer Science.
In this example, we define a parent class called ‘Person’ and a child class called ‘Student’ that inherits from the ‘Person’ class. The ‘Student’ class overrides the ‘introduce’ method of the ‘Person’ class to include the ‘major’ attribute. We then create two instances of the classes, ‘person1’ and ‘student1’, and pass them as arguments to the ‘print_info’ function, which calls the ‘introduce’ method of each object.
By using the ‘super()’ function, we can access the methods of the parent class from the child class, allowing us to build more complex class hierarchies with shared functionality. Python keywords like ‘class’, ‘def’, ‘super()’, and ‘inheritance’ are essential tools for object-oriented programming and can greatly simplify the creation of large and complex programs.
Advanced Techniques for Using Python Keywords
Python keywords are an important aspect of the language, and they can be used in a variety of advanced programming techniques. Here are some techniques for harnessing the power of Python keywords in your code:
- Using the with statement: The with statement is used for working with context managers. Context managers are objects that define how a resource will be managed, such as opening and closing a file. Using the with statement ensures that the resource is properly managed, even if an error occurs. Here is an example:
1with open('file.txt', 'r') as f:
2data = f.read()
This code opens the file ‘file.txt’ in read mode and assigns the contents to the variable data. The with statement ensures that the file is properly closed, even if an error occurs.
- Using the yield keyword: The yield keyword is used to define a generator function. A generator function returns an iterator, which can be used to iterate over a sequence of values. Here is an example:
1def generate_numbers():
2for i in range(5):
3yield i
4for number in generate_numbers():
5print(number)
This code defines a generator function that returns the numbers 0 to 4. The for loop iterates over the generator and prints each number.
- Using the async and await keywords: The async and await keywords are used to define asynchronous functions. Asynchronous functions allow other code to execute while a long-running operation is being performed, such as waiting for a network request. Here is an example:
1import asyncio
2async def fetch_data():
3 print('Fetching data...')
4 await asyncio.sleep(1)
5 print('Data fetched!')
6async def main():
7 task = asyncio.create_task(fetch_data())
8 print('Other code...')
9 await task
10asyncio.run(main())
This code defines an asynchronous function fetch_data() that waits for one second before printing ‘Data fetched!’. The main() function creates a task for fetch_data(), prints ‘Other code…’, and then waits for the task to complete before exiting.
Python Keywords vs Identifiers: What’s the Difference?
In Python, keywords are a set of reserved words that have a specific meaning and purpose within the language. They are used to define the syntax and structure of the programming language, and cannot be used as variable names or identifiers. Identifiers, on the other hand, are user-defined names that represent variables, functions, classes, and other objects in a Python program.
Here are some examples of Python keywords:
1and, as, assert, break, class, continue, def, del, elif, else, except, False, finally, for, from, global, if, import, in, is, lambda, None, nonlocal, not, or, pass, raise, return, True, try, while, with, yield
To identify whether a word is a Python keyword or not, you can use the ‘keyword’ module in Python. This module provides a list of all the keywords in the language, which you can use to check if a word is a keyword or not.
Here is an example code snippet that demonstrates how to identify a Python keyword:
1import keyword
2
3# check if a word is a Python keyword
4word = 'if'
5if keyword.iskeyword(word):
6 print(word, 'is a Python keyword')
7else:
8 print(word, 'is not a Python keyword')
9
10Output:
11if is a Python keyword
The difference between Python keywords and identifiers is that keywords are predefined words in the Python language that have a specific meaning and purpose, whereas identifiers are user-defined names that represent variables, functions, classes, and other objects in a Python program. Identifiers are not reserved words and can be any valid name that follows the rules of Python naming conventions, such as starting with a letter or underscore, containing only letters, underscores, or digits, and not being a keyword.
It is important to avoid using Python keywords as identifiers in your program, as this can cause errors and unexpected behavior. Instead, use descriptive names that are not keywords to represent your variables, functions, classes, and other objects in Python.
Best Practices for Optimizing Code with Python Keywords
Python keywords are an essential part of the language and can make your code more concise and readable. Here are some best practices for optimizing your code using Python keywords:
How to write clean, efficient code with Python keywords:
- Use descriptive names for your variables, functions, and classes. Avoid using generic names like ‘x’ or ‘temp’, as they can make your code difficult to understand.
- Use the appropriate keywords for each task. For example, use ‘for’ for loops, ‘if’ for conditional statements, ‘def’ for function definitions, and ‘class’ for class definitions.
- Avoid using reserved keywords as variable names, as this can cause errors in your code.
Best practices for optimizing code using Python keywords:
- Use ‘with’ statements when working with resources that need to be cleaned up, such as files or network connections. This ensures that the resource is released when you are done using it, even if an error occurs.
- Use list comprehensions instead of loops whenever possible. List comprehensions are more concise and faster than loops.
- Use the ‘in’ keyword to check if an item is in a list or dictionary, rather than iterating over the entire list or dictionary.
Examples of valid and invalid identifiers:
- Valid identifiers include names that start with a letter or underscore, followed by any combination of letters, numbers, and underscores. Examples of valid identifiers include ‘my_variable’, ‘myFunction’, and ‘_my_variable’.
- Invalid identifiers include names that start with a number, contain spaces or special characters, or are the same as a reserved keyword. Examples of invalid identifiers include ‘2nd_variable’, ‘my variable’, and ‘if’.
By following these best practices, you can write clean, efficient code that is easy to read and maintain.
Common Mistakes with Python Keywords and How to Avoid Them
Using Python keywords incorrectly is a common problem for new programmers. Below is a list of some typical errors and advice on how to avoid them:
- Iterative operation: Using phrases like “break,” “continue,” or “return” outside of a loop is a typical error. This may result in abrupt program termination or unanticipated outcomes. Make sure to only use these keywords within loops or functions to avoid making this error.
- Another frequent error is improperly employing the “return” statement. To end a function and provide the caller with a value, use the “return” statement. The function might not return the desired value or might not return anything at all if the “return” statement is not utilized correctly. Use the “return” statement at the end of a function and return the anticipated value to prevent making this error.
- Using the incorrect syntax for try-except blocks is a common error when handling exceptions. It’s crucial to catch the right exception and deal with it correctly when writing a try-except block. Failing to do so could result in a software crash or unanticipated outcomes. Make sure to catch the right exception and handle it correctly to avoid making this error.
- Lambda functions: Although they are a powerful tool in Python, lambda functions can be challenging to comprehend and effectively use. Not utilizing the proper syntax for lambda functions is a frequent error. It’s crucial to comprehend lambda functions’ syntax and how to give parameters to them. Make sure you comprehend the syntax and practice utilizing lambda functions to prevent making this error.
- Conditional statements: These are used to control how a program works based on certain conditions. A common mistake is not knowing how conditional statements work or not using the right syntax. To avoid this mistake, make sure you understand the syntax and logic of conditional statements and test them thoroughly.
- True, False, or None: In Python, the keywords for Boolean values and null values are True, False, and None. Not utilizing them correctly, such as using “True” as a string or “None” as an integer, is a common error. To prevent making this error, be sure to comprehend how to employ these keywords effectively and do so.
- Control flow and structure: Using the incorrect control flow or structure in a program is another typical error. Unexpected outcomes or blunders may emerge from this. Use the proper syntax for control flow statements and plan out the program’s structure and flow in advance to prevent making this error.
- Variable handling: A typical mistake in variable handling is using keywords as variable names or using the wrong data type for a variable. To avoid this mistake, use the right data type for each variable and follow the rules for naming variables.
Future of Python Keywords: New Additions and Changes
Python has a stable set of keywords, and it is relatively rare for new keywords to be added to the language. However, existing keywords can be modified or deprecated over time as the language evolves. Here are some recent changes to Python keywords:
- In Python 3.10, the async keyword has been added to the list of context managers. This allows it to be used with statements, similar to the await keyword.
- The with statement has been updated to allow multiple items to be passed to the as clause, separated by commas. This allows for cleaner, more readable code when working with multiple resources.
- In Python 3.8, the yield keyword was made an expression instead of a statement. This allows it to be used in combination with the := (walrus) operator to simplify code that generates and consumes values in a loop.
While there are no new keywords currently on the horizon for Python, there are always discussions around potential additions to the language. Here are some proposals for new Python keywords that are currently being discussed:
- case keyword for pattern matching: A proposal to add a case keyword to the language to improve support for pattern matching in Python. This would allow for more concise and readable code when working with complex data structures.
- sub keyword for regular expression matching: A proposal to add a sub keyword to the language to simplify regular expression matching in Python. This would provide a more intuitive syntax for replacing text within a string.
Overall, Python’s keyword set is unlikely to undergo significant changes, as the language’s stability is a key feature that makes it popular among developers. However, as the language evolves, it’s possible that small additions or changes to existing keywords will be made to improve its functionality and readability.
Best Practices for Working with Python Keywords in Large Projects
When working on large Python projects with multiple developers, it’s important to establish collaborative practices for managing Python keywords. Here are some best practices for working with Python keywords in large projects:
A. Collaborative Practices:
- Establish coding conventions and standards for the project, including the use of Python keywords.
- Use version control tools like Git to manage changes to code that involves Python keywords.
- Clearly document the purpose and usage of Python keywords in the codebase to aid other developers.
B. Managing Python Keywords:
- Use descriptive variable and function names to avoid using keywords as identifiers.
- Consider using alternative syntax or constructs when possible to avoid using keywords in situations where they can cause conflicts.
- Regularly review the use of Python keywords in the codebase to ensure they are being used correctly and consistently.
By following these best practices, you can ensure that your team is effectively using Python keywords in a collaborative environment and avoiding potential conflicts or errors.
Conclusion
In conclusion, Python keywords are an essential part of the Python programming language. They have specific meanings and functions that cannot be changed or used as variable names or identifiers. It’s important to be aware of the common mistakes associated with using Python keywords and to avoid them in your code.
When used correctly, Python keywords can be powerful tools for writing clean and efficient code. It’s important to follow best practices for optimizing code using Python keywords, including using meaningful and valid identifiers.
However, these concepts can sometimes pose challenges, especially in assignments where students are required to use keywords and identifiers accurately. If you’re struggling with errors in your Python assignments related to these topics, private tutoring can provide the guidance you need to overcome these hurdles and build confidence in your coding abilities.
As Python continues to evolve, new keywords may be added or existing ones may be changed. It’s important to stay up-to-date with these changes and adapt your code accordingly.
Overall, learning how to use Python keywords effectively can greatly enhance your programming skills and open up new possibilities for your projects. So keep learning and exploring the world of Python programming!
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are keywords in Python?
Keywords in Python are predefined reserved words that have specific meanings and purposes in the language. They are used to define the structure and syntax of the code, and cannot be used as variable names or identifiers.
What is keyword class in Python?
‘Class’ is a reserved keyword in Python that is used to define a new class, which is a user-defined data type. It is used to create objects with specific attributes and behaviors, and is an essential part of object-oriented programming in Python.
What is tuple in Python?
In Python, a tuple is an ordered collection of elements that are immutable and enclosed in parentheses (). Tuples can be used to store related data that should not be modified, such as coordinates or names of colors. They can also be used to return multiple values from a function.
What are the 8 features of Python?
The eight features of Python are:
Simplicity,Readability,Flexibility,Object-orientedness,Platform independence,High-level built-in data structures,Strong introspection capabilities and Large standard library
How many keywords are there in Python?
Python has a total of 35 keywords that are reserved and cannot be used as variable names or identifiers.
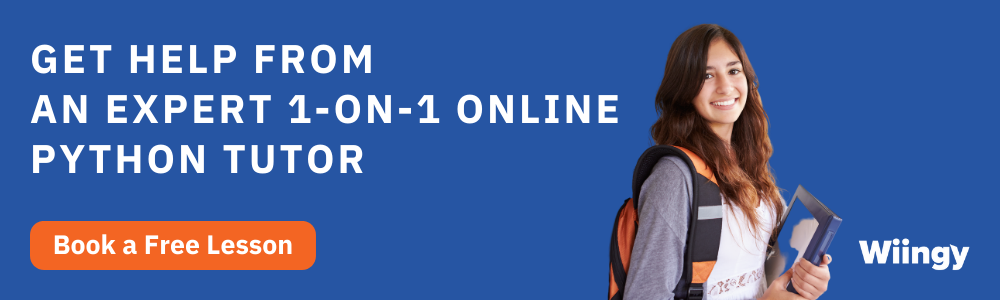
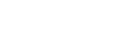
Jan 30, 2025
Was this helpful?