What are Python Data Types?
We need to use data in order to make our programs function. Data is the term used in computer science to describe the information that a computer processes. The various categories of data that are used in python to represent a wide range of values are known as python data types. A program’s functionality can be significantly impacted by the type of data we use in it. Therefore, understanding python data types is crucial for programming.
There are many different data types in the widely used programming language Python. These data types are used to represent various types of data, including dates, text, and numbers. In this article, we will discuss the different data types in Python and how they are used.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Numeric Data Types
In Python, numbers are represented by numeric data types. There are three main numeric Python data types:
- Integer (int): Integers are whole numbers, meaning they do not have any decimal places. Integers can be positive or negative, and they have no upper limit to their value.
Examples of how to use int in code:
1x = 10 y = -5 z = 0
Common operations and functions used with int:
- Addition: x + y
- Subtraction: x – y
- Multiplication: x * y
- Division: x / y
- Modulus: x % y
- Power: x ** 2
- Float (float): Floats are used to represent numbers that have decimal places. They can be positive or negative, and they have a limited range of values.
Examples of how to use float in code:
1x = 3.14 y = -2.5 z = 0.0
Common operations and functions used with float:
- Addition: x + y
- Subtraction: x – y
- Multiplication: x * y
- Division: x / y
- Modulus: x % y
- Power: x ** 2
- Complex (complex): Complex numbers are used to represent numbers that have both a real and imaginary part. They are expressed in the form of a + bj, where a is the real part and b is the imaginary part.
Examples of how to use complex in code:
1x = 3 + 4j y = 2 - 5j z = 0j
Common operations and functions used with complex:
- Addition: x + y
- Subtraction: x – y
- Multiplication: x * y
- Division: x / y
String Data Type
In Python, a series of characters are represented by the string data type. Single or double quotes are used to enclose strings. They may contain any alphabetical, numeric, or symbolic combination.
Examples of how to create and manipulate strings in code:
1# Creating a string
2message = "Hello, World!"
3
4# Accessing characters in a string
5print(message[0])
6# Output: H
7print(message[-1])
8# Output: !
9
10# Slicing a string
11print(message[0:5])
12# Output: Hello
13print(message[7:])
14# Output: World!
15
16# Concatenating strings
17first_name = "John"
18last_name = "Doe"
19full_name = first_name + " " + last_name
20print(full_name)
21# Output: John Doe
22
23# Converting case
24print(message.upper())
25# Output: HELLO, WORLD!
26print(message.lower())
27# Output: hello, world!
28
29# Replacing characters
30new_message = message.replace("World", "Python Data Types") print(new_message)
31# Output: Hello, Python Data Types!
Common string operations and functions:
- Length: len()
- Concatenation: +
- Slicing: []
- Replace: replace()
- Upper and lower case: upper(), lower()
- Strip whitespace: strip()
Boolean Data Type
The boolean data type in Python is used to represent truth values. It can have only two values: True and False. Boolean values are often used in programming to make decisions based on whether a condition is true or false.
Examples of how to use boolean values in code:
1x = 10
2y = 5
3# Comparing values print(x > y)
4# Output: True print(x == y)
5# Output: False print(x != y)
6# Output: True
7# Logical operators print(x > y and x != y)
8# Output: True print(x < y or x == y)
9# Output: False
10# Assigning boolean values
11is_raining = True
12is_sunny = False
Common boolean operations and functions:
- Comparison operators: >, <, ==, !=, >=, <=
- Logical operators: and, or, not
- Converting values to boolean: bool()
Sequence Data Types
In Python, collections of values are represented by sequence data types. Lists, tuples, and range are the three primary sequence data types in Python.
Explanation of sequence data types in Python:
- Lists: Lists are used to represent an ordered collection of values. Lists are mutable, which means that we can add, remove, or modify items in the list.
- Tuples: Tuples are used to represent an ordered collection of values. Tuples are immutable, which means that once we create a tuple, we cannot add, remove, or modify items in the tuple.
- Range: Range is used to represent a sequence of numbers. It is often used in loops to iterate over a specific range of values.
Examples of how to create and manipulate each sequence data type in code:
1# Creating a list
2fruits = ["apple", "banana", "cherry"]
3# Accessing items in a list print(fruits[0])
4# Output: apple print(fruits[-1])
5# Output: cherry
1# Modifying items in a list
2fruits[1] = "orange"
3print(fruits)
4# Output: ['apple', 'orange', 'cherry']
5# Creating a tuple
6months = ("January", "February", "March")
1# Accessing items in a tuple
2print(months[0])
3# Output: January
4print(months[-1])
5# Output: March
6
7# Creating a range
8numbers = range(0, 10)
9
10# Iterating over a range
11for number in numbers:
12print(number)
13# Output: 0 1 2 3 4 5 6 7 8 9
Common sequence operations and functions:
- Length: len()
- Concatenation: +
- Repetition: *
- Slicing: []
- Modifying: append(), insert(), remove(), pop()
- Converting to other sequence types: list(), tuple()
Set and Dictionary Data Types
Python uses the set and dictionary data types to represent collections of values that aren’t necessarily in any particular order.
Explanation of the set and dictionary data types in Python
- Set: Sets are used to represent an unordered collection of unique values. Sets are mutable, which means that we can add, remove, or modify items in the set.
- Dictionary: Dictionaries are used to represent a collection of key-value pairs. Each key is associated with a value, and we can use the key to access the corresponding value. Dictionaries are mutable, which means that we can add, remove, or modify key-value pairs in the dictionary.
Examples of how to create and manipulate sets and dictionaries in code:
1# Creating a set
2fruits = {"apple", "banana", "cherry"}
3# Adding items to a set
4fruits.add("orange")
5print(fruits)
6# Output: {'cherry', 'apple', 'banana', 'orange'}
1# Removing items from a set
2fruits.remove("banana")
3print(fruits)
4# Output: {'cherry', 'apple', 'orange'}
5# Creating a dictionary
6person = { "name": "John", "age": 30, "city": "New York" }
# Accessing items in a dictionary print(person[“name”]) # Output: John print(person.get(“age”)) # Output: 30
# Modifying items in a dictionary person[“age”] = 40 print(person) # Output: {‘name’: ‘John’, ‘age’: 40, ‘city’: ‘
Type Conversion in Python
In Python, type conversion refers to the transformation of one data type into another. Python comes with built-in functions for converting data between different data types.
Explanation of type conversion in Python:
- Implicit Type Conversion: Python automatically converts one data type to another when necessary using implicit type conversion. For instance, Python will convert the integer to a float before performing the addition if we attempt to add an integer and a float.
- Explicit Type Conversion: By using built-in functions, we can also explicitly convert data from one type to another. For instance, we can convert an integer to a string using the str() function.
Examples of how to convert data types in code:
1# Implicit Type Conversion
2x = 10
3# int
4
5y = 3.14
6# float
7
8z = x + y
9print(z)
10# Output: 13.14
1# Explicit Type Conversion
2a = "10"
3# string
4
5b = int(a)
6print(b)
7# Output: 10
8
9c = "3.14"
10# string
11
12d = float(c)
13print(d)
14# Output: 3.14
Common type conversion functions:
- int(): Converts to an integer
- float(): Converts to a float
- str(): Converts to a string
- bool(): Converts to a boolean
- list(): Converts to a list
- tuple(): Converts to a tuple
- set(): Converts to a set
- dict(): Converts to a dictionary
Conclusion
The various Python data types and their applications were covered in this article. We discussed a variety of data types, including boolean, string, boolean, sequence (lists, tuples, and range), set, and dictionary data types. We also talked about data type conversion and how to change one type of data into another.
Programming requires a thorough understanding of data types because doing so can increase code productivity and reduce bugs. However, applying this knowledge in assignments or debugging type-related errors in real-world scenarios can be challenging.
If you are struggling with data type issues or Python-related errors in your assignments, private tutoring can help you with Python assignments, providing tailored guidance to address these challenges effectively. With the right support, you can build confidence in using Python data types correctly and write cleaner, more efficient code.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs:
What are Python’s four different data types?
There are more than 4 python data types , but the four main built-in data types are integer, float, string, and boolean.
What are the 5 data types in Python?
The five main built-in python data types are integer, float, string, boolean, and complex.
What is a datatype in Python?
A data type in Python is a category of values that defines the operations that can be performed on those values.
How many data types are in Python?
Python has many built-in data types, including numeric types (int, float, complex), string, boolean, sequence types (list, tuple, range), and set and dictionary types.
Other Useful Reads
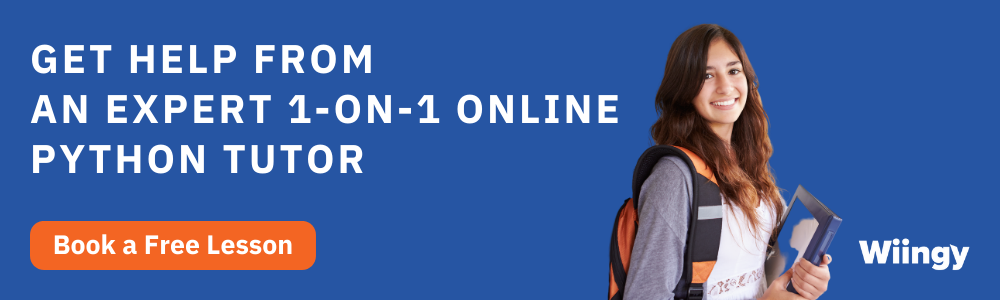
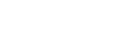
Jan 30, 2025
Was this helpful?