Python Tutorials
What are Python Operators?
Operators are special symbols or characters that are used in programming to do things to one or more operands.Variables, values, or expressions could be used as these operands. Like other programming languages, Python offers a wide range of operators that can be used to alter data in various ways.
Operators are an important part of programming languages like Python because they make it easier and faster for programmers to do different things with data.We can carry out operations like addition, subtraction, multiplication, division, comparison, and many more thanks to operators.
We’ll talk about Python operators and their types in this blog article. We will focus on bitwise operators, identity operators, membership operators, comparison operators, arithmetic operators, and more.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Python Arithmetic Operators
Arithmetic operators are used to perform mathematical operations on numeric operands. Python provides the following arithmetic operators:
- Addition (+): This operator is used to add two or more values. For example, 2 + 3 = 5.
- Subtraction (-): This operator is used to subtract one value from another. For example, 5 – 2 = 3.
- Multiplication (*): This operator is used to multiply two or more values. For example, 2 * 3 = 6.
- Division (/): This operator is used to divide one value by another. In Python 3, the division operator always returns a float value. For example, 6 / 3 = 2.0.
- Modulus (%): This operator returns the remainder of a division operation. For example, 7 % 3 = 1.
- Exponentiation (**): This operator is used to raise a number to a certain power. For example, 2 ** 3 = 8.
- Floor Division (//): This operator performs integer division and returns the floor value of the quotient. For example, 7 // 3 = 2.
Here are some example code snippets that demonstrate the use of arithmetic operators in Python:
- Addition (+)
num1 = 10
num2 = 5
sum = num1 + num2
print("The sum is:", sum)
Output: The sum is: 15
- Subtraction (-)
num1 = 10
num2 = 5
diff = num1 - num2
print("The difference is:", diff)
Output: The difference is: 5
- Multiplication (*)
num1 = 10
num2 = 5
prod = num1 * num2
print("The product is:", prod)
Output: The product is: 50
- Division (/)
num1 = 10
num2 = 5
quo = num1 / num2
print("The quotient is:", quo)
Output: The quotient is: 2.0
- Modulus (%)
num1 = 10
num2 = 3
rem = num1 % num2
print("The remainder is:", rem)
Output: The remainder is: 1
- Exponentiation (**)
num1 = 2
num2 = 3
result = num1 ** num2
print("The result is:", result)
Output: The result is: 8
- Floor Division (//)
num1 = 7
num2 = 3
result = num1 // num2
print("The result is:", result)
Output: The result is: 2
Python Assignment Operators
In Python, assignment operators are used to assign values to variables. They are a shorthand way of performing an arithmetic operation and then assigning the result back to the same variable. Assignment operators combine the operation and assignment of a variable in one step, making the code shorter and more concise. Python provides several types of assignment operators, including simple assignment, addition assignment, subtraction assignment, multiplication assignment, division assignment, modulus assignment, exponentiation assignment, floor division assignment, and bitwise assignment.
Here are some example code snippets that demonstrate the use of assignment operators in Python:
- Simple assignment (=)
x = 10
print(x)
Output: 10
In this example, the value 10 is assigned to the variable x using the assignment operator.
- Addition assignment (+=)
x = 10
x += 5
print(x)
Output: 15
In this example, the value 5 is added to the existing value of the variable x using the addition assignment operator (+=).
- Subtraction assignment (-=)
x = 10
x -= 5
print(x)
Output: 5
In this example, the value 5 is subtracted from the existing value of the variable x using the subtraction assignment operator (-=).
- Multiplication assignment (*=)
x = 10
x *= 5
print(x)
Output: 50
In this example, the existing value of the variable x is multiplied by 5 using the multiplication assignment operator (*=).
- Division assignment (/=)
x = 10
x /= 5
print(x)
Output: 2.0
In this example, the existing value of the variable x is divided by 5 using the division assignment operator (/=).
- Modulus assignment (%=)
x = 10
x %= 3
print(x)
Output: 1
In this example, the existing value of the variable x is divided by 3 using the modulus assignment operator (%=), and the remainder is assigned back to x.
- Exponentiation assignment (**=)
x = 2
x **= 3
print(x)
Output: 8
In this example, the existing value of the variable x is raised to the power of 3 using the exponentiation assignment operator (**=).
- Floor division assignment (//=)
x = 7
x //= 3
print(x)
Output: 2
In this example, the existing value of the variable x is divided by 3 using the floor division assignment operator (//=), and the result is assigned back to x.
- Bitwise assignment
x = 0b101
x |= 0b010
print(bin(x))
Output: 0b111
In this example, the bitwise OR assignment operator (|=) is used to perform a bitwise OR operation between the existing value of the variable x (0b101) and the binary value 0b010, and the result (0b111) is assigned back to x.
Python Comparison Operators
Explanation of comparison operators: In Python, comparison operators are used to compare two values and return a Boolean value (True or False) based on the result of the comparison. Comparison operators are used to test the relationship between two values and are an important part of programming. Python provides several types of comparison operators, including equal to, not equal to, greater than, less than, greater than or equal to, and less than or equal to.
Example code snippets for comparison operators: Here are some example code snippets that demonstrate the use of comparison operators in Python:
- Equal to (==)
x = 10
y = 5 + 5
print(x == y)
Output: True
In this example, the values of x and y are compared using the equal to operator (==), which returns True because they have the same value.
- Not equal to (!=)
x = 10
y = 5
print(x != y)
Output: True
In this example, the values of x and y are compared using the not equal to operator (!=), which returns True because they have different values.
- Greater than (>)
x = 10
y = 5
print(x > y)
Output: True
In this example, the values of x and y are compared using the greater than operator (>), which returns True because x is greater than y.
- Less than (<)
x = 10
y = 15
print(x < y)
Output: True
In this example, the values of x and y are compared using the less than operator (<), which returns True because x is less than y.
- Greater than or equal to (>=)
x = 10
y = 10
print(x >= y)
Output: True
In this example, the values of x and y are compared using the greater than or equal to operator (>=), which returns True because x is equal to y.
- Less than or equal to (<=)
x = 10
y = 15
print(x <= y)
Output: True
In this example, the values of x and y are compared using the less than or equal to operator (<=), which returns True because x is less than y.
Python Logical Operators
A. Explanation of logical operators: In Python, logical operators are used to combine multiple Boolean expressions into a single expression. Logical operators include AND, OR, and NOT, and are used to evaluate whether a condition is True or False. Logical operators are useful for controlling the flow of a program, as they allow programmers to create complex conditions that must be met for certain code to be executed.
B. Example code snippets for logical operators:
- Logical AND (and)
x = 5
y = 10
if x > 0 and y < 20:
print("Both conditions are True")
Output: Both conditions are True
In this example, the logical AND operator (and) is used to combine two Boolean expressions. The if statement checks if both conditions are True, and since x is greater than 0 and y is less than 20, the print statement is executed.
- Logical OR (or)
x = 5
y = 10
if x < 0 or y > 20:
print("At least one condition is True")
Output: At least one condition is True
In this example, the logical OR operator (or) is used to combine two Boolean expressions. The if statement checks if at least one condition is True, and since x is not less than 0 but y is greater than 20, the print statement is executed.
- Logical NOT (not)
x = True
if not x:
print("x is False")
else:
print("x is True")
Output: x is True
In this example, the logical NOT operator (not) is used to invert the value of a Boolean expression. The if statement checks if x is not True, but since x is True, the else statement is executed and “x is True” is printed.
Python Identity Operators
A. Explanation of identity operators:
In Python, identity operators are used to compare the memory location of two objects. Identity operators include is and is not, and are used to check if two objects are the same object in memory. Identity operators are useful for checking if two variables refer to the same object, which can be important when working with mutable objects like lists and dictionaries.
B. Example code snippets for identity operators:
- is
x = [1, 2, 3]
y = [1, 2, 3]
z = x
if x is y:
print("x and y refer to the same object")
if x is z:
print("x and z refer to the same object")
Output: x and z refer to the same object
In this example, the identity operator is (is) is used to check if x and y refer to the same object in memory. Since x and y have the same values but are different objects, the first if statement is False. However, z is assigned to the same object as x, so the second if statement is True.
- is not
x = [1, 2, 3]
y = [1, 2, 3]
if x is not y:
print("x and y do not refer to the same object")
Output: x and y do not refer to the same object
In this example, the identity operator is not (is not) is used to check if x and y do not refer to the same object in memory. Since x and y have the same values but are different objects, the if statement is True.
Python Membership Operators A. Explanation of membership operators In Python, membership operators are used to test whether a value or variable is a member of a sequence or not. The membership operators are ‘in’ and ‘not in’.
The ‘in’ operator returns True if a value is found in the sequence and False otherwise. For example:
my_list = [1, 2, 3, 4, 5]
if 3 in my_list:
print("3 is present in my_list")
Output:
3 is present in my_list
The ‘not in’ operator returns True if a value is not found in the sequence and False otherwise. For example:
my_list = [1, 2, 3, 4, 5]
if 6 not in my_list:
print("6 is not present in my_list")
Output:
6 is not present in my_list
B. Example code snippets for:
- in
- not in
Example code snippet for in:
my_string = "Hello World"
if 'l' in my_string:
print("l is present in my_string")
Output:
l is present in my_string
Example code snippet for not in:
my_string = "Hello World"
if 'x' not in my_string:
print("x is not present in my_string")
Output:
x is not present in my_string
Python Bitwise Operators
A. Explanation of bitwise operators
In Python, bitwise operators are used to perform bitwise operations on integers. Bitwise operators operate on bits and perform the AND, OR, XOR, and NOT operations.
The bitwise AND operator returns a 1 in each bit position where both operands have a 1, and a 0 otherwise. For example:
a = 10 # Binary representation: 1010
b = 6 # Binary representation: 0110
c = a & b # Binary representation: 0010
print(c) # Output: 2
The bitwise OR operator returns a 1 in each bit position where either or both operands have a 1, and a 0 otherwise. For example:
a = 10 # Binary representation: 1010
b = 6 # Binary representation: 0110
c = a | b # Binary representation: 1110
print(c) # Output: 14
The bitwise XOR operator returns a 1 in each bit position where only one of the operands has a 1, and a 0 otherwise. For example:
a = 10 # Binary representation: 1010
b = 6 # Binary representation: 0110
c = a ^ b # Binary representation: 1100
print(c) # Output: 12
The bitwise NOT operator returns the complement of the operand, i.e., it flips all the bits. For example:
a = 10 # Binary representation: 1010
b = ~a # Binary representation: 0101
print(b) # Output: -11
Note: The output is a negative number because the binary representation of -11 is 11111111111111111111111111110101 in two’s complement notation.
The left shift operator shifts the bits of the operand to the left by a specified number of positions, and fills the empty bits with 0s. For example:
a = 10 # Binary representation: 1010
b = a <<
Operator Precedence
A. Explanation of operator precedence:
Operator precedence refers to the order in which operators are evaluated in an expression. This is important because it can affect the outcome of an expression. Python has a set of rules for operator precedence, which are used to determine the order in which operators are evaluated.
The following is a list of Python operators in order of precedence, from highest to lowest:
- Parentheses – ()
- Exponentiation – **
- Multiplication, Division, Modulus, and Floor Division – *, /, %, //
- Addition and Subtraction – +, –
- Bitwise Shifts – <<, >>
- Bitwise AND – &
- Bitwise XOR – ^
- Bitwise OR – |
- Comparison Operators – ==, !=, >, <, >=, <=
- Logical NOT – not
- Logical AND – and
- Logical OR – or
B. Example code snippets:
- In the expression 3 + 4 * 5, multiplication has a higher precedence than addition, so the expression is evaluated as 3 + (4 * 5) = 23.
- In the expression 2 ** 3 ** 2, exponentiation has a higher precedence than exponentiation, so the expression is evaluated as 2 ** (3 ** 2) = 2 ** 9 = 512.
- In the expression 10 + 5 % 3 * 2, multiplication and modulus have the same precedence, so they are evaluated from left to right. The expression is evaluated as 10 + (5 % 3) * 2 = 10 + 2 * 2 = 14.
X. Associativity of Operators
A. Explanation of associativity of operators:
Associativity of operators refers to the order in which operators of the same precedence are evaluated in an expression. Operators can be left-associative or right-associative. Left-associative means that the operators are evaluated from left to right, while right-associative means that the operators are evaluated from right to left.
In Python, most operators are left-associative, meaning that they are evaluated from left to right. The only right-associative operator is the exponentiation operator (**).
B. Example code snippets:
- In the expression 4 – 3 + 2, the addition and subtraction operators have the same precedence and are left-associative. Therefore, the expression is evaluated as (4 – 3) + 2 = 1 + 2 = 3.
- In the expression 2 ** 3 ** 2, the exponentiation operator is right-associative, meaning that it is evaluated from right to left. Therefore, the expression is evaluated as 2 ** (3 ** 2) = 2 ** 9 = 512.
- In the expression 5 * 3 / 2, the multiplication and division operators have the same precedence and are left-associative. Therefore, the expression is evaluated as (5 * 3) / 2 = 15 / 2 = 7.5.
Equality Comparison on Floating-Point Values
A. Explanation of equality comparison on floating-point values:
In Python, comparing floating-point values for exact equality can be problematic due to precision issues. This is because floating-point numbers are represented in binary, and some decimal values cannot be represented exactly in binary. Thus, comparisons of floating-point values are often done using a tolerance, meaning the values are considered equal if they are within a certain range of each other.
For example, consider the following code snippet:
a = 0.1 + 0.2
b = 0.3
print(a == b)
One might expect the output of this code to be True, since 0.1 + 0.2 is indeed equal to 0.3 in decimal notation. However, due to the way floating-point numbers are represented in binary, a and b are not exactly equal. Thus, the output of this code will be False.
To compare floating-point values with a tolerance in Python, you can use the built-in math.isclose() function. This function takes two arguments, the values to be compared, and an optional rel_tol argument that specifies the relative tolerance. The default value of rel_tol is 1e-9, which is often sufficient for most use cases.
Here is an example:
import math
a = 0.1 + 0.2
b = 0.3
print(math.isclose(a, b))
This code will output True, since a and b are considered close enough to be equal with the default tolerance.
B. Example code snippets:
import math
a = 0.1 + 0.2
b = 0.3
print(math.isclose(a, b)) # Output: True
c = 0.123456789
d = 0.123456788
print(math.isclose(c, d)) # Output: False
e = 1000000.01
f = 1000000.00
print(math.isclose(e, f, rel_tol=1e-2)) # Output: True
Conclusion
In this blog post, we have covered Python operators. Operators are symbols that perform operations on one or more operands, and they are an essential part of any programming language. In Python, there are various types of operators, such as arithmetic, assignment, comparison, logical, membership, and bitwise operators.
We started by defining operators and discussing their importance in Python. We then covered the different types of operators and provided code snippets for each. We also discussed operator precedence and associativity, two important concepts that help determine the order in which operators are evaluated.
Finally, we discussed the issues with comparing floating-point values for exact equality and provided a solution using the math.isclose() function.
Operators are an essential part of any programming language, and Python is no exception. Understanding how operators work and how to use them effectively is crucial for writing efficient and effective code. By using the examples and explanations provided in this blog post, you should be well-equipped to start using operators in your Python code
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are the types of Python Operators?
Python has a wide range of operators which are used to perform different operations. There are seven categories of operators in Python which are:
Arithmetic Operators: used to perform mathematical operations like addition, subtraction, multiplication, division, and modulus
Comparison Operators: used to compare two values and return Boolean values of True or False
Assignment Operators: used to assign values to variables
Logical Operators: used to combine conditional statements and return Boolean values of True or False
Bitwise Operators: used to perform bitwise operations on integers
Membership Operators: used to test if a value is a member of a sequence
Identity Operators: used to compare the memory locations of two objects
What is an operator in Python?
Operators in Python are special symbols that represent specific actions. These actions can be mathematical operations like addition, subtraction, multiplication, division, etc., or logical operations like AND, OR, and NOT. Python has various types of operators that are used to perform different types of operations.
How to use %= in Python?
In Python, the %= operator is used to perform the modulus operation on two operands and then assigns the result to the left operand. For example, if we have two variables a and b, and we want to perform the modulus operation on them and assign the result to a, we can use the %= operator as follows:
a %= b
This is equivalent to writing:
a = a % b
What does -= do in Python?
In Python, the -= operator is used to perform subtraction and assignment in a single operation. For example, if we have two variables a and b, and we want to subtract the value of b from a and then assign the result to a, we can use the -= operator as follows:
a -= b
This is equivalent to writing:
a = a – b
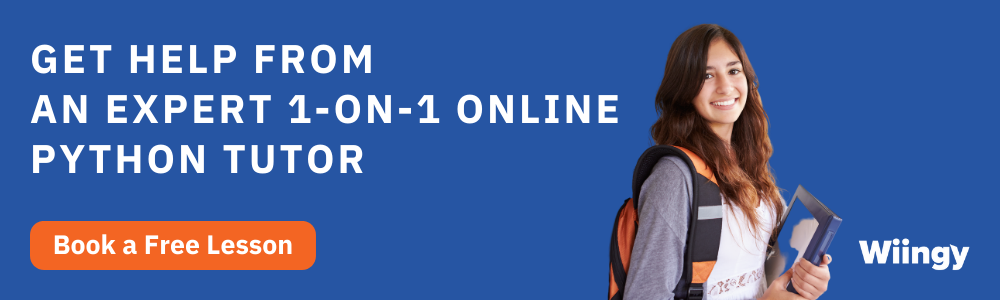
Written by by
Rahul LathReviewed by by
Arpit Rankwar