Python Tutorials
What are For Loops in Python?
Python for loop iterates over a sequence of values in a data structure like a list, tuple, or string. For each item in the sequence, the loop runs a block of code. This lets the programmer do a set of actions for each item in the sequence.
Python programmers rely heavily on for loops due to their ability to automate repetitive tasks and process large amounts of data. They allow the programmer to quickly and easily handle tasks that are done repeatedly by iterating over a range of values and running code on each value.
With for loops, the programmer can iteratively access and modify a single item in a collection, making them useful for processing data structures like lists, dictionaries, and sets.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Numeric Range Loop:
A specific kind of for loop called a numeric range loop iterates over a range of numeric values. It enables the programmer to specify a range’s beginning, ending, and step values before executing a block of code for each value.
A. Syntax of Numeric Range Loop:
The syntax of a numeric range loop in Python is as follows:
i in range(start, stop, step): </span>
<span style="font-family: -apple-system, BlinkMacSystemFont, "Segoe UI", Roboto, Oxygen-Sans, Ubuntu, Cantarell, "Helvetica Neue", sans-serif;"># code to be executed</span>
<span style="font-family: -apple-system, BlinkMacSystemFont, "Segoe UI", Roboto, Oxygen-Sans, Ubuntu, Cantarell, "Helvetica Neue", sans-serif;">for each value in the range
Here, ‘start’ specifies the starting value of the range, ‘stop’ specifies the ending value of the range (exclusive), and ‘step’ specifies the increment between each value in the range.
B. of Numeric Range Loop:
Example 1: Printing numbers from 1 to 10 using a numeric range loop
for i in range(1, 11): print(i)
#Output
1 2 3 4 5 6 7 8 9 10
Example 2: Printing even numbers from 2 to 10 using a numeric range loop
for i in range(2, 11, 2): print(i)
#Output
2 4 6 8 10
Example 3: Summing numbers from 1 to 10 using a numeric range loop
sum = 0 for i in range(1, 11): sum += i print(sum)
#Output
55
Three-Expression Loop:
The three-expression loop is a type of for loop in Python that includes three expressions: initialization, condition, and increment/decrement. The initialization expression sets the starting value of the loop variable, the condition expression checks if the loop should continue or terminate, and the increment/decrement expression updates the loop variable after each iteration.
A. Syntax of Three-Expression Loop:
The syntax of the three-expression loop in Python is as follows:
for i in range(start, stop, step):
# code to be executed for each value in the range
Here, ‘start’ specifies the starting value of the loop variable, ‘stop’ specifies the ending value of the loop variable (exclusive), and ‘step’ specifies the increment or decrement value.
B. Examples of Three-Expression Loop:
Example 1: Printing numbers from 1 to 10 using a three-expression loop
for i in range(1, 11, 1): print(i)
#Output
1 2 3 4 5 6 7 8 9 10
Example 2: Printing even numbers from 2 to 10 using a three-expression loop
for i in range(2, 11, 2): print(i)
#Output
2 4 6 8 10
Collection-Based or Iterator-Based Loop
A. Definition of Collection-Based or Iterator-Based Loop:
The collection-based or iterator-based loop is a type of for loop in Python that iterates over a collection of elements, such as a list, tuple, or dictionary. It uses an iterator to retrieve each element in the collection and executes a block of code for each element.
B. Syntax of Collection-Based or Iterator-Based Loop:
The syntax of the collection-based or iterator-based loop in Python is as follows:
for element in collection: # code to be executed for each element in the collection
Here, ‘element’ is the variable that holds each element in the collection, and ‘collection’ is the collection of elements to iterate over.
C. Examples of Collection-Based or Iterator-Based Loop:
Example 1: Printing each element in a list using a collection-based loop
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
#Output
apple banana cherry
Example 2: Iterating over a dictionary using a collection-based loop
person = {"name": "John", "age": 36, "country": "USA"} for key, value in person.items(): print(key, ":", value)
#Output
name : John age : 36 country : USA
The Python for Loop:
The Python for loop is a control flow statement that allows the programmer to iterate over a sequence of elements, such as a list or string, and execute a block of code for each element. It is a powerful tool for automating repetitive tasks and processing large amounts of data.
A. Syntax of Python for Loop:
The syntax of the Python for loop is as follows:
for variable in sequence: # code to be executed for each element in the sequence
Here, ‘variable’ is the variable that holds each element in the sequence, and ‘sequence’ is the sequence of elements to iterate over.
B. Examples of Python for Loop:
Example 1 : Printing each character in a string using a Python for loop
text = "Python for loops are awesome" for character in text: print(character)
#Output
P y t h o n f o r l o o p s a r e a w e s o m e
Example 2: Summing numbers from a list using a Python for loop
/ numbers = [1, 2, 3, 4, 5] sum = 0 for number in numbers: sum += number print(sum)
#Output
15
Example 3: Printing a multiplication table using a nested Python for loop
for i in range(1, 11): for j in range(1, 11): print(i, "*", j, "=", i*j)
#Output
1 * 1 = 1 1 * 2 = 2 1 * 3 = 3 ... 10 * 8 = 80 10 * 9 = 90 10 * 10 = 100
Iterables:
Any object that can be iterated over using a for loop is considered an iterable in Python. Iterables include lists, tuples, strings, dictionaries, sets, and any object that implements the iter() method.
Examples of Iterables:
Examples of iterables in Python include:
- Lists: [1, 2, 3, 4, 5]
- Tuples: (1, 2, 3, 4, 5)
- Strings: “Hello, World!”
- Dictionaries: {“name”: “John”, “age”: 36}
- Sets: {1, 2, 3, 4, 5}
Iterators:
An object that enables iteration over an iterable, such as a list or string, is called an iterator. It keeps track of each item’s current location within the iterable while retrieving one item at a time.
Examples of Iterators:
Examples of iterators in Python include:
- The range() function: range(1, 11)
- The enumerate() function: enumerate([“apple”, “banana”, “cherry”])
- The zip() function: zip([1, 2, 3], [4, 5, 6])
Python For Loop’s Complete Functionality/Working:
The Python for loop repeatedly accesses an iterable object, extracting each item in turn and running a block of code for each one. The for loop retrieves each item in the iterable using an iterator, and iterations are repeated until the iterable is empty.
Examples of Python for Loop:
Example 1: Printing each element in a list using a for loop
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
apple banana cherry
Example 2: Iterating over a dictionary using a for loop
person = {"name": "John", "age": 36, "country": "USA"} for key in person: print(key, ":", person[key])
#Output
name : John age : 36 country : USA
Example 3: Using a for loop with the range() function to print numbers from 1 to 10
for i in range(1, 11): print(i)
#Output
1 2 3 4 5 6 7 8 9 10
Iterating Through a Dictionary:
A key-value pair in a dictionary can be accessed and a block of code can be run for each pair by using a Python for loop to iterate through the dictionary. When processing data or carrying out operations on each key-value pair, this can be helpful.
Examples of iterating through a dictionary using Python for Loop:
Example 1: Printing each key-value pair in a dictionary
person = {"name": "John", "age": 36, "country": "USA"} for key, value in person.items(): print(key, ":", value)
#Output
name : John age : 36 country : USA
Example 2: Calculating the total value of a dictionary of prices and quantities
prices = {"apple": 0.5, "banana": 0.25, "cherry": 1.0} quantities = {"apple": 5, "banana": 10, "cherry": 2} total = 0 for fruit, price in prices.items(): total += price * quantities[fruit] print(total)
#Output
5.5
The range() Function:
Python has a built-in function called range() that produces a series of numbers. It is commonly used in conjunction with for loops to iterate over a range of values.
A. Syntax of range() Function:
The syntax of the range() function in Python is as follows:
range(start, stop, step)
Here, ‘start’ is the starting value of the sequence (inclusive), ‘stop’ is the ending value of the sequence (exclusive), and ‘step’ is the increment or decrement between each value in the sequence.
B. Examples of range() Function:
Example 1: Printing numbers from 1 to 10 using the range() function
for i in range(1, 11): print(i)
#Output
1 2 3 4 5 6 7 8 9 10
Example 2: Printing even numbers from 2 to 10 using the range() function
for i in range(2, 11, 2): print(i)
#Output
2 4 6 8 10
Altering for Loop Behavior
Using keywords like “break” and “continue” to change the loop’s direction can change how a Python for loop behaves. These keywords enable the programmer to skip over particular elements or end the loop early depending on particular circumstances.
A. Examples of changing the behavior of Python for Loop:
Example 1: Using the ‘break’ keyword to terminate a loop early
fruits = ["apple", "banana", "cherry"] for fruit in fruits: if fruit == "banana": break print(fruit)
#Output
Apple
Example 2: Using the ‘continue’ keyword to skip over certain elements in a loop
for i in range(1, 11): if i % 2 == 0: continue print(i)
#Output
1 3 5 7 9
The else Clause:
After a Python for loop has finished iterating through all of the elements in the iterable, the else clause is put to use. If the loop ends normally without being terminated by a “break” statement, it is typically used to run a block of code.
Examples of else clause in Python for Loop:
Example 1: Checking if a number is prime using a for loop and else clause
number = 17 for i in range(2, number): if number % i == 0: print(number, "is not prime") break else: print(number, "is prime")
#Output
17 is prime
Example 2: Searching for an element in a list using a for loop and else clause
fruits = ["apple", "banana", "cherry"] search_term = "orange" for fruit in fruits: if fruit == search_term: print(search_term, "is in the list") break else: print(search_term, "is not in the list")
#Output
orange is not in the list
Conclusion
The fundamentals of Python for loops, including iterating over numeric ranges, collections, and dictionaries, have been covered in this article. Along with the use of the range() function and changing the behavior of for loops with words like “break” and “continue,” we have also covered the ideas of iterables and iterators. Finally, we discussed the else clause in for loops and how it can be used to execute code based on whether the loop succeeds or fails.
When automating repetitive tasks and processing large amounts of data, the Python for loop is a crucial tool. Writing effective Python code requires an understanding of for loop concepts, including iterables, iterators, and the range() function. Understanding for loops can boost a programmer’s productivity and make their code easier to read and maintain.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is the for loop in Python?
In a Python for loop, a control flow statement, a block of code is executed for each item in an iterable object as the iteration continues. It is frequently used to process large amounts of data and automate repetitive tasks.
What are the 3 types of loops in Python?
The three types of loops in Python are:
for loop
while loop
do-while loop (although this is not a built-in loop in Python)
How do you write a loop in Python?
To write a loop in Python, you can use the ‘for’ or ‘while’ keywords, followed by a block of code that will be executed for each iteration of the loop. For example, a simple for loop that prints the numbers 1 to 10 would be written as:
for i in range(1, 11): print(i)
How to use \n in for loop in Python?
You can use the ‘\n’ character in a for loop in Python to create a new line. For example, to print a list of items on separate lines, you can use the following code:
fruits = [“apple”, “banana”, “cherry”] for fruit in fruits: print(fruit + ‘\n’)
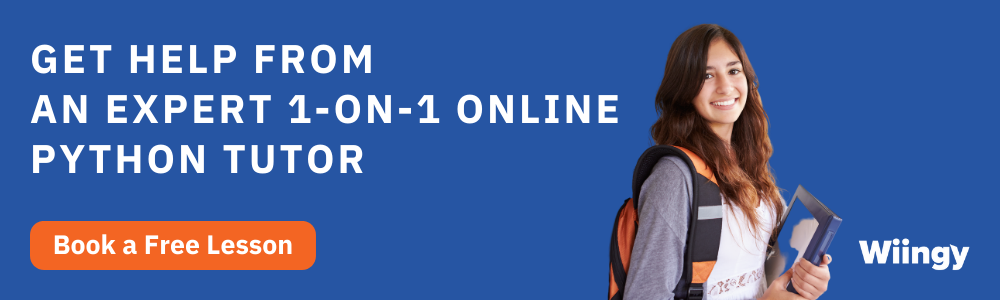
Written by by
Rahul LathReviewed by by
Arpit Rankwar