What are Class and Static Methods in Python?
Class methods and static methods can be used in Python, an object-oriented programming language. These methods are frequently used in conjunction with instance methods and are used to carry out specific tasks within a class. Similar to static methods, class methods are methods that are part of the class itself rather than a class instance. However, they serve various functions and are applied in various ways.
This article’s goals are to describe the distinctions between Python’s class methods and static methods and to provide usage examples.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Class Methods
In contrast to a class instance, a class method is a method that is a component of the class itself. It is used to perform operations related to the class as a whole instead of a specific instance of the class.
A class method is defined using the @classmethod decorator.
It takes the cls parameter as its first argument, which refers to the class itself.
How to define a class method in Python:
1class MyClass:
2 @classmethod
3 def my_class_method(cls, arg1, arg2):
4 # Method body
How to call a class method:
Class methods are called using the class name, rather than an instance of the class.
1MyClass.my_class_method(arg1, arg2)
Examples of class methods:
A common use case for class methods is to create alternative constructors for a class. For example:
1class MyClass:
2 def __init__(self, arg1, arg2):
3 # Instance variables initialization
4
5 @classmethod
6 def from_string(cls, string):
7 # Parse string and create instance variables
8 return cls(arg1, arg2)
Use cases for class methods:
Create alternative constructors for a class.
Manage class-level variables.
Implement factory methods.
Static Methods
A static method is one that is part of the class itself, as opposed to an instance of the class. It is used to execute operations unrelated to specific instances of the class:
A static method is defined using the @staticmethod decorator.
It does not take any special first parameter like cls or self.
How to define a static method in Python:
1class MyClass:
2 @staticmethod
3 def my_static_method(arg1, arg2):
4 # Method body
How to call a static method:
Static methods are called using the class name, rather than an instance of the class.
1MyClass.my_static_method(arg1, arg2)
Examples of static methods:
A common use case for static methods is to create utility functions that are related to the class, but do not require any instance variables. For example:
1class MyClass:
2 @staticmethod
3 def is_valid_input(string):
4 # Check if input string is valid
5 return True/False
Use cases for static methods:
- Make utility functions related to the class but without the need for instance variables.
- Implement techniques that don’t alter the class’s state.
- Implement methods that can be used without first creating a class instance.
To sum up, both class methods and static methods are helpful tools for carrying out class-specific tasks. Despite some similarities, they serve different functions and have different usages. By understanding the differences between class methods and static methods, you can choose the appropriate method for your specific use case.
Differences between Class Methods and Static Methods
Class methods and static methods are both part of the class itself, but their functions and applications are distinct. The main distinctions between class methods and static methods are as follows:
- Syntax: Static methods are defined using the @staticmethod decorator and do not take a special first parameter, whereas class methods are defined using the @classmethod decorator and take the cls parameter as their first argument.
- Application: Static methods are used to carry out operations that are not specific to any one instance of the class, whereas class methods are used to carry out operations that are related to the class as a whole.
- Call: The class name is used to invoke class methods, and it is also used to invoke static methods.
- Purpose: Class methods are often used to create alternative constructors for a class or manage class-level variables, while static methods are often used to create utility functions that are related to the class, but do not require any instance variables.
- When deciding whether to use a class method or a static method, consider the purpose of the method and whether it is related to the class as a whole or to a specific instance of the class. Class methods are useful for managing class-level variables or creating alternative constructors, while static methods are useful for creating utility functions that do not require any instance variables.
In summary, class methods and static methods have different purposes and are used in different ways. By understanding the differences between class methods and static methods, you can choose the appropriate method for your specific use case.
Conclusion
In conclusion, class methods and static methods are crucial Python tools that let you carry out operations inside a class. You can control class-level variables, develop alternate constructors, and develop utility functions associated with the class by using class methods and static methods. In order to select the best method for your unique use case, it is crucial to comprehend the distinctions between class methods and static methods.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is the difference between class method and static method in Python?
In Python, the main distinction between a class method and a static method is that the former is used to carry out operations related to the class as a whole, whereas the latter is used to carry out operations unrelated to any particular instance of the class.
What is the difference between static and class method?
Both static methods and class methods are part of the class itself, but class methods are used for tasks pertaining to the class as a whole while static methods are used for tasks unrelated to any particular instance of the class.
When should I use static method in Python?
When you need to do something in Python that isn’t related to any particular instance of the class, like writing a utility function, you should use a static method.
What is the difference between static method and dynamic method in Python?
In Python, static and dynamic methods are two different things. Dynamic methods can be added to a class instance at runtime in contrast to static methods, which are methods that are part of the class itself.
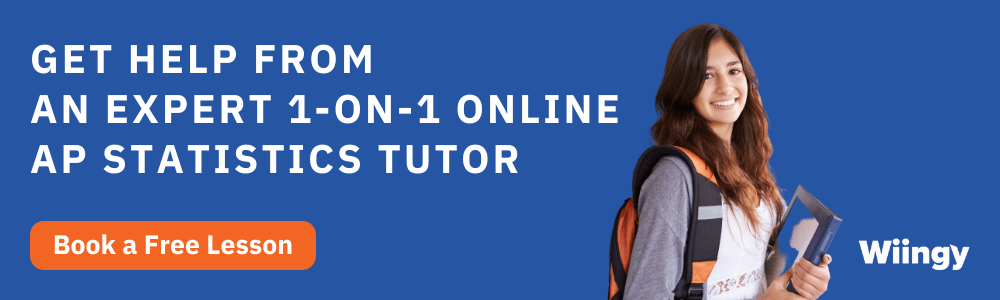
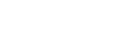
Jan 30, 2025
Was this helpful?