What are Python Classes and Objects?
Python is an object-oriented programming language, so classes and objects play a significant role in its functionality. In essence, a class serves as a template for building objects. It specifies a group of properties and operations that a class object may have. On the other hand, an object is a specific instance of a class.
It is essential to comprehend classes and objects in Python because they are a fundamental idea that is applied to almost every aspect of the language. Whether you’re writing a straightforward script or a sophisticated application, classes and objects will almost certainly be necessary.
In Python, classes and objects make it simpler to organize and manage code.You can write cleaner, more modular code that is simpler to maintain and modify by combining related functions and data. By grouping related functions and data together, you can create cleaner, more modular code that is easier to maintain and modify. Additionally, using classes and objects can help you avoid code duplication, which can save time and reduce errors.
Overall, understanding classes and objects in Python is essential for any developer who wants to write efficient, scalable, and maintainable code.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Classes and Objects in Python
The relationship between classes and objects in Python is simple. An object is an instance of a class, whereas a class serves as a template for building objects. To define a class in Python, you use the class keyword, followed by the class name and a colon. For example:
1class MyClass:
2 pass
To create an object from a class, you use the class name followed by parentheses. This will call the constructor method, which is a special method that initializes the object. For example:
1my_object = MyClass()
Once you have created an object, you can access its attributes and methods using dot notation. For example, if MyClass had an attribute called my_attribute and a method called my_method, you could access them like this:
1my_object.my_attribute
2my_object.my_method()
Here’s an example of a class and object in Python:
1class Dog:
2 def __init__(self, name, breed):
3 self.name = name
4 self.breed = breed
5
6 def bark(self):
7 print("Woof!")
8
9my_dog = Dog("Max", "Labrador")
10print(my_dog.name) # Output: Max
11my_dog.bark() # Output: Woof!
In this example, we define a Dog class with a constructor method that takes a name and breed parameter. We then create an object of the Dog class called my_dog with the name “Max” and breed “Labrador”. We then access the object’s name attribute using dot notation and call the bark() method.
Class Attributes and Methods
Classes in Python can also have class attributes and methods in addition to instance attributes and methods. Class methods are methods that are called on the class itself rather than on a specific instance of the class, whereas class attributes are attributes that are shared by all instances of the class.
To define a class attribute or method in Python, you use the @classmethod decorator. Class attributes are defined within the class body, while class methods are defined like regular methods, but with the @classmethod decorator. For example:
1class MyClass:
2 class_attribute = "Hello, world!"
3
4 @classmethod
5 def my_class_method(cls):
6 print(cls.class_attribute)
In this example, we define a class attribute called class_attribute with the value “Hello, world!”. We also define a class method called my_class_method() that prints the value of class_attribute.
To access a class attribute or call a class method, you can use either the class name or an instance of the class. For example:
1print(MyClass.class_attribute) # Output: Hello, world!
2MyClass.my_class_method() # Output: Hello, world!
3
4my_object = MyClass()
5print(my_object.class_attribute) # Output: Hello, world!
6my_object.my_class_method() # Output: Hello, world!
In this example, we access the class attribute and call the class method using both the class name and an instance of the class.
Here’s another example that demonstrates how to use class attributes and methods:
1class Circle:
2 pi = 3.14
3
4 def __init__(self, radius):
5 self.radius = radius
6
7 def get_circumference(self):
8 return 2 * self.pi * self.radius
9
10 @classmethod
11 def set_pi(cls, pi):
12 cls.pi = pi
13
14my_circle = Circle(5)
15print(my_circle.get_circumference()) # Output: 31.4
16
17Circle.set_pi(3.14159)
18print(my_circle.get_circumference()) # Output: 31.4159
In this example, we define a Circle class with a class attribute called pi and a constructor method that takes a radius parameter. We then define an instance method called get_circumference() that calculates the circumference of the circle. Finally, we define a class method called set_pi() that sets the value of pi.
We create an object of the Circle class called my_circle with a radius of 5. We then call the get_circumference() method to calculate the circumference of the circle, which is 31.4.
We then call the set_pi() class method to change the value of pi to 3.14159. We then call the get_circumference() method again to calculate the circumference of the circle with the new value of pi, which is 31.4159. Note that the value of pi is shared by all instances of the Circle class.
Python classes and objects are, in conclusion, fundamental concepts in object-oriented programming. You can write more efficient, scalable, and maintainable code if you know how to define classes, create objects, and use class attributes and methods. You ought to have a strong foundation for using Python classes and objects after reading this guide.
Instance Attributes and Methods
Python classes can also have instance attributes and methods in addition to class attributes and methods. While instance methods operate on particular instances of a class, instance attributes are attributes that are unique to each instance of a class.
In Python, you simply define the attribute or method inside the class and use the self keyword to refer to the instance when defining an instance attribute or method. For example:
1class Person:
2 def __init__(self, name, age):
3 self.name = name
4 self.age = age
5
6 def say_hello(self):
7 print(f"Hello, my name is {self.name} and I am {self.age} years old.")
In this example, we define a Person class with an instance attribute called name and age and an instance method called say_hello(). We use the self keyword to refer to the instance of the class when setting and accessing the instance attributes, and when calling the instance method.
To create an object with specific instance attributes, you simply pass the values as parameters to the class constructor. For example:
1person1 = Person("Alice", 25)
2person2 = Person("Bob", 30)
3
4person1.say_hello() # Output: Hello, my name is Alice and I am 25 years old.
5person2.say_hello() # Output: Hello, my name is Bob and I am 30 years old.
In this example, we create two objects of the Person class with different values for the name and age instance attributes. We then call the say_hello() instance method on each object to print a greeting.
Inheritance in Python
A key idea in object-oriented programming is inheritance, which enables you to build new classes off of pre existing ones. In Python, you can define a class that inherits from another class by specifying the parent class in parentheses after the class name. For example:
1class Animal:
2 def speak(self):
3 print("I am an animal.")
4
5class Dog(Animal):
6 def bark(self):
7 print("Woof!")
8
9my_dog = Dog()
10my_dog.speak() # Output: I am an animal.
11my_dog.bark() # Output: Woof!
In this example, we define a Animal class with an instance method called speak(). We then define a Dog class that inherits from Animal and has an instance method called bark(). We create an object of the Dog class called my_dog and call both the inherited speak() method and the bark() method.
You can also access the methods of the parent class from the child class using the super() function. For example
1class Animal:
2 def speak(self):
3 print("I am an animal.")
4
5class Dog(Animal):
6 def speak(self):
7 super().speak()
8 print("I am a dog.")
9
10 def bark(self):
11 print("Woof!")
12
13my_dog = Dog()
14my_dog.speak() # Output: I am an animal. I am a dog.
In this example, we override the speak() method in the Dog class, but we also call the speak() method of the parent class using super().speak(). This allows us to add behavior to the method while still retaining the original functionality.
Polymorphism in Python
Another key idea in object-oriented programming is polymorphism, which enables you to represent different types of objects using a single interface. Polymorphism is possible in Python through method overloading and inheritance.
Simply create a parent class with a method that takes a parameter, and then create child classes that override the method with various implementations to define polymorphic relationships between classes. For example:
1class Shape:
2 def area(self):
3 pass
4
5class Square(Shape):
6 def __init__(self, side):
7 self.side = side
8
9 def area(self):
10 return self.side ** 2
11
12class Circle(Shape):
13 def __init__(self, radius):
14 self.radius = radius
15
16 def area(self):
17 return 3.14 * self.radius ** 2
18
19my_square = Square(5)
20my_circle = Circle(3)
21
22print(my_square.area()) # Output: 25
23print(my_circle.area()) # Output: 28.26
In this example, we define a Shape class with an abstract area() method that does nothing. We then define two child classes, Square and Circle, that inherit from Shape and override the area() method with their own implementations. We create objects of each class, and call the area() method on each object to calculate the area of the shape.
You can also achieve polymorphism through method overloading, which allows you to define multiple methods with the same name but different parameters. However, method overloading is not supported natively in Python. Instead, you can use default parameters or variable-length argument lists to achieve similar functionality. Here’s an example:
1class Calculator:
2 def add(self, x, y=0):
3 return x + y
4
5 def multiply(self, *args):
6 result = 1
7 for arg in args:
8 result *= arg
9 return result
10
11my_calculator = Calculator()
12
13print(my_calculator.add(2, 3)) # Output: 5
14print(my_calculator.add(2)) # Output: 2
15print(my_calculator.multiply(2, 3, 4)) # Output: 24
In this example, we define a Calculator class with two methods, add() and multiply(). The add() method takes two parameters, x and y, but y has a default value of 0, allowing us to call the method with only one argument. The multiply() method uses a variable-length argument list (*args) to allow us to pass any number of arguments to the method.
Encapsulation in Python
Encapsulation is the practice of keeping an object’s internal components hidden and only revealing the information that is absolutely necessary to the outside world. Encapsulation is possible in Python thanks to access modifiers.
Access modifiers are keywords that determine the visibility and accessibility of class attributes and methods. There are three types of access modifiers in Python:
- Public: Public attributes and methods are accessible from anywhere in the program, both inside and outside the class. Public attributes and methods are not prefixed with an underscore.
- Private: Private attributes and methods are only accessible from within the class. Private attributes and methods are prefixed with a double underscore, like __attribute or __method.
- Protected: Protected attributes and methods are accessible from within the class and its subclasses. Protected attributes and methods are prefixed with a single underscore, like _attribute or _method.
Here’s an example of encapsulation in Python:
1class BankAccount:
2 def __init__(self, balance):
3 self.__balance = balance
4
5 def deposit(self, amount):
6 self.__balance += amount
7
8 def withdraw(self, amount):
9 if amount > self.__balance:
10 print("Insufficient funds.")
11 else:
12 self.__balance -= amount
13
14 def get_balance(self):
15 return self.__balance
16
17my_account = BankAccount(100)
18my_account.deposit(50)
19my_account.withdraw(75)
20print(my_account.get_balance()) # Output: 75
In this example, we define a BankAccount class with a private instance attribute called __balance and three instance methods called deposit(), withdraw(), and get_balance(). The deposit() method adds an amount to the balance, the withdraw() method subtracts an amount from the balance if there are sufficient funds, and the get_balance() method returns the current balance.
Because the __balance attribute is private, it cannot be accessed directly from outside the class. Instead, we use the deposit(), withdraw(), and get_balance() methods to interact with the object.
Real World Examples
Python classes and objects are used extensively in real-world programming to model real-world objects and systems. Here are a few examples:
- Creating a BankAccount class to represent individual accounts and using inheritance to create specialized account types like SavingsAccount or CheckingAccount is one way to model a bank account system.
- When simulating a university’s registration process, you could create a class called Student to represent each individual student while using inheritance to create different types of students, such as Graduate or Undergraduate.
- When modeling a game engine, you could make a class called GameObject to represent specific game elements, such as characters or items, and then use inheritance to make more specialized object types, such as PlayerCharacter or EnemyCharacter.
- Social media platform modeling: To represent individual users, you could create a User class, and you could use encapsulation to safeguard sensitive user information like passwords or email addresses.
Conclusion
Python classes and objects are effective tools for developing complex, scalable, and maintainable applications.
Understanding how to define classes, create objects, employ inheritance and polymorphism, and implement encapsulation will enable you to write more effective and efficient code.
By following best practices for object-oriented programming, you can create code that is easy to understand, modify, and extend.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are classes and objects in Python?
Classes and objects are fundamental concepts in object-oriented programming, according to A. In Python, a class is a blueprint or template for creating objects, while an instance of a class is an object.
What is the difference between object and class Python?
A class is a blueprint or template for constructing objects, while an instance of a class is an object. In other words, a class specifies the attributes and methods that an object can have, whereas an object is a particular instance of these attributes and methods.
What is __ init __ in Python?
__init__ is a special method in Python classes that is used to initialize the object’s attributes when it is created. It is called a constructor method and is automatically called when an object is created from a class.
What is the use of object () class in Python?
Python’s object() class is a built-in class that serves as the base class for all other classes. It defines the most fundamental methods and attributes that all Python objects must have.
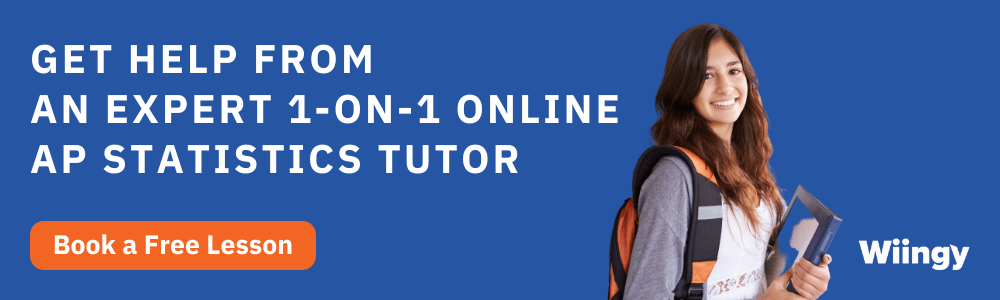
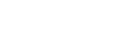
Jan 30, 2025
Was this helpful?