What is the NZEC Error in Python?
You might encounter an “NZEC” error message when running Python code. This denotes that the program did not exit with a successful status code and stands for “Non-Zero Exit Code.” The NZEC error can happen for a number of reasons, but it typically means that the program ran into an unexpected issue or error.
This article’s goal is to give readers a thorough study manual on Python’s NZEC error. The common causes of the NZEC error, methods for determining the error’s root cause, various debugging techniques to resolve the NZEC error, best practices for avoiding the NZEC error, other common Python errors, and the significance of comprehending the NZEC error and knowing how to effectively resolve it will all be covered.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
What Causes the NZEC Error in Python?
There are numerous causes for the NZEC error. The following are the most typical reasons for the Python NZEC error:
- Input/Output Errors: This error can happen if the program is given input that is not what it is supposed to be. For instance, if a program expects an integer input and a string is provided, it can cause the NZEC error.
- The NZEC error can be brought on by infinite loops, which are loops that never end and continue running indefinitely.
- Division by Zero is a mathematical mistake that can result in the NZEC error.
- The NZEC error can be brought on by a program using excessive amounts of memory.
- Incorrect Syntax – The NZEC error may be brought on by incorrect syntax in the code.
Here are some code examples that can cause the NZEC error:
1#Example 1: Infinite Loop
2while True:
3 print("Hello, world!")
4
5#Example 2: Division by Zero
6a = 10
7b = 0
8c = a/b
9print(c)
10
11#Example 3: Improper Syntax
12
13print("Hello, world!)
To identify the cause of the NZEC error, you can follow the steps below:
- Read the error message carefully – The error message can provide information about the cause of the error.
- Check the input – Ensure that the input provided to the program is as expected.
- Check the output – Ensure that the output generated by the program is as expected.
- Use print statements – Insert print statements in the code to help identify where the error occurs.
How to Debug the NZEC Error
Debugging is the process of finding and correcting code errors. The debugging methods listed below can be used to fix the NZEC error:
Print statements – Insert print statements in the code to help identify where the error occurs.
1#Example
2a = int(input())
3b = int(input())
4print(a+b)
5Try-except blocks - Use try-except blocks to catch and handle errors.
6python
7Copy code
8#Example
9try:
10 a = int(input())
11 b = int(input())
12 print(a/b)
13except ZeroDivisionError:
14 print("Cannot divide by zero")
Code optimization – Optimize the code to reduce memory usage and execution time.
1#Example
2#Inefficient code
3def fibonacci(n):
4 if n <= 1:
5 return n
6 else:
7 return fibonacci(n-1) + fibonacci(n-2)
8
9#Optimized code
10def fibonacci(n):
11 if n <= 1:
12 return n
13 else:
14 a, b = 0, 1
15 for i in range(n-1):
16 a, b = b, a + b
17 return b
How to Prevent the NZEC Error in Python
Writing clear and effective code requires taking steps to avoid the NZEC error. The following are some top tips for avoiding the NZEC error:
Verify the input – Always verify the input to the program to make sure it is in the desired format.
Avoid infinite loops – Ensure that there is a way for the program to exit the loop.
Use try-except blocks – Use try-except blocks to catch and handle errors.
Optimize the code – Optimize the code to reduce memory usage and execution time.
Write clean code – Ensure that the code is free of syntax errors and follows best practices.
Here are some code examples that are less prone to the NZEC error:
1#Example 1: Proper Input Handling
2try:
3 a = int(input())
4 b = int(input())
5 print(a+b)
6except ValueError:
7 print("Invalid input provided")
8
9#Example 2: Proper Exit Condition for Loops
10n = int(input())
11i = 1
12while i <= n:
13 print(i)
14 i += 1
15
16#Example 3: Clean Code
17def add_numbers(a, b):
18 return a + b
By following these best practices, you can reduce the likelihood of encountering the NZEC error in your Python code.
Other Common Errors in Python
There are other typical errors that you might run into while working with Python in addition to the NZEC error. Some of these errors include:
SyntaxError – This error occurs when there is a syntax error in the code.
NameError – This error occurs when a variable or function name is not defined.
TypeError – This error occurs when there is a type mismatch in the code.
ValueError – This error occurs when there is an invalid value provided to a function or variable.
You can apply methods similar to those used for the NZEC error to locate and fix these errors. To find and correct the error, carefully read the error message, examine the input and output, and employ debugging techniques.
Conclusion
In this article, we covered the Python NZEC error, its typical causes, how to recognize and troubleshoot it, best practices for avoiding it, other Python common errors, and the significance of comprehending the Python NZEC error and effectively resolving it. You can write clear, effective, and error-free code by using the advice and methods provided in this article. Always pay close attention to the error message, verify the input and output, and use debugging techniques to find and correct any mistakes in your code.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What does Python’s non-zero exit code mean?
Python’s non-zero exit codes indicate that the program did not terminate successfully. It means that the program encountered an unexpected problem or error during execution.
How to solve runtime error in Java in Codechef?
You can use the steps below to fix a runtime Java error in Codechef:
Try to determine the error’s cause by carefully reading the error message.
Implement debugging strategies like try-catch blocks, print statements, and code optimization.
Make sure the program is receiving accurate input.
Verify that the code is memory-optimized and look for memory leaks.
What is NZEC in Python?
NZEC stands for “Non-Zero Exit Code,” and it is an error that occurs when a Python program does not exit with a successful status code. It usually indicates that the program encountered an unexpected problem or error during execution
What is the full form of NZEC?
The full form of NZEC is “Non-Zero Exit Code.”
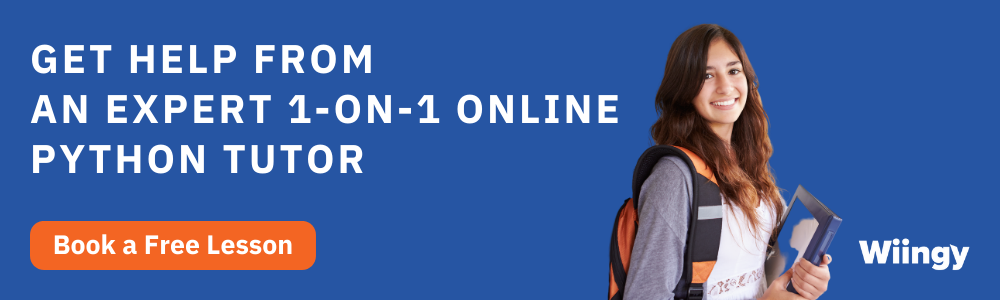
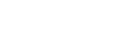
Jan 30, 2025
Was this helpful?