Python Tutorials
Python Functions aid in improving the organization, modularity, readability, debuggability, and maintenance of the code. They help us save time and effort by allowing us to reuse code rather than having to write the same code repeatedly for a program.
Functions also make it simpler to communicate functionality to other programmers because they give that information in a clear and concise manner.
A function in programming is a reusable section of code that completes a particular task. It enables you to call a group of statements by using the name of the function. Functions can take input values known as parameters and can also return output values.
Types of Functions in Python:
In Python, there are two types of functions – built-in functions and user-defined functions.
- Built-in functions: These are the functions that come with Python and are already defined for us. For example, print(), input(), len(), etc. These functions can be called directly without defining them first.
- User-defined functions: These are the functions that we define ourselves to perform a specific task. User-defined functions can be called multiple times in a program and can take input values known as parameters and can also return output values.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Defining Functions
A. Syntax:
In Python, the “def” keyword is used along with the function name and parentheses to define a function. The parentheses list any parameters that the function accepts. A colon (“:”) is then used to denote the beginning of the function body. The code that the function runs when called is contained in the body of the function.
Here’s the syntax for defining a function:
def function_name(parameter1, parameter2, ...): # function body
B. Examples:
Creating a Function without Any Parameters:
- In this example, we’ll define a function called “greet” that simply prints a greeting message.
def greet(): print("Hello, welcome to Python Functions!")
To call the “greet” function, we simply use the function name followed by parentheses:
greet()
Output:
Hello, welcome to Python Functions!
Creating a Function with Parameters:
- In this example, we’ll define a function called “add_numbers” that takes two parameters and returns their sum.
def add_numbers(num1, num2): sum = num1 + num2 return sum
To call the “add_numbers” function, we pass in two arguments as parameters:
result = add_numbers(5, 7) print(result)
12
Creating a Function with Parameters and Return Value:
- In this example, we’ll define a function called “calculate_area” that takes two parameters – length and width – and returns the area of a rectangle.
def calculate_area(length, width): area = length * width return area
To call the “calculate_area” function, we pass in two arguments as parameters:
result = calculate_area(10, 5) print(result)
50
C. Docstrings:
Function documentation is provided using docstrings. They are the first statement in a function definition and are enclose in triple quotes. There are two types of docstrings: single-line and multi-line.
Single-Line Docstring:
A single-line docstring is a concise description of the function and is enclosed in triple quotes on a single line immediately below the function definition.
Here’s an example:
def greet(name): """Prints a greeting message.""" print("Hello, " + name + "!")
Multi-Line Docstring:
A multi-line docstring provides a more detailed description of the function and can be used to specify the purpose of the function, its parameters, return values, and any exceptions that may be raised. It is enclosed in triple quotes and can span multiple lines.
Here’s an example:
def calculate_area(length, width): """ Calculates the area of a rectangle. Parameters: length (int): The length of the rectangle. width (int): The width of the rectangle. Returns: int: The area of the rectangle. """ area = length * width return area
Calling Functions
A. Syntax:
To call a function in Python, you simply use the function name followed by parentheses. If the function takes parameters, you include them inside the parentheses.
Here’s the syntax for calling a function:
function_name(argument1, argument2, ...)
B. Examples:
Calling a Function:
- In this example, we’ll call the “greet” function defined earlier, which takes a name parameter and prints a greeting message.
def greet(name): """Prints a greeting message.""" print("Hello, " + name + "!") greet("John")
Hello, John!
Calling a Function of a Module:
- Python comes with a set of built-in modules that contain functions that can be used in your program. To call a function from a module, you first need to import the module using the “import” keyword.
Here’s an example:
import math result = math.sqrt(25) print(result)
5.0
In this example, we first import the “math” module, which contains a function called “sqrt” that calculates the square root of a number. We then call the “sqrt” function with the argument 25 and store the result in the “result” variable. Finally, we print the value of “result”.
Return Value From a Function
A. Syntax:
To return a value from a function in Python, you use the “return” keyword followed by the value that you want to return.
Here’s the syntax for returning a value from a function:
def function_name(arguments): ... return value
B. Examples:
Return a Single Value:
In this example, we’ll define a function that takes two parameters and returns their sum.
def add_numbers(num1, num2): """Returns the sum of two numbers.""" return num1 + num2 result = add_numbers(10, 5) print(result)
15
Return Multiple Values:
In Python, you can return multiple values from a function by separating them with commas. The values are returned as a tuple.
Here’s an example:
def get_name_and_age(): """Returns a tuple containing name and age.""" name = "John" age = 30 return name, age result = get_name_and_age() print(result)
#Output
('John', 30)
Control Statements in Functions
A. The pass Statement:
Sometimes, you may want to define a function without adding any functionality to it. In such cases, you can use the “pass” statement, which does nothing.
Here’s an example:
def dummy_function(): pass
Scope and Lifetime of Variables
A. Local Variables in Function:
A variable defined inside a function is called a local variable. It can only be accessed within the function and its lifetime is limited to the duration of the function call.
Here’s an example:
def calculate_area(length, width): """Calculates the area of a rectangle.""" area = length * width print("The area of the rectangle is:", area) calculate_area(5, 10)
The area of the rectangle is: 50
In this example, the “area” variable is a local variable, which is defined inside the “calculate_area” function and can only be accessed within it.
B. Global Variables in Function:
A variable defined outside a function is called a global variable. It can be accessed both inside and outside the function.
Here’s an example:
message = "Hello, World!" def display_message(): """Displays the global message.""" print(message) display_message()
Hello, World!
Using the global Keyword in Function:
If you want to modify the value of a global variable inside a function, you need to use the “global” keyword to declare it as a global variable.
Here’s an example:
message = "Hello, World!" def change_message(): """Changes the value of the global message.""" global message message = "Hello, Python!" change_message() print(message) Output:
Hello, Python!
C. Nonlocal Variables in Function:
A variable defined in an enclosing function is called a nonlocal variable. It can be accessed and modified by the inner function.
Here’s an example:
def outer_function(): """Defines a nonlocal variable and an inner function.""" message = "Hello, World!" def inner_function(): """Modifies the value of the nonlocal variable.""" nonlocal message message = "Hello, Python!" inner_function() print(message) outer_function()
Hello, Python!
In this example, the “message” variable is defined in the outer function and is accessed and modified by the inner function using the “nonlocal” keyword. The output of the program is “Hello, Python!”, which confirms that the value of the nonlocal variable has been modified by the inner function.
Python Function Arguments:
A. Positional Arguments:
The most prevalent kind of arguments in Python are positional arguments. The arguments are matched based on position when you call a function.
Here’s an example:
def greet(name, message): """Greets the user with a custom message.""" print(f"{message}, {name}!") greet("John", "Hello") Output:
Hello, John!
In this example, the “name” and “message” arguments are positional arguments.
B. Keyword Arguments:
Keyword arguments are passed to a function using their names. This allows you to specify the arguments in any order, as long as you use their names.
Here’s an example:
def greet(name, message): """Greets the user with a custom message.""" print(f"{message}, {name}!") greet(message="Hello", name="John")
Hello, John!
In this example, the “name” and “message” arguments are keyword arguments.
C. Default Arguments:
Default arguments are used when you don’t provide a value for an argument. They are specified using the “=” operator.
Here’s an example:
def greet(name, message="Hello"): """Greets the user with a custom message.""" print(f"{message}, {name}!") greet("John")
In this example, the “message” argument has a default value of “Hello”, so we can call the function with only the “name” argument.
D. Variable-length Arguments:
Variable-length arguments allow you to pass a variable number of arguments to a function. They are specified using the “*” operator.
Here’s an example:
def add_numbers(*args): """Returns the sum of a variable number of arguments.""" result = 0 for num in args: result += num return result print(add_numbers(1, 2, 3)) print(add_numbers(1, 2, 3, 4, 5))
6 15
In this example, the “args” argument is a variable-length argument, which allows us to pass a variable number of arguments to the function.
E. Arbitrary Arguments:
Arbitrary arguments are similar to variable-length arguments, but they are specified using the “**” operator. They allow you to pass a variable number of keyword arguments to a function.
Here’s an example:
def print_info(**kwargs): """Prints the keyword arguments.""" for key, value in kwargs.items(): print(f"{key}: {value}") print_info(name="John", age=30, country="USA")
name: John age: 30 country: USA
In this example, the “kwargs” argument is an arbitrary argument, which allows us to pass a variable number of keyword arguments to the function.
Recursive Functions:
A. Definition:
A recursive function is a function that calls itself. It’s useful for solving problems that can be broken down into smaller sub-problems.
B. Examples:
def factorial(n): """Returns the factorial of n.""" if n == 0: return 1 else: return n * factorial(n-1) print(factorial(5))
120
In this example, the “factorial” function is a recursive function that calculates the factorial of a number.
Anonymous or Lambda Functions:
A. Definition:
An anonymous function is a function that is not bound to a name. In Python, anonymous functions are created using the “lambda” keyword.
B. Examples:
add = lambda x, y: x + y print(add(1, 2))
#Output
3
In this example, we have defined an anonymous function called “add” that takes two arguments and returns their sum. The function is called using the “add(1, 2)” syntax.
Lambda functions are commonly used with higher-order functions like “map”, “filter”, and “reduce” to perform operations on collections of data.
numbers = [1, 2, 3, 4, 5] squares = list(map(lambda x: x**2, numbers)) print(squares)
[1, 4, 9, 16,a 25]
In this example, we have used the “map” function with an anonymous function to calculate the squares of a list of numbers. The resulting squares are stored in a new list called “squares”.
Built-in Functions
A. The filter() Function:
The filter() function in Python is used to filter out elements from a given sequence based on a certain condition. It returns an iterator that contains the elements for which the given condition is True.
Syntax:
filter(function, sequence)
Example:
def is_even(num): return num % 2 == 0 numbers = [1, 2, 3, 4, 5, 6] even_numbers = list(filter(is_even, numbers)) print(even_numbers)
[2, 4, 6]
In this example, we have defined a function called “is_even” that returns True if a number is even. We then use the filter() function with this function and a list of numbers to create a new list that only contains the even numbers.
B. The map() Function:
The map() function in Python is used to apply a function to each element in a given sequence and returns an iterator containing the results.
Syntax:
map(function, sequence)
Example:
def square(num): return num**2 numbers = [1, 2, 3, 4, 5] squared_numbers = list(map(square, numbers)) print(squared_numbers)
[1, 4, 9, 16, 25]
In this example, we have defined a function called “square” that returns the square of a number. We then use the map() function with this function and a list of numbers to create a new list that contains the squares of each number in the original list.
C. The reduce() Function:
The reduce() function in Python is used to apply a function to a sequence of elements in a cumulative way and returns a single value.
Syntax:
reduce(function, sequence)
Example:
from functools import reduce def multiply(x, y): return x * y numbers = [1, 2, 3, 4, 5] product = reduce(multiply, numbers) print(product)
120
In this example, we have defined a function called “multiply” that returns the product of two numbers. We then use the reduce() function with this function and a list of numbers to calculate the product of all the numbers in the list.
Conclusion:
A. Summary of Python Functions:
Python functions are reusable blocks of code that complete a particular task. They can accept arguments and return values, and they are defined using the “def” keyword. Python provides several built-in functions, such as filter(), map(), and reduce(), that can be used to manipulate sequences of data.
B. Tips for Writing Good Functions:
- Use descriptive function names.
- Use comments to explain the purpose of the function and its parameters.
- Keep the function short and focused on a single task.
- Use meaningful variable names.
- Write test cases to ensure that the function works correctly.
C. Further Resources for Learning About Python Functions:
- Python documentation: https://docs.python.org/3/tutorial/controlflow.html#defining-functions
- Python for Everybody by Charles Severance: https://www.py4e.com/html3/04-functions
- Learn Python the Hard Way by Zed A. Shaw: https://learnpythonthehardway.org/book/ex18.html
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
1. What are the 4 types of functions in Python?
There are four types of functions in Python:
Built-in Functions
User-defined Functions
Anonymous/Lambda Functions
Recursive Functions
2. What are Python functions?
A function is a block of code that performs a specific task. It takes input, performs certain operations, and produces an output. In Python, a function is defined using the def keyword followed by the function name, parameters (if any), and a colon. The body of the function is indented and contains the code that performs the task.
3. What are five Python functions?
There are many built-in functions in Python, but five commonly used functions are:
print()
len()
range()
input()
type()
4. What are the top 5 functions in Python?
The top 5 functions in Python may vary depending on the context of their use. However, some of the commonly used functions are:
print()
len()
range()
input()
str()
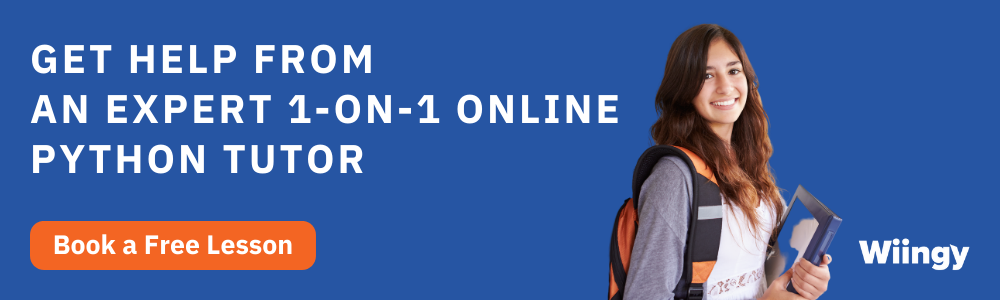
Written by by
Rahul LathReviewed by by
Arpit Rankwar