In this article
What is Inheritance in Python?
What is Inheritance in Python?
Types of Inheritance in Python
Syntax for Implementing Inheritance in Python
The Super() Function in Python
Overriding Methods in Python
Polymorphism in Python
Benefits of Using Inheritance in Python
Best Practices for Using Inheritance in Python
Conclusion
FAQs
What is Inheritance in Python?
In Python, inheritance is a key component of object-oriented programming (OOP). It is the mechanism by which a class can obtain the attributes and methods (properties) of another class. In essence, inheritance is the process of adding new properties to an existing class to create a new one.
By minimizing code duplication, inheritance facilitates code organization and increases code reusability.
As changes made to the base class automatically propagate to the derived class, it makes the code easier to maintain and modify.
Complex classes can be built on top of simpler classes thanks to inheritance.
It permits polymorphism, the capacity for objects to assume various forms.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
What is Inheritance in Python?
In Python, inheritance is described as the process of adding new properties to an existing class (the base class) to create a new class (the derived class). The base class’s attributes and methods can be accessed by the derived class as though they were defined there.
For instance, if we have a base class called “Animal” that has methods like eat() and sleep() as well as attributes like name, age, and weight, we can create a derived class called “Dog” that inherits these attributes and methods. In addition to the attributes and methods that were inherited from the base class, the derived class may also have some of its own.
Types of Inheritance in Python
The various inheritance types that Python supports are described below:
Single Inheritance:
Single inheritance is the most basic type of inheritance, where a derived class inherits properties from a single base class. Here is an example of single inheritance:
1class Animal:
2 def __init__(self, name, age):
3 self.name = name
4 self.age = age
5
6 def eat(self):
7 print("The animal is eating...")
8
9class Dog(Animal):
10 def bark(self):
11 print("Woof woof!")
12
13d = Dog("Rover", 3)
14print(d.name) # Output: Rover
15d.eat() # Output: The animal is eating...
16d.bark() # Output: Woof woof!
In this example, the class “Dog” is derived from the base class “Animal”. The derived class “Dog” inherits the attributes “name” and “age”, and the method “eat()” from the base class “Animal”. The derived class “Dog” also has its own method “bark()”.
Multiple Inheritance:
Multiple inheritance is a type of inheritance where a derived class inherits properties from two or more base classes. Here is an example of multiple inheritance:
1class Bird:
2 def fly(self):
3 print("The bird is flying...")
4
5class Mammal:
6 def run(self):
7 print("The mammal is running...")
8
9class Bat(Bird, Mammal):
10 pass
11
12b = Bat()
13b.fly() # Output: The bird is flying...
14b.run() # Output: The mammal is running…
In this example, the class “Bat” is derived from two base classes “Bird” and “Mammal”. The derived class “Bat” inherits the method “fly()” from the base class “Bird” and the method “run()” from the base class “Mammal”.
Multi-level Inheritance:
Multi-level inheritance is a type of inheritance where a derived class is created from another derived class. Here is an example of multi-level inheritance:
1class Animal:
2 def eat(self):
3 print("The animal is eating...")
4
5class Dog(Animal):
Syntax for Implementing Inheritance in Python
To implement inheritance in Python, we use the following syntax:
1class BaseClass:
2 # Attributes and methods of the base class
3
4class DerivedClass(BaseClass):
5 # Attributes and methods of the derived class
In this syntax, the derived class is created by inheriting from the base class using the syntax “class DerivedClass(BaseClass):”. The derived class can then access the attributes and methods of the base class as if they were defined within the derived class.
Here is an example of how to implement inheritance in Python:
1class Animal:
2 def __init__(self, name, age):
3 self.name = name
4 self.age = age
5
6 def eat(self):
7 print("The animal is eating...")
8
9class Dog(Animal):
10 def bark(self):
11 print("Woof woof!")
12
13d = Dog("Rover", 3)
14print(d.name) # Output: Rover
15d.eat() # Output: The animal is eating...
16d.bark() # Output: Woof woof!
In this example, the class “Dog” is derived from the base class “Animal”. The derived class “Dog” inherits the attributes “name” and “age”, and the method “eat()” from the base class “Animal”. The derived class “Dog” also has its own method “bark()”.
The Super() Function in Python
We can call a method from the base class using the built-in Python function super(). It is often used in method overriding to call the overridden method of the base class.
The syntax for using the super() function is as follows
super().method()
In this syntax, “super()” refers to the parent class, and “method()” refers to the method we want to call from the parent class.
Here is an example of using the super() function in Python:
1class Animal:
2 def eat(self):
3 print("The animal is eating...")
4
5class Dog(Animal):
6 def eat(self):
7 super().eat()
8 print("The dog is also eating...")
9
10d = Dog()
11d.eat() # Output: The animal is eating... \n The dog is also eating…
In this example, the class “Dog” overrides the method “eat()” of the base class “Animal”. The overridden method in the derived class calls the overridden method of the base class using the super() function.
Overriding Methods in Python
Redefining a method that was initially defined in the base class in the derived class is a process known as method overriding. The overridden method in the derived class is executed in place of the original method in the base class when the method is called on an object of the derived class.
Here is an example of method overriding in Python:
1class Animal:
2 def make_sound(self):
3 print("The animal makes a sound...")
4
5class Dog(Animal):
6 def make_sound(self):
7 print("The dog barks...")
8
9a = Animal()
10a.make_sound() # Output: The animal makes a sound...
11
12d = Dog()
13d.make_sound() # Output: The dog barks…
In this example, the class “Dog” overrides the method “make_sound()” of the base class “Animal”. When the method is called on an object of the derived class “Dog”, the overridden method in the derived class is executed instead of the original method in the base class.
Polymorphism in Python
Polymorphism is a crucial component of Python’s object-oriented programming. Objects can alter their appearance or behavior depending on the circumstance in which they are used. Python uses method overloading and overriding to implement polymorphism.
Method overriding is the process of a derived class providing its own implementation for a method that is already defined in the base class. But when a class has multiple methods with the same name but different parameter values, this is known as method overloading.
Here is an example of polymorphism in Python:
1class Animal:
2 def make_sound(self):
3 print("The animal makes a sound...")
4
5class Dog(Animal):
6 def make_sound(self):
7 print("The dog barks...")
8
9class Cat(Animal):
10 def make_sound(self):
11 print("The cat meows...")
12
13def animal_sounds(animal):
14 animal.make_sound()
15
16a = Animal()
17d = Dog()
18c = Cat()
19
20animal_sounds(a) # Output: The animal makes a sound...
21animal_sounds(d) # Output: The dog barks...
22animal_sounds(c) # Output: The cat meows…
In this example, the function “animal_sounds()” takes an object of the base class “Animal” as an argument. When the function is called with objects of the derived classes “Dog” and “Cat”, the overridden method in the derived class is executed instead of the original method in the base class.
Benefits of Using Inheritance in Python
There are several advantages to using inheritance in Python programming, including:
- Reusable code: By allowing developers to reuse code from pre-existing classes, inheritance lowers the amount of new code that must be written.
- Using inheritance, developers can structure their code into a hierarchy of related classes, which makes it simpler to understand and modify.
- Code duplication is decreased by inheritance, which enables the construction of complex classes on top of simpler classes.
- Simple maintenance: Modifications to the base class automatically affect the derived class, simplifying code maintenance.
Best Practices for Using Inheritance in Python
It’s crucial to adhere to these best practices in order to use inheritance in Python effectively:
- When used excessively, inheritance can result in code that is complex and challenging to maintain. Only use inheritance when it makes sense and helps to organize and reuse code.
- Avoid having deep hierarchies: Deep inheritance hierarchies can be challenging to understand and maintain. Try to keep inheritance hierarchies shallow.
- Avoid circular dependencies: Be careful not to create circular dependencies between classes. This can cause problems with import statements and can make code difficult to understand and maintain.
- Use abstract classes and interfaces: Abstract classes and interfaces can help to define the structure and behavior of classes in an inheritance hierarchy, making the code easier to understand and maintain.
Understanding inheritance is a significant step in mastering Python’s object-oriented programming. To further enhance your skills, consider exploring personalized python tutoring online. Wiingy connects students with experienced tutors who can provide one-on-one guidance, helping you delve deeper into concepts like inheritance, polymorphism, and beyond
Conclusion
Python object-oriented programming is dependent on inheritance. It makes code easier to maintain and modify by enabling developers to create new classes by inheriting or acquiring the properties of existing classes. We talked about what inheritance is, how it works in Python, the different kinds of inheritance, how to implement inheritance using syntax, how to override methods, and more.
In Python programming, inheritance is a fundamental idea that newcomers must grasp in order to use it effectively. By building complex classes on top of simpler classes using inheritance, programmers can eliminate duplication of code and enhance code organization.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is Inheritance in Python?
In Python, inheritance is a mechanism that allows one class to inherit or acquire the properties of another. It allows for the creation of new classes by reusing existing code.
What are the 5 types of inheritance in Python?
Single inheritance, multiple inheritance, multi-level inheritance, hierarchical inheritance, and hybrid inheritance are the five types of inheritance in Python.
What is init in Python?
When an object is created in Python, the init method is called. It is employed to set the object’s attributes to zero.
What is inheritance and multiple inheritance in Python?
Adding properties from an existing class to a new class is known as inheritance in Python. Multiple inheritance refers to a derived class that has properties from two or more base classes.
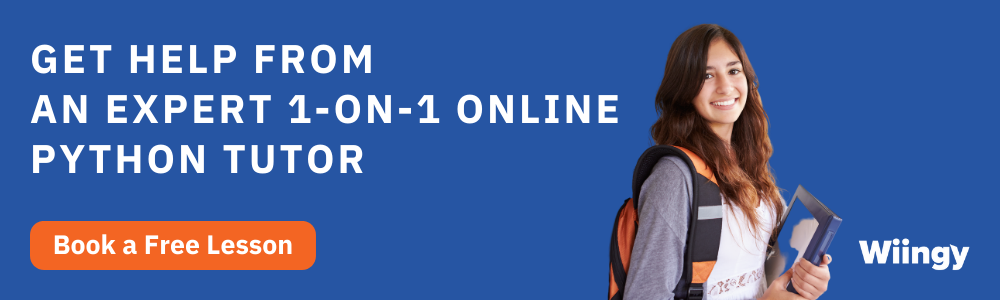
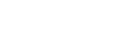
Apr 15, 2025
Was this helpful?