The global keyword is one of the fundamental concepts in Python, allowing programmers to access and modify global variables within functions and other scopes. In this article, we will examine the syntax, behavior, and applications of the global keyword in Python. Understanding the global keyword is crucial for writing robust and efficient Python code, and it can help you avoid common programming errors and improve your skills.
Explanation of global keyword:
Python’s global keyword is a reserved word that can be used to declare a variable within a function or local scope as a reference to a global variable. Any variable declared inside a function is assumed to be a local variable whose scope is restricted to the function itself and which can’t be accessed or modified from outside the function. However, the global keyword must be used when declaring a variable for global use.
Importance of understanding global keyword:
The global keyword allows you to access and modify global variables within different scopes without introducing naming conflicts or unintended side effects, which makes understanding it crucial for writing accurate and effective Python code. Furthermore, by allowing you to reuse a variable across various functions and modules, using global variables can help your code become simpler and more modular. Nevertheless, using global variables carries some risks and trade-offs, such as lowering your code’s readability and maintainability or making it more challenging to test and debug.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
What is Global Keyword in Python?
A variable declared within a function or a local scope may refer to a global variable rather than a local variable by using the global keyword in Python. The syntax of using global keyword is as follows:
1global variable_name
This statement tells Python that the variable_name refers to a global variable, not a local variable. If the variable_name doesn’t already exist in the global scope, Python will make it.
How global keyword works?
The global keyword instructs Python to look for the variable name in the global scope rather than the local scope when used within a function or a local scope. The variable can be accessed and changed from within the function if it is found in the global scope. If the variable is not already present in the global scope, Python will create it there and initialize it with None by default. Here is an example of using global keyword:
1x = 10 # global variable
2def foo():
3 global x
4 x = 20 # modifying global variable
5foo()
6print(x) # prints 20
In this example, we define a global variable x with a value of 10. Then, we define a function foo() that modifies the value of x using the global keyword. When we call foo(), it changes the value of x to 20, and when we print x outside the function, it prints 20, because x is now a global variable.
Examples of global keyword in Python:
Example of using global keyword in a function:
1count = 0 # global variable
2def increment():
3 global count
4 count += 1
5
6increment()
7print(count) # prints 1
In this example, we define a global variable count with a value of 0. Then, we define a function increment() that uses the global keyword to increment the value of count by 1. When we call increment(), it changes the value of count to 1, and when we print count outside the function, it prints 1, because count is now a global variable.
Example of using global keyword outside a function:
1def foo():
2 global x
3 x = 10 # defining global variable
4
5foo()
6print(x) # prints 10
In this example, we define a function foo() that defines a global variable x with a value of 10, using the global keyword. When we call foo(), it creates a global variable x and initializes it with the value of 10. When we print x outside the function, it prints 10, because x is now a global variable.
These examples show how the global keyword can be used to define and alter global variables within various scopes, allowing you to create Python code that is more adaptable and reusable. Nevertheless, using global variables has some drawbacks and risks, such as the potential for unintended consequences and increased difficulty in maintaining and understanding the code. Therefore, when working with global variables in Python, it’s crucial to use the global keyword sparingly and adhere to some best practices.
When to use Global Keyword in Python
The global keyword should only be used when absolutely necessary because overuse can result in difficult-to-read and maintainable code. The global keyword should be used whenever we need to access or modify a global variable inside of a function. The following are a few typical scenarios in which the global keyword is helpful:
When global keyword is necessary:
Instead of creating a new local variable with the same name when we need to change the value of a global variable inside of a function, we must use the global keyword to indicate that we want to change the global variable.
1count = 0 # global variable
2
3def increment():
4 global count
5 count += 1
6
7increment()
8print(count) # prints 1
Accessing a global variable in a function:
When we need to access the value of a global variable inside a function, we can use the variable directly, without the global keyword. However, if we want to modify the variable, we have to use the global keyword to indicate that we want to modify the global variable.
1count = 0 # global variable
2
3def print_count():
4 print(count)
5
6def increment():
7 global count
8 count += 1
9
10increment()
11print_count() # prints 1
When not to use Global Keyword
Reducing readability:
Because it is not always obvious where a variable is defined and how it is used, using global variables can make the code more difficult to read and comprehend. In general, it is better to pass arguments between functions and use local variables because it is simpler to follow the data flow and comprehend the logic of the code.
Creating unintended consequences:
The use of global variables can also result in unintended side effects, where a change in one area of the code has unexpected effects on other areas of the code. This may make it more difficult to maintain and debug the code. In general, it is preferable to use local variables or other methods rather than global variables whenever possible.
Alternatives to Global Keyword
In Python, we have a number of alternatives to using global variables that can help us avoid any potential issues. Here are some common techniques:
Using function arguments and return values:
To transfer data between various sections of the code, we can pass arguments between functions and return values from functions rather than global variables. Because it is now clear where the data is coming from and going to, the code is now simpler to read and comprehend.
1def increment(count):
2 count += 1
3 return count
4
5count = 0
6count = increment(count)
7print(count) # prints 1
Using classes and objects:
Using classes and objects, one can represent intricate data structures and systems by encapsulating data and behavior in a self-contained unit. We can prevent the use of global variables, keep the code more streamlined, and maintain modularity by defining methods that operate on the data of the object.
1class Counter:
2 def __init__(self):
3 self.count = 0
4
5 def increment(self):
6 self.count += 1
7
8c = Counter()
9c.increment()
10print(c.count) # prints 1
Using closures:
With the aid of closures, functions can be written that retain the contents of the scopes they are enclosed in even after they have finished running. We can avoid using global variables while still allowing data to be shared between different sections of the code by using closures.
1def counter():
2 count = 0
3 def increment():
4 nonlocal count
5 count += 1
6 return count
7 return increment
8
9c = counter()
10print
Best Practices
Proper usage of Global Keyword:
Use the global keyword sparingly and only when absolutely necessary. It should not be used to create new global variables or to access global variables outside of functions; instead, it should be used to modify or access global variables inside of functions. It’s crucial to ensure that the code is clear and understandable when using the global keyword and to be mindful of any potential negative effects or unintended consequences.
Best practices for maintaining code readability:
Local variables and function arguments should be used instead of global variables whenever possible to maintain code readability. Additionally, it is crucial to avoid naming conflicts, use descriptive and clear variable names, and logically group related functions and data. These best practices can help us write more readable, maintainable, and debuggable code.
Pitfalls to avoid:
Using global variables frequently leads to unintended side effects, naming conflicts, and more difficult-to-read and-understand code, among other common pitfalls. The global keyword should be used sparingly, global variables should not be used outside of functions, and variable names should be simple and descriptive in order to avoid these pitfalls.
Conclusion
The global keyword in Python, its definition, practical applications, and potential pitfalls have all been covered in this article. We have also looked into alternatives to global variables, such as classes and objects, closures, and function arguments and return values. We’ve covered the ideal ways to use the global keyword while preserving code readability.
For code to be simple to read, maintain, and debug, the global keyword must be used properly. We can write more reliable, adaptable, and scalable code by employing global variables sparingly and only when absolutely necessary, as well as by adhering to best practices for preserving readability.
The global keyword is a potent tool for transferring data between various components of a program, but it must be used with caution. We can write code that is simpler to read, comprehend, and maintain by adhering to best practices and avoiding common pitfalls. It is crucial to keep these factors in mind and aim for code that is both functional and elegant as we continue to create new Python programs and applications.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is global keyword in Python?
Python uses the global keyword to designate a variable as a global variable, allowing access and modification from anywhere in the program.
Is global keyword needed in Python?
Python does not always require the global keyword, but it can be helpful for accessing or changing global variables inside of functions.
What is local and global in Python?
Local variables in Python are variables that are declared inside a function and are only accessible from within that function. On the other hand, global variables are those that are defined outside of any function and can be used by any part of the program.
What is the difference between global and nonlocal keywords in Python?
A variable is designated as a global variable by the keyword global, whereas a variable is designated as a nonlocal variable by the keyword nonlocal, meaning that it is defined in an enclosing function and can be accessed and modified by nested functions.
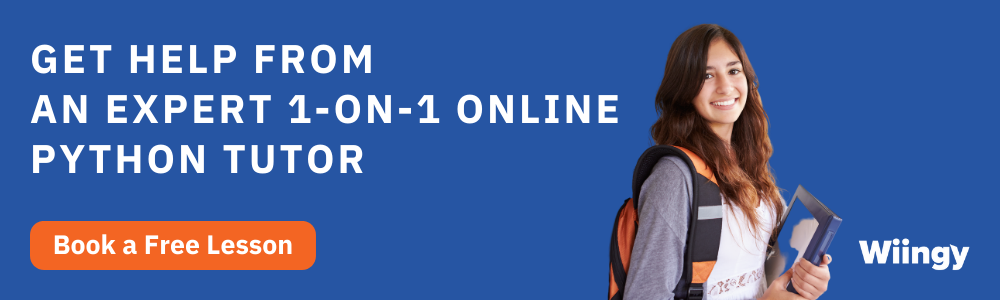
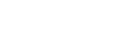
Jan 30, 2025
Was this helpful?