What are Python Arrays?
A collection of identically data typed elements are kept together in contiguous memory locations by an array, a type of data structure. These elements can be accessed by either a subscript value or an index. The size of an array is fixed at the time of creation and cannot be changed dynamically.
A. Benefits of using arrays
Large amounts of data can be stored and handled conveniently and effectively with the help of arrays. Using index-based operations, they enable simple access to and modification of individual elements. Additionally, because they are designed for sequential access, arrays perform some operations more quickly than other data structures.
B. Basic structure of an array
An array’s basic building block is a group of elements, each of which is denoted by an index or a subscript value. The index starts from 0 and goes up to the size of the array minus one. An array can only contain elements of the same data type.
For example, the following code creates an array of integers with five elements:
1# Python Arrays snippet for 5 Elements
2my_array = [1, 2, 3, 4, 5]
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Differences between Lists and Arrays
Lists are used to group items together that typically include components from various data types. Another essential element that collects multiple instances of the same data type is an array. List is unable to handle mathematical operations. The array can control mathematical operations.
List structure and functionality
Another type of data structure in Python that can hold a group of elements is a list. A list’s size can be adjusted dynamically, unlike arrays. Lists can have elements of various data types and are created using square brackets.
1my_list = [1, 'hello', 3.14, True]
Array structure and functionality
Arrays, on the other hand, have a fixed size and can only store elements of the same data type. They are created using the array module in Python.
1import array my_array = array.array('i', [1, 2, 3, 4, 5])
Comparing Lists and Arrays
Lists and arrays each have advantages and drawbacks of their own. Lists are more adaptable and can accommodate items of various sizes and data types. Arrays, on the other hand, use less memory and process information faster. Generally speaking, arrays are a better option if you need to work with large amounts of data that are all the same type. Lists are the way to go if you need to store data of various sizes or types.
Array Representations
A. One-dimensional arrays
The simplest kind of arrays are one-dimensional ones, which have just one row of elements. Each element’s index reflects its location within the array.
1my_array = array.array('i', [1, 2, 3, 4, 5]) print(my_array[0]) # Output: 1 print(my_array[4]) # Output: 5
B. Multidimensional arrays
Multidimensional arrays consist of multiple rows and columns of elements. They are useful for storing and manipulating data in a tabular format. In Python, you can create multidimensional arrays using nested lists or the numpy library.
1# Using nested lists
2my_array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
3print(my_array[0][0])
4# Output: 1
5print(my_array[2][2])
6# Output: 9
7
8# Using numpy
9import numpy as np
10my_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) print(my_array[0][0])
11# Output: 1
12print(my_array[2][2])
13# Output: 9
When to use Python Arrays
A. Use cases for arrays
When you need to store a group of elements with the same data type and perform operations on them, arrays come in handy. Some common use cases for arrays include:
- Storing and manipulating data in a tabular format, such as in spreadsheets or databases
- Implementing algorithms that require sequential access to data, such as sorting or searching
- Storing large amounts of data in a way that is efficient and easy to access
B. Advantages of arrays over other data structures
Arrays have several advantages over other data structures:
- They allow for efficient random access to elements
- They are optimized for sequential access, making them faster than other data structures for certain types of operations
- They have a fixed size, which can help prevent memory errors and improve performance
- They are easy to implement and use in most programming languages
C. When not to use arrays
Python Arrays may not be the best choice for all situations. Some cases where other data structures may be more appropriate include:
- When you need to store data of different data types or sizes
- When you need to add or remove elements dynamically
- When memory usage is a concern and you have limited memory available
How to use Python Arrays
Here’s how to use arrays in python:
In Python, you can define an array using either a list or the array module. Here is an example of how to define an array using the array module:
1array my_array = array.array('i', [1, 2, 3, 4, 5])
Complexities of arrays
Arrays have several complexities that you should be aware of:
- They have a fixed size, which means that you cannot add or remove elements once the array has been created.
- Indexing and searching can be slow for very large arrays.
- Arrays require contiguous memory, which can make them difficult to work with in certain situations.
Find the length of arrays
To find the length of an array in Python, you can use the len() function. Here is an example:
import array my_array = array.array(‘i’, [1, 2, 3, 4, 5]) print(len(my_array)) # Output: 5
Array Indexing in Python
A. Basic indexing
The process of accessing individual elements within an array using their index is known as array indexing. In Python, array indexing starts at 0. Here is an example:
1import array my_array = array.array('i', [1, 2, 3, 4, 5]) print(my_array[0]) # Output: 1 print(my_array[4]) # Output: 5
B. Advanced indexing
You can choose elements from an array using Boolean or integer arrays thanks to advanced indexing. Here is an example:
1import numpy as np my_array = np.array([1, 2, 3, 4, 5])
2bool_array = np.array([True, False, True, False, True])
3print(my_array[bool_array])
4# Output: [1, 3, 5]
C. Slicing an array
Slicing allows you to select a range of elements from an array. Here is an example:
1import array my_array = array.array('i', [1, 2, 3, 4, 5])
2print(my_array[1:4])
3# Output: [2, 3, 4]
Search through Python Arrays
A. Linear search
The linear search algorithm is a simple method for navigating an array. It involves iterating through each element of the array to see if they all match the search value. Here is an example implementation of linear search in Python:
1def linear_search(arr, search_value): for i in range(len(arr)): if arr[i] == search_value: return i return -1
B. Binary search
A sorted array can be searched through more quickly with binary search. It entails repeatedly halving the array and determining whether the middle element corresponds to the search value. Here is an example implementation of binary search in Python:
1def binary_search(arr, search_value): low = 0 high = len(arr) - 1 while low <= high: mid = (low + high) // 2 if arr[mid] == search_value: return mid elif arr[mid] < search_value: low = mid + 1 else: high = mid - 1 return -1
C. Other search algorithms
Other search algorithms for arrays include interpolation search, exponential search, and jump search. These algorithms are more specialized and may not be appropriate for all situations.
Loop through Python Arrays
A. Basic iteration
The fundamental iteration process entails looping through every element of the array and applying a specific operation to each element. Here is an example implementation of basic iteration in Python:
1my_array = [1, 2, 3, 4, 5] for i in range(len(my_array)): print(my_array[i])
B. Advanced iteration
Using built-in Python functions or libraries to manipulate the array is referred to as advanced iteration. Here is an example implementation of advanced iteration using the NumPy library:
1import numpy as np my_array = np.array([1, 2, 3, 4, 5]) for elem in np.nditer(my_array): print(elem)
C. Examples of loop-based operations
Loop-based operations can be used to perform a variety of operations on arrays, including:
- Adding all the elements in an array together
- Finding the minimum or maximum value in an array
- Reversing the order of elements in an array
Array Methods for performing operations
A. Change an existing value
To change an existing value in an array, you can use indexing to access the element you want to change and then assign a new value to it. Here is an example:
1my_array = [1, 2, 3, 4, 5] my_array[0] = 6 print(my_array)
2# Output: [6, 2, 3, 4, 5]
B. Add a new value
To add a new value to an array, you can use the append() method. Here is an example:
1my_array = [1, 2, 3, 4, 5] my_array.append(6) print(my_array) # Output: [1, 2, 3, 4, 5, 6]
C. Remove a value
To remove a value from an array, you can use the remove() method. Here is an example:
1my_array = [1, 2, 3, 4, 5] my_array.remove(3) print(my_array) # Output: [1, 2, 4, 5]
D. Other array methods
Other useful array methods in Python include:
- sort(): sorts the elements in the array in ascending order.
1my_array = [5, 3, 1, 4, 2] my_array.sort() print(my_array) # Output: [1, 2, 3, 4, 5]
- index(): returns the index of the first occurrence of a given element in the array.
1my_array = [1, 2, 3, 4, 5] index_of_three = my_array.index(3) print(index_of_three) # Output: 2
- count(): returns the number of times a given element appears in the array.
1my_array = [1, 2, 3, 3, 4, 5] count_of_threes = my_array.count(3) print(count_of_threes) # Output: 2
- extend(): appends the elements of another array to the end of the current array.
1my_array = [1, 2, 3, 4, 5] new_array = [6, 7, 8, 9, 10] my_array.extend(new_array) print(my_array) # Output: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
- reverse(): reverses the order of elements in the array.
1my_array = [1, 2, 3, 4, 5] my_array.reverse() print(my_array) # Output: [5, 4, 3, 2, 1]
The use of powerful data structures like arrays in a variety of programming tasks is widespread. Students can build solid foundational knowledge in Python programming by grasping the fundamentals of arrays and knowing how to use them efficiently.
Conclusion
In conclusion, arrays are a crucial data structure in Python that enable effective data storing and retrieval. Despite having some advantages over lists in terms of performance and memory usage, they are similar to lists. Applications for python arrays can range from straightforward calculations to intricate data analysis.
Understanding the fundamental syntax and operations is crucial when working with python arrays. It is also helpful to understand the different types of arrays and their uses. Additionally, performance can be significantly enhanced by using the right search and iteration algorithms. Finally, it is important to pay attention to memory usage and avoid creating unnecessarily large arrays..
Python arrays can be learned more about using a variety of resources. The official Python documentation, online tutorials, courses, and programming forums and communities are a few useful resources.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are arrays in Python?
Arrays are a data structure in Python that allow for efficient storage and retrieval of data. They are similar to lists, but have some advantages in terms of performance and memory usage.
What are the 4 types of array in Python?
The four types of python arrays are one-dimensional arrays, multidimensional arrays, jagged arrays, and dynamic arrays.
How to make arrays in Python?
To create an array in Python, you can use the array module or create a list and convert it to an array using the array() method.
What are good arrays in Python?
Good arrays in Python are those that are appropriately sized for the data being stored and accessed efficiently. Additionally, using appropriate algorithms and methods can greatly improve performance.
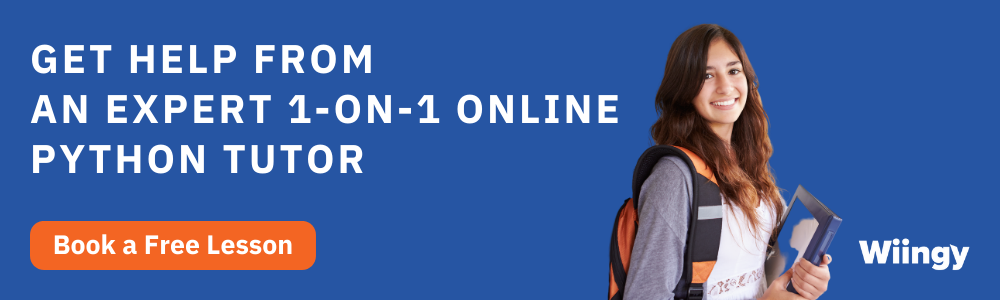
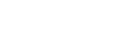
Jan 30, 2025
Was this helpful?