Python Tutorials
Problem-solving is at the heart of programming, and control flow in python is a key idea that facilitates efficient problem-solving. Simply put, control flow describes the sequence in which statements are carried out within a program.
It enables programmers to specify how the program ought to act in response to specific circumstances and take appropriate action. Python uses control flow, a fundamental idea in programming that is present in almost all other programming languages as well.
What is Control Flow in Python?
The order in which statements are carried out by a program is referred to as control flow. It is a fundamental concept that allows programmers to control the execution of their code based on certain conditions. Control flow in Python is achieved through various constructs such as if-else statements, loops, and functions.
Importance of Control Flow in Programming:
Control flow is an essential concept in programming as it allows developers to write programs that can make decisions based on certain conditions. This makes programs more flexible and adaptable, enabling them to handle different scenarios effectively. Control flow constructs also make code more readable and easier to maintain.
Basic Concepts of Control Flow:
There are several basic concepts of control flow that are important to understand. These include conditions, loops, functions, and branching. Conditions are used to test if a certain condition is true or false, while loops are used to repeat a block of code multiple times. Functions are used to break code into reusable pieces, and branching is used to redirect the flow of execution based on certain conditions.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Sequential Control Flow
Statements in a program are said to follow a sequential control flow when they are executed in the order specified by the programmer. In other words, each statement is executed sequentially, and the order in which they are written affects how they are executed.
Definition:
Sequential control flow is the default mode of execution in a program. It refers to the sequential execution of statements, where each statement is executed one after the other.
Examples:
Consider the following code:
x = 5 y = 10 z = x + y print(z)
In this example, the code is executed sequentially. First, the value of x is set to 5, then the value of y is set to 10, then the sum of x and y is calculated and stored in z, and finally, the value of z is printed to the console.
Default mode:
Sequential control flow is the default mode of execution in a program, and it is used in most cases. However, it is not always the most efficient or effective way to solve problems, especially when dealing with complex programs or large amounts of data. In these cases, other control flow constructs such as loops and functions are used to improve the efficiency and effectiveness of the code.
Selection Control Flow
Selection control flow is a type of control flow that is used to make decisions and branching in a program. It allows the program to choose between different paths of execution based on certain conditions.
Definition:
Selection control flow is a type of control flow that allows the program to choose between different paths of execution based on certain conditions. It is achieved through constructs such as if-else statements and switch statements.
Examples:
Consider the following code:
x = 5 if x > 10: print("x is greater than 10") else: print("x is less than or equal to 10")
In this example, the program checks whether the value of x is greater than 10. If it is, it prints the message “x is greater than 10.” Otherwise, it prints the message “x is less than or equal to 10.”
Used for decisions and branching:
Programming decisions and branches can be implemented with the help of selection control flow. When conditions are met, the program can move on to the next section of code. This is helpful when tackling complicated issues that call for a variety of responses depending on the specifics of the situation.
Repetition Control Flow
A specialized form of control flow called “repetition” is employed when it’s necessary to run the same piece of code multiple times. It allows the program to automate repetitive tasks and perform them more efficiently.
Definition:
Repeatation control flow is a type of control flow that is employed to repeatedly execute a block of code. It is achieved through constructs such as for loops and while loops.
Examples:
Consider the following code:
for i in range(5): print(i)
In this example, the program uses a for loop to print the values of i from 0 to 4. The code inside the for loop is executed five times, with the value of i changing each time.
Another example:
x = 1 while x <= 10: print(x) x += 1
In this instance, the program prints the values of x from 1 to 10 using a while loop. While the condition x = 10 is true, the code inside the while loop is run repeatedly.
When dealing with tasks that need to be repeated repeatedly, repetition control flow is helpful. It enables the program to more effectively complete repetitive tasks by automating them.
Operators Used in Control Flow
A fundamental idea in programming, operators are heavily utilized in control flow constructs. There are several types of operators used in control flow, including comparison operators, boolean operators, and mixing operators.
Comparison Operators
Comparison operators are used to compare two values and return a boolean value (True or False) based on the comparison result. The syntax for comparison operators in Python is:
- > : Greater than
- < : Less than
- >= : Greater than or equal to
- <= : Less than or equal to
- == : Equal to
- != : Not equal to
Examples:
x = 5 y = 10 print(x > y) # False print(x < y) # True print(x == y) # False print(x != y) # True
Boolean Operators
Multiple boolean values can be combined using boolean operators to produce a boolean result. The syntax for boolean operators in Python is:
- and : Returns True if both operands are True
- or : Returns True if at least one operand is True
- not : Returns the opposite boolean value of the operand
Examples:
x = 5 y = 10 print(x > 2 and y < 20) # True print(x < 2 or y > 20) # False print(not x > y) # True
Mixing Operators
To create more complex conditions, mixing operators combine boolean and comparison operators. The syntax for mixing operators in Python is:
- and : Returns True if both conditions are True
- or : Returns True if at least one condition is True
Examples:
x = 5 y = 10 z = 15 print(x < y and y < z) # True print(x > y or y < z) # True
Conditional Statements
Control flow employs conditional statements to execute specific code blocks in response to predetermined circumstances. There are several types of conditional statements in Python, including if statements, ternary conditional operator, and switch-case statements.
If Statements
If statements are used to execute a block of code if a certain condition is True. The syntax for if statements in Python is:
if condition: # Code to execute if condition is True
Logical Operators
Multiple conditions can be combined in if statements using logical operators. The logical operators in Python are:
- and : Returns True if both conditions are True
- or : Returns True if at least one condition is True
- not : Returns the opposite boolean value of the operand
Nested If Statements
If statements that are nested inside of one another are known as nested if statements. When several conditions must be verified in a particular sequence, they are used.
Ternary Conditional Operator
The ternary conditional operator is a shorthand way of writing if-else statements. It is used when a single statement needs to be executed based on a specific condition. The syntax for the ternary conditional operator in Python is:
result = value_if_true if condition else value_if_false
Examples:
x = 5 y = 10 result = "x is less than y" if x < y else "x is greater than or equal to y" print(result) # x is less than y
Switch-Case Statement
Using the switch-case statement, various blocks of code can be run depending on various values.
Loop Statements
To repeatedly run a block of code, loop statements are used in control flow. There are two types of loop statements in Python: while loops and for loops.
While Loop Statements
While loop statements are used to repeat a block of code as long as a certain condition is True. The syntax for while loop statements in Python is:
while condition: # Code to execute while condition is True
Examples:
x = 1 while x <= 10: print(x) x += 1
For Loop Statements
For loop statements are used to repeat a block of code for each element in a sequence. The syntax for for loop statements in Python is:
for variable in sequence: # Code to execute for each element in sequence
Examples:
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
The range() function:
The range() function is used in for loops to generate a sequence of numbers. The syntax for the range() function in Python is:
range(start, stop, step)
For Else Statement
The for-else statement is used to execute a block of code when a for loop has finished iterating through all the elements in a sequence. The syntax for the for-else statement in Python is:
for variable in sequence: # Code to execute for each element in sequence else: # Code to execute when for loop has finished
Examples:
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit) else: print("No more fruits")
Control Statements:
Control statements are used in control flow to alter the direction in which a program is executed. The sys.exit() function, break statements, and continue statements are the three different types of control statements available in Python.
Break Statements:
Break statements are used to terminate a loop prematurely. The syntax for break statements in Python is:
while condition: # Code to execute while condition is True if some_condition: break
Examples:
x = 1 while x <= 10: print(x) x += 1 if x == 5: break
Continue Statements:
Continue statements are used to skip over an iteration of a loop. The syntax for continue statements in Python is:
while condition: # Code to execute while condition is True if some_condition: continue
Examples:
x = 1 while x <= 10: if x == 5: x += 1 continue print(x) x += 1
Ending a Program with sys.exit()
The sys.exit() function is used to terminate a program prematurely. The syntax for the sys.exit() function in Python is:
import sys sys.exit()
Examples:
import sys x = 1 while x <= 10: print(x) x += 1 if x == 5: sys.exit()
This code will terminate the program when x is equal to 5.
Conclusion
In conclusion, control flow is a key idea in programming that enables programmers to regulate the sequence in which statements are executed. Sequential, selection, and repetition control flow are just a few of the different types of control flow constructs available in Python. Writing efficient and effective programs requires a thorough understanding of control flow and its effective application.
Summary of Control Flow Concepts:
Control flow refers to the order in which statements are executed in a program. Sequential, selection, and repetition control flow are just a few of the different types of control flow constructs available in Python. These building blocks give programmers the ability to make choices, reuse code, and manage how a program is executed.
Tips for using Control Flow in Python:
- Use if-else statements to make decisions based on certain conditions.
- Use for and while loops to repeat code multiple times.
- Use break and continue statements to change the flow of execution of a loop.
- Use the sys.exit() function to terminate a program prematurely.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs:
What is control flow in programming?
In programming, the sequence in which statements are executed within a program is referred to as control flow. It enables programmers to control how code is executed based on specific criteria, enhancing the adaptability and flexibility of their programs.
What are types of control flow?
Python supports three different types of control flow: sequential, selection, and repetition.
What is flow of function in Python?
The order in which statements are executed within a function is referred to as the flow of a function in Python. If statements and loops are examples of control flow constructs that can be used within functions to manage the execution flow.
Which Python keyword is used for loop control flow?
“break” and “continue” are the Python keywords used for loop control flow. The word “break” is used to end a loop early, whereas the word “continue” is used to skip an iteration of a loop.
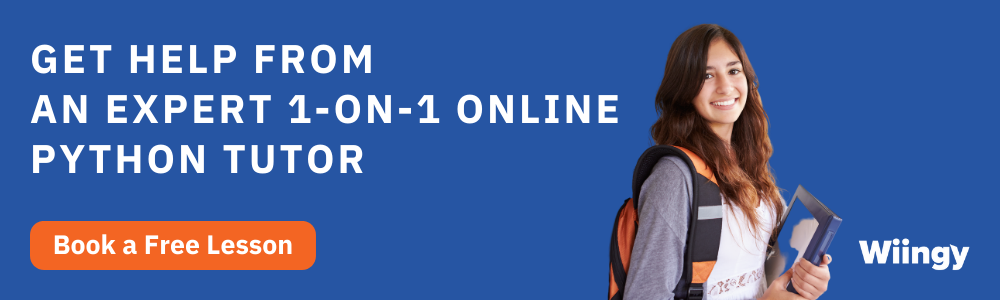
Written by by
Rahul LathReviewed by by
Arpit Rankwar