Python Tutorials
What are Operator Functions in Python?
Operators are special symbols used in Python programming to do things like add, subtract, compare, and check if something is true. These operators work with data to achieve an outcome. There are numerous operators available in Python for various operations.
Programming languages require operators as a fundamental component. They aid in carrying out numerous actions on data and values, enhancing the efficiency and readability of the code. Programmers can write programs with fewer lines of code that are clear and simple to understand by employing operators.
In this blog post, we will discuss different types of operators functions in Python, including unary operators, logical operators, identity operators, membership operators, bitwise operators, and other operators. We will also provide relevant examples to illustrate how these operators work in Python.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
List of Other Python Operators
A. Unary operators are those that perform operations on a single operand. In Python, there are three unary operators: +, -, and ~.
(unary plus) The unary plus operator returns the positive value of the operand.
Example:
(unary minus) The unary minus operator returns the negative value of the operand.
Example:
~ (bitwise NOT) The bitwise NOT operator returns the complement of the operand’s binary representation.
Example:
B. Logical operators are used to perform logical operations on values or expressions. Python has three logical operators: and, or, and not.
- and (logical AND) The logical AND operator returns True if both operands are True.
Example:
- or (logical OR) The logical OR operator returns True if at least one operand is True.
Example:
- not (logical NOT) The logical NOT operator returns the opposite of the operand’s value. If the operand is True, it returns False, and if the operand is False, it returns True.
Example:
C. Identity Operators are used to compare the memory locations of two objects. Python has two identity operators: is and is not.
- is (identity test) The is operator returns True if both operands refer to the same object.
Example:
- is not (negated identity test) The is not operator returns True if both operands do not refer to the same object.
Example:
D. Membership operators are used to test if a value or variable is present in a sequence or container. Python has two membership operators: in and not in.
- in (membership test) contd. if 2 in x: print(“2 is present in x”)
- not in (negated membership test) The not in operator returns True if the value or variable is not present in the sequence or container.
Example:
E. Bitwise Operators are used to perform operations on binary numbers. Python has five bitwise operators: &, |, ^, <<, and >>.
- & (bitwise AND) The bitwise AND operator performs a logical AND operation on the binary representation of the operands.
Example:
- | (bitwise OR) The bitwise OR operator performs a logical OR operation on the binary representation of the operands.
Example:
- ^ (bitwise XOR) The bitwise XOR operator performs a logical XOR operation on the binary representation of the operands.
Example:
- << (left shift) The left shift operator shifts the bits of the left operand to the left by the number of positions specified in the right operand.
Example:
- (right shift) The right shift operator shifts the bits of the left operand to the right by the number of positions specified in the right operand.
Example:
F. Other Operators Apart from the above operators, Python also provides some other useful functions that perform specific operations on values or variables.
- abs(x) The abs function returns the absolute value of a number.
Example:
- round(number[, ndigits]) The round function returns the rounded value of a number up to the specified number of digits.
Example:
- index_of(item) The index_of function returns the index of the specified item in a sequence or container.
Example:
- concat(s1, s2) The concat function concatenates two strings and returns the resulting string.
Example:
Example Code Snippets for Other Operators in Python
Code snippets and explanation for each operator:
(unary plus): The unary plus operator can be used to indicate a positive value. For example:
(unary minus): The unary minus operator can be used to indicate a negative value. For example:
~ (bitwise NOT): The bitwise NOT operator can be used to invert the bits of an integer. For example:
and (logical AND): The logical AND operator returns True if both operands are True, otherwise it returns False. For example:
or (logical OR): The logical OR operator returns True if at least one of the operands is True, otherwise it returns False. For example:
not (logical NOT): The logical NOT operator returns the opposite Boolean value of the operand. For example:
is (identity test): The identity test operator checks if two variables refer to the same object in memory. For example:
is not (negated identity test): The negated identity test operator checks if two variables do not refer to the same object in memory. For example:
in (membership test): The membership test operator checks if a value is present in a sequence. For example:
not in (negated membership test): The negated membership test operator checks if a value is not present in a sequence. For example:
& (bitwise AND): The bitwise AND operator performs a binary AND operation on two integers. For example:
| (bitwise OR): The bitwise OR operator performs a binary OR operation on two integers. For example:
^ (bitwise XOR): The bitwise XOR operator performs a binary XOR operation on two integers. For example:
<< (left shift): The left shift operator shifts the bits of an integer to the left. For example:
(right shift): The right shift operator shifts the bits of an integer to the right. For example:
Explanation of the inputs and output of each operator:
The inputs and output of each operator depend on the specific operation being performed. In general, operators take one or more operands as input and return a result.
For example, the + operator takes two operands and returns their sum as output. The ~ operator takes one operand and returns the bitwise NOT of that operand as output. The and operator takes two operands and returns True if both operands are True, otherwise it returns False.
Example use cases for each operator:
(unary plus): The unary plus operator is typically used to explicitly indicate a positive value, although this is often unnecessary since positive values are assumed by default.
(unary minus): The unary minus operator is used to indicate a negative value. This is often used when subtracting values or when dealing with negative numbers.
~ (bitwise NOT): The bitwise NOT operator can be used to invert the bits of an integer. This can be useful in certain situations, such as when working with binary data or when performing certain cryptographic operations.
and (logical AND): The logical AND operator is typically used to check if two conditions are both True. This can be useful in control flow statements, such as if-else statements, to determine which branch of code to execute.
or (logical OR): The logical OR operator is typically used to check if at least one of two conditions is True. This can also be useful in control flow statements to determine which branch of code to execute.
not (logical NOT): The logical NOT operator is typically used to negate a Boolean value. This can be useful in situations where you want to invert the result of a condition.
is (identity test): The identity test operator is used to check if two variables refer to the same object in memory. This can be useful in situations where you want to avoid creating unnecessary copies of data.
is not (negated identity test): The negated identity test operator is used to check if two variables do not refer to the same object in memory. This can be useful in situations where you want to ensure that two objects are distinct.
in (membership test): The membership test operator is typically used to check if a value is present in a sequence. This can be useful when working with lists or other data structures.
not in (negated membership test): The negated membership test operator is used to check if a value is not present in a sequence. This can also be useful when working with lists or other data structures.
& (bitwise AND): The bitwise AND operator is typically used to perform bitwise operations on integers. This can be useful in certain situations, such as when working with binary data or when performing certain cryptographic operations.
| (bitwise OR): The bitwise OR operator is also typically used to perform bitwise operations on integers. This can also be useful when working with binary data or when performing certain cryptographic operations.
^ (bitwise XOR): The bitwise XOR operator is typically used to perform bitwise operations on integers. This can also be useful when working with binary data or when performing certain cryptographic operations.
<< (left shift): The left shift operator is used to shift the bits of an integer to the left. This can be useful when working with binary data or when performing certain cryptographic operations.
(right shift): The right shift operator is used to shift the bits of an integer to the right. This can also be useful when working with binary data or when performing certain cryptographic operations.
Conclusion
In conclusion, operators are an essential part of Python programming. They provide a way to perform various mathematical and logical operations on data. In this blog post, we have discussed some of the most common operators in Python, including unary operators, logical operators, identity operators, membership operators, bitwise operators, and other operators. We have also provided code snippets and example use cases for each operator.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is an example of a code snippet?
One example of a code snippet can be a CSS code that styles a specific element on a web page, like changing the background color of a button. Here’s an example of a CSS code snippet:
button {
background-color: #2ecc71;
color: #fff;
border: none;
padding: 10px 20px;
border-radius: 5px;
cursor: pointer;
}
How do you write a code snippet?
One way to write a code snippet is by using a code snippet generator or repository that provides pre-written code snippets for specific tasks. These snippets can save time and effort, especially for frequently used tasks. To use a code snippet generator, simply search for the specific task or language you’re using, and select the relevant snippet to copy and paste into your code. There are several online code snippet generators available, like CodePen and GitHub Gist.
What does := mean in programming?
One meaning of := in programming is as a syntax for named parameters or keyword arguments in some languages like Python and Rust. This syntax allows you to pass arguments to a function by specifying the name of the argument along with its value, like this:
# Function call with named arguments
def my_function(arg1=1, arg2=2):
print(arg1, arg2)
my_function(arg1=10, arg2=20) # Output: 10 20
What is snippet in Python with example?
In Python, a snippet is a small piece of code that performs a specific task or solves a particular problem. Here’s an example of a Python snippet that calculates the factorial of a number using recursion:
# Calculate factorial using recursion
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n-1)
print(factorial(5)) # Output: 120
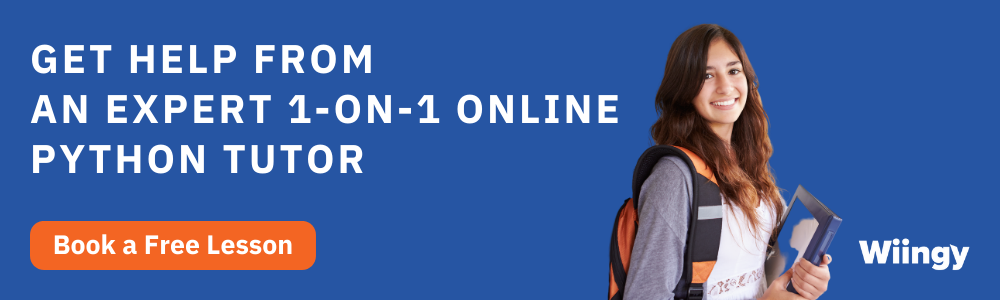
Written by by
Rahul LathReviewed by by
Arpit Rankwar