Python Tutorials
What are Exceptions?
An essential part of Python programming is handling exceptions. Python raises an exception if a mistake happens while a program is running. Unexpected behavior, incorrect input, and syntax mistakes are just a few of the causes that can result in an exception being raised. In order to prevent the program from crashing, these exceptions must be handled.
This article’s goal is to serve as a thorough introduction to Python exception handling, outlining the various exception types, the try-except block’s use for handling them, and where examples are required.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Types of Exceptions in Python
There are numerous built-in exceptions in Python that may be raised while a program is running. Typical exclusions include:
NameError: This exception is raised when an undefined variable or function is used.
TypeError: This exception occurs when an operation or function is applied to an object of inappropriate type.
ValueError: This exception is raised when a function receives an argument of the correct type but an inappropriate value.
ZeroDivisionError: This exception is raised when a division operation is performed with a divisor of zero.
IndexError: This exception is raised when an index is out of range.
KeyError: This exception is raised when a non-existent key is used to access a dictionary.
FileNotFoundError: This exception is raised when an attempt is made to access a file that does not exist.
IOError: This exception is raised when an I/O operation fails.
ImportError: This exception is raised when a module cannot be imported.
SyntaxError: This exception occurs when there is a syntax error in the code.
AttributeError: This exception is raised when an object does not have the attribute being referenced.
KeyboardInterrupt: This exception is raised when the user interrupts the program execution with a keyboard interrupt (Ctrl + C).
MemoryError: This exception is raised when an operation runs out of memory.
NotImplementedError: This exception is raised when an abstract method is called that has not been implemented.
OverflowError: This exception is raised when a calculation exceeds the maximum limit of a numeric type.
RuntimeError: This exception is raised when a runtime error occurs that does not fit into any other category.
StopIteration: This exception is raised to signal the end of an iterator.
SystemExit: This exception is raised when the program is terminated.
Exceptions are raised automatically by the interpreter whenever an error occurs during program execution.
The try-except Block
Python uses the try-except block to catch and deal with exceptions. The try-except block has the following syntax:
try:
# code that might raise an exception
except ExceptionType:
# code to handle the exception
In this block, the code that might raise an exception is placed inside the try block. If an exception is raised, the interpreter looks for an except block that matches the type of the exception raised. If a matching except block is found, the code inside that block is executed to handle the exception.
Here is an example of using the try-except block to handle the ZeroDivisionError exception:
try:
x = 10 / 0
except ZeroDivisionError:
print("Division by zero is not allowed")
In this example, the code inside the try block attempts to divide the number 10 by zero, which is not allowed. This results in a ZeroDivisionError exception being raised. The except block that follows catches this exception and prints a message to the console.
The finally Block
Another block that can be used with the try-except block to guarantee that specific code is executed whether or not an exception is raised is the finally block. The finally block’s syntax is as follows:
try:
# code that might raise an exception
except ExceptionType:
# code to handle the exception
finally:
# code that will always be executed
In this block, the code inside the try block is executed, and if an exception is raised, the code inside the except block is executed to handle the exception. Regardless of whether or not an exception is raised, the code inside the finally block is always executed.
Here is an example of using the finally block to close a file:
try:
f = open("file.txt")
# code to read or write to the file
finally:
f.close()
In this example, the code inside the try block opens a file for reading or writing. If an exception is raised during the execution of this code, the file may be left open and cause issues. To ensure that the file is always closed, the finally block is used to close the file.
Multiple Except Blocks
You might occasionally need to handle a variety of exception types in your code. To handle various exception types in Python, you can use multiple except blocks. The following syntax should be used when using multiple except blocks:
try:
# code that might raise an exception
except ExceptionType1:
# code to handle ExceptionType1
except ExceptionType2:
# code to handle ExceptionType2
When the try block’s code is executed in this block, the interpreter searches for an except block that matches the type of the exception raised if one is raised. The code contained in an except block that matches is executed to handle the exception if one is found. The interpreter will execute the first except block that matches the type of the exception raised if there are multiple except blocks present.
Here is an example of using multiple except blocks to handle different types of exceptions:
try:
# code that might raise an exception
except NameError:
# code to handle a NameError
except ValueError:
# code to handle a ValueError
In this example, the code inside the try block might raise a NameError or a ValueError. If a NameError is raised, the code inside the first except block will be executed to handle the exception. If a ValueError is raised, the code inside the second except block will be executed instead.
Raising Exceptions
You can use the raise keyword in your code to manually raise exceptions in addition to handling those that are raised automatically. The following is the syntax for raising an exception:
raise ExceptionType("Error message")
In this block, the raise keyword is used to raise an exception of the specified type, with an optional error message. This can be useful in situations where you want to signal an error condition in your code.
Here is an example of raising a ValueError exception:
x = -1
if x < 0:
raise ValueError("x cannot be negative")
In this example, the code checks if the variable x is negative. If it is, a ValueError exception is raised with the message “x cannot be negative”.
Custom Exceptions
In addition to the built-in exceptions that Python provides, you can create your own special exceptions to deal with specific error situations in your code. You must define a new class that derives from the default Exception class in order to create a special exception. The syntax for creating a custom exception is as follows:
class CustomException(Exception):
pass
In this block, a new class called CustomException is defined that inherits from the built-in Exception class. You can then use this new class to raise exceptions in your code.
Here is an example of creating and using a custom exception:
class InvalidInputError(Exception):
def __init__(self, input_value):
self.input_value = input_value
self.message = f"Invalid input: {input_value}"
def calculate_square_root(num):
if num < 0:
raise InvalidInputError(num)
return math.sqrt(num)
In this example, a new class called InvalidInputError is defined that inherits from the built-in Exception class. The class takes an input value and generates an error message that includes the input value. The calculate_square_root function checks if the input number is negative and raises an InvalidInputError exception if it is.
Conclusion
Finally, managing exceptions is an essential component of Python programming. By being aware of the various exception types in Python and being familiar with the try-except block, finally block, multiple except blocks, and raise keyword, you can create code that gracefully handles errors and guards against program crashes. Additionally, you can create your own special exceptions to deal with specific error situations in your code.
Remember that handling errors is an ongoing process that demands constant observation and updating. As your code evolves, so do the potential sources of errors. Therefore, it’s crucial to keep an eye out for potential errors in your code and make the necessary corrections. By following the best practices for handling exceptions, you can create more dependable and durable code.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are exception handling in Python?
The process of identifying, dealing with, and recovering from errors that happen during program execution is referred to as exception handling in Python. When an error occurs, one of the many built-in exceptions in Python, including NameError, TypeError, ValueError, and others, can be raised. You can handle these exceptions and guarantee that your code is error-resistant by utilizing the try-except block, finally block, multiple except blocks, and raise keyword.
What is the most common exception handling in Python?
The try-except block is the most popular exception handling method in Python. During the course of a program, exceptions may occur, and this block is used to catch and handle them. The try block is used to contain code that might cause an exception, and the except block is used to execute code that will handle the exception if one arises.
How many types of errors What is exception handling in Python?
There are numerous built-in exceptions in Python that can be thrown when something goes wrong while the program is running. Errors can take many different forms, including syntax mistakes, name errors, type errors, value errors, zero division errors, index mistakes, key mistakes, file not found mistakes, and more. In order to make sure that your code runs correctly, exception handling in Python is the process of identifying and dealing with these errors.
What is a custom exception in Python?
In Python, a custom exception is one that you create on your own to address particular error scenarios. You must define a new class that derives from the default Exception class in order to create a custom exception. Then, you can raise exceptions in your code by using this new class.
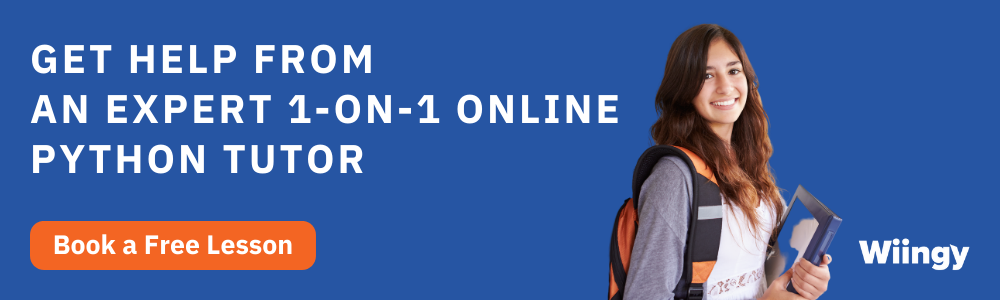
Written by by
Rahul LathReviewed by by
Arpit Rankwar