What is Any All in Python?
Python provides many built-in functions that simplify programming tasks. Among these functions are any() and all(). Any() All() function allows us to check whether any or all elements in an iterable object meet certain conditions. In this blog post, we will explore the functionality of these two functions and how they can be used in different scenarios.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
How to use the any() function in Python
Explanation of any() function
The any() function takes an iterable object (e.g. a list, tuple, or set) as an argument and returns True if at least one element in the iterable object is True. Otherwise, it returns False.
Code Snippets
- Checking for digits in a string
The any() function can be used to check if a string contains any digits. In the example below, we use the isdigit() method to check if each character in the string is a digit. The any() function returns True if any of the characters in the string are digits.
1string = 'abc123'
2contains_digit = any(char.isdigit() for char in string)
3print(contains_digit) # True
- Checking for letters in a string
Similarly, the any() function can be used to check if a string contains any letters. In the example below, we use the isalpha() method to check if each character in the string is a letter. The any() function returns True if any of the characters in the string are letters.
1string = 'abc123'
2contains_letter = any(char.isalpha() for char in string)
3print(contains_letter) # True
- Combining multiple conditions with logical OR
The any() function can also be used to combine multiple conditions with the logical OR operator. In the example below, we check if a string contains either digits or letters.
1string = 'abc123'
2contains_digit_or_letter = any(char.isdigit() or char.isalpha() for char in string)
3print(contains_digit_or_letter) # True
Use of any() with:
- Arrays
The any() function can be used with arrays as well. In the example below, we check if any element in the array is greater than 5.
1array = [1, 2, 3, 6, 4]
2greater_than_five = any(element > 5 for element in array)
3print(greater_than_five) # True
- Dictionaries
The any() function can be used with dictionaries as well. In the example below, we check if any value in the dictionary is greater than 5.
1dictionary = {'a': 1, 'b': 6, 'c': 3}
2greater_than_five = any(value > 5 for value in dictionary.values())
3print(greater_than_five) # True
- Strings
The any() function can also be used with strings. In the example below, we check if any of the strings in the list contain the letter ‘a’.
1string_list = ['apple', 'banana', 'orange']
2contains_a = any('a' in string for string in string_list)
3print(contains_a) # True
How to Use the all() Function in Python
Explanation of all() function
The all() function takes an iterable object as an argument and returns True if all elements in the iterable object are True. Otherwise, it returns False.
Example code snippets for:
- Checking for letters in a string
The all() function can be used to check if a string contains only letters. In the example below, we use the isalpha() method to check if each character in the string is a letter. The all() function returns True if all of the characters in the string are letters.
1string = 'abc123'
2contains_digit = any(char.isdigit() for char in string)
3print(contains_digit) # True
- Checking for digits in a string
Similarly, the all() function can be used to check if a string contains only digits. In the example below, we use the isdigit() method to check if each character in the string is a digit. The all() function returns True if all of the characters in the string are digits.
1string = 'abc123'
2contains_letter = any(char.isalpha() for char in string)
3print(contains_letter) # True
- Combining multiple conditions with logical AND
The all() function can also be used to combine multiple conditions with the logical AND operator. In the example below, we check if a string contains both letters and digits.
1string = 'abc123'
2contains_digit_or_letter = any(char.isdigit() or char.isalpha() for char in string)
3print(contains_digit_or_letter) # True
Use of all() with:
- Arrays
The all() function can be used with arrays as well. In the example below, we check if all elements in the array are greater than 0.
1array = [1, 2, 3, 4, 5]
2greater_than_zero = all(element > 0 for element in array)
3print(greater_than_zero) # True
- Dictionaries
The all() function can be used with dictionaries as well. In the example below, we check if all values in the dictionary are greater than 0.
1dictionary = {'a': 1, 'b': 2, 'c': 3}
2greater_than_zero = all(value > 0 for value in dictionary.values())
3print(greater_than_zero) # True
- Strings
The all() function can also be used with strings. In the example below, we check if all strings in the list contain the letter ‘a’.
1string_list = ['apple', 'banana', 'orange']
2contains_a = all('a' in string for string in string_list)
3print(contains_a) # False
Conclusion
In this blog post, we have explored the any() and all() functions in Python. We have seen how these functions can be used to check if any or all elements in an iterable object meet certain conditions. We have provided examples of using these functions with arrays, dictionaries, and strings.
The any() and all() functions are powerful tools for simplifying programming tasks. These functions allow us to easily check whether any or all elements in an iterable object meet certain conditions. By using these functions, we can write more concise and readable code.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What does any () do in Python?
In Python, the any() function can also be used to check if a boolean condition is true for any element in an iterable. For example:
1my_list = [False, False, True, False]result = any(my_list)print(result)Output: True
Here, the any() function returns True because there is at least one element in the list ‘my_list’ that is true (in this case, the third element is True).
Can we use any () in string?
We can also use any() function to check if a character or substring exists in a string or not. For example:
my_string = “Hello, World!”
result = any(char in my_string for char in ‘aeiou’)
print(result)
Output: True
Here, the any() function checks if any of the characters in the string ‘aeiou’ exist in the string ‘my_string’. Since the letter ‘o’ exists in ‘my_string’, the any() function returns True.
What is the difference between any and all in pandas?
In pandas, any() and all() are functions that are used to check the truth value of an entire DataFrame or Series. The main difference between the two functions is that any() returns True if any value in the DataFrame or Series is True, while all() returns True only if all values in the DataFrame or Series are True. The any() and all() functions can also be used with conditions, in which case they return True if the condition is True for any or all values, respectively. These functions are useful for filtering or selecting data based on certain conditions or criteria.
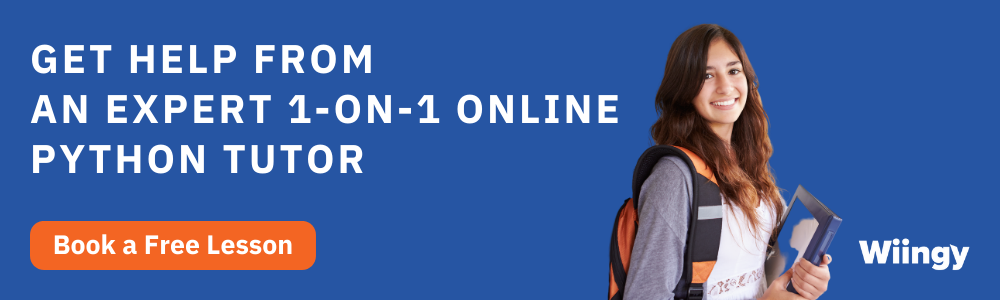
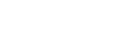
Jan 30, 2025
Was this helpful?