Python Tutorials
Pyramid patterns are fascinating and ubiquitous in programming. These patterns, crafted with care and precision, are not only visually appealing but also critical for honing logical thinking and problem-solving skills. Learning to build a pyramid program in python is not just an exercise in creativity but a path towards understanding fundamental programming constructs.
This detailed guide will show you how to use Python, one of the most popular and easy-to-learn programming languages, to make different pyramid patterns. We will look at different kinds of pyramid patterns, ranging from easy to hard and everything in between. These include inverted pyramids, number pyramids, star pyramids, and more.
This guide to making python pyramid patterns is perfect for you if you are a beginner who wants to learn how to code or an experienced coder who wants to review the basics. Grab your virtual tools, and let us get started on this fun coding adventure!
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Understanding Pyramid Patterns
Why Learn Pyramid Patterns in Python?
- Enhances Logical Thinking: Pyramid patterns involve systematic structuring, fostering logical thinking.
- Builds Programming Skills: Understanding the core concept of patterns helps in tackling complex programming problems.
- Visual Representation: A great way to visualize loop functionality.
Using Python to Create Pyramid Patterns
Python’s simplicity and readability make it an excellent choice for creating pyramid patterns. You can experiment with loops and print functions to create different shapes and designs.
Python Loops: A Quick Refresher
For Loop
Used to iterate over a sequence and execute a block of code for each element.
for i in range(5):
print('*' * i)
While Loop
Executes a block of code as long as a condition remains true.
i = 0
while i < 5:
print('*' * i)
i += 1
Understanding Python’s Print Function
The print function in Python is versatile and allows control over what and how something is printed. For example, print(‘*’ * i) prints the asterisk i times.
Simple Pyramid Patterns
Creating a Simple Pyramid Pattern
Here’s how you can create a basic pyramid pattern using a for loop:
for i in range(5):
print(' ' * (5 - i - 1) + '*' * (2 * i + 1))
Understanding the Code: How the Simple Pyramid Works
The code creates a pyramid with 5 rows. The spaces ‘ ‘ control the indentation, while the asterisks * form the pyramid.
Inverted Pyramid Patterns
Creating an Inverted Pyramid Pattern
Inverting a pyramid can be a fun and creative way to explore pyramid program in python. Here’s how to create an inverted pyramid pattern:
for i in range(5, 0, -1):
print(' ' * (5 - i) + '*' * (2 * i - 1))
Decoding the Inverted Pyramid: A Deep Dive into the Code
- Line by Line Explanation:
- range(5, 0, -1): This loops from 5 down to 1.
- print(‘ ‘ * (5-i) + ‘*’ * (2*i-1)): Controls the spaces and asterisks to create the inverted effect.
Common Errors and Their Solutions:
- Syntax Errors: Check for unmatched parentheses or improper indentation.
- Logical Errors: Pay attention to the range; improper values might not print the pattern correctly.
Number Pyramid Patterns
Building a Number Pyramid Pattern
Numbers can also be used to create beautiful pyramid patterns:
for i in range(1, 6):
print(' ' * (5 - i) + ' '.join(map(str, range(1, i + 1))))
The Logic Behind Number Pyramid Patterns
The code forms a number pyramid with 5 rows. range(1, 6) controls the number of rows, and ‘ ‘.join(map(str, range(1, i+1))) converts numbers to strings and joins them with spaces.
Common Errors:
- Type Errors: Ensure that numbers are converted to strings before joining.
- Range Errors: Adjust the range according to the desired pyramid size.
Star Pyramid Patterns
Crafting a Star Pyramid Pattern
Star patterns are among the most common types of python pyramid pattern. Here’s how you can build one:
for i in range(1, 6):
print(' ' * (5 - i) + '* ' * i)
Unraveling the Star Pyramid: A Code Analysis
- The outer loop determines the number of rows.
- The print statement, with spaces and asterisks, forms the star pyramid.
Potential Mistakes and Fixes:
- Indentation Errors: Be mindful of Python’s indentation rules.
- Print Statement Errors: Check the sequence of spaces and asterisks.
Diamond Pyramid Patterns
Constructing a Diamond Pyramid Pattern
Diamond patterns are intriguing and can be crafted using the pyramid pattern using python:
n = 5
for i in range(n):
print(' ' * (n - i - 1) + '* ' * (i + 1))
for i in range(n - 2, -1, -1):
print(' ' * (n - i - 1) + '* ' * (i + 1))
Demystifying the Diamond Pyramid: A Look at the Code
The code above creates a diamond shape using two loops. The first forms the top half, and the second creates the bottom half.
Common Challenges:
- Understanding the Structure: Break down the code into two parts to understand the top and bottom halves.
- Alignment Issues: Carefully manage spaces and asterisks to align the pattern properly.
Hollow Pyramid Patterns
Designing a Hollow Pyramid Pattern
Hollow pyramid patterns are unique, allowing you to create a pyramid shape with only the borders filled with characters. Here’s a simple way to design a hollow pyramid pattern using pyramid program in python:
n = 5
for i in range(n):
if i == 0 or i == n - 1:
print('*' * (2 * i + 1))
else:
print('*' + ' ' * (2 * i - 1) + '*')
The Hollow Pyramid Decoded: Understanding the Code
- First and Last Rows: These are filled entirely with asterisks.
- Middle Rows: Only the first and last characters are asterisks; the rest are spaces.
Common Errors:
- Boundary Errors: The hollow effect might not be achieved if the conditions for the first and last rows are not defined properly.
- Spacing Errors: Incorrect spaces can lead to misalignment.
Character Pyramid Patterns
Creating a Character Pyramid Pattern
A character pyramid pattern utilizes characters to form a pyramid. Here’s a guide to create such a pattern:
n = 5
character = 'A'
for i in range(n):
print(' ' * (n - i - 1) + character * (2 * i + 1))
character = chr(ord(character) + 1)
Character Pyramid Patterns: A Walkthrough of the Code
This code prints a pyramid pattern with characters, incrementing from ‘A’ upwards.
Possible Errors and Solutions:
- Character Increment Errors: Ensure the character is incremented properly using chr(ord(character) + 1).
- Alignment Errors: Adjust spaces to align the pyramid correctly.
Advanced Pyramid Patterns
Building Advanced Pyramid Patterns
Advanced patterns combine different elements and concepts:
n = 5
for i in range(n):
print(' ' * (n - i - 1) + str(i + 1) + ' ' * (2 * i - 1) + str(i + 1) if i != 0 else str(i + 1))
Deciphering Advanced Pyramid Patterns: A Code Breakdown
This code forms a number pyramid with numbers on both sides and spaces in between.
Common Mistakes and Solutions:
- Complex Logic: Break down the logic into parts to understand better.
- Formatting Errors: Correctly format the print statement to achieve the desired pattern.
Tips and Tricks for Pyramid Patterns
- Common Mistakes and How to Avoid Them: Understand the structure of the pattern, correct use of loops, proper alignment, and syntax rules.
- Optimizing Your Pyramid Pattern Code: Utilize functions, clean code practices, and efficient algorithms.
Conclusion
From simple to complex, pyramid patterns using python offer an engaging way to learn coding, logic, and creativity. Experiment with different characters, numbers, and structures to deepen your understanding.
Continue to experiment, practice, and study different patterns and algorithms. Resources like Python’s official documentation can be an excellent place to explore further.
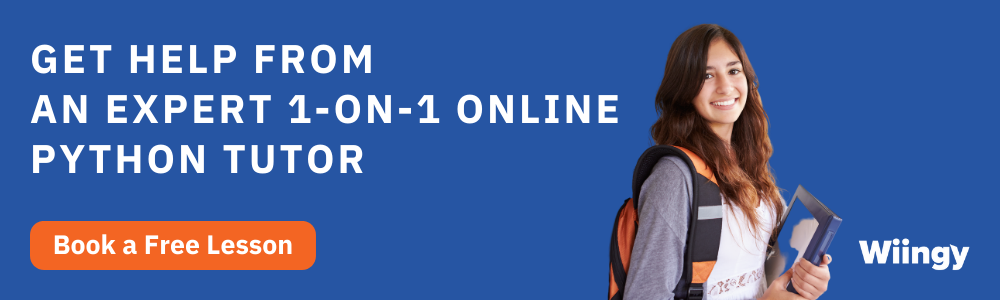
FAQs
What are some common challenges when creating pyramid patterns?
Common challenges include understanding the structure of the pattern, ensuring correct use of loops, achieving proper alignment, and adhering to syntax rules.
How can I optimize my pyramid pattern code?
To optimize your pyramid pattern code, you can utilize functions, adopt clean code practices, and employ efficient algorithms. This involves understanding the core logic of the patterns, reducing redundancy, and improving readability.
Where can I find more resources to learn about pyramid patterns in Python?
Python’s official documentation is an excellent resource for learning more about pyramid patterns in Python. It provides detailed explanations of various Python features, including loops and functions, which are essential for creating pyramid patterns.
How to print Pascal’s Triangle?
The article does not provide specific information on how to print Pascal’s Triangle in Python. However, there are many resources available online which provide guides and tutorials on this topic.
How to print Floyd’s Triangle?
The article does not provide specific information on how to print Floyd’s Triangle in Python. Just like with Pascal’s Triangle, you can find numerous guides and tutorials online that show how to print Floyd’s Triangle in Python.
Written by
Rahul LathReviewed by
Arpit Rankwar