Python decorators allow you to change a function’s or a class’s behavior without having to make a direct change to the source code. A decorator, then, is a function that accepts another function as an input, modifies it by adding some functionality, and then returns the modified function. As a result, code can be written more clearly and modularly because the original function can continue to be used while new functionality is added using decorators.
Python decorators are helpful because they make it simple to change function behavior without having to create a new function with the modified behavior. The use of them in Python libraries and frameworks makes it crucial for developers to comprehend how they operate.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Prerequisites for learning python decorators
It’s critical to have a solid grasp of the following Python concepts before diving into decorators:
- Functions: decorators are functions that take other functions as inputs, so it is important to understand how to define and call functions in Python.
- First-class objects: In Python, functions are first-class objects, allowing them to be used as parameters in other functions and as values to be returned from them.
- Inner functions: An inner function is a function that is defined inside of another function. Since decorators use this idea, it’s critical to comprehend how inner functions operate.
- Returning functions from functions: A function can be returned from another function just like any other value. This is also a key concept in decorators.
What are Python Decorators
In Python, a decorator is a function that accepts an argument from another function, modifies it with new functionality, and then returns the updated function. Here is an illustration of a decorator that augments a function with a timer:
1import time
2
3def timer_decorator(func):
4 def wrapper(*args, **kwargs):
5 start_time = time.time()
6 result = func(*args, **kwargs)
7 end_time = time.time()
8 print(f"Elapsed time: {end_time - start_time} seconds")
9 return result
10 return wrapper
11
12@timer_decorator
13def my_function():
14 # some code here
The timer_decorator function is a decorator that takes a function as an input, and returns a new function wrapper that adds timing functionality to the original function. The @timer_decorator syntax is a shortcut for applying the decorator to the my_function function.
Here are some other examples of simple decorators that modify function behavior:
- Memoization: a decorator that caches the results of a function so that it doesn’t have to be recalculated every time it’s called.
- Logging: a decorator that logs the input and output of a function to a file or console.
- Authentication: a decorator that checks if the user is authenticated before executing a function.
In conclusion, decorators are an important concept in Python programming, as they allow for easy modification of function behavior without having to change the source code directly. Understanding the prerequisites and syntax for creating decorators is essential for any Python developer who wants to write clean and modular code.
Decorating Functions with Parameters
Adding *args and **kwargs to the wrapper function in Python allows you to decorate functions that accept arguments. This enables the decorator to call the original function with any number of arguments. Here is an illustration of a decorator that increases a function’s result by a specified factor:
1def multiply(factor):
2 def decorator(func):
3 def wrapper(*args, **kwargs):
4 result = func(*args, **kwargs)
5 return result * factor
6 return wrapper
7 return decorator
8
9@multiply(2)
10def my_function(x):
11 return x + 1
The multiply function is a decorator factory that returns a decorator function that takes a function as an input and returns a new function that multiplies the result of the original function by factor. The @multiply(2) syntax applies the decorator to the my_function function with factor=2.
Reusing Python Decorators
By using decorators on multiple functions, they can be reused in Python. This can be accomplished by creating a decorator function and using the @decorator syntax to apply it to numerous functions. Here’s an example of a decorator that adds timing functionality to multiple functions:
1import time
2
3def timer(func):
4 def wrapper(*args, **kwargs):
5 start_time = time.time()
6 result = func(*args, **kwargs)
7 end_time = time.time()
8 print(f"Elapsed time: {end_time - start_time} seconds")
9 return result
10 return wrapper
11
12@timer
13def my_function1():
14 # some code here
15
16@timer
17def my_function2():
18 # some code here
The timer function is a decorator that adds timing functionality to a function. By applying it to my_function1 and my_function2, both functions will have timing functionality added to them.
Decorating Functions With Arguments
In Python, it is also possible to use decorators with functions that take arguments. This can be done by using the functools.wraps function to preserve the name and docstring of the original function. Here’s an example of a decorator that adds authentication functionality to a function that takes arguments:
1import functools
2
3def authenticate(func):
4 @functools.wraps(func)
5 def wrapper(*args, **kwargs):
6 # check authentication here
7 result = func(*args, **kwargs)
8 return result
9 return wrapper
10
11@authenticate
12def my_function(x, y):
13 return x + y
The authenticate function is a decorator that enhances a function with authentication capabilities. The name and docstring of the original function are kept by using functools.wraps. My_function now needs authentication before it can run; it still accepts two arguments and returns their sum.
Finally, Python decorators are an effective tool for changing the behavior of classes and functions. Functions like timing, memoization, logging, and authentication can be added using them. Developers can create code that is cleaner and more modular by learning how to decorate functions with arguments, reuse decorators, and decorate functions with parameters.
Real World Examples
Python decorators are used extensively in real-world applications to add functionality to code. Here are a few instances of decorators being used in actual applications:
- Function timing: For profiling and optimization, decorators can be used to track how long a function takes to run.
- Debugging code: Decorators can be used to add debugging information to a function, such as printing the input and output values.
- Slowing down code: Decorators can be used to simulate slow connections or high latency environments for testing purposes.
- Registering plugins: Decorators can be used to register plugins or extensions for a program, allowing for dynamic loading of functionality.
- Creating singletons: Dcorators can be used to enforce a singleton pattern, ensuring that only one instance of a class is created.
- Caching return values: Decorators can be used to cache the return value of a function, so that it doesn’t have to be recalculated every time the function is called.
- Adding information about units: Decorators can be used to add information about units to a function’s return value, making it easier to work with scientific or engineering data.
- Validating JSON: Decorators can be used to validate the input and output of a function, ensuring that it conforms to a specific format such as JSON.
Fancy Decorators
In addition to the basic functionality of decorators, there are several advanced concepts that can be used to create more complex decorators:
- Decorating classes: decorators can be used to modify the behavior of classes in Python, such as adding new methods or modifying existing ones.
- Nesting decorators: multiple decorators can be applied to a single function, allowing for more complex behavior.
- Creating decorators with arguments: decorators can be created that take arguments, allowing for more fine-grained control over their behavior.
- Stateful decorators: decorators can be created that maintain state between function calls, allowing for more complex functionality such as memoization or caching.
Conclusion
In conclusion, decorators are a useful tool for enhancing the functionality of Python code. Developers can write cleaner, more modular code by understanding the fundamentals of decorators as well as more complex ideas like nesting decorators and creating decorators with arguments. The key takeaways of using decorators effectively are:
- Decorators make it simple to change class and function behavior without altering the source code.
- Decorators are useful for a wide range of tasks, including validating JSON, caching return values, and timing functions.
- More complex functionality is possible thanks to sophisticated ideas like nesting decorators and creating decorators with arguments.
To use decorators effectively in Python programming, it’s crucial to comprehend the prerequisites for learning them, such as functions and first-class objects.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What do decorators do in Python?
In Python, decorators allow you to change a function’s or a class’s behavior without having to make a direct change to the source code. They are functions that accept the input of another function, modify it by adding some functionality, and then return the altered function.
What is Python decorator in Python?
In Python, a decorator is a function that accepts an argument of another function, modifies it, and then returns the modified function. This makes it simple to change the behavior of a function without having to directly alter the source code.
What are the different decorators in Python?
Some different decorators in Python include:
Timing decorators
Debugging decorators
Caching decorators
Authentication decorators
Validation decorators
Logging decorators
Singleton decorators
Plugin decorators
What is the decorator for main Python?
Answer: The if __name__ == “__main__”: statement is not a decorator in Python, but rather a conditional statement that checks if the current module is being run as the main program or if it is being imported as a module into another program.
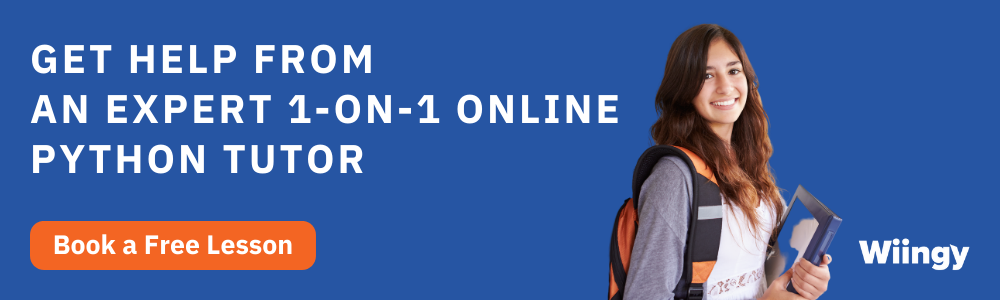
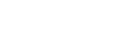
Jan 30, 2025
Was this helpful?