Python Tutorials
What are args and kwargs in Python?
args is a syntax used to pass a variable number of non-keyword arguments to a function. The asterisk () symbol is used to represent *args in the function definition, and it allows you to pass any number of arguments to the function. These arguments are then stored in a tuple within the function.
kwargs, on the other hand, is a syntax used to pass a variable number of keyword arguments to a function. The double asterisk () symbol is used to represent **kwargs in the function definition, and it allows you to pass any number of keyword arguments to the function. These arguments are then stored in a dictionary within the function.
Purpose of *args and **kwargs
In order to give your code more flexibility, *args and **kwargs allow you to pass any number of arguments to a function. This is especially helpful if you are unsure of the exact number of arguments you will need to pass.
Let’s use the example of writing a function to add two numbers together. Any number of arguments can be passed to the function using *args, and they will all be added together. This means that the function will still function if you add two numbers, three numbers, or even ten numbers.
Importance of *args and **kwargs in Python programming
*args and **kwargs are important concepts in Python programming because they allow you to create more flexible and dynamic functions. They also help you to write more concise code, as you don’t need to write separate functions for different numbers of arguments.
In addition, *args and **kwargs can be used in combination with other Python concepts, such as decorators, to create even more powerful functions.
Overall, understanding *args and **kwargs is an important part of learning Python programming, and can help you to write more efficient and flexible code.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Syntax of *args and **kwargs
A. Explanation of *args syntax
The syntax for args is to use an asterisk () followed by a parameter name in the function definition. This parameter name can be anything you choose, but it is common to use “args”. Here is an example:
def my_function(*args): # function code here
B. Explanation of **kwargs syntax
The syntax for kwargs is to use two asterisks () followed by a parameter name in the function definition. This parameter name can be anything you choose, but it is common to use “kwargs”. Here is an example:
def my_function(**kwargs): # function code here
C. Code examples of *args and **kwargs
Here are some code examples of *args and **kwargs in action:
# Example of using *args
def my_function(*args): for arg in args:
print(arg) my_function(1, 2, 3, 4, 5)
# Output: 1 2 3 4 5
# Example of using **kwargs def my_function(**kwargs):
for key, value in kwargs.items():
print(f"{key}: {value}")
my_function(name="John", age=25, location="New York")
# Output: name: John, age: 25, location: New York
How *args and **kwargs work
*args and **kwargs are used to pass a variable number of arguments to a function. With *args, you can pass any number of non-keyword arguments to a function, and they will be packed into a tuple. With **kwargs, you can pass any number of keyword arguments to a function, and they will be packed into a dictionary.
A. Code example of *args and **kwargs in action
Here is an example of how *args and **kwargs can be used in a function to accept a variable number of arguments:
def calculate_total(*args, **kwargs): total = sum(args) for key, value in kwargs.items(): total += value return total result = calculate_total(1, 2, 3, fee=4, tax=5) print(result) # Output: 15
In the above example, we define a function calculate_total that accepts both *args and **kwargs. The function calculates the total sum of all the non-keyword arguments passed through *args and all the keyword arguments passed through **kwargs.
B. Comparison with regular function arguments
Python’s regular function arguments are defined with specific parameter names, and you must pass them to the function in a specific order. You can pass the function any number of arguments in any order using *args and **kwargs. This makes *args and **kwargs more flexible than regular function arguments.
Use cases of *args and **kwargs
A. Handling variable-length arguments in functions
*args and **kwargs are commonly used in functions that need to handle a variable number of arguments. For example, if you are writing a function that needs to work with any number of inputs, *args and **kwargs can be used to pass those inputs to the function.
B. Passing multiple arguments to a function using a single parameter
*args and **kwargs can be used to pass multiple arguments to a function using a single parameter. This can make your code more concise and readable, as you don’t need to write separate function parameters for each argument.
C. Creating flexible and reusable functions
By using *args and **kwargs, you can create functions that are more flexible and reusable. For example, you can use *args and **kwargs to write a function that performs a series of operations on a variable number of inputs, regardless of their data type. This can make your code more generic and less error-prone.
D. Code examples of *args and **kwargs in different scenarios
Here are some code examples of *args and **kwargs in different scenarios:
# Example of using *args to calculate the average of a variable number of inputs
def calculate_average(*args):
total = sum(args) return total / len(args)
result = calculate_average(1, 2, 3, 4, 5)
print(result)
# Output: 3.0
# Example of using **kwargs to create a function that accepts any number of keyword arguments
def print_kwargs(**kwargs):
for key, value in kwargs.items():
print(f"{key}: {value}")
print_kwargs(name="John", age=25, location="New York")
#Output: name: John, age: 25, location: New York
# Example of using *args and **kwargs together to handle a variable number of inputs and keyword arguments
def handle_inputs(*args, **kwargs):
for arg in args: print(arg) for key, value in kwargs.items(): print(f"{key}: {value}") handle_inputs(1, 2, 3, name="John", age=25, location="New York")
# Output: 1 2 3 name: John, age: 25, location: New York
In the first example, we use *args to calculate the average of a variable number of inputs. In the second example, we use **kwargs to create a function that can accept any number of keyword arguments. In the third example, we use both *args and **kwargs together to handle a variable number of inputs and keyword arguments.
Limitations of *args and **kwargs
While *args and **kwargs can be very useful, they also have some limitations to be aware of.
A. Difficulty in debugging and testing functions with *args and **kwargs
Functions that use *args and **kwargs can be more difficult to debug and test because the number and types of arguments passed to the function can vary. This can make it harder to catch errors and ensure that the function is working correctly.
B. Inability to use *args and **kwargs with functions that have fixed parameter lists
*args and **kwargs cannot be used with functions that have a fixed parameter list. This means that if you have a function that requires a specific set of arguments, you cannot use *args and **kwargs to pass additional arguments.
C. Code examples of *args and **kwargs misuse
Here are some examples of how *args and **kwargs can be misused:
# Misuse of *args by passing a non-iterable argument def my_function(*args): for arg in args: print(arg) my_function(1, 2, 3, 4, 5, "hello") # TypeError: 'str' object is not iterable
# Misuse of **kwargs by passing an unknown argument def my_function(**kwargs): for key, value in kwargs.items(): print(f"{key}: {value}") my_function(name="John", age=25, location="New York", hobby="guitar")
# TypeError: 'hobby' is an invalid keyword argument for this function
In the first example, the *args syntax is misused by passing a non-iterable argument, which results in a TypeError. In the second example, the **kwargs syntax is misused by passing an unknown argument, which also results in a TypeError.
Ordering Arguments in a Function With the Asterisk Operators: * & **
You can also use the asterisk operators * and ** to order arguments in a function.
A. Explanation of how to use the asterisk operators * and ** to order arguments in a function
The * operator can be used to unpack iterables like tuples or lists, while the ** operator can be used to unpack dictionaries. This can be useful when you want to pass the elements of an iterable or the key-value pairs of a dictionary as separate arguments to a function.
B. Code examples of using the * and ** operators to order arguments in a function
Here are some examples of using the * and ** operators to order arguments in a function:
# Using * to unpack an iterable def my_function(a, b, c): print(a, b, c) my_list = [1, 2, 3] my_function(*my_list)
# Output: 1 2 3
# Using ** to unpack a dictionary def my_function(name, age, location): print(f"{name} is {age} years old and lives in {location}") my_dict = {"name": "John", "age": 25, "location": "New York"} my_function(**my_dict)
#Output: John is 25 years old and lives in New York
In the first example, we use the * operator to unpack the elements of the list and pass them as separate arguments to the function. In the second example, we use the ** operator to unpack the key-value pairs of the dictionary and pass them as separate keyword arguments to the function.
Conclusion
In conclusion, *args and **kwargs are strong tools that can increase the flexibility and reusability of your code. They give you the option to pass a function a variable number of arguments, which is advantageous in a variety of circumstances. They do, however, have a few drawbacks, such as testing and debugging issues and the inability to use them with functions that have fixed parameter lists.
Understanding the syntax and operation of *args and **kwargs is crucial for making effective use of them in your code. Additionally, it’s crucial to thoroughly test your functions to make sure they are operating as intended and to prevent abusing *args and **kwargs by passing improperly formatted or unidentified arguments.
In general, *args and **kwargs are strong tools that can help you write more effective and reusable functions as well as make your code more dynamic and flexible. *Args and **Kwargs can be a useful addition to your Python programming toolkit if used and cared for properly.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is **kwargs in Python?
kwargs is a syntax used in Python functions to pass a variable number of keyword arguments to the function. Any number of keyword arguments can be passed to the function, and they are then stored in a dictionary. The function definition uses the double asterisk () symbol to designate **kwargs.
What is args and kwargs in Python practices?
Python programmers frequently use *args and **kwargs to build functions that can take a variety of arguments. A function can receive a variable number of non-keyword arguments using *args and a variable number of keyword arguments using **kwargs.
What is the difference between args and kwargs function?
Keyword arguments are abbreviated as **kwargs. The use of keywords and the return of values as a dictionary are the only things that set it apart from args.
What is args and kwargs in Python constructor?
In Python, *args and **kwargs can also be used in class constructors to pass a variable number of arguments to the constructor. This can be useful when you want to create instances of a class with a variable number of properties.
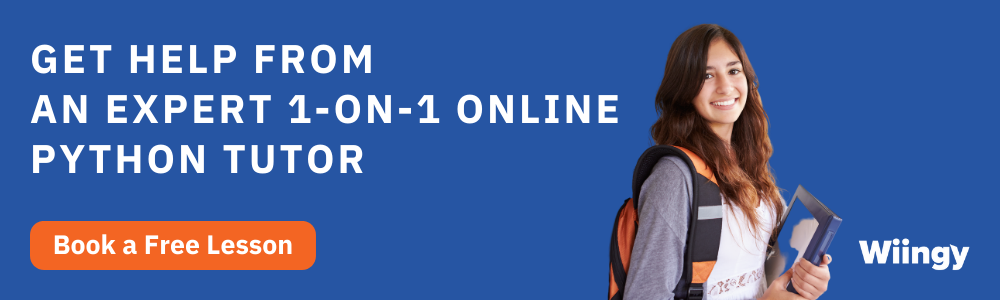
Written by by
Rahul LathReviewed by by
Arpit Rankwar