Python Tutorials
What are Built-In Exceptions in Python?
Data analysis, web development, and artificial intelligence are just a few of the many uses for the well-known programming language Python. Python programming errors can happen for a number of reasons, including invalid input, syntax mistakes, or unexpected behavior.
While writing code in Python, errors can occur due to various reasons, such as invalid input, syntax errors, or unexpected behavior.
To handle such errors, Python provides a set of built-in exceptions. These exceptions are predefined in Python and can be raised by the interpreter or by the user’s code. The common and uncommon built-in exceptions in Python will be covered in this article, along with how to handle them.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Common Built-In Exceptions
These exceptions include:
TypeError – This exception is raised when an operation or function is applied to an object of inappropriate type. For example, trying to concatenate a string and an integer will result in a TypeError.
Examples:
To handle a TypeError, we can use a try-except block. For example:
While writing Python code, one frequently runs into a number of built-in exceptions that
NameError – This exception is raised when a name or variable is not defined. For example, if we try to access a variable that has not been defined, a NameError will be raised.
Example:
To handle a NameError, we can use a try-except block. For example:
ValueError – When an operation or function is applied to an object with an inappropriate value, this exception is thrown. A ValueError will be raised, for instance, if an attempt is made to convert a string to an integer when the string does not represent an acceptable integer.
Example:
To handle a ValueError, we can use a try-except block. For example:
IndexError – When an index is outside of bounds, this exception is thrown. An IndexError will be raised, for instance, if an element in a list is attempted to be accessed using an index that does not exist.
Example:
To handle an IndexError, we can use a try-except block. For example:
KeyError – This exception is raised when a key is not found in a dictionary. For example, trying to access a value in a dictionary with a key that does not exist will result in a KeyError.
Example:
To handle a KeyError, we can use a try-except block. For example:
Less Common Built-In Exceptions
In addition to the common built-in exceptions, Python also provides several less common built-in exceptions. These exceptions are less frequently encountered but are still important to know. Some of these exceptions include:
AssertionError – This exception is raised when an assertion fails. Assertions are used to check that certain conditions are met, and if they are not, an AssertionError is raised.
Example:
To handle an AssertionError, we can use a try-except block. However, it is not recommended to handle assertion errors, as they are meant to signal programming errors and should be fixed instead of caught.
StopIteration – This exception is raised to signal the end of an iterator. When there are no more items to iterate over, a StopIteration exception is raised.
Example:
To handle a StopIteration, we can use a try-except block. However, it is not recommended to catch StopIteration exceptions, as they are meant to signal the end of the iterator.
KeyboardInterrupt – This exception is raised when the user interrupts the program by pressing Ctrl+C. This is commonly used to stop a long-running program.
Example:
To stop the program, the user can press Ctrl+C, which will raise a KeyboardInterrupt exception.
To handle a KeyboardInterrupt, we can use a try-except block. However, it is not recommended to catch KeyboardInterrupt exceptions, as they are meant to signal the user’s intent to interrupt the program.
Base Exception Class
All of Python’s built-in exceptions belong to the BaseException class, which is their superclass. It outlines the fundamental structure and operation of a Python exception. The BaseException class is the parent of all other built-in exceptions. The BaseException class has a number of methods, including str() and repr(), that can be used to modify an exception’s behavior. It is significant to remember that one of the BaseException class’s subclasses should be raised rather than the BaseException class itself.
To use the BaseException class, we can create a subclass and customize its behavior as needed. For example:
This creates a custom exception called MyException that inherits from BaseException. We can then raise this exception and provide a custom message:
Creating Custom Exceptions
Python gives us the option to create our own unique exceptions in addition to the built-in exceptions. To give more detailed information about errors that happen in our programs, we can use custom exceptions. A new class that derives from one of the built-in exception classes, like Exception or ValueError, can be used to create a custom exception.
For example:
This creates a custom exception called MyException that inherits from the built-in Exception class. We can then raise this exception in our code:
We can also add custom attributes and methods to our custom exception class to provide more information or behavior. For example:
This creates a custom exception called MyException that has a custom code attribute and a customized str() method. We can then raise this exception and provide a custom message and code:
Exception Handling Best Practices
It’s critical to adhere to best practices when handling exceptions in Python in order to create robust, maintainable code. Some best practices for exception handling in Python include:
Be specific about the exceptions you catch: Let other exceptions propagate up the call stack and only catch the ones you are familiar with handling.
Use multiple except blocks: Don’t use a generic except block; instead, use distinct except blocks for each type of exception you want to catch.
Use finally to clean up resources: Use a finally block to ensure that any resources that were acquired are released, regardless of whether an exception was raised.
Don’t suppress exceptions: Avoid suppressing exceptions by catching them and doing nothing with them. Instead, either handle the exception or let it propagate up the call stack.
Use logging to record exceptions: Use Python’s logging module to record exceptions and other error information, instead of printing them to stdout or stderr.
Examples of good exception handling practices:
Examples of bad exception handling practices:
This code catches all exceptions but does nothing with them, which can make it difficult to debug errors.
Conclusion
In this article, we covered both the more frequent and less frequent built-in Python exceptions. We also discussed the BaseException class, making unique exceptions, and the best ways to handle exceptions in Python. Python programmers can write more reliable and maintainable code and handle errors more skillfully by being aware of these concepts.
In summary, built-in exceptions in Python are exceptions that are already defined and can be thrown either by the interpreter or by user code. These exceptions are divided into a number of groups, including TypeError, LookupError, and ArithmeticError. The BaseException class, which provides an exception’s fundamental structure and behavior, is the superclass of all built-in exceptions. By defining a new class that derives from one of the existing exception classes, custom exceptions can be made.
Errors are a common part of software development, so it’s crucial to understand built-in exceptions in Python and how to handle them effectively. Programmers can produce more dependable and robust software if they are able to recognize and handle exceptions.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are the built-in exceptions in Python?
Predefined exceptions that can be thrown by the interpreter or by user code make up Python’s built-in exceptions.
What is a built-in exception?
A built-in exception is a predefined exception in Python that can be raised by the interpreter or by the user’s code.
What are the 4 types of exceptions in Python?
The 4 types of exceptions in Python are SyntaxError, TypeError, NameError, and IndexError.
What are the 3 major exception types in Python?
The 3 major exception types in Python are SyntaxError, Exceptions, and SystemExit.
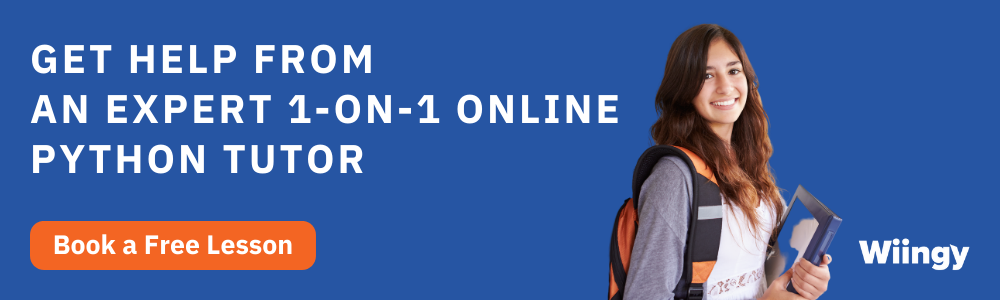
Written by by
Rahul LathReviewed by by
Arpit Rankwar