Python Tutorials
File Handling in Python
File handling is one of the most crucial components of Python programming. File handling in Python describes how a program communicates with files stored on a computer’s hard drive. Python’s file handling features let programmers make, read, write, and modify files.
Data appending to an existing file is a key idea in file handling. When you want to add more data to a file without overwriting its current contents, this is especially helpful. Any programmer who wishes to work with files in Python must first understand how to append to a file.
This article will provide a thorough guide to appending to a file in Python, complete with an overview of the concept, an explanation of its significance, and examples.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Appending to a File in Python
Appending to a file in Python is a simple process that can be done in a few different ways. Here are two of the most common methods:
A. Using the open() function in “a” mode
The “a” mode in the open() function stands for “append.” This mode allows you to open a file in append mode, which means that any data you write to the file will be added to the end of its existing contents.
Syntax of the “a” mode
The syntax for opening a file in “a” mode is as follows:
file = open("filename", "a")
In this syntax, “filename” is the name of the file you want to open, and “a” is the mode you want to open it in.
Opening a file in “a” mode
To open a file in “a” mode, you can use the following code:
file = open("example.txt", "a")
This code will open the file “example.txt” in append mode. If the file does not exist, it will be created.
B. Using the with statement in “a” mode
Using the with statement is an additional Python method for appending to a file. The with statement makes handling files in Python easier by taking care of automatically closing the file when you’re done with it.
Syntax of the with statement in “a” mode
The syntax for using the with statement in “a” mode is as follows:
with open("filename", "a") as file:
In this syntax, “filename” is the name of the file you want to open, and “a” is the mode you want to open it in.
Appending to a file using the with statement
To append to a file using the with statement, you can use the following code:
with open("example.txt", "a") as file:
file.write("This is some text that will be appended to the file.")
This code will open the file “example.txt” in append mode and write the text “This is some text that will be appended to the file.” to the end of the file.
C. Examples of appending to a file in Python
Here are a few examples of how to append to a file in Python:
# Example 1: Using the open() function in "a" mode
file = open("example.txt", "a")
file.write("This is some text that will be appended to the file.")
file.close()
# Example 2: Using the with statement in "a" mode
with open("example.txt", "a") as file:
file.write("This is some more text that will be appended to the file.")
Best Practices
There are a few best practices to remember when appending to a file in Python. These best practices cover proper file handling techniques, maintaining code readability, and avoiding pitfalls.
A. Proper file handling practices
The with statement, which ensures that the file is automatically closed, and checking to see if the file already exists before attempting to open it in append mode are all examples of proper file handling procedures in Python.
For example, you could use the following code to check whether a file exists before you try to open it in append mode:
import os.path
if os.path.isfile("example.txt"):
with open("example.txt", "a") as file:
file.write("This is some text that will be appended to the file.")
else:
print("The file does not exist.")
B. Best practices for maintaining code readability
To maintain code readability, it’s important to use descriptive variable names and comments to explain what your code is doing. This is especially important when working with file handling, as file operations can be complex and difficult to understand.
For example, you could use the following code to append a list of numbers to a file:
# Append a list of numbers to a file
numbers = [1, 2, 3, 4, 5]
with open("numbers.txt", "a") as file:
for number in numbers:
file.write(str(number) + "\n")
In this code, the variable “numbers” is used to store the list of numbers that will be appended to the file. The comment above the code explains what the code does in plain English.
C. Pitfalls to avoid
When appending to a file in Python, there are a few pitfalls to watch out for. Forgetting to add the newline character (“n”) at the end of each line you write to the file is a common error. This may cause the text to be written on a single line, which could make it challenging to read.
Another common error is to overwrite a file’s existing contents rather than adding to them. This can occur if you fail to open the file in append mode or open it in the incorrect mode.
To avoid these pitfalls, always double-check your code to make sure you’re using the correct mode when opening the file, and make sure to include the newline character at the end of each line you write to the file.
Always double-check your code to make sure you’re opening the file in the right mode, and make sure to add the newline character at the end of each line you write to the file to avoid these pitfalls. By following best practices for file handling and code readability, you can ensure that your code is easy to understand and maintain. With the examples and tips provided in this article, you should now have a solid foundation for appending to a file in Python.
Conclusion
The two most popular ways to append to a file in Python have been covered in this article, including using the open() function in “a” mode and the with statement in “a” mode. Best practices for file handling, code readability, and avoidable pitfalls have also been discussed.
For your code to be reliable and secure, good file handling procedures are essential. You can avoid data corruption and other errors that can happen when working with files by adhering to best practices for file handling.
Every programmer should be aware of the fundamental idea of appending to a file in Python. Your code will be clear, readable, and simple to maintain if you adhere to the recommendations and examples given in this article. More complex file handling situations will probably come up as you work with Python more, but if you have a firm grasp of the fundamentals, you’ll be ready to handle them.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
How do you append to a file in Python?
In Python, you can append to a file by using the with statement in “a” mode or the open() function in “a” mode.
What is append() in Python?
In Python, the append() method is used to append an item to the end of a list. It has nothing to do with managing files specifically.
Can you write append file in Python?
In Python, you can append to a file by using the open() function in “a” mode or the with statement in “a” mode.
How do I append a list into a txt file in Python?
To append a list to a text file in Python, you can use a for loop to iterate through the list and write each item to the file using the write() method. For example:
my_list = [1, 2, 3, 4, 5]
with open(“my_file.txt”, “a”) as file:
for item in my_list:
file.write(str(item) + “\n”)
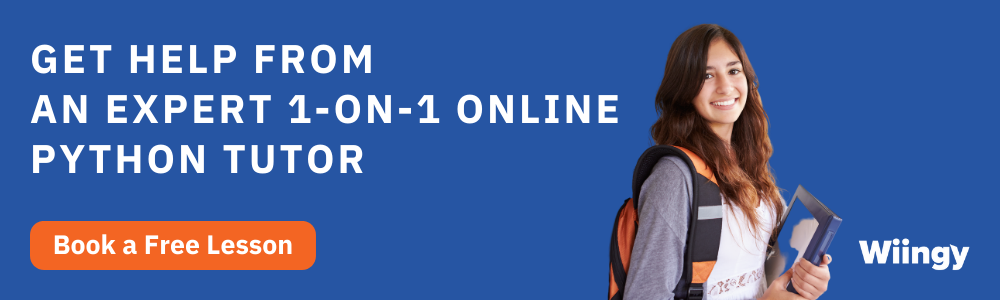
Written by by
Rahul LathReviewed by by
Arpit Rankwar