Python Tutorials
What is File Handling in Python?
File handling is an important part of programming, and any programmer needs to know how to open, read, and write files in Python. A computer file is a collection of information that can be accessed, read, and modified with the help of a computer program.
Python’s in-built functions and methods make it easy to manage files effectively. This article will cover the basics of working with Python files, including opening, reading, and writing in a variety of modes.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Writing to a File in Python
Python offers the ability to write data to files in addition to reading from them. When you want to create or update a file’s contents, this is helpful. A file must first be opened in write mode using the open() function before being written to using the write() or writelines() methods.
A. Using the write() method
The write() method is used to write a string to a file.
Writing a single line:
file = open("example.txt", "w")
file.write("This is a single line.")
file.close()
In this example, we opened the file “example.txt” in write mode, wrote a single line to the file using the write() method, and closed the file using the close() method.
Writing multiple lines:
file = open("example.txt", "w")
file.write("Line 1\n")
file.write("Line 2\n")
file.write("Line 3\n")
file.close()
In this example, we wrote multiple lines to the file by calling the write() method multiple times, each time with a new line character “\n” to separate the lines.
B. Using the writelines() method
The writelines() method is used to write multiple lines to a file.
Writing multiple lines as a list:
lines = ["Line 1\n", "Line 2\n", "Line 3\n"]
file = open("example.txt", "w")
file.writelines(lines)
file.close()
In this example, we created a list of strings, each representing a line in the file, and then used the writelines() method to write all the lines to the file.Writing multiple lines as a string:
lines = "Line 1\nLine 2\nLine 3\n"
file = open("example.txt", "w")
file.writelines(lines)
file.close()
In this example, we created a single string with all the lines separated by new line characters “\n” and used the writelines() method to write the string to the file.
Best Practices
A. Proper file handling practices:
- Before opening a file, make sure it already exists.
- When working with files, use error handling to catch any exceptions that might happen.
- Depending on the kind of operation you want to run, use the appropriate file modes.
B. Best practices for maintaining code readability:
- To make your code more understandable, give your variables and functions names that are descriptive.
- Use comments to clarify difficult or crucial portions of your code.
- To make your code easier to read and comprehend, divide it up into logical sections.
C. Pitfalls to avoid:
- To prevent unintentionally overwriting or deleting data, always open a file in the proper mode.
- When finished working with a file, always close it to prevent memory leaks and other problems.
- When working with large files, exercise caution because reading or writing a lot of data can impact performance.
An essential skill for any programmer is the ability to open, read, and write files in Python. You can quickly change the contents of a file in a variety of ways by utilizing the built-in functions and methods offered by Python. To avoid common pitfalls and issues, it’s crucial to adhere to best practices for maintaining code readability and proper file handling procedures. You can master using files in Python with practice and careful attention to detail.
Conclusion
We covered the opening, reading, and writing of files in Python in this article. We discussed the different ways that a file can be opened as well as the various techniques for reading and writing data to files. Best practices for file handling were also covered, including how crucial it is to properly close files and keep your code readable.
Importance of proper file handling practices:
In order to guarantee the security and integrity of your data, proper file handling procedures are crucial. Performance problems, security breaches, and data loss can occur when best practices are not followed. When opening a file, you should always use the proper mode. You should also always close files when you are finished working with them.
Final thoughts and future considerations:
It is critical to continue practicing good file handling techniques as you learn and hone your Python skills and to stay current on industry trends and best practices. By doing so, you can ensure that your code is reliable, efficient, and secure.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
How do you read and then write to a file in Python?
In Python, you can read and write to files by opening them in the proper mode (for example, “r+” for read and write), reading the contents, making any necessary changes, and then writing the changes back to the file.
How do you open a file for writing in Python?
To open a file for writing in Python, you can use the open() function with the “w” mode, like this:
file = open(“example.txt”, “w”)To open a file for writing in Python, you can use the open() function with the “w” mode, like this:
file = open(“example.txt”, “w”)
How would you open a file for reading in Python?
To open a file for reading in Python, you can use the open() function with the “r” mode, like this:
file = open(“example.txt”, “r”)
How do you append data to an existing file in Python?
To append data to an existing file in Python, you can use the “a” mode when opening the file, like this:
file = open(“example.txt”, “a”)
file.write(“This is new data to be appended.”)
file.close()
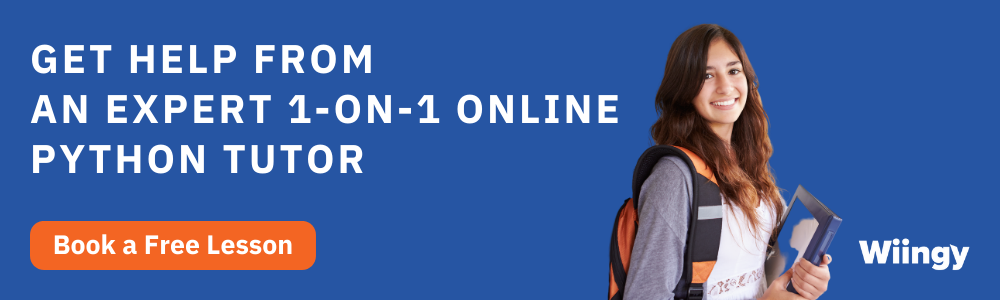
Written by by
Rahul LathReviewed by by
Arpit Rankwar