Python Tutorials
What is Encapsulation in Python?
Encapsulation, which is the practice of obscuring data or implementation details within a class, is one of the fundamental concepts of object-oriented programming (OOP). This method combines the methods that act on the data with the data itself into a single unit. This enables the creation of objects with a predetermined set of attributes and behaviors that can be accessed and changed in a controlled way.
Encapsulation is a technique for hiding a class’ internal operations and exposing only the information required for external interactions. It enables the creation of objects with clearly defined behaviors and properties, enhancing their usability and security. Encapsulation restricts access to a class’s data to its methods and keeps the class’s variables private.
Reasons to use encapsulation in python:
- Only the necessary information is exposed and the implementation details are concealed from the outside world, the code is made more secure.
- It allows for the creation of objects with clearly defined properties and behaviors, which facilitates the reuse of the code throughout the program.
- The implementation details are concealed and only the necessary methods are exposed, it aids in maintaining a clean codebase.
Python supports encapsulation through the use of classes and is an object-oriented programming language. Python class variables can be made private, public, or protected to achieve encapsulation.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Encapsulation in Python
Encapsulation is achieved in Python through the use of classes. A class is a blueprint for creating objects, and it defines the properties and behaviors of an object. In Python, encapsulation is achieved through the use of access modifiers and getter and setter methods.
A. Defining classes in Python
In Python, classes are defined using the “class” keyword followed by the name of the class. The class definition can contain class variables and methods.
Public access
Public variables and methods are those that can be accessed by any part of the program. In Python, variables and methods are public by default, and they do not require any special keyword to be declared as public.
Example:
class Car:
color = "red"
def start(self):
print("Car started!")
In the above example, the “color” variable and the “start” method are public and can be accessed by any part of the program.
Private access
Private variables and methods are those that can only be accessed within the class. In Python, private variables and methods are declared by prefixing them with double underscores (__).
Example:
class Car:
__engine_capacity = "2000cc"
def __start_engine(self):
print("Engine started!")
In the above example, the “__engine_capacity” variable and the “__start_engine” method are private and can only be accessed within the “Car” class.
Protected access
Protected variables and methods are those that can be accessed within the class and its subclasses. In Python, protected variables and methods are declared by prefixing them with a single underscore (_).
Example:
class Car:
_mileage = 0
def _drive(self):
self._mileage += 10
In the above example, the “_mileage” variable and the “_drive” method are protected and can be accessed within the “Car” class and its subclasses.
B. Access Modifiers
Access to variables and methods in a class can be managed using access modifiers. Underscores are used in Python to create access modifiers.
Private access modifier
As mentioned earlier, private variables and methods in Python are declared by prefixing them with double underscores (__). Private variables cannot be accessed outside the class, and private methods can only be called from within the class.
Example:
class Person:
__name = "John"
def __greet(self):
print("Hello, my name is", self.__name)
p = Person()
p.__name # this will give an error as __name is a private variable
p.__greet() # this will give an error as __greet is a private method
Protected access modifier
Protected variables and methods in Python are declared by prefixing them with a single underscore (_). Protected variables can be accessed within the class and its subclasses, but they cannot be accessed outside the class hierarchy.
Example:
class Animal:
_legs = 4
def _walk(self):
print("Animal is walking")
class Dog(Animal):
def bark(self):
print("Woof!")
d = Dog()
print(d._legs) # this will print 4 as _legs is a protected variable
d._walk() # this will call the _walk method from the Animal class
Public access modifier
Public variables and methods in Python do not require any access modifier, and they can be accessed by any part of the program.
Example:
class Rectangle:
length = 5
breadth = 10
def area(self):
return self.length * self.breadth
r = Rectangle()
print(r.area()) # this will print 50 as the area method is public
C. Getter and Setter Methods
Getter and setter methods are used to access and modify private variables in a class. Getter methods are used to get the value of a private variable, and setter methods are used to set the value of a private variable.
Example:
class Person:
__name = "John"
def get_name(self):
return self.__name
def set_name(self, name):
self.__name = name
p = Person()
print(p.get_name()) # this will print John
p.set_name("Mike")
print(p.get_name()) # this will print Mike
D. Property Decorators
Python provides property decorators to simplify the process of using getter and setter methods. Property decorators are used to define getter and setter methods for a property.
Example:
class Person:
def __init__(self, name):
self.__name = name
@property
def name(self):
return self.__name
@name.setter
def name(self, name):
self.__name = name
p = Person("John")
print(p.name) # this will print John
p.name = "Mike"
print(p.name) # this will print Mike
Advantages of Encapsulation in Python
A. Code Reusability
By enabling the creation of objects with clearly defined properties and behaviors, encapsulation promotes code reusability. This makes it simpler to reuse the code without having to change it in various areas of the program.
B. Security
By obscuring a class’s internal operations and exposing only what is necessary for external users to interact with the class, encapsulation enhances security. This stops unauthorized access to a class’s internal data.
C. Maintaining a Clean Codebase
By hiding a class’s implementation details and exposing only the necessary methods, encapsulation aids in maintaining a clean codebase.
Examples of Encapsulation in Python
Examples that highlight the use of private attributes, getter and setter methods, and property decorators can be used to illustrate encapsulation in Python.
A. Creating a class with private attributes
/Code start/
class BankAccount:
def __init__(self):
self.__balance = 0
def deposit(self, amount):
self.__balance += amount
def withdraw(self, amount):
if amount > self.__balance:
print("Insufficient balance")
else:
self.__balance -= amount
def get_balance(self):
return self.__balance
b = BankAccount()
b.deposit(1000)
b.withdraw(500)
print(b.get_balance()) # this will print 500
class Employee:
def __init__(self):
self.__salary = 0
def set_salary(self, salary):
self.__salary = salary
def get_salary(self):
return self.__salary
e = Employee()
e.set_salary(50000)
print(e.get_salary()) # this will print 50000
In the above example, the “salary” attribute is declared as private, and it can only be accessed through the “get_salary” method and modified through the “set_salary” method. This ensures that the internal data of the “Employee” class is secure and can only be accessed and modified in a controlled manner.
C. Implementing Property Decorators
class Person:
def __init__(self, name):
self.__name = name
@property
def name(self):
return self.__name
@name.setter
def name(self, name):
self.__name = name
p = Person("John")
print(p.name) # this will print John
p.name = "Mike"
print(p.name) # this will print Mike
In the above example, the “name” attribute is declared as private, and it can only be accessed and modified through the “name” property decorator. This simplifies the process of using getter and setter methods and ensures that the internal data of the “Person” class is secure and can only be accessed and modified in a controlled manner.
Encapsulation vs. Abstraction
A. Explanation of Abstraction
Another fundamental idea in object-oriented programming is abstraction, which refers to the practice of hiding a class’s implementation specifics and exposing only its core functionality. Abstraction gives the class a high-level perspective that makes it simpler to use and comprehend.
B. Differences between Encapsulation and Abstraction
Encapsulation is the practice of keeping a class’ internal data hidden and only exposing what is required for external interactions. On the other hand, abstraction is the practice of hiding a class’s implementation specifics and exposing only its core features. Encapsulation deals with data concealment, whereas abstraction simplifies a class’s user interface.
C. How Encapsulation and Abstraction are used together
In object-oriented programming, encapsulation and abstraction are combined to produce well-structured, maintainable code. Encapsulation offers security by hiding a class’s internal data, and abstraction streamlines a class’s interface to make it simpler to use and comprehend. Encapsulation and abstraction work together to produce reusable, modular code.
Conclusion
A key idea in Python and object-oriented programming is encapsulation. By concealing a class’s internal data and only exposing that which is required for external interaction, it offers security. Additionally, encapsulation encourages code reuse, maintains a clean codebase, and offers flexibility by enabling implementation details to be changed without having an impact on other parts of the system.
It’s crucial to comprehend the benefits of encapsulation as a beginner to Python and object-oriented programming. Encapsulation enables you to write well-organized, maintainable code, which is crucial for developing intricate software systems. To implement encapsulation effectively, it’s also crucial to practice using private variables, getter and setter methods, and property decorators in your code. With practice, you will become more proficient in using encapsulation and other object-oriented programming concepts in Python.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is encapsulation with example?
Encapsulation is the practice of keeping a class’ implementation details a secret and revealing only the information required for external interactions. Using the double underscore (__) prefix to declare a class variable private and using a getter method to access it are two examples of encapsulation in Python
Example:
class Person:
__name = “John”
def get_name(self):
return self.__name
p = Person()
print(p.get_name()) # this will print John
What is encapsulation and Abstraction in Python?
Encapsulation and abstraction are two core principles of object-oriented programming in Python. Encapsulation is the practice of hiding the internal data of a class and exposing only what is necessary for the outside world to interact with the class. Abstraction is the practice of hiding the implementation details of a class and exposing only the essential features.
How many types of encapsulation are there in Python?
In Python, there are three types of encapsulation:
Private access: declared using double underscores (__)
Protected access: declared using a single underscore (_)
Public access: default access modifier
What is encapsulation in Python educative?
They cover the idea of encapsulation in Python educational in their course on python object-oriented programming. Encapsulation is covered in the course along with other fundamental concepts of OOP like inheritance, polymorphism, and abstraction.
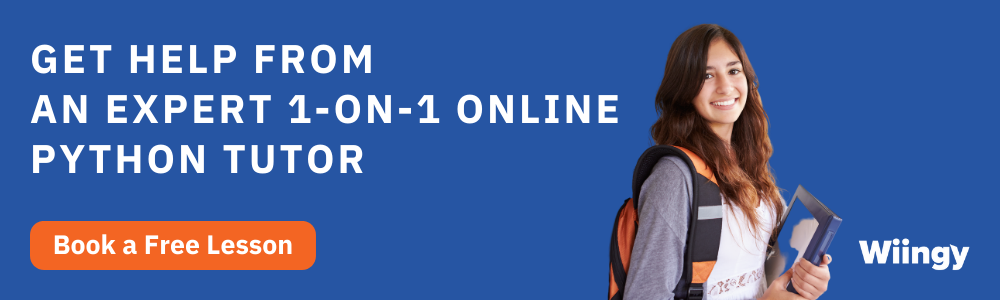
Written by by
Rahul LathReviewed by by
Arpit Rankwar