What is Python Syntax?
Writing good Python code requires an understanding of the language’s syntax. The fundamental grammar of Python, including its keywords, data types, operators, control flow statements, functions, and methods, will be covered in this article.
Definition of Python Syntax
The set of guidelines that specify the organization and sequencing of statements in a programming language are referred to as “syntax.” The python syntax is a collection of guidelines that define how the language can be expressed and used. When writing code, Python syntax comprises words, operators, expressions, statements, and other components.
In Python, words with a specific meaning that are not allowed to be used as variable names or other identifiers are known as “keywords.” The words “if,” “else,” “while,” “for,” “break,” “continue,” and “return” are examples of Python keywords. These keywords are employed, among other things, in control flow statements, loops, and functions.
Python uses symbols called operators to carry out operations on data. To add two integers, for instance, use the “+” operator. To determine whether one value is greater than another, use the “>” operator. A number of different operators are available in Python, including arithmetic, comparison, logical, assignment, and bitwise operators.
Expressions are made by putting together values, variables, and operators. Expressions can then be evaluated to get a result. For instance, the calculation “2 + 3” results in the number 5.
Statements are directives that instruct Python to carry out a particular action. For instance, the “if” statement is used to conditionally execute code while the “print()” expression is used to output output to the console.
Importance of Understanding Python Syntax
Understanding the foundation of Python syntax, as it sets the rules for how code is written and executed. Mastering these basics is essential for building effective and error-free programs. If you find yourself struggling with Python syntax, private tutoring can help Python beginners grasp these fundamental concepts with clarity and confidence. It can be the most efficient resource to master Syntax in Python.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Python Basics
Variables and Data Types
In Python, variables are used to store data values that can be used later in the program. Defining variables in Python is simple, you just need to assign a value to a variable using the ‘=’ operator. For example, to define a variable ‘x’ and assign it the value 5, you can write:
1x = 5
Python has several built-in data types that can be used to store different kinds of data. These include:
Numeric Types: Python has two numeric types, integers and floating-point numbers. Integers are whole numbers, while floating-point numbers have a decimal point. Examples:
1x = 10
2y = 3.5
Boolean Type: Boolean values are used to represent true or false values. In Python, the boolean values are ‘True’ and ‘False’. Examples:
1x = True
2y = False
String Type: Strings are used to store text data. In Python, strings are defined using either single quotes or double quotes. Examples:
1x = 'Hello, world!'
2y = "Python is fun"
Sequence Types: Sequence types are used to store collections of items, such as lists or tuples. Examples:
1x = [1, 2, 3, 4]
2y = (1, 2, 3, 4)
Mapping Types: Mapping types are used to store key-value pairs, such as dictionaries. Examples:
1x = {'name': 'John', 'age': 30}
2y = {1: 'one', 2: 'two', 3: 'three'}
Set Types: Sets are used to store unique values in Python. Examples:
1x = {1, 2, 3}
2y = {'apple', 'banana', 'orange'}
None Type: None is a special value in Python that represents the absence of a value. Examples:
1x = None
Operators
Operators are used in Python to perform operations on data. There are several types of operators in Python, including:
Arithmetic Operators: Arithmetic operators are used to perform mathematical operations on numeric values. Examples:
1x = 10 + 5 # addition
2y = 10 - 5 # subtraction
3z = 10 * 5 # multiplication
4w = 10 / 5 # division
Comparison Operators: Comparison operators are used to compare values and return a boolean value. Examples:
1x = 10 > 5 # greater than
2y = 10 < 5 # less than
3z = 10 == 5 # equal to
4w = 10 != 5 # not equal to
Logical Operators: Logical operators are used to combine boolean values and return a boolean value. Examples:
1x = True and False # and
2y = True or False # or
3z = not True # not
Assignment Operators: Assignment operators are used to assign a value to a variable. Examples:
1x = 10 # equals
2x += 5 # plus equals
3x -= 5 # minus equals
4x *= 5 # multiply equals
5x /= 5 # divide equals
Bitwise Operators: Bitwise operators are used to perform bitwise operations on numeric values. Examples:
1x = 10 & 5 # and
Assignment Operators: Assignment operators are used to assign a value to a variable. Examples:
1x = 10 # equals
2x += 5 # plus equals
3x -= 5 # minus equals
4x *= 5 # multiply equals
5x /= 5 # divide equals
Control Flow Statements
Code execution can be managed using control flow statements that are based on loops or specific situations. Conditional statements and loop statements are the two different categories of control flow statements in Python.
Using conditional expressions, different parts of code can be run depending on whether a condition is true or false. If, elif, and else are the three different categories of conditional statements in Python.
The if statement is used to check if a condition is true and execute a block of code if it is. Here is an example:
1x = 5
2if x > 3:
3print("x is greater than 3")
In this example, the condition x > 3 is true, so the code block under the if statement will be executed and the message “x is greater than 3” will be printed.
The elif statement is used to check additional conditions if the first condition in the if statement is false. Here is an example:
1x = 2
2if x > 5:
3 print("x is greater than 5")
4elif x > 2:
5 print("x is greater than 2 but less than or equal to 5")
6else:
7 print("x is less than or equal to 2")
In this example, the first condition (x > 5) is false, so the elif statement is checked. The second condition (x > 2) is true, so the code block under the elif statement will be executed and the message “x is greater than 2 but less than or equal to 5” will be printed.
The else statement is used to execute a block of code if none of the conditions in the if or elif statements are true. Here is an example:
1x = 1
2if x > 5:
3 print("x is greater than 5")
4elif x > 2:
5 print("x is greater than 2 but less than or equal to 5")
6else:
7 print("x is less than or equal to 2")
In this example, both the first condition (x > 5) and the second condition (x > 2) are false, so the else statement is executed and the message “x is less than or equal to 2” will be printed.
Loop statements are used to execute a block of code repeatedly as long as a certain condition is true. The three types of loop statements in Python are for loop, while loop, and break and continue statements.
The for loop is used to iterate over a sequence of elements and execute a block of code for each element. Here is an example:
1fruits = ["apple", "banana", "cherry"]
2for fruit in fruits:
3 print(fruit)
In this example, the for loop iterates over the sequence of fruits and executes the print statement for each fruit, printing “apple”, “banana”, and “cherry” on separate lines.
The while loop is used to execute a block of code as long as a certain condition is true. Here is an example:
1i = 1
2while i < 5:
3 print(i)
4 i += 1
In this example, the while loop executes the print statement for i = 1, i = 2, i = 3, and i = 4, printing these values on separate lines.
Break and Continue statements
The break statement is used to exit a loop prematurely, while the continue statement is used to skip over a certain iteration of a loop. Here’s an example of using break and continue in a for loop:
1# using break in a for loop
2for i in range(5):
3 if i == 3:
4 break
5 print(i)
6
7# using continue in a for loop
8for i in range(5):
9 if i == 3:
10 continue
11 print(i)
In the first loop, the code will print numbers 0, 1, and 2, but when i becomes equal to 3, the break statement will be executed, and the loop will terminate prematurely.
In the second loop, the code will print numbers 0, 1, 2, and 4, but when i becomes equal to 3, the continue statement will be executed, and the loop will skip the iteration where i is equal to 3.
Functions and Methods
Functions and methods are reusable blocks of code that perform a specific task. Functions and methods help to organize code into smaller, more manageable pieces. In Python, a function is defined using the “def” keyword, followed by the function name and parentheses, and then a colon.
Here’s an example of a simple function that takes two arguments and returns their sum:
1def add_numbers(x, y):
2 sum = x + y
3 return sum
This function takes two arguments, x and y, and returns their sum using the “+” operator. The “return” statement is used to specify what value the function should return.
Functions can take zero or more arguments. Arguments are values that are passed to a function when it is called. Parameters are the variables that are used to receive these arguments. In Python, you can define a function with default values for its parameters. Here’s an example:
1def say_hello(name="there"):
2 print("Hello, " + name + "!")
This function takes one parameter, “name”, which has a default value of “there”. If no value is passed for “name”, the default value is used. Here’s how you can call this function:
1say_hello() # prints "Hello, there!"
2say_hello("John") # prints "Hello, John!"
Returning Values from Functions
Functions can also return a value. Here’s an example of a function that returns the square of a number:
1def square(x):
2 return x * x
This function takes one argument, “x”, and returns its square using the “*” operator. Here’s how you can call this function:
1result = square(5)
2print(result) # prints 25
Lambda Functions
Functions without names are referred to as lambda functions or anonymous functions. They are often employed for brief, straightforward tasks that are performed only once. The “lambda” keyword is used to define lambda functions, and is then followed by the function body and arguments.
Here’s an example of a lambda function that returns the square of a number:
1square = lambda x: x * x
2result = square(5)
3print(result) # prints 25
Methods and Object-Oriented Programming
Methods are functions that are associated with objects in object-oriented programming. They are defined within a class and are used to perform operations on objects of that class. Here’s an example of a simple class with a method:
1class Dog:
2 def __init__(self, name):
3 self.name = name
4
5 def bark(self):
6 print("Woof! My name is " + self.name)
7
8my_dog = Dog("Fido")
9my_dog.bark() # prints "Woof! My name is Fido"
This code defines a class called “Dog” with a method called “bark”. The “bark” method prints a message with the dog’s name. We then create an instance of the “Dog” class and call the “bark” method on that instance. The output is “Woof! My name is Fido”.
Python Syntax in Practice
Writing Basic Programs – Now that we have learned the basics of Python syntax, it’s time to start writing some actual programs! To start, let’s write a program that prints out the lyrics to the song “99 Bottles of Beer on the Wall”.
If you’re new to programming, creating your first Python program can seem daunting. Learn how private tutoring can help you with your first Python program by offering step-by-step guidance and hands-on support.
Building small programs like this not only reinforces fundamental syntax but also lays the groundwork for tackling more complex projects with ease.
Here’s the code:
1for i in range(99, 0, -1):
2 print(f"{i} bottles of beer on the wall, {i} bottles of beer.")
3 print(f"Take one down, pass it around, {i-1} bottles of beer on the wall.\n")
This program uses a for loop to count down from 99 to 1, printing out the lyrics for each verse of the song.
Debugging Techniques – Even experienced programmers make mistakes from time to time, so it’s important to know how to debug your code when things go wrong. One common debugging technique is to use print statements to check the value of variables at different points in your code. For example:
1x = 5
2y = 7
3z = x + y
4print(f"The value of z is: {z}")
This program sets the variables x and y to the values 5 and 7, respectively, then adds them together and stores the result in the variable z. The print statement then displays the value of z on the screen, allowing you to check that the calculation was performed correctly.
Using Libraries and Modules– Python includes a wide variety of built-in libraries and modules that can be used to perform more advanced tasks. For example, the math module provides a variety of mathematical functions, such as sqrt() for calculating square roots and sin() for calculating sine values. Here’s an example of how to use the math module:
1import math
2x = 16
3y = math.sqrt(x)
4print(f"The square root of {x} is {y}")
This program imports the math module, then uses the sqrt() function to calculate the square root of the variable x. The result is stored in the variable y, which is then displayed on the screen.
File Input and Output- Python can also be used to read and write files on your computer. For example, here’s how to read the contents of a file named “myfile.txt” and display them on the screen:
1with open("myfile.txt", "r") as f:
2 contents = f.read()
3 print(contents)
This program uses the open() function to open the file named “myfile.txt” in read mode. The with statement ensures that the file is automatically closed when the program finishes. The read() function then reads the contents of the file into the variable contents, which is displayed on the screen using a print statement.
You can also write to files using the write() function, like this:
1with open("myfile.txt", "w") as f:
2 f.write("Hello, world!")
This program opens the file named “myfile.txt” in write mode, then writes the string “Hello, world!” to the file using the write() function.
Advanced Python Syntax
Advanced Control Flow Statements– Advanced control flow statements are used to control the flow of execution of a program. Some of the advanced control flow statements in Python include:
Try-Except: This statement is used to handle exceptions that occur in a program. It allows the programmer to anticipate possible errors and define what should be done when those errors occur. Here’s an example:
1try:
2 num = int(input("Enter a number: "))
3 print("The number is:", num)
4except ValueError:
5 print("Invalid input. Please enter a valid number.")
In this example, the program asks the user to enter a number. If the user enters a string instead of a number, a ValueError will occur. The try-except statement is used to handle this error and print a custom error message.
While-Else: This statement is used to execute a block of code when the while loop completes successfully. Here’s an example:
1i = 0
2while i < 5:
3 print(i)
4 i += 1
5else:
6 print("The while loop has completed successfully.")
In this example, the program prints the values of i from 0 to 4 using a while loop. After the while loop completes successfully, the else block is executed and a message is printed.
Decorators and generators are advanced features in Python that allow programmers to modify the behavior of functions and create iterators, respectively.
Decorators: A decorator is a function that takes another function as input and returns a modified version of that function. Decorators are used to add functionality to existing functions without modifying their code. Here’s an example:
1def my_decorator(func):
2 def wrapper():
3 print("Before the function is called.")
4 func()
5 print("After the function is called.")
6 return wrapper
7
8@my_decorator
9def say_hello():
10 print("Hello, World!")
11
12say_hello()
In this example, the my_decorator function is defined as a decorator. The say_hello function is decorated using the @my_decorator syntax. When say_hello is called, the decorator modifies its behavior by adding print statements before and after the function call.
Generators: A generator is a type of iterator that is defined using a function. Generators allow programmers to create iterators that are more memory-efficient than traditional iterators. Here’s an example:
1def my_range(n):
2 i = 0
3 while i < n:
4 yield i
5 i += 1
6
7for x in my_range(5):
8 print(x)
In this example, the my_range function is defined as a generator. When the my_range function is called, it returns an iterator that generates values from 0 to n-1. The for loop is used to iterate over the values generated by the iterator and print them.
Exception handling is the process of anticipating possible errors and defining what should be done when those errors occur. Python provides several built-in exceptions that can be used to handle errors in a program.
Here’s an example of exception handling in Python:
1try:
2 num = int(input("Enter a number: "))
3 result = 10 / num
4 print("The result is:", result)
5except ValueError:
6 print("Invalid input. Please enter a valid number.")
7except ZeroDivisionError:
8 print("Cannot divide by zero.")
In this example, the program asks the user to enter a number. If the user enters a string instead of a number, a ValueError will occur. If the user enters zero, a ZeroDivisionError will occur. In such situations, we can use exception handling to gracefully handle the errors and prevent the program from crashing. Here is an example of how exception handling can be used to handle errors:
1try:
2 num = int(input("Enter a number: "))
3 result = 100 / num
4except ValueError:
5 print("Invalid input. Please enter a valid integer.")
6except ZeroDivisionError:
7 print("Cannot divide by zero.")
8else:
9 print("Result is:", result)
10finally:
11 print("Program execution complete.")
In this example, the program uses a try-except block to catch any ValueError or ZeroDivisionError that may occur. If a ValueError occurs, the program will print an error message asking the user to enter a valid integer. If a ZeroDivisionError occurs, the program will print an error message stating that division by zero is not possible. If neither error occurs, the program will print the result.
The else block is executed if no exceptions are raised in the try block. The finally block is always executed, whether an exception is raised or not. It is used for any cleanup operations that need to be performed before the program exits.
Regular Expressions
Regular expressions are a powerful tool for pattern matching and text manipulation. They are widely used in data processing, text analysis, and web development.
Here is an example of how regular expressions can be used to find all occurrences of a specific pattern in a string:
1import re
2text = "The quick brown fox jumps over the lazy dog."
3pattern = r"\b\w{4}\b"
4matches = re.findall(pattern, text)
5print(matches)
In this example, the program uses the re.findall() function to find all occurrences of words that are exactly four letters long. The r”\b\w{4}\b” pattern matches any word boundary (\b) followed by exactly four word characters (\w{4}) and another word boundary. The findall() function returns a list of all matches found in the text. In this case, it will print [‘quick’, ‘brown’, ‘jumps’, ‘over’, ‘lazy’, ‘dog’].
Regular expressions can be used for a wide range of pattern matching tasks, such as finding email addresses, phone numbers, or specific words or phrases in a text.
Conclusion
In this guide, we covered the basics of Python syntax including variables and data types, operators, control flow statements, functions and methods, and advanced syntax including decorators, generators, exception handling, and regular expressions. We also looked at how to write basic programs, debugging techniques, using libraries and modules, and file input and output. By understanding Python syntax, you can write efficient and effective code for a variety of applications.
Python syntax can seem intimidating at first, but with practice and patience, you can become proficient in writing Python code. Remember to take advantage of Python’s built-in functions and libraries to simplify your code and make it more efficient. Don’t be afraid to ask for help from the Python community or refer to online resources for guidance.
If you are struggling with errors in your Python assignments related to syntax, private tutoring can provide personalized support to help you master these concepts and complete your assignments confidently. Don’t be afraid to ask for help from the Python community or refer to online resources for guidance.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is the Python syntax?
The syntax for Python is characterized by its use of whitespace indentation to denote code blocks. It also uses keywords and symbols such as colons and parentheses to indicate the beginning and end of code blocks and function definitions.
What is an example of Python syntax?
An example of Python syntax is:
x = 10
if x > 5:
print(“x is greater than 5”)
else:
print(“x is less than or equal to 5”)
How many different types of basic syntax are there in Python?
There is no definitive answer to this question, as it depends on how one defines “basic syntax.” Some sources may list a few dozen basic syntax elements, while others may list hundreds or more.
How easy is Python syntax?
Many people find Python syntax relatively easy to learn and use, particularly compared to other programming languages. The use of whitespace indentation and clear, concise syntax can make Python code easier to read and understand.
What is the basic syntax?
In Python, the basic syntax includes the use of whitespace indentation to denote code blocks, the use of keywords such as “if,” “else,” and “while” to control program flow, and the use of functions and modules to organize code.
What is Python Class syntax?
In Python, Class syntax allows you to create objects with their own methods and attributes. It involves defining a class with a set of methods and attributes, and then creating objects or instances of that class to use in your code.
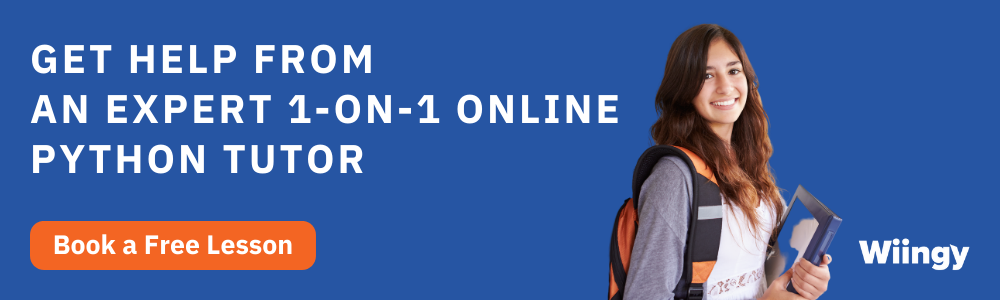
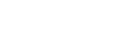
Jan 30, 2025
Was this helpful?