Python Tutorials
The pass statement is one of the features that makes Python a user-friendly programming language.
The Python Pass Statement is a null statement that has no effect in Python programming. When the code block must contain a statement but no action is required, this placeholder statement is used. The pass statement is a way of telling Python that nothing should happen.
The pass statement is used in Python programming when you need to have a statement in a code block but you do not want any action to be taken. For instance, if you are writing a program and you need to add a function that you will implement later, you can use the pass statement to let Python know that you are not ready to implement the function at that moment.
It is also used to create a structure or template for future use. Additionally, it enables programmers to write code in a more structured manner.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Syntax of the pass statement in Python
A. Explanation of the pass statement syntax:
The syntax of the pass statement is simple. It consists of the keyword pass followed by a colon (:). The pass statement is used to indicate that the code block is empty and that no action is required.
B. Code example of the pass statement:
The following code example shows how the pass statement can be used in Python programming:
# Example 1: Using pass statement in a function def my_function(): pass
# Example 2: Using pass statement in a loop for i in range(5): pass
# Example 3: Using pass statement in a class class MyClass: pass
In example 1, the pass statement is used in a function to create an empty code block. In example 2, the pass statement is used in a loop to create an empty loop body. In example 3, the pass statement is used in a class to create an empty class body.
In conclusion, the pass statement is a useful feature in Python programming. It allows programmers to create empty code blocks without generating errors and is used to create a structure or template for future use. As a result, it is an essential concept that every Python programmer should know.
How the pass statement in Python works
The pass statement is used in Python programming to create an empty or insufficient code block. The pass statement has no effect on the Python interpreter; it simply causes it to move on to the next statement. The pass statement instructs Python to do nothing and serves as a placeholder. Because of this, it can be helpful in situations where you want to define a function, class, or loop but are unsure of how it will be implemented.
A. Code example of the pass statement in action:
Consider the following code example:
def my_function(): pass for i in range(5): pass
In the first line of the code, we have defined a function called my_function() that does nothing. The pass statement is used in place of the function body, indicating that we do not know what the function should do yet. Similarly, in the second line of the code, we have defined a loop with the pass statement in the loop body. This tells Python that the loop does nothing for now.
B. Comparison with other control statements:
The pass statement is different from other control statements in Python, such as the break, continue and return statements. While these statements alter the flow of control in a program, the pass statement does not. The pass statement simply tells Python to do nothing. This makes it useful for creating a placeholder for future code implementation, as we will discuss in the next section.
Use cases of the pass statement:
The pass statement is frequently used as a placeholder for later code implementation. When writing a program and need to add a function or class that you will later implement, this is especially helpful. You can create a function or class that is empty and won’t produce any errors when the program is run by using the pass statement.
- Placeholder for code to be implemented by others:
When working in a team, the pass statement is also beneficial. You can use the pass statement to make a placeholder for code that will be implemented by others if you’re working on a project with other programmers. As a result, you can define the code’s structure without being aware of the specifics of its implementation.
- Handling unexpected exceptions or errors:
The pass statement can be used to deal with unforeseen errors or exceptions. If your program encounters an exception or error that you did not anticipate, you can use the pass statement to ignore it and continue with the program execution. This can prevent your program from crashing or generating errors.
- Code examples of the pass statement in different scenarios:
# Example 1: Using pass statement to handle exceptions try: # Some code that may raise an exception except Exception: pass
# Example 2: Using pass statement as a placeholder for future code def my_function(): pass
# Example 3: Using pass statement to define a class with empty body class MyClass: pass
In Example 1, the pass statement is used to handle an unexpected exception. If the code block in the try statement raises an exception, the pass statement is executed and the program continues. In Example 2, the pass statement is used as a placeholder for future code implementation. In Example 3, the pass statement is used to define a class with an empty body.
Limitations of the pass statement:
The pass statement has the drawback of producing unused and superfluous code. If you use the pass statement too frequently, your code might become useless. Your program may become more challenging to read and maintain as a result.
Conclusion:
A. Summary of the importance and limitations of the pass statement:
In conclusion, the pass statement is a straightforward but crucial aspect of Python programming. It is used to create empty or incomplete code blocks, and as a placeholder for future code implementation or code to be implemented by others. The pass statement is also useful for handling unexpected exceptions or errors. However, overuse of the pass statement can result in unused and unnecessary code.
B. Recommendations for using the pass statement effectively and avoiding common mistakes:
It is advised to use the pass statement sparingly and only when necessary in order to use it effectively. It’s crucial to watch out for the pass statement being used to hide logical mistakes or add extra code. It ought to be utilized as a stand-in for future or future-to-be-implemented code instead. To make your code more readable and maintainable, it’s also crucial to properly document the use of the pass statement in it.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs:
What is an example for pass statement in Python?
An example of the pass statement in Python is as follows:
def my_function(): pass
What is pass in Python and give one example?
In Python, pass is a null statement that does nothing. An example of pass in Python is as follows:
for i in range(5): pass
What is break and pass statement in Python?
Break and pass are both control statements in Python, but they serve different purposes. Break is used to terminate a loop or switch statement, while pass is used as a placeholder for future code implementation or for code to be implemented by others.
Is pass an empty statement in Python?
Yes, pass is an empty statement in Python that does nothing.
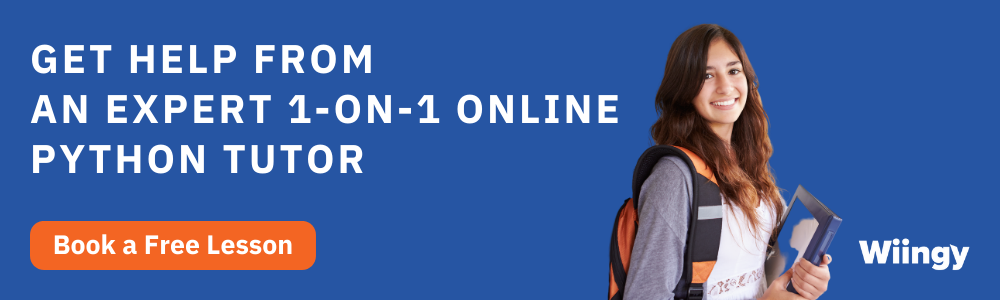
Written by by
Rahul LathReviewed by by
Arpit Rankwar