Python Tutorials
What are Comparison Operators in Python?
Comparison operators are used to determine whether two values are greater than, less than, equal, or greater than or equal to each other when they are compared. In Python, the following comparison operators are available:
- == (equal to)
- != (not equal to)
- > (greater than)
- < (less than)
- >= (greater than or equal to)
- <= (less than or equal to)
Programming requires the use of comparison operators because they enable condition-based decision-making. For instance, comparison operators can be used to determine whether a number is positive or negative or whether a string equals a specific value. They are also used in loops and conditional statements to control program flow.
Basic Concepts of Chaining Comparison Operators:
Using multiple comparison operators in a single line of code to compare multiple values is known as chaining comparison operators. To create complex comparisons in Python, we can chain comparison operators together. For example, we can chain two or more comparison operators to check if a value falls within a range of values.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Chaining Comparison Operators in Python
By connecting several comparison operations using logical operators, Python’s chaining comparison operators allows for the simplification of multiple comparison operations.
In a chained comparison, logical operators like and, or, and are used to combine two or more comparison operators. This enables you to use a single expression to compare various values or variables.
A. Definition:
In order to compare multiple values, Python allows you to chain comparison operators together in a single expression. In comparison to using a single comparison operator, this enables us to perform comparisons that are more intricate.
Can You Chain == in Python?
Yes, you can chain == in Python. For example, a == b == c is a valid expression in Python, which means that a, b, and c are all equal. However, it’s important to note that chaining == can sometimes be confusing and lead to unexpected results, especially if non-constant expressions are involved.
Non-Transitive Operators:
Some comparison operators, such as > and <, are transitive, which means that if a > b and b > c, then a > c. However, some comparison operators, such as ==, are not transitive, which means that if a == b and b == c, it does not necessarily mean that a == c.
Non-Constant Expressions:
When chaining comparison operators, it’s important to be aware of non-constant expressions, which can lead to unexpected results. For example, a < b < a + 1 is not the same as a < b and b < a + 1, because the expression a + 1 is evaluated each time the expression is executed.
Ugly Chain:
Chaining comparison operators can sometimes result in long and difficult to read expressions, which can be referred to as an “ugly chain”. It’s important to use parentheses to clarify the order of operations when chaining comparison operators.
B. Examples:
- Chaining Two Comparison Operators:
We can chain two comparison operators to compare two values. For example:
x = 5 y = 10 if 1 < x < 10: print("x is between 1 and 10") if x < y and y < 20: print("x is less than y and y is less than 20")
- Chaining Three or More Comparison Operators:
We can chain three or more comparison operators to make more complex comparisons. For example:
x = 5 y = 10 z = 15 if x < y < z: print("x is less than y, which is less than z") if x == y == z: print("x, y, and z are all
Comparison Operators in Python
A. Comparison Operator Syntax:
The syntax for comparison operators in Python is as follows:
- == (equal to)
- != (not equal to)
- > (greater than)
- < (less than)
- >= (greater than or equal to)
- <= (less than or equal to)
- is (object identity)
- is not (negated object identity)
- in (membership)
- not in (negated membership)
B. Examples:
- == Operator:
The == operator compares two values to check if they are equal. For example:
x = 5 y = 5 if x == y: print("x and y are equal")
- != Operator:
The != operator compares two values to check if they are not equal. For example:
x = 5 y = 10 if x != y: print("x and y are not equal")
- < and > Operators:
The < and > operators compare two values to check if one is less than or greater than the other. For example:
x = 5 y = 10 if x < y: print("x is less than y") if y > x: print("y is greater than x")
- <= and >= Operators:
The <= and >= operators compare two values to check if one is less than or equal to, or greater than or equal to, the other. For example:
x = 5 y = 5 if x <= y: print("x is less than or equal to y") if y >= x: print("y is greater than or equal to x")
- is and is not Operators:
The is and is not operators compare the object identity of two values. For example:
x = [1, 2, 3] y = [1, 2, 3] z = x if x is z: print("x and z refer to the same object") if x is not y: print("x and y refer to different objects")
- in and not in Operators:
The in and not in operators check if a value is a member of a sequence, such as a string or list. For example:
x = "hello" if "h" in x: print("h is in x") if "a" not in x: print("a is not in x")
Logical Operators in Python
A. Logical Operator Syntax:
The syntax for logical operators in Python is as follows:
- and (logical and)
- or (logical or)
- not (logical not)
B. Examples:
- and Operator:
The and operator returns True if both expressions evaluate to True. For example:
x = 5 y = 10 z = 15 if x < y and y < z: print("x is less than y, which is less than z")
- or Operator:
The or operator returns True if at least one expression evaluates to True. For example:
x = 5 y = 10 if x < y or y > 20: print("either x is less than y, or y is greater than 20")
- not Operator:
The not operator returns the opposite boolean value of the expression that follows it. For example:
x = 5 y = 10 if not(x < y): print("x is not less than y")
This will print nothing because the expression x < y is True, but when we apply not to it, the resulting value is False.
It is worth noting that the logical operators in Python have short-circuit evaluation. This means that if the first operand of an and expression is False, the second operand is not evaluated, and if the first operand of an or expression is True, the second operand is not evaluated. This can lead to more efficient code and prevent potential errors.
Operator Precedence and Associativity
Operator precedence determines the order in which operators are evaluated in an expression. For example, multiplication and division have a higher precedence than addition and subtraction. Associativity, on the other hand, determines the order in which operators of the same precedence are evaluated. For example, addition and subtraction have left-to-right associativity, which means that expressions are evaluated from left to right. In Python, operator precedence and associativity are defined by the language specification.
Examples:
Consider the following expression:
x = 2 + 3 * 4
According to operator precedence, multiplication has a higher precedence than addition, so the expression is evaluated as follows:
x = 2 + (3 * 4) # x is now 14
Consider the following expression:
x = 10 - 5 + 2
According to associativity, expressions are evaluated from left to right when they have the same precedence. So, the expression is evaluated as follows:
x = (10 - 5) + 2 # x is now 7
Best Practices for Chaining Comparison Operators
Look at the best practices for Chaining Comparison Operators:
Use Parentheses to Control Order of Operations:
When chaining comparison operators, it is often a good idea to use parentheses to make the order of operations clear. For example, consider the following expression:
x = 1 < 2 < 3 == 3
This expression is equivalent to:
x = (1 < 2) and (2 < 3) and (3 == 3)
Using parentheses makes the order of operations clear and can help prevent errors.
B. Use Clear and Concise Code:
When chaining comparison operators, it is important to write clear and concise code. Avoid using long chains of operators or nested parentheses, as this can make your code difficult to read and understand.
C. Test Your Code with Various Inputs:
When writing code that chains comparison operators, it is important to test your code with various inputs to ensure that it works correctly in all cases.
D. Avoid Complex Chains:
Finally, it is generally a good idea to avoid complex chains of comparison operators whenever possible. Complex chains can be difficult to read and understand, and can also be error-prone. Instead, try to break down complex chains into smaller, more manageable pieces.
Conclusion
Chaining comparison operators is a technique in programming where multiple comparison operators are used together to create a more complex expression. In Python, comparison operators are used to compare two values and return a boolean value (True or False). Chaining comparison operators allows for more complex logical expressions to be written, which can be useful in a variety of programming contexts.
Tips for Using Comparison Operators in Python:
- Always use the correct syntax for comparison operators in Python.
- Use parentheses to control the order of operations in chained comparison expressions.
- Use clear and concise code to make your code easier to read and understand.
- Test your code with various inputs to ensure that it works correctly in all cases.
- Avoid complex chains of comparison operators whenever possible.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is chaining comparison operators?
Chaining comparison operators is a technique in programming where multiple comparison operators are used together to create a more complex expression. This allows for more complex logical expressions to be written, which can be useful in a variety of programming contexts.
What is chaining comparison operators in Python?
In Python, chaining comparison operators involves using multiple comparison operators in a single expression to create a more complex logical expression. This allows for more complex logical expressions to be written, which can be useful in a variety of programming contexts.
What are the 3 types of comparison operators?
The three types of comparison operators are:
Equality operators: == and !=
Ordering operators: <, >, <=, and >=
Identity operators: is and is not
What are 4 state 4 comparison operators available in Python?
Python Comparison Operators
These operators are equal, not equal, greater than, less than, greater than or equal to and less than or equal to.
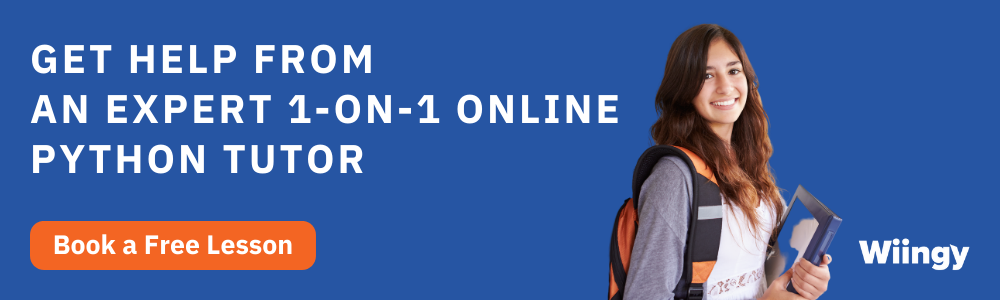
Written by
Rahul LathReviewed by
Arpit Rankwar