The continue statement is one of Python’s most useful constructs because it allows the programmer to direct how the code in a loop is executed.
The Python Continue Statement is used to jump to the next iteration of a loop without executing the remaining code in the current iteration. It is typically used within a loop to skip over certain iterations based on certain conditions.
The loop’s execution can be steered in a more precise direction with the help of the continue statement. When working with large datasets or complex algorithms, developers often find it helpful to be able to skip over certain iterations of a loop based on certain conditions.
Python’s continue statement is a game-changer for programmers looking to improve their code’s efficiency and effectiveness. When working with large datasets or computationally intensive algorithms, saving time and resources by skipping over unnecessary iterations can be invaluable.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Syntax of Continue statement
The syntax of the continue statement in Python is very simple. It consists of the keyword “continue” followed by a semicolon (;). Here’s an example:
1for i in range(10): if i % 2 == 0: continue print(i)
In this example, the “for” loop iterates over the range from 0 to 9. The “if” statement checks if the current value of “i” is even. If it is, the “continue” statement is executed, which skips over the remaining code in the current iteration of the loop and moves on to the next iteration. If it is not even, the print() function is called, which outputs the current value of “i”.
A. Code example of continue statement
Here’s another example that shows how the continue statement can be used to skip over certain iterations of a loop:
1numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] for num in numbers: if num % 2 == 0: continue print(num)
In this example, the “for” loop iterates over the list of numbers from 1 to 10. The “if” statement checks if the current number is even. If it is, the “continue” statement is executed, which skips over the remaining code in the current iteration of the loop and moves on to the next iteration. If it is not even, the print() function is called, which outputs the current number.
Overall, the continue statement is a powerful tool for controlling the flow of execution within a loop in Python. By using it effectively, developers can write more efficient and effective code that can handle complex tasks with ease.
How continue statement works
The current loop iteration is immediately finished when the continue statement is encountered within a loop, and the next loop iteration begins. This means that the loop will continue as if the current iteration had never happened, skipping over any remaining code within the current iteration.
A. Explanation of how continue statement skips iteration
The Python interpreter immediately executes the next iteration of the loop after the continue statement is executed, skipping any remaining code in the current iteration. This enables the programmer to skip over particular loop iterations based on specific criteria.
B. Code example of continue statement in action
Here’s an example that shows how the continue statement can be used to skip over certain iterations of a loop:
1for i in range(10): if i % 2 == 0: continue print(i)
In this example, the “for” loop iterates over the range from 0 to 9. The “if” statement checks if the current value of “i” is even. If it is, the “continue” statement is executed, which skips over the remaining code in the current iteration of the loop and moves on to the next iteration. If it is not even, the print() function is called, which outputs the current value of “i”.
C. Comparison with other control statements
The break statement and the pass statement, two other Python control statements, are frequently contrasted with the continue statement. The continue statement is used to skip over some iterations of a loop depending on certain conditions, whereas the break statement is used to exit a loop entirely and the pass statement is used to do nothing (and is frequently used as a placeholder).
Use cases of continue statement
In Python, the continue statement is a potent tool for managing how a loop executes its code. Here are some common use cases for the continue statement:
A. Skipping loop iterations with specific conditions
The continue statement is frequently used to skip over specific loop iterations based on predetermined criteria. For instance, you might want to skip over any values that satisfy a particular requirement in a loop that iterates over a list of values. In this situation, you can skip over those values and proceed to the following iteration by using the continue statement.
B. Simplifying complex nested loops
The continue statement can also be used to streamline intricate nested loops. In some circumstances, you might have a loop inside another loop and want to skip over specific iterations of the inner loop depending on specific circumstances. You can avoid those iterations and make your code simpler by utilizing the continue statement.
C. Improving code readability and maintainability
The continue statement can also be used to improve code readability and maintainability. By using the continue statement to skip over certain iterations of a loop, you can make your code more concise and easier to understand. This can make it easier for other developers to read and maintain your code in the future.
D. Code examples of continue statement in different scenarios
Here are a few examples of how the continue statement can be used in different scenarios:
Example 1: Skipping over even numbers in a loop
1for i in range(10): if i % 2 == 0: continue print(i)
Example 2: Skipping over vowels in a string
1word = "hello world" for letter in word: if letter in "aeiou": continue print(letter)
Limitations of continue statement
There are some restrictions and potential pitfalls to be aware of even though the continue statement can be a powerful tool for managing the execution of a loop.
A. Misuse of continue statement leading to logical errors
Misusing the continue statement in a way that causes logical mistakes in your code is a common error when using it. For instance, you risk getting unexpected outcomes or even infinite loops if you use the continue statement to skip over some iterations of a loop but fail to update the variables or data structures properly.
B. Risk of creating an infinite loop
The risk of inadvertently creating an infinite loop exists when using the continue statement. You risk creating a loop that never ends if you use the continue statement to skip over too many loop iterations or if you incorrectly update your loop variables. It may be very challenging to troubleshoot this and may cause your program to hang or crash.
C. Code examples of continue statement misuse
Here are a few examples of how the continue statement can be misused, leading to logical errors or infinite loops:
Example 1: Misuse of continue statement in a while loop
1i = 0 while i < 10: if i == 5: continue print(i) i += 1
In this example, we use the continue statement to skip over the value of i when it equals 5. However, we don’t increment i inside the loop, so we end up with an infinite loop that prints out the value of 0 repeatedly.
Example 2: Skipping over too many iterations in a for loop
1for i in range(10): if i % 2 == 0: continue i += 2 print(i)
In this example, we use the continue statement to skip over any even values of i. However, we then try to increment i by 2 inside the loop, which effectively skips over every other odd value of i. This results in only half of the expected values being printed out, and can cause logical errors in more complex programs.
Conclusion
In conclusion, Python’s continue statement is an effective tool for managing the execution of a loop. However, it is important to use it correctly and avoid common mistakes that can lead to logical errors or infinite loops.
To use the continue statement effectively and avoid common mistakes, it is important to:
- Make sure to update your loop variables or data structures correctly when using the continue statement to skip over iterations.
- Avoid skipping over too many iterations of a loop, as this can cause logical errors or infinite loops.
- Test your code thoroughly to make sure it behaves as expected, especially when using the continue statement in complex algorithms or programs.
By following these recommendations, you can use the continue statement effectively and write more efficient and effective code in Python.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
Q: What is break and continue statement in Python?
The continue statement is used to skip over specific iterations of a loop based on specific conditions, whereas the break statement is used to exit a loop.
Q: What does the continue statement do?
A: In Python, the continue statement is used to skip the remaining lines of code in an iterative loop and move on to the next one.
Q: What is the advantage of continue statement in Python?
The continue statement in Python has the benefit of enabling programmers to write more effective and efficient code by omitting pointless loop iterations, which can be crucial when working with large datasets or computationally demanding algorithms.
Q: What is the syntax for continue Statement?
The syntax for the continue statement in Python is very simple. It consists of the keyword “continue” followed by a semicolon (;).
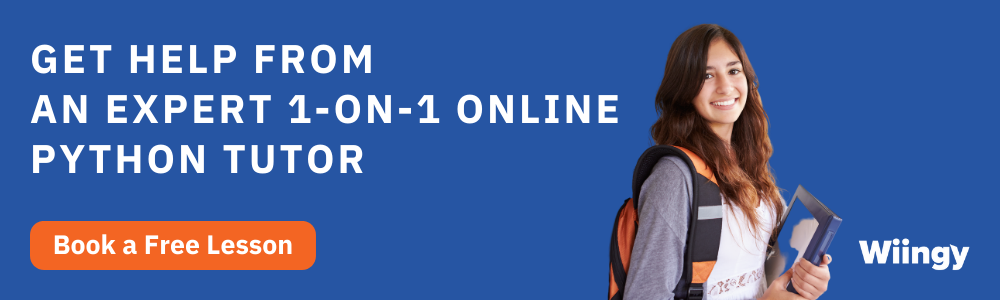
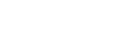
Jan 30, 2025
Was this helpful?