What is Polymorphism in Python?
The ability of an object or function to assume various forms is referred to as polymorphism, which is a fundamental concept in computer programming. This idea is crucial for programmers to comprehend because it enables developers to write more adaptable and reusable code. The function of polymorphism in Python and its significance in programming will be discussed in this guide.
Polymorphism is derived from the Greek words “morph” and “poly,” which both mean many. In programming, it refers to the ability of an object or function to take on multiple forms. This implies that depending on the circumstance, the same object or function can be used in a variety of ways. Programmers can create more adaptable, reusable, and upkeep-free code by using polymorphism.
Programming requires polymorphism because it reduces code duplication, enhances code organization, and makes code easier to read. Developers can create more modular, testable code by allowing objects and functions to take on various forms. A further benefit of polymorphism is that it makes it simpler to write code that works with a variety of data types, enabling programmers to create more adaptable and reusable software.
Polymorphism is supported in Python, a dynamically typed language, at both the function and object levels. Accordingly, Python objects and functions can adopt various forms based on the situation in which they are used. In Python, polymorphism is accomplished by combining operator overloading, function overloading, function overriding, and duck typing.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Polymorphism in Functions
Python supports polymorphism in functions through function overloading and function overriding. Functions are a fundamental building block of programming.
A. Function Overloading
Developers can define multiple functions with the same name but different arguments by using the function overloading technique. Python evaluates the arguments passed to a function when it is called and calls the appropriate version of the function. As a result, developers can create code that is more adaptable and reusable.
For example, consider the following code:
1def add(a, b):
2 return a + b
3
4def add(a, b, c):
5 return a + b + c
In this example, we have defined two functions with the same name, “add”, but different numbers of arguments. When the “add” function is called, Python checks the number of arguments passed to the function and calls the appropriate version of the function.
Function Overriding
Using the technique of “function overriding,” programmers can define a new version of an existing function in a subclass. This enables programmers to change a function’s behavior without changing the original function. The version of the function in the subclass is called when the function is called rather than the version in the parent class.
For example, consider the following code:
1class Animal:
2 def make_sound(self):
3 print("The animal makes a sound.")
4
5class Cat(Animal):
6 def make_sound(self):
7 print("The cat meows.")
8
9class Dog(Animal):
10 def make_sound(self):
11 print("The dog barks.")
In this example, we have defined three classes: Animal, Cat, and Dog. The Animal class has a method called “make_sound”, which prints a generic message. The Cat and Dog classes override the “make_sound” method to print a more specific message.
Polymorphism in Objects
Developers can use operators and functions in various ways depending on the type of object being used thanks to polymorphism in objects. In Python, operator overloading and duck typing are used to create polymorphic objects.
A. Operator Overloading
Developers can specify the behavior of operators (+, -, *, /, etc.) for their unique objects by using operator overloading. This makes code more readable and simple to use by enabling objects to be used with operators in the same way as built-in types.
For example, consider the following code:
1class Point:
2 def __init__(self, x, y):
3 self.x = x
4 self.y = y
5
6 def __add__(self, other):
7 return Point(self.x + other.x, self.y + other.y)
8
9p1 = Point(1, 2)
10p2 = Point(3, 4)
11p3 = p1 + p2
12print(p3.x, p3.y)
In this example, we have defined a class called “Point”, which represents a point in two-dimensional space. We have defined the add method, which allows us to use the “+” operator to add two Point objects together. When the “+” operator is used with two Point objects, Python calls the add method to perform the addition.
B. Duck Typing
Python’s “duck typing” idea enables programmers to use objects based on their behavior rather than their type. This means that regardless of an object’s actual type, it can be used as that type if it behaves like that type. Developers can work with objects that have different types but similar behavior by using duck typing, which makes their code more flexible and reusable.
For example, consider the following code:
1class Duck:
2 def quack(self):
3 print("Quack!")
4
5class Person:
6 def quack(self):
7 print("I'm quacking like a duck!")
8
9def make_quack(obj):
10 obj.quack()
11
12duck = Duck()
13person = Person()
14
15make_quack(duck)
16make_quack(person)
In this example, we have defined a class called “Duck” and a class called “Person”. Both classes have a method called “quack”, which prints a message. We have also defined a function called “make_quack”, which takes an object and calls its “quack” method. The function works with both the Duck and Person objects because they both have a “quack” method, even though they are different types.
Advantages of Polymorphism
Programmers can benefit from polymorphism’s code reuse, flexibility, extensibility, and simpler debugging.
A. Code Reusability
By enabling programmers to reuse the same code for various data types, polymorphism encourages code reuse. As a result, less code needs to be written and code maintenance is made simpler over time.
B. Flexibility
Code can be more flexible thanks to polymorphism, which enables objects and functions to take on different forms. Because of this, it is simpler to write code that works with various data types, enabling developers to produce more adaptable and reusable software.
C. Extensibility
Polymorphism makes it easier to extend existing code by allowing developers to modify the behavior of existing functions and objects without modifying the original code. This makes it easier to add new features and functionality to existing software.
D. Easier Debugging
By encouraging modularity in the code and minimizing duplication, polymorphism can facilitate debugging. Bugs are simpler to find and address when code is written in a modular manner, and the likelihood of bugs being introduced in the first place is decreased by cutting down on code duplication.
Examples of Polymorphism in Python
Python uses polymorphism, a fundamental idea, in many different parts of the language. Here are some examples of polymorphism in Python:
A. Function Overloading example
1def add(a, b):
2 return a + b
3
4def add(a, b, c):
5 return a + b + c
6
7print(add(1, 2)) # Output: 3
8print(add(1, 2, 3)) # Output: 6
B. Function Overriding example
1class Animal:
2 def make_sound(self):
3 print("The animal makes a sound.")
4
5class Cat(Animal):
6 def make_sound(self):
7 print("The cat meows.")
8
9class Dog(Animal):
10 def make_sound(self):
11 print("The dog barks.")
12
13my_pet = Cat()
14my_pet.make_sound() # Output: The cat meows.
C. Operator Overloading example
1class Point:
2 def __init__(self, x, y):
3 self.x = x
4 self.y = y
5
6 def __add__(self, other):
7 return Point(self.x + other.x, self.y + other.y)
8
9p1 = Point(1, 2)
10p2 = Point(3, 4)
11p3 = p1 + p2
12print(p3.x, p3.y) # Output: 4 6
D. Duck Typing example
1class Duck:
2 def quack(self):
3 print("Quack!")
4
5class Person:
6 def quack(self):
7 print("I'm quacking like a duck!")
8
9def make_quack(obj):
10 obj.quack()
11
12duck = Duck()
13person = Person()
14
15make_quack(duck) # Output: Quack!
16make_quack(person) # Output: I'm quacking like a duck!
Polymorphism vs. Inheritance
In object-oriented programming, polymorphism and inheritance are related ideas, but they have different functions.
A. Explanation of Inheritance
In object-oriented programming, the mechanism of inheritance enables the creation of new classes based on preexisting classes. The superclass, an existing class from which the new class, known as a subclass, inherits its methods and properties. This makes it simpler to create complex software systems and permits code reuse.
B. Differences between Polymorphism and Inheritance
While both polymorphism and inheritance encourage modularity and code reuse, their objectives are different. While new classes can be created based on existing classes through inheritance, polymorphism allows objects and functions to take on multiple forms. While inheritance encourages code reuse and modularity, polymorphism promotes flexibility and extensibility.
C. How Polymorphism and Inheritance are used together
In object-oriented programming, polymorphism and inheritance are frequently combined to produce more adaptable and reusable code. While polymorphism enables developers to change the behavior of those classes without altering the original code, inheritance enables developers to reuse code from pre-existing classes. This makes it simpler to gradually add new functionality to software systems and to expand the existing code. Polymorphism can also be used to create abstract classes, which can be used as models for other classes to be derived from. It is possible to build software systems that are more modular, extensible, and maintainable by combining polymorphism and inheritance.
Polymorphism in Classes
Polymorphism in classes refers to a class’s capacity to assume various forms. In Python, method overriding and abstract classes are used to create polymorphic classes.
A. Abstract Classes
Abstract classes serve as models for other classes to inherit from even though they cannot be instantiated. Any class that derives from an abstract class must implement the set of methods that they define. By specifying a set of behaviors that all classes ought to implement, this enables developers to produce more modular and extensible code.
B. Method Overriding
Method overriding is a technique that allows subclasses to override the methods of their parent classes. Programmers can therefore change the behavior of existing methods without altering the source code. The version in the subclass is called when the overridden method is invoked rather than the version in the parent class.
Conclusion
To sum up, polymorphism is a crucial Python concept that enables programmers to create more adaptable, reusable, and maintainable code. Function overloading, function overriding, operator overloading, duck typing, abstract classes, and method overriding are all used in combination to achieve polymorphism.
By comprehending polymorphism, programmers can build software systems that are more extensible, maintainable, and modular so they can grow over time to meet changing needs.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is polymorphism in Python?
Python’s polymorphism concept enables objects and functions to take on various forms. In object-oriented programming, it is a fundamental idea that encourages adaptability and code reuse.
What is polymorphism and its types in Python?
Duck typing, function overloading, function overriding, operator overloading, abstract classes, and method overriding are all techniques used in Python to achieve polymorphism.
What is polymorphism and example?
Polymorphism is a concept in programming that refers to the ability of an object or function to take on multiple forms. An example of polymorphism in Python is operator overloading, where custom objects can be used with operators (+, -, *, /) in the same way as built-in types.
What is polymorphism in Python real example?
Use of the “+” operator with various data types, such as adding two integers, two floats, or two strings, is a practical illustration of polymorphism in Python. The versatility and reuse of polymorphism can be seen in the fact that the same operator can be applied to various data types.
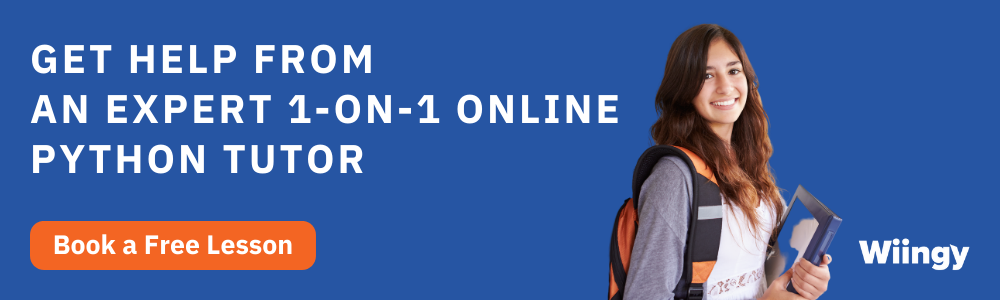
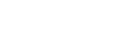
Jan 30, 2025
Was this helpful?