Python Tutorials
In Python, a generator is a special kind of function that allows you to produce a series of values one at a time rather than all at once. Python Generators can be iterated over using a for loop or other iteration techniques by returning an iterator object when it is called.
Generators only produce a new value when the generator is called again. They return values from functions one at a time using the “yield” keyword.
The goal of generator in python is to offer an effective method for producing lengthy data sequences without having to do so all at once.
Generators in python are an important because they let programmers make long sequences of data quickly and with little use of memory. They also enable the creation of infinite sequences, which is useful for a wide range of applications, such as mathematical functions and simulations.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Understanding Generators in Python
The “yield” keyword instructs a generator to output a string of values one at a time. When a generator function is called, it returns an iterator object that can be iterated over using a for loop or other iteration methods. Each time the generator is called, it resumes execution from the point where it last yielded a value and continues until the next “yield” statement is encountered.
Comparison with other Python data structures and functions:
Although Python’s generators and other data structures and functions are similar, they also differ significantly in several key ways. For instance, because generators don’t produce all the data at once, they use less memory than lists. As they can generate an endless sequence of data, they are also more adaptable than other data structures.
Advantages of using generators:
The use of generators in Python has a number of benefits. They use less memory than lists, which is crucial when creating lengthy data sequences. As they can generate an endless sequence of data, they are also more adaptable than other data structures. Additionally, by passing data from one generator to another for additional processing, generators can be used to build data processing pipelines.
Building Generators with Generator Expressions
The quickest way to create a generator in Python is with a generator expression. It is comparable to a list comprehension, but it creates a generator object rather than a list. The syntax for a generator expression is similar to that of a list comprehension, but with parentheses instead of square brackets. For example, the following generator expression generates a sequence of even numbers:
even_nums = (num for num in range(10) if num % 2 == 0)
Code example of a generator expression:
Here is an example of a generator expression that generates the first 10 Fibonacci numbers:
fibonacci = (lambda n: ((1 + 5 ** 0.5) ** n - (1 - 5 ** 0.5) ** n) / (2 ** n * 5 ** 0.5))(x) for x in range(10)
This generator expression uses a lambda function to calculate the nth Fibonacci number and generates the first 10 numbers using a for loop. The generator can then be iterated over using a for loop or other iteration methods.
Using Python Generators
A. Explanation of how to use generators:
In Python, using a generator is as simple as calling the generator function and iterating over the results with a for loop or another iteration technique. Additionally, generators can be passed as return values or used as arguments in other functions. In order to iterate over the values more than once, you must create a new generator because generators can only be iterated over once.
B. Code examples of using generators to solve common problems:
Reading Large Files:
- Generators can be used to efficiently read large files in Python. Instead of reading the entire file into memory at once, the generator reads one line at a time and yields the line to the calling function. Here’s an example:
def read_large_file(file_path): with open(file_path) as f: for line in f: yield line.strip()
Generating an Infinite Sequence:
- Generators can also be used to generate an infinite sequence of values, which can be useful for a variety of applications. Here’s an example that generates an infinite sequence of random numbers between 0 and 1:
import random def generate_random_numbers(): while True: yield random.random()
Detecting Palindromes:
- Generators can be used to detect palindromes (strings that are the same when read forwards and backwards) in Python. Here’s an example:
def is_palindrome(word): return word == word[::-1] def find_palindromes(words): for word in words: if is_palindrome(word): yield word
Creating Data Pipelines:
- Generators can also be used to create data pipelines in Python, where one generator produces data that is then passed to another generator for further processing. Here’s an example:
def read_lines(file_path): with open(file_path) as f: for line in f: yield line.strip() def filter_lines(lines, pattern): for line in lines: if pattern in line: yield line def count_lines(lines): count = 0 for line in lines: count += 1 yield count
This example defines three generator functions that read lines from a file, filter lines that contain a specific pattern, and count the number of lines that pass through the filter. The generators can be combined into a pipeline by chaining them together using the “yield from” statement.
Understanding the Python Yield Statement
A. Explanation of the yield statement and its syntax:
Python generators use the “yield” statement to produce a series of values one at a time. A generator function returns the value and halts execution when it encounters the “yield” statement. Execution picks up where it left off the next time the generator is called.
B. Code examples of using yield to create generators:
Here’s an example of a generator that produces a sequence of square numbers:
def square_numbers(n): for i in range(n): yield i ** 2
This generator function uses the “yield” statement to produce the square of each number in the range of 0 to n.
Using Advanced Generator Methods
A. Explanation of advanced generator methods:
Python generators also provide several advanced methods that can be used to interact with the generator while it is running. These methods include:
- .send(): Sends a value back to the generator and resumes execution.
- .throw(): Raises an exception in the generator and resumes execution.
- .close(): Closes the generator and frees up any resources it was using.
B. Code examples of using .send(), .throw(), and .close() methods:
Using the .send() method:
- The .send() method is used to send a value back to the generator and resume execution. Here’s an example:
def countdown(start): while start > 0: val = yield start if val is not None: start = val else: start -= 1
This generator function counts down from a given starting value, and can also be reset to a new starting value using the .send() method. Here’s an example of how to use the .send() method to reset the countdown:
c = countdown(5) print(next(c)) # 5 print(next(c)) # 4 c.send(3) print(next(c)) # 3 print(next(c)) # 2
Using the .throw() method:
- The .throw() method is used to raise an exception in the generator and resume execution. Here’s an example:
def countdown(start): while start > 0: try: val = yield start except ValueError: start = 0 else: if val is not None: start = val else: start -= 1
This generator function also counts down from a given starting value, but raises a ValueError exception if the generator receives a negative value. Here’s an example of how to use the .throw() method to raise the exception:
c = countdown(5) print(next(c)) # 5 print(next(c)) # 4 c.throw(ValueError) print(next(c)) # StopIteration
Using the .close() method:
- The .close() method is used to close the generator and free up any resources it was using. Here’s an example:
def countdown(start): while start > 0: val = yield start if val is not None: start = val else: start -= 1 c = countdown(5) print(next(c)) # 5 print(next(c)) # 4 c.close() print(next(c)) # StopIteration
This generator function also counts down from a given starting value, but can be closed using the .close() method. Once the generator is closed, any further calls to next() will raise a StopIteration exception.
Profiling Generator Performance
A. Explanation of how to profile generator performance:
The process of measuring a generator’s efficiency and speed in terms of memory usage and execution time is known as profiling generator performance. The built-in cProfile module in Python, which offers thorough reports of the time spent in each function and how frequently it was called, is one of many tools and methods for profiling generator performance.
To use cProfile, you can run your generator code using the following command:
-m cProfile your_script.py
This will generate a report showing the total time spent in each function, including the generator functions, and the number of calls to each function.
B. Code examples of profiling generator performance:
Here’s an example of how to use cProfile to profile the performance of a simple generator function that generates numbers from 1 to 1000:
import cProfile def my_generator(): for i in range(1, 1001): yield i cProfile.run('list(my_generator())')
This code uses cProfile to run the generator function and convert the generated numbers into a list. The resulting report shows the time spent in each function, including the generator function, and the number of calls to each function.
Limitations of Generators
A. Inability to index or slice generator objects:
The inability to index or slice generators like regular lists or tuples is one of their main drawbacks. This is due to the fact that generators create values as they are needed and do not store all of their values in memory at once. As a result, accessing a particular value in a generator requires going through all of the previous values first.
B. Difficulty in debugging and testing generator functions:
A further drawback of generators is that they can be challenging to test and debug, particularly if they are complicated or contain numerous yield statements. This is due to the fact that generator functions run in small batches rather than all at once as the values are requested. This can make it challenging to pinpoint the exact location of a bug or error.
C. Code examples of generator function misuse:
Here’s an example of how generator functions can be misused, leading to memory errors:
def bad_generator(): result = [] for i in range(100000000): result.append(i) yield result
This generator function appends each number to a list and yields the entire list at each iteration. However, since the list is never cleared, it will continue to grow until it consumes all available memory.
Conclusion
A. Summary of the importance and limitations of generators:
Generators are an important tool in Python programming for generating sequences of values on the fly, without storing them all in memory at once. They can be used to process large data sets, generate infinite sequences, and create data pipelines. However, generators also have some limitations, including the inability to index or slice generator objects, and the difficulty in debugging and testing complex generator functions.
B. Recommendations for using generators effectively and avoiding common mistakes:
To use generators effectively, it’s important to understand their limitations and how they work. You should avoid misusing generator functions, such as by appending values to a list without clearing it, and use them only when they are appropriate for the task at hand. You can also use profiling tools to measure the performance of your generator functions and identify potential bottlenecks. By using generators effectively, you can write more efficient and scalable Python code.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are Python generators used for?
Python generators can be used to instantly generate a series of values rather than having to generate and store them all at once in memory. They can therefore be used to process massive data sets, produce infinite sequences, and build data pipelines.
Are generators good in Python?
Yes, generators are a powerful feature in Python and are widely used in many applications. They can help reduce memory usage and increase efficiency by generating data only when it is needed. However, they also have some limitations, such as the inability to index or slice generator objects.
What is the difference between an iterator and a generator?
An iterator is an object that implements the iterator protocol, which consists of two methods: __iter__() and __next__(). Iterators can be used to traverse a sequence of values one by one. A generator, on the other hand, is a type of iterator that is defined using a special syntax and generates values on the fly using the yield keyword. While all generators are iterators, not all iterators are generators.
Is Python generator lazy?
Yes, Python generators are often referred to as “lazy iterators” because they only generate values as they are needed. This means that they do not generate all values at once, but rather generate each value on demand as the generator is iterated over. This lazy behavior can help reduce memory usage and increase efficiency when working with large data sets or infinite sequences.
Other Useful Reads
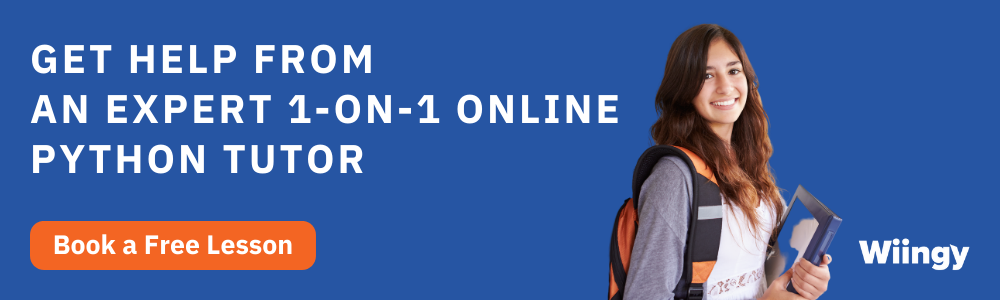
Written by by
Rahul LathReviewed by by
Arpit Rankwar