Python Tutorials
What is Operator Overloading in Python?
Operator overloading is a feature of Python that lets you use the same operator for more than one task.It lets programmers change the way an operator works by letting them define their own implementation for a certain operator for a certain class or object.The term “magic methods” refers to functions or techniques that are used to overload the operator.
Python overloading Because it enables developers to create user-defined objects that can act like built-in types, operator overloading is a crucial Python feature. Developers can specify how an object of a certain class will interact with other objects or with built-in operators by overloading the operators. Python is now more usable and flexible as a result.
We will talk about operator overloading in Python, why it’s important, and how to do it in this blog post. We will also show you some code examples to help you better understand how to use operator overloading in Python.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
How to Overload Operators in Python?
Magic Methods and Their Usage
In Python, operator overloading is implemented using special functions or methods called magic methods. These methods have double underscores (__) at the beginning and end of their names. For example, the addition operator (+) is overloaded using the add method, and the less than operator (<) is overloaded using the lt method.
Magic methods are called automatically by Python when a particular operator is used with a user-defined object. For example, if you add two objects of a class that has overloaded the addition operator, Python will call the add method to perform the addition operation.
Example Code Snippets
Here are some examples of how to overload operators in Python:
- Overloading the Addition Operator- To overload the addition operator, you can define the add method in your class. The following example shows how to overload the addition operator for a class that represents a point in two-dimensional space.
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
x = self.x + other.x
y = self.y + other.y
return Point(x, y)
In the above example, we have defined the add method that takes another point object as an argument and returns a new point object with the sum of the x and y coordinates.
- Overloading the Less Than Operator– To overload the less than operator, you can define the lt method in your class. The following example shows how to overload the less than operator for a class that represents a rectangle.
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
def __lt__(self, other):
return self.area() < other.area()
In the above example, we have defined the lt method that compares the area of two rectangle objects and returns True if the area of the first rectangle is less than the area of the second rectangle.
Overloading Binary + Operator in Python
A. Explanation of Binary + Operator Overloading-
The binary addition operator (+) is one of the most commonly overloaded operators in Python. It is used to add two operands and produce a new value. In Python, we can overload the binary + operator to define the addition operation for our own classes.
To overload the binary + operator, we need to define the add method in our class. This method takes two operands as input and returns the result of the addition operation. When we use the + operator with our class objects, Python automatically calls the add method to perform the addition operation.
B. Example Code Snippets
Here’s an example of how to overload the binary + operator in Python:
In the above example, we have defined a class Fraction that represents a fraction with a numerator and a denominator. We have overloaded the binary + operator using the add method. This method takes another fraction object as input, adds the two fractions, and returns a new fraction object.
We have also defined the str method to display the fraction object in a readable format.
Here’s how to use the Fraction class with the overloaded binary + operator:
f1 = Fraction(1, 2)
f2 = Fraction(3, 4)
f3 = f1 + f2
print(f3) # Output: 5/4
In the above code, we have created two fraction objects f1 and f2, and added them using the + operator. The output is the result of the addition operation, which is a new fraction object with the value 5/4.
How Does Operator Overloading Actually Work?
Operator overloading works by using the magic methods in Python. When we use an operator with a user-defined object, Python automatically calls the corresponding magic method to perform the operation.
For example, when we use the + operator with two objects of a class that has overloaded the + operator, Python calls the add method of that class to perform the addition operation.
Similarly, when we use the < operator with two objects of a class that has overloaded the < operator, Python calls the lt method of that class to perform the less than operation.
Here’s an example of how operator overloading works in Python:
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
new_x = self.x + other.x
new_y = self.y + other.y
return Vector(new_x, new_y)
def __lt__(self, other):
return (self.x ** 2 + self.y ** 2) < (other.x ** 2 + other.y ** 2)
v1 = Vector(1, 2)
v2 = Vector(3, 4)
# Addition operator overloading
v3 = v1 + v2
print(v3.x, v3.y) # Output: 4 6
# Less than operator overloading
print(v1 < v2
Overloading Comparison Operators in Python
Explanation of Comparison Operator Overloading
In addition to the binary + operator, we can also overload other operators in Python, such as the comparison operators (<, >, <=, >=, ==, and !=). Comparison operator overloading allows us to define how objects of our class should be compared to each other.
To overload a comparison operator, we need to define the corresponding magic method in our class. For example, to overload the less than (<) operator, we need to define the lt method in our class. When we use a comparison operator with our class objects, Python automatically calls the corresponding magic method to perform the comparison operation.
Example Code Snippets- Here’s an example of how to overload the less than (<) operator in Python:
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __lt__(self, other):
return (self.x ** 2 + self.y ** 2) < (other.x ** 2 + other.y ** 2)
v1 = Vector(1, 2)
v2 = Vector(3, 4)
print(v1 < v2) # Output: True
In the above example, we have defined a class Vector that represents a two-dimensional vector with x and y coordinates. We have overloaded the less than (<) operator using the lt method. This method takes another vector object as input, compares the magnitudes of the two vectors, and returns a boolean value based on the comparison result.
Here’s how to use the Vector class with the overloaded less than (<) operator:
v1 = Vector(1, 2)
v2 = Vector(3, 4)
v3 = Vector(2, 3)
print(v1 < v2) # Output: True
print(v2 < v3) # Output: False
print(v1 < v3) # Output: True
In the above code, we have created three vector objects v1, v2, and v3, and compared them using the less than (<) operator. The output is the result of the comparison operation, which is a boolean value based on the magnitudes of the vectors.
Advantages of Operator Overloading in Python
Explanation of Benefits of Operator
Overloading Operator overloading provides several benefits in Python. It allows us to define our own operators and customize their behavior for our own classes. This can make our code more concise, readable, and intuitive.
For example, if we define a class that represents a complex number, we can overload the binary + operator to define the addition operation for complex numbers. This allows us to write code like this:
c1 = Complex(1, 2)
c2 = Complex(3, 4)
c3 = c1 + c2
This code is much more concise and readable than the alternative, which would be something like:
c1 = Complex(1, 2)
c2 = Complex(3, 4)
c3 = Complex(c1.real + c2.real, c1.imag + c2.imag)
Example Code Snippets
Here’s an example of how operator overloading can make our code more concise:
class Complex:
def __init__(self, real, imag):
self.real = real
self.imag = imag
def __add__(self, other):
new_real = self.real + other.real
new_imag = self.imag + other.imag
return Complex(new_real, new_imag)
c1 = Complex(1, 2)
c2 = Complex(3, 4)
c3 = c1 + c2
print(c3.real, c3.imag) # Output: 4 6
In the above example, we have defined a class Complex that represents a complex number with a real and imaginary part. We have overloaded the binary + operator using the add method to define the addition operation for complex numbers. This method takes another complex number as input, adds the real and imaginary parts of the two numbers, and returns a new Complex object.
We have then created three Complex objects c1, c2, and c3, and added c1 and c2 using the overloaded binary + operator. The result is a new Complex object c3 with a real part of 4 and an imaginary part of 6.
Overall, operator overloading is a powerful feature of Python that allows us to define our own operators and customize their behavior for our own classes. By doing so, we can make our code more concise, readable, and intuitive.
Overloading Built-in Functions
Python comes with a set of built-in functions that work with various types of objects. These functions are called “built-in” because they are included in the Python language itself and do not require any additional libraries or modules to use.
One of the advantages of using operator overloading is that it allows us to extend the functionality of these built-in functions to work with our own classes. This can make our code more concise, readable, and easier to work with.
- Giving Length to Your Objects Using len()
The len() function is used to determine the length of an object, such as a string or a list. To make our own objects work with len(), we can define a method called len() in our class.
Here’s an example:
class MyClass:
def __init__(self, data):
self.data = data
def __len__(self):
return len(self.data)
obj = MyClass([1, 2, 3, 4, 5])
print(len(obj)) # Output: 5
In the above example, we have defined a class called MyClass that stores a list of data. We have then defined the len() method to return the length of the data list. We can now use the len() function with objects of this class to determine their length.
- Making Your Objects Work With abs()
The abs() function is used to determine the absolute value of a number. To make our own objects work with abs(), we can define a method called abs() in our class.
Here’s an example:
class Complex:
def __init__(self, real, imag):
self.real = real
self.imag = imag
def __abs__(self):
return (self.real ** 2 + self.imag ** 2) ** 0.5
c = Complex(3, 4)
print(abs(c)) # Output: 5.0
In the above example, we have defined a class called Complex that represents a complex number with a real and imaginary part. We have then defined the abs() method to return the magnitude of the complex number, which is calculated using the Pythagorean theorem. We can now use the abs() function with objects of this class to determine their magnitude.
- Printing Your Objects Prettily Using str()
The str() function is used to convert an object to a string. To make our own objects work with str(), we can define a method called str() in our class.
Here’s an example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f"{self.name} is {self.age} years old"
p = Person("John", 30)
print(str(p)) # Output: John is 30 years old
In the above example, we have defined a class called Person that stores a name and an age. We have then defined the str() method to return a string representation of the Person object. We can now use the str() function with objects of this class to convert them to a string.
- Representing Your Objects Using repr()
The repr() function is used to obtain a string representation of an object that can be used to recreate the object. To make our own objects work with repr(), we can define a method called repr() in our class.
Here’s an example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __repr__(self):
return f"
Examples of Operator Overloading in Python
A. Explanation of real-world use cases for operator overloading:
Operator overloading can be used in many real-world scenarios to make code more readable and intuitive. Let’s explore some examples of how operator overloading can be useful:
- Overloading “<” Operator:
In some cases, it may be useful to compare objects of a custom class using the “<” operator. For example, let’s say we have a class “Rectangle” that represents a rectangle with a certain height and width. We may want to compare two rectangles to see which one has a greater area. We can achieve this by overloading the “<” operator in the Rectangle class.
- Overloading “+” Operator:
We can also overload the “+” operator to perform custom operations on objects of a class. For example, let’s say we have a class “Fraction” that represents a fraction with a numerator and denominator. We may want to add two fractions together to get a new fraction. We can overload the “+” operator in the Fraction class to perform this operation.
- Overloading Comparison Operators:
In some cases, we may want to compare objects of a custom class using comparison operators such as “>”, “<=”, etc. For example, let’s say we have a class “Person” that represents a person with a certain age. We may want to compare two people to see who is older. We can overload the comparison operators in the Person class to perform this operation.
- Overloading Equality Operator:
We can also overload the equality operator “==” to perform custom comparisons on objects of a class. For example, let’s say we have a class “Point” that represents a point in 2D space with an x and y coordinate. We may want to compare two points to see if they are the same point. We can overload the equality operator in the Point class to perform this comparison.
B. Example code snippets:
- Overloading “<” Operator:
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
def __lt__(self, other):
return self.area() < other.area()
# Create two rectangles
r1 = Rectangle(3, 4)
r2 = Rectangle(4, 5)
# Compare the two rectangles
if r1 < r2:
print("r2 has a greater area than r1")
else:
print("r1 has a greater area than r2")
2. Overloading “+” Operator:
class Fraction:
def __init__(self, numerator, denominator):
self.numerator = numerator
self.denominator = denominator
def __add__(self, other):
new_numerator = (self.numerator * other.denominator) + (other.numerator * self.denominator)
new_denominator = self.denominator * other.denominator
return Fraction(new_numerator, new_denominator)
def __str__(self):
return f"{self.numerator}/{self.denominator}"
# Create two fractions
f1 = Fraction(1, 2)
f2 = Fraction(1, 4)
# Add the two fractions together
result = f1 + f2
# Print the result
print(result) # Output: 3/8
- Overloading Comparison Operators:
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def __lt__(self, other):
return self.area() < other.area()
def area(self):
return self.width * self.height
# create two rectangle objects
r1 = Rectangle(2, 5)
r2 = Rectangle(3, 4)
# compare the rectangles based on their area
if r1 < r2:
print("r1 is smaller than r2")
else:
print("r1 is bigger than r2")
In this example, we overload the less-than operator (<) to compare the rectangles based on their area. The __lt__ method is called when the < operator is used with rectangle objects. The method returns True if the area of the current rectangle is less than the area of the other rectangle.
- Overloading Equality Operator:
class Student:
def __init__(self, name, age):
self.name = name
self.age = age
def __eq__(self, other):
return self.name == other.name and self.age == other.age
# create two student objects
s1 = Student("Alice", 25)
s2 = Student("Bob", 30)
s3 = Student("Alice", 25)
# compare the students for equality
if s1 == s2:
print("s1 is equal to s2")
else:
print("s1 is not equal to s2")
if s1 == s3:
print("s1 is equal to s3")
else:
print("s1 is not equal to s3")
In this example, we overload the equality operator (==) to compare the students based on their name and age. The __eq__ method is called when the == operator is used with student objects. The method returns True if the name and age of the current student are equal to the name and age of the other student.
Magic Methods for Operator Overloading in Python
A. Explanation of magic methods and their usage for operator overloading: In Python, operators are implemented as special methods, called “magic methods” or “dunder methods” (short for “double underscore” methods), that have special names enclosed in double underscores. By defining these methods in our classes, we can customize how operators behave with our objects, which is known as operator overloading.
Here are some common magic methods for operator overloading:
- Binary Operators:
- __add__(self, other): Implement the addition operator +.
- __sub__(self, other): Implement the subtraction operator -.
- __mul__(self, other): Implement the multiplication operator *.
- __truediv__(self, other): Implement the true division operator /.
- __floordiv__(self, other): Implement the floor division operator //.
- __mod__(self, other): Implement the modulo operator %.
- __pow__(self, other[, modulo]): Implement the power operator **.
- Comparison Operators:
- __eq__(self, other): Implement the equality operator ==.
- __ne__(self, other): Implement the not equal operator !=.
- __lt__(self, other): Implement the less than operator <.
- __le__(self, other): Implement the less than or equal operator <=.
- __gt__(self, other): Implement the greater than operator >.
- __ge__(self, other): Implement the greater than or equal operator >=.
- Assignment Operators:
- __iadd__(self, other): Implement the in-place addition operator +=.
- __isub__(self, other): Implement the in-place subtraction operator -=.
- __imul__(self, other): Implement the in-place multiplication operator *=.
- __itruediv__(self, other): Implement the in-place true division operator /=.
- __ifloordiv__(self, other): Implement the in-place floor division operator //=.
- __imod__(self, other): Implement the in-place modulo operator %=.
- __ipow__(self, other[, modulo]): Implement the in-place power operator **=.
- Unary Operators:
- __neg__(self): Implement the negation operator -.
- __pos__(self): Implement the unary plus operator +.
- __abs__(self): Implement the absolute value operator abs().
- __invert__(self): Implement the bitwise inversion operator ~.
- Mathematical Operators:
- __round__(self[, n]): Implement the round() function.
- __floor__(self): Implement the math.floor() function.
- __ceil__(self): Implement the math.ceil() function.
- __trunc__(self): Implement the math.trunc() function.
B. Example code snippets:
- Binary Operators:
Binary operators include addition (+), subtraction (-), multiplication (*), division (/), modulo (%), etc. The following is an example of overloading the “+” operator for a custom class:
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
x = self.x + other.x
y = self.y + other.y
return Point(x, y)
p1 = Point(1, 2)
p2 = Point(3, 4)
p3 = p1 + p2
print(p3.x, p3.y) # Output: 4 6
- Comparison Operators:
Comparison operators include greater than (>), less than (<), equal to (==), etc. The following is an example of overloading the “>” operator for a custom class:
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def __gt__(self, other):
area1 = self.width * self.height
area2 = other.width * other.height
return area1 > area2
r1 = Rectangle(4, 5)
r2 = Rectangle(3, 6)
print(r1 > r2) # Output: True
- Assignment Operators:
Assignment operators include +=, -=, *=, etc. The following is an example of overloading the “+=” operator for a custom class:
class MyList:
def __init__(self, elements):
self.elements = elements
def __iadd__(self, other):
self.elements.extend(other.elements)
return self
l1 = MyList([1, 2, 3])
l2 = MyList([4, 5, 6])
l1 += l2
print(l1.elements) # Output: [1, 2, 3, 4, 5, 6]
- Unary Operators:
Unary operators include negation (-), inversion (~), etc. The following is an example of overloading the “-” operator for a custom class:
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __neg__(self):
return Vector(-self.x, -self.y)
v1 = Vector(3, 4)
v2 = -v1
print(v2.x, v2.y) # Output: -3 -4
- Mathematical Operators:
Mathematical operators include pow (), floor division (//), etc. The following is an example of overloading the “” operator for a custom class:
class MyNumber:
def __init__(self, value):
self.value = value
def __pow__(self, other):
return MyNumber(self.value ** other.value)
n1 = MyNumber(2)
n2 = MyNumber(3)
n3 = n1 ** n2
print(n3.value) # Output: 8
Conclusion
In this blog post, we have discussed the concept of operator overloading in Python. We have explained the importance of operator overloading and its usage in Python. We have also provided a brief overview of the topics covered in this article.
We have discussed how to overload operators in Python using magic methods and provided code snippets for various types of operators such as binary, comparison, assignment, unary, and mathematical operators. We have also explained the mechanism behind operator overloading and the advantages of using operator overloading in Python.
Furthermore, we have discussed the need for operator overloading and provided examples of real-world use cases for operator overloading. Finally, we have explained the magic methods used for operator overloading and provided code snippets for each of them.
Overall, operator overloading is a powerful tool in Python that allows us to customize the behavior of operators for our own classes. By overloading operators, we can make our code more readable, efficient, and easier to maintain.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is operator overloading in Python?
Operator overloading is a technique in Python that allows the use of built-in operators for user-defined objects. This means that the behavior of an operator can be changed depending on the type of operands used in the operation. By overloading an operator, it becomes possible to perform operations that were not originally supported by the operator. For example, by overloading the + operator, we can concatenate two strings or add two numbers stored in custom objects.
What is operator overloading with example?
One example of operator overloading can be seen with the == operator. This operator is used for comparing two values for equality. However, it can also be used to compare two objects of a user-defined class. Consider the following example:
class Employee:
def __init__(self, id, name):
self.id = id
self.name = name
def __eq__(self, other):
return self.id == other.id
e1 = Employee(1, "John")
e2 = Employee(1, "Jack")
if e1 == e2:
print("They are the same employee")
else:
print("They are different employees")
In the above example, we have defined a custom class “Employee” with two attributes “id” and “name”. We have also defined the eq method to overload the == operator for this class. This method takes two arguments, self and other, which represent the two objects being compared. The method compares the id attributes of the two objects and returns True if they are the same.
What is operator overloading with syntax?
In Python, operator overloading is achieved by defining special methods that start and end with two underscores. These methods are also known as “magic methods” or “dunder methods” (short for “double underscore”). Here is a list of some of the common dunder methods used for operator overloading in Python:
__add__(self, other) - Overloads the + operator for addition
__sub__(self, other) - Overloads the - operator for subtraction
__mul__(self, other) - Overloads the * operator for multiplication
__truediv__(self, other) - Overloads the / operator for division
__mod__(self, other) - Overloads the % operator for modulus
__lt__(self, other) - Overloads the < operator for less than comparison
__le__(self, other) - Overloads the <= operator for less than or equal to comparison
__eq__(self, other) - Overloads the == operator for equality comparison
__ne__(self, other) - Overloads the != operator for inequality comparison
__gt__(self, other) - Overloads the > operator for greater than comparison
__ge__(self, other) - Overloads the >= operator for greater than or equal to comparison
The syntax for defining an operator overload is:
class MyClass:
def __add__(self, other):
# code to define the behavior of the + operator
pass
In this example, we have defined the add method for a custom class “MyClass”. This method takes two arguments, self and other, which represent the two objects being added. The method should return a new object that represents the result of the addition. Note that the pass statement is just a placeholder and should be replaced with actual code. The same syntax can be used to define other dunder methods for operator overloading.
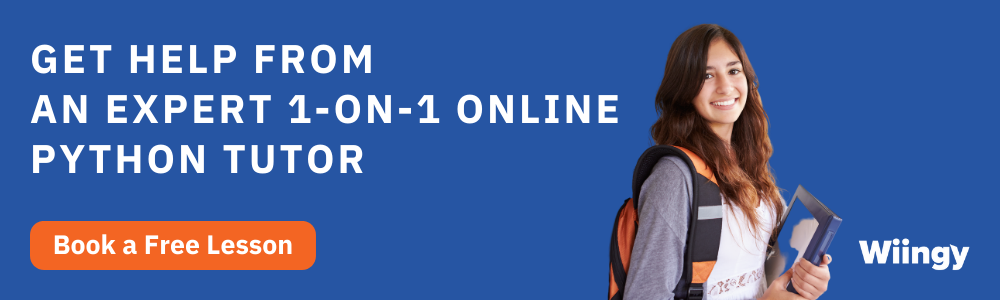
Written by by
Rahul LathReviewed by by
Arpit Rankwar