Python Tutorials
What are the looping techniques in Python?
Looping is a programming idea that lets a set of instructions be run over and over again until a certain condition is met. It is an important part of programming because it lets developers automate tasks that are done over and over again and process large amounts of data quickly.
Looping techniques in python are essential to programming because they make difficult tasks simpler and code more effective. Developers can perform the same task more than once by using loops, which saves time and effort. By repeatedly running a code block, loops also enable the processing of large datasets.
There are two main kinds of loops in Python: for loops and while loops. While loops are used when the number of iterations is unknown and for loops when the number of iterations is known.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
For Loops
A. Syntax
The syntax for a for loop in Python is as follows:
for variable in sequence: # Code to be executed
B. Examples
Looping with condition at top, middle, bottom
- Looping through a String
string = "Hello, World!" for char in string: print(char)
#Output
H e l l o , W o r l d !
- Looping through a List
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
#Output
apple banana cherry
- Looping through a Range of Numbers
for i in range(3): print(i)
#Output
0 1 2
- Looping through a Dictionary
person = {"name": "John", "age": 36, "country": "USA"} for key in person: print(key, ":", person[key])
#Output
name : John age : 36 country : USA
- Looping through Multiple Lists
fruits = ["apple", "banana", "cherry"] colors = ["red", "yellow", "green"] for fruit, color in zip(fruits, colors): print(fruit, "is", color)
#Output
apple is red banana is yellow cherry is green
- Nested Loops
for i in range(3): for j in range(3):
print(i, j)
#Output
0 0 0 1 0 2 1 0 1 1 1 2 2 0 2 1 2 2
C. The range() Function
Python has a built-in function called range() that produces a series of numbers. Start, Stop, and Step are the three parameters it requires. The start parameter specifies the starting number of the sequence, the stop parameter specifies the ending number of the sequence, and the step parameter specifies the step size between each number in the sequence.
for i in range(0, 10, 2): print(i)
#Output
0 2 4 6 8
D. The enumerate() Function
The enumerate() function is a built-in function in Python that adds a counter to an iterable object. It returns an enumerate object that contains pairs of elements and their corresponding index.
fruits = ["apple", "banana", "cherry"] for i, fruit in enumerate(fruits): print(i, fruit)
E. The zip() Function
A built-in Python function called zip() combines multiple iterables into a single iterable. It accepts two or more iterables as arguments and outputs an iterator of tuples, where each tuple’s i-th element is taken from one of the input iterables.
fruits = ["apple", "banana", "cherry"] colors = ["red", "yellow", "green"] for fruit, color in zip(fruits, colors): print(fruit, "is", color)
#Output
apple is red banana is yellow cherry is green
In the example above, the zip() function combines the fruits and colors lists into a single iterable that can be looped over using a for loop. The for loop unpacks each tuple containing the corresponding elements from both lists and prints the output. If the input iterables are of different lengths, zip() stops as soon as the shortest iterable is exhausted.
While Loops in Python
Another type of loop in Python is the while loop, which iterates over a block of code as long as a given condition is true. The syntax of a while loop is as follows:
while condition: # code to be executed
Every time the loop iterates, the condition, which is a boolean expression, is evaluated. The code inside the loop is executed if the condition is satisfied. Until the condition is satisfied or a break statement is reached, this process keeps running.
It’s important to remember that while loops have the potential to result in infinite loops, or loops that never end. This may occur if the while loop’s condition is always true. It’s important to ensure that there is a way for the condition to eventually become false.
Examples
Simple While Loop
Here is an example of a simple while loop that prints the numbers 0 through 4:
i = 0 while i < 5: print(i) i += 1
#Output
0 1 2 3 4
Looping Until a Condition is Met
This example demonstrates how to use a while loop to repeatedly ask the user to enter a number until a valid input is received:
while True: try: number = int(input("Enter a number: ")) break except ValueError: print("Invalid input. Try again.")
In this example, the while loop will continue to run until a valid integer input is received from the user. If the user enters an invalid input, such as a string or a floating-point number, the loop will continue to ask for input until a valid integer is entered.
Looping Through a List with a While Loop
Here is an example of how to loop through a list using a while loop:
fruits = ["apple", "banana", "cherry"] i = 0 while i < len(fruits): print(fruits[i]) i += 1
#Output
apple banana cherry
Nested While Loops
Nested while loops are similar to nested for loops in that they allow you to iterate over multiple lists or ranges. Here is an example of nested while loops:
i = 0 while i < 5: j = 0 while j < 3: print(i, j) j += 1 i += 1
#Output
0 0 0 1 0 2 1 0 1 1 1 2 2 0 2 1 2 2 3 0 3 1 3 2 4 0 4 1 4 2
Control Statements in Loops
You can run a block of code repeatedly by using loop control statements. There are two types of loops: for statements loop a predetermined number of times, and incrementing index variables keep track of each iteration.
A. Break Statement
When you want to end a loop early, you use the break statement. When a loop encounters a break statement, the loop is immediately broken, and control is moved to the statement that follows the loop.
i = 0 while i < 5: if i == 3: break print(i) i += 1
#Output
0 1 2
In this example, the loop is terminated prematurely when the value of i is equal to 3. Therefore, the output only includes the numbers 0, 1, and 2.
B. Continue Statement
The continue statement is used to skip over the rest of the code inside a loop for the current iteration and move on to the next iteration.
i = 0 while i < 5: i += 1 if i == 3: continue print(i)
#Output:
1 2 4 5
In this example, when the value of i is equal to 3, the continue statement skips over the print statement and moves on to the next iteration of the loop.
C. else Clause in Loops
In loops, the else clause is used to specify a block of code that should be run after all of the loop’s iterations have been completed. The else block is only executed if the loop completes all of its iterations without encountering a break statement.
- For Else Statement
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit) else: print("No more fruits.") Output:
apple banana cherry No more fruits.
In this example, the else block is executed after the for loop completes all of its iterations and prints “No more fruits.”
- While Else Statement
i = 0 while i < 5: print(i) i += 1 else: print("Loop completed.") Output:
0 1 2 3 4 Loop completed.
In this example, the else block is executed after the while loop completes all of its iterations and prints “Loop completed.”
List Comprehensions
List comprehensions in Python are a quick way to make lists. They let you make a new list by applying an expression to each item in an existing list or sequence. List comprehensions are often used as a more readable and efficient alternative to using a for loop.
A. Syntax
The syntax for a list comprehension is as follows:
new_list = [expression for item in sequence]
The expression is evaluated for each item in the sequence, and the results are collected into a new list.
Examples
- Basic List Comprehension
numbers = [1, 2, 3, 4, 5] squares = [x ** 2 for x in numbers] print(squares)
#Output
[1, 4, 9, 16, 25]
In this example, the list comprehension creates a new list called “squares” by squaring each element in the “numbers” list.
- List Comprehension with if Statement
numbers = [1, 2, 3, 4, 5] even_numbers = [x for x in numbers if x % 2 == 0] print(even_numbers) </span>
#Output
[2, 4]
In this example, the list comprehension creates a new list called “even_numbers” that only includes the even numbers from the “numbers” list.
- List Comprehension with if-else Statement
numbers = [1, 2, 3, 4, 5] even_or_odd = ["even" if x % 2 == 0 else "odd" for x in numbers] print(even_or_odd)
#Output
['odd', 'even', 'odd', 'even', 'odd']
In this example, the list comprehension creates a new list called “even_or_odd” that checks if each element in the “numbers” list is even or odd and adds the corresponding string to the new list.
- Nested List Comprehension
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] flattened_matrix = [x for row in matrix for x in row] print(flattened_matrix)
#Output
[1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, the list comprehension creates a new list called “flattened_matrix” by flattening the nested list “matrix” into a single list.
Generator Expressions
Generator expressions are like list comprehensions, but instead of making a list, they make an object called a generator. This means that the values are made as needed, on the fly, instead of making the whole list at once. Generator expressions can be used to save memory and improve performance when dealing with large datasets.
A. Syntax
The syntax for a generator expression is similar to that of a list comprehension, but with parentheses instead of square brackets:
generator_object = (expression for item in sequence)
B. Examples
- Basic Generator Expression:
Here is a basic example of a generator expression that generates a sequence of numbers from 0 to 9:
gen_expr = (x for x in range(10)) for i in gen_expr: print(i)
#Output
0 1 2 3 4 5 6 7 8 9
- Generator Expression with if Statement:
We can use the if statement to filter out values from the generator expression. In the following example, we generate a sequence of numbers from 0 to 9, but only output the even numbers:
gen_expr = (x for x in range(10) if x % 2 == 0) for i in gen_expr: print(i)
#Output
0 2 4 6 8
- Nested Generator Expression:
We can also nest generator expressions. In the following example, we generate a sequence of numbers from 0 to 9, but only output the even numbers that are also divisible by 3:
gen_expr = (x for x in (y for y in range(10)) if x % 2 == 0 and x % 3 == 0) for i in gen_expr: print(i) Output:
0 6
In this example, we first generate a sequence of numbers from 0 to 9 using the inner generator expression, and then filter out the even numbers that are also divisible by 3 using the outer generator expression.
Generator expressions are a powerful feature of Python, and can be used to generate sequences of values on-the-fly without the need for creating intermediate lists or arrays.
Conclusion:
Programming’s fundamental concept of looping enables you to repeatedly carry out a set of instructions. For loops, while loops, list comprehensions, and generator expressions are just a few of the looping constructs that Python offers. For loops are used to repeatedly iterate through a list of values or a range of numbers. While loops are used to repeatedly execute a code block until a certain condition is met. List comprehensions and generator expressions are used to generate sequences of values on-the-fly, and are especially useful for working with large datasets.
B. Tips for Using Looping Techniques in Python:
Here are a few tips for using looping techniques in Python:
- Be mindful of performance: when working with large datasets, it’s important to use looping techniques that are optimized for speed and memory usage. For example, list comprehensions and generator expressions can be much faster than for loops for generating sequences of values.
- Use control statements wisely: control statements such as break, continue, and else can make your code more efficient and easier to read, but be sure to use them wisely and sparingly.
- Practice, practice, practice: the best way to master looping techniques in Python is to practice writing code. Try out different examples and experiment with different approaches to see what works best for your needs.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs:
What are the 3 types of loops in Python?
The 3 types of loops in Python are for loops, while loops, and do-while loops (although do-while loops are not commonly used in Python).
What are the looping techniques in Python?
Looping techniques in Python refer to the various ways that you can perform a set of instructions repeatedly in Python, including for loops, while loops, list comprehensions, and generator expressions.
What are the 2 main types of loops in Python?
The 2 main types of loops in Python are for loops and while loops.
What are the 3 types of loop control statements with Python code as an example?
The 3 types of loop control statements in Python are break, continue, and else. Here are some examples of each:
# break statement example for i in range(10): if i == 5: break print(i) # continue statement example for i in range(10): if i % 2 == 0: continue print(i) # else statement example for i in range(10): if i == 5: break print(i) else: print(“Loop finished without reaching break statement”)
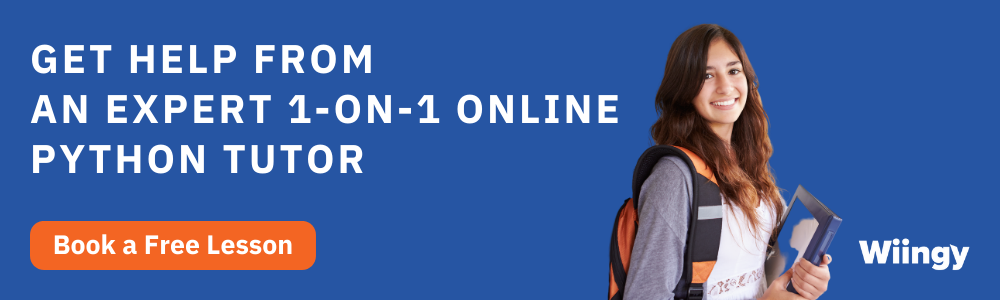
Written by by
Rahul LathReviewed by by
Arpit Rankwar