What is a Static Variable in Python?
Static variables in Python are global variables used by every instance of a class. In contrast to instance variables, static variables are part of the class itself and are not associated with any particular. This indicates that the static variable’s value is shared by every instance of a class.
When a class is defined, the static keyword is used to define variables, which are initialized just once. They remain valuable for the duration of the program, and any modifications have an impact on every instance of the class.
An in-depth explanation of static variables in Python, including their definition, usage, and examples, is the aim of this article.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Instance Variables vs. Static Variables in Python
Variables that are defined inside a class and are unique to each object or instance of the class are known as instance variables. They are created when an object is instantiated and each instance of the class may have a different value for them.
The’static’ keyword is used to define static variables, which are shared by all instances of the class. They are made when a class is defined, and they continue to be valuable for the duration of the program.
To summarize, the key differences between instance variables and static variables are:
Static variables are shared by all instances of the class, whereas instance variables are unique to each object or instance of the class.
Static variables are created when a class is defined, whereas instance variables are created when an object is instantiated.
In Python, instance variables include the following examples:
1class Car:
2 def __init__(self, make, model):
3 self.make = make
4 self.model = model
5
6car1 = Car("Toyota", "Camry")
7car2 = Car("Honda", "Accord")
8
9print(car1.make) # Output: Toyota
10print(car2.make) # Output: Honda
In this example, ‘make’ and ‘model’ are instance variables of the Car class, and they have different values for each instance of the class.
Examples of static variables in Python include:
1class Student:
2 count = 0 # static variable
3
4 def __init__(self, name):
5 self.name = name
6 Student.count += 1 # increment count on each instance creation
7
8student1 = Student("John")
9student2 = Student("Mary")
10
11print(Student.count) # Output: 2
In this example, ‘count’ is a static variable of the Student class, and it is shared by all instances of the class. The value of ‘count’ is incremented each time a new instance of the class is created.
How to Define Static Variables in Python
In Python, you must use the’static’ keyword before the variable name to define a static variable. Here’s the syntax:
1class MyClass:
2 static_var = value
Where ‘MyClass’ is the name of the class, ‘static_var’ is the name of the static variable, and ‘value’ is the initial value of the static variable.
Anywhere in the class definition, including inside methods, class methods, and static methods, is a place where static variables can be defined.
Here are some examples of defining static variables in Python:
1class Circle:
2 pi = 3.14 # static variable
3
4 def __init__(self, radius):
5 self.radius = radius
6
7 def area(self):
8 return Circle.pi * self.radius ** 2
9
10circle1 = Circle(5)
11print(circle1.area()) # Output: 78.5
In this example, ‘pi’ is a static variable of the Circle class, and it is used inside the ‘area’ method to calculate the area of a circle.
Here’s another example of defining static variables inside a class method:
1class Employee:
2 num_employees = 0 # static variable
3
4 def __init__(self, name, salary):
5 self.name = name
6 self.salary = salary
7 Employee.num_employees += 1
8
9 @classmethod
10 def get_num_employees(cls):
11 return cls.num_employees
12
13employee1 = Employee("John Doe", 50000)
14employee2 = Employee("Jane Smith", 60000)
15
16print(Employee.get_num_employees()) # Output: 2
In this example, ‘num_employees’ is a static variable of the Employee class, and it is incremented each time a new instance of the class is created inside the ‘init’ method. The ‘get_num_employees’ class method is used to access the value of the static variable.
In conclusion, static variables are an important concept in Python programming, and understanding their usage and definition can be useful in writing efficient and effective code.
Accessing Static Variables in Python
In Python, you can use the class name or the instance name, the dot (.) operator, and the name of the static variable to access a static variable. Here’s the syntax:
1ClassName.static_var
2instance_name.static_var
When accessing a static variable, you don’t need to create an instance of the class. The value of the static variable is the same for all instances of the class.
The key differences between accessing static variables and instance variables are:
Accessing static variables uses the class name or instance name followed by the dot (.) operator, while accessing instance variables uses only the instance name followed by the dot (.) operator.
Static variables can be accessed without creating an instance of the class, while instance variables can only be accessed through an instance of the class.
Here are some examples of accessing static variables in Python:
1class BankAccount:
2 interest_rate = 0.05 # static variable
3
4account1 = BankAccount()
5account2 = BankAccount()
6
7print(BankAccount.interest_rate) # Output: 0.05
8print(account1.interest_rate) # Output: 0.05
9print(account2.interest_rate) # Output: 0.05
In this example, ‘interest_rate’ is a static variable of the BankAccount class, and it is accessed using both the class name and instance names.
When to Use Static Variables in Python
Static variables can be useful in various programming scenarios, such as:
- When you need to maintain a value that is common to all instances of a class.
- When you want to avoid redundant computation or data storage.
- When you need to keep track of certain statistics or counters.
The benefits of using static variables include:
Effective memory usage: Static variables can save memory because they are shared by all instances of the class and only need to be stored once.
Simpler code maintenance: Static variables make it simple to keep track of frequent values or statistics, which can make it easier to maintain and debug code.
Here are some examples of when to use static variables:
1class Logger:
2 log_file = "app.log" # static variable
3
4 @staticmethod
5 def log(message):
6 with open(Logger.log_file, "a") as f:
7 f.write(message + "\n")
8
9Logger.log("Application started.")
In this example, ‘log_file’ is a static variable of the Logger class, and it is used inside the ‘log’ static method to write messages to a log file. By using a static variable, the log file name is common to all instances of the class, and it can be easily changed if needed.
1class Counter:
2 count = 0 # static variable
3
4 def __init__(self):
5 Counter.count += 1
6
7 @staticmethod
8 def get_count():
9 return Counter.count
10
11counter1 = Counter()
12counter2 = Counter()
13
14print(Counter.get_count()) # Output: 2
In this example, ‘count’ is a static variable of the Counter class, and it is used inside the ‘init’ method to count the number of instances of the class. By using a static variable, the counter is common to all instances of the class, and it can be easily accessed using the ‘get_count’ static method.
Conclusion
Static variables are a crucial part of Python programming that facilitate effective memory management and simpler code maintenance. Understanding static variables will help you write better code and approach problems in programming more successfully. If you want to avoid duplicating computation or data storage, maintain a value that is common to all instances of a class, or keep track of specific statistics or counters, keep in mind to use static variables.
You can define a static variable by using the ‘static’ keyword before the variable name, and you can access a static variable by using the class name or instance name followed by the dot (.) operator.
You can make difficult programming tasks simpler and increase program performance by using static variables in your code. It is crucial to remember that static variables should only be used sparingly and when absolutely necessary. After reading this article, you ought to have a firm grasp on static variables in Python and know how to use them efficiently in your programs.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
Can you make static variables in Python?
In Python, you can create static variables by adding the keyword “static” before the variable name.
What is static variable with example?
A class-level Python variable that is shared by every instance of the class is known as a static variable. Here’s an illustration:
1class Car: num_wheels = 4 # static variablecar1 = Car()car2 = Car()print(car1.num_wheels) # Output: 4print(car2.num_wheels) # Output: 4In this example, 'num_wheels' is a static variable of the Car class, and it is shared by all instances of the class.
What purpose does static serve in Python?
In Python, class-level variables and methods that are shared by all instances of the class are defined using the “static” keyword.
What is a static variable?
In Python, a static variable is one that is shared by every instance of the class.
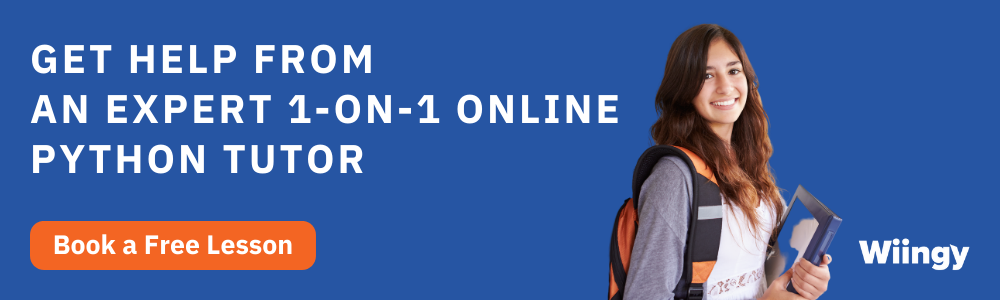
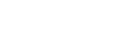
Jan 30, 2025
Was this helpful?