What are While Loops in Python?
Python while loops are fundamental programming constructs that let you run a block of code repeatedly until a certain condition is satisfied. While a specific condition is still true, a while loop is used to iterate through a block of code. Because of this, while loops are perfect for tasks that must be repeated until a particular condition is met.
While loops are different from for loops because while for loops are used to go through a list of values, while while loops run a block of code until a certain condition is met. While the number of iterations is unknown before a while loop begins, it is known before a for loop begins how many times the loop will run.
While loops can have advantages and disadvantages. One advantage is that while loops are flexible and can execute indefinitely until a certain condition is met. A disadvantage is that while loops can lead to infinite loops, which can cause your program to become unresponsive and crash.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Basic While Loop Syntax
The basic syntax for a while loop in Python is as follows:
1while condition:
2# code to be executed
3# while condition is true
4# (body of the loop)
5# ...
6# update condition (if necessary)
A while loop has four parts: setting up the loop, condition for loop execution, body of the loop, and updating the loop condition.
A. Setting up the loop:
The word “while” is used to create the loop, which is then followed by a condition that must be true for the loop to work. A comparison or logical expression that evaluates to True or False typically serves as this condition.
B. Condition for loop execution:
The expression that is tested at the start of each loop iteration is the requirement for the loop to execute. The loop will run if the condition is True. The loop will end and the program will move on to the next line of code if the condition is False.
C. Body of the loop:
The section of code that runs repeatedly while the condition is True is known as the body of the loop. A single statement or a collection of statements encased in curly braces can make up this block of code.
D. Updating the loop condition:
The updating of the condition that is checked at the start of each loop iteration is the final step of a while loop. A function that returns a value or changing the value of a variable can both be used to implement this update.
Overall, while loops are a powerful and flexible construct in Python that can be used to execute a block of code repeatedly until a specific condition is met. Understanding the basic syntax and differences between for and while loops can help students develop their programming skills and solve problems more efficiently.
Examples of Using While Loops
While loops are an effective tool that can be applied to numerous programming issues. Here are some examples of using while loops in Python:
A. Counting with a while loop:
You can use a while loop to count from one number to another by updating a counter variable until it reaches a specified value. For example, the following code will count from 1 to 10:
1count = 1 while count <= 10: print(count) count += 1
B. Repeating user input until valid:
You can use a while loop to prompt the user to enter input repeatedly until it meets certain criteria. For example, the following code will prompt the user to enter a number between 1 and 10 and will keep asking until a valid number is entered:
1num = 0 while num < 1 or num > 10: num = int(input("Enter a number between 1 and 10: ")) print("You entered:", num)
C. Iterating over a list with a while loop:
You can use a while loop to iterate over a list of values by using an index variable that increments with each iteration. For example, the following code will iterate over a list of names and print each name:
1names = ["Alice", "Bob", "Charlie", "David"] index = 0 while index < len(names): print(names[index]) index += 1
D. Computing the factorial of a number:
You can use a while loop to compute the factorial of a number by multiplying a variable by successively smaller numbers until it reaches 1. For example, the following code will compute the factorial of 5:
1num = 5 fact = 1 while num > 0: fact *= num num -= 1 print("Factorial of 5 is:", fact)
Infinite Loops and How to Avoid Them
While loops are effective, when used improperly they can also lead to issues. The occurrence of infinite loops is a common problem that can make your program unresponsive and crash.
A. Definition of an infinite loop:
A loop that keeps running forever because the condition that controls it never becomes false is called an “infinite loop.” This can occur if the loop condition is not set up correctly or if the loop’s code does not modify the condition.
B. Examples of infinite loops:
One example of an infinite loop is the following code, which will continue to print “Hello” forever because the condition “True” never becomes false:
1while True: print("Hello")
Another example is the following code, which will continue to prompt the user for input even when they enter a valid number:
1num = 0 while num < 1 or num > 10: num = int(input("Enter a number between 1 and 10: "))
C. Techniques for avoiding infinite loops:
To avoid infinite loops, it is important to ensure that the condition that controls the loop eventually becomes false. Here are some techniques for avoiding infinite loops:
- Make sure the loop condition is properly set up and will eventually become false.
- Include a break statement inside the loop that will break out of the loop when a certain condition is met.
- Use a for loop instead of a while loop when the number of iterations is known beforehand.
- Ensure that the code inside the loop changes the loop condition in some way, such as updating a counter variable or using a function that returns a value.
For example, the following code will break out of the loop when the user enters a valid number:
1num = 0 while True: num = int(input("Enter a number between 1 and 10: ")) if num >= 1 and num <= 10: break print("You entered:", num)
The user will be prompted until they enter a number between 1 and 10 in this code. The loop will end when a valid number is entered, and the program will move on to the following line of code.
Students can improve their programming skills and create more robust and effective programs by learning how to use while loops correctly and how to avoid infinite loops.
Nested While Loops
Loops that are contained within another loop are called nested while loops. They are an effective programming technique that can be used to resolve challenging issues that call for numerous iterations. In a while loop that is nested, the inner loop will run entirely each time the outer loop runs.
A. Definition of nested loops:
Loops that are contained within another loop are nested loops. This can be done in Python by nesting one while loop inside another.
B. Examples of nested while loops:
Here is an example of a nested while loop that prints out a multiplication table:
1i = 1 while i <= 10: j = 1 while j <= 10: print(i * j, end='\t') j += 1 print() i += 1
C. Advantages and disadvantages of nested loops:
The advantages of nested loops include their ability to solve complex problems that require multiple iterations and their flexibility. However, nested loops can also be slower and harder to read than simpler loops.
The break and continue Statements
It is possible to change the way a loop behaves by using the break and continue statements. The continue statement skips to the next iteration of the loop, whereas the break statement exits a loop early.
A. Definition and purpose of break and continue statements:
While the continue statement skips one iteration of the loop and moves on to the next one, the break statement is used to exit a loop early.
B. Examples of using break and continue in a while loop:
Here is an example of using the break statement to exit a loop when a certain condition is met:
1i = 0 while i < 10: i += 1 if i == 5: break print(i)
This code will print the numbers 1 through 4 and then exit the loop when i becomes equal to 5.
Here is an example of using the continue statement to skip over an iteration of the loop:
1i = 0 while i < 10: i += 1 if i % 2 == 0: continue print(i
This code will print only the odd numbers between 1 and 10 by skipping over the even numbers with the continue statement.
The else Clause in While Loops
A while loop’s else clause is a section of code that is run after the loop has finished running, but only if a break statement did not cause the loop to end prematurely.
A. Definition and purpose of the else clause in while loops:
After the while loop has completed running, a block of code is executed using the else clause. Only if the loop was not terminated prematurely by a break statement will this block of code be carried out.
B. Examples of using the else clause in while loops:
Here is an example of using the else clause in a while loop to print a message if a number is not found in a list:
1numbers = [1, 2, 3, 4, 5] i = 0 while i < len(numbers): if numbers[i] == 6: print("Number found!") break i += 1 else: print("Number not found.")
This code will search for the number 6 in the list of numbers. If the number is found, the message “Number found!” will be printed, and the loop will exit. If the number is not found, the message “Number not found.” will be printed after the loop has finished executing.
One-Line while Loops
One-line while loops, also known as while loop comprehensions, are a concise way of writing while loops that can fit on a single line. They are useful when you need to perform a simple operation on each iteration of the loop.
A. Explanation of one-line while loops:
A one-line while loop is a concise way of writing a while loop that can fit on a single line. They are useful when you need to perform a simple operation on each iteration of the loop.
B. Example of a one-line while loop:
Here is an example of a one-line while loop that computes the sum of the first 10 natural numbers:
1sum = 0 i = 1 while i <= 10: sum += i; i += 1 print("Sum of first 10 natural numbers:", sum)
This code computes the sum of the first 10 natural numbers using a one-line while loop that increments the variable i and adds it to the variable sum.
Conclusion
Python’s while loops are an effective programming construct that let you run a block of code repeatedly until a certain condition is satisfied. While loops are different from for loops in that the former are used to execute a block of code until a condition is satisfied, whereas the latter are used to iterate over a sequence of values. Nested while loops can be used to solve complex problems that require multiple iterations, and the break and continue statements can be used to modify the behavior of a loop.
While loops are adaptable and can run indefinitely until a specific condition is satisfied, they are best used for tasks that need to be repeated until a particular condition is met. While loops, on the other hand, can result in infinite loops, which can make your program unresponsive and crash. While loops can also be slower and more difficult to read than simpler loops.
While loops are a powerful programming construct that can be used to solve a variety of programming problems. When used properly, while loops can help students develop their programming skills and solve problems more efficiently. To create robust and effective programs, it’s crucial to be aware of their limitations and combine them with other programming techniques.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are while loops in Python?
While loops in Python are a type of loop that allows the repeated execution of a block of code as long as a specified condition is true.
How do you do a while loop in Python?
A: In Python, you must first specify the condition to be tested before you can create a while loop. Then you specify the code that will run inside the loop. Finally, you ensure that the condition will be changed in some way on each iteration so that the loop will eventually exit.
What is an example of a while loop?
Here is an example of a while loop that counts from 1 to 10:
count = 1 while count <= 10: print(count) count += 1
Q: What is the syntax of a while loop?
The syntax of a while loop in Python is as follows:
while condition: # code to be executed # while condition is true # … # update condition (if necessary)
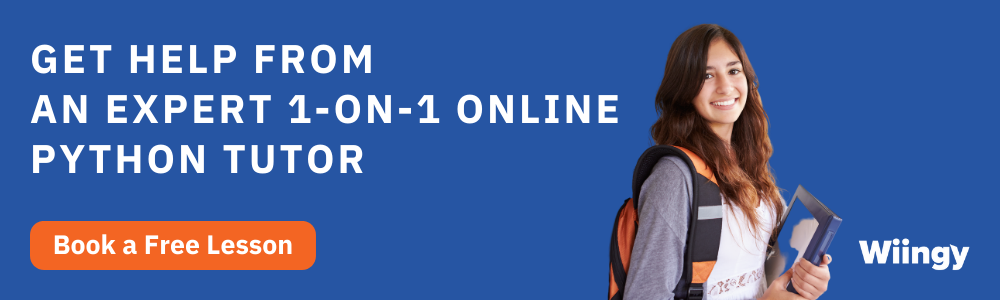
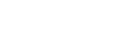
Jan 30, 2025
Was this helpful?