What are Errors and Exceptions in Python?
Writing instructions in a language that computers can understand is a complicated process called programming. Even the best programmers, though, occasionally make errors. These errors and exceptions can result in a program crashing or producing incorrect output. We will talk about errors and exceptions in Python, their types, and how to handle them in this article.
Programming errors are mistakes that prevent a program from running because they are made in the code. Exceptions, on the other hand, are unanticipated events that happen while a program is being executed and stop the program’s regular flow.
For any programmer, understanding errors and exceptions is crucial. Programmers can write better code, find and correct errors, and gracefully handle exceptions by becoming more familiar with them. This may result in more dependable, effective programs that deliver precise results.
A thorough explanation of Python errors and exceptions is given in this article. We will first go over the different kinds of errors that can happen in Python, such as runtime, logical, and syntax errors. The handling of exceptions, their types, and try-except blocks, raise statements, and finally blocks will then be covered in detail.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Types of Errors in Python
Syntax errors, logical errors, and runtime errors are the three main categories of errors in Python.
A. Syntax Errors
When a programmer disregards the syntax of the Python language, syntax errors happen. The Python interpreter picks up on these errors during compilation, and they prevent the program from running. Missing parentheses, incorrect indentation, and misspelled keywords are examples of common syntax errors.
Example:
1print("Hello World'
The above code will result in a syntax error because the closing quote is missing.
B. Logical Errors
When a program runs flawlessly but generates incorrect results because of a logic error, this is referred to as a logical error. These errors can be challenging to find because they won’t affect how the program functions.
Example:
1radius = 5
2area = 3.14 * radius * radius
3print("The area of the circle is", area)
The above code will run without any errors, but the output will be incorrect because the formula used to calculate the area of a circle is incorrect.
C. Runtime Errors
Runtime errors happen when a program encounters unforeseen issues while being executed. These mistakes, which are also referred to as exceptions, can be brought on by a number of things, including incorrect user input, a lack of memory, or division by zero.
Example:
1num1 = 10
2num2 = 0
3result = num1 / num2
4print(result)
The above code will result in a runtime error because dividing by zero is not allowed.
Exceptions in Python
Exceptions are unanticipated occurrences that happen while a program is running and stop the program’s regular flow. Try-except blocks, the raise command, and finally blocks are used in Python to handle exceptions.
A. Definition of Exceptions
An exception is a circumstance that prevents a program from running normally. The program halts when an exception occurs and switches to the closest exception handler.
B. Types of Exceptions
Built-in exceptions and user-defined exceptions are the two main categories of exceptions in Python.
Built-in Exceptions
Python has many built-in exceptions that are raised when specific errors occur during program execution. Some common built-in exceptions include TypeError, ValueError, and ZeroDivisionError.
Example:
1num1 = "10"
2num2 = 5
3result = num1 + num2
4print(result)
The above code will result in a TypeError because you cannot add a string and an integer.
User-Defined Exceptions
To handle particular errors that are not covered by the built-in exceptions, programmers can also create their own exceptions. By defining a new class that derives from the Exception class, user-defined exceptions can be created.
Example:
1class NegativeNumberError(Exception):
2 pass
3
4def calculate_square_root(num):
5 if num < 0:
6 raise NegativeNumberError("Cannot calculate square root of a negative number")
7 return math.sqrt(num)
8
9calculate_square_root(-5)
The above code will raise a user-defined exception called NegativeNumberError if the input number is negative.
C. How Exceptions Work
In Python, exceptions are managed using the try-except block. The code that might cause an exception is contained in the try block, and the code that handles the exception is contained in the except block.
The try-except block
1try:
2 num1 = int(input("Enter a number: "))
3 num2 = int(input("Enter another number: "))
4 result = num1 / num2
5 print(result)
6except ZeroDivisionError:
7 print("Cannot divide by zero")
8except ValueError:
9 print("Invalid input")
The above code uses a try-except block to handle two exceptions: ZeroDivisionError and ValueError. If the user enters invalid input or tries to divide by zero, the corresponding except block will be executed.
The raise statement
The raise statement is used to raise an exception manually. This can be useful when you want to handle specific errors or create your own exceptions.
Example:
1def calculate_square_root(num):
2 if num < 0:
3 raise ValueError("Cannot calculate square root of a negative number")
4 return math.sqrt(num)
5
6calculate_square_root(-5)
The above code uses the raise statement to raise a ValueError if the input number is negative.
The finally block
The finally block is used to execute code that must always run, regardless of whether an exception was raised or not.
Example:
1try:
2 file = open("data.txt", "r")
3 # some code here
4except FileNotFoundError:
5 print("File not found")
6finally:
7 file.close()
The above code uses the finally block to ensure that the file is closed, even if an exception was raised.
By learning how to handle them, you can create more robust and reliable programs that produce accurate results. Remember to always use try-except blocks to handle exceptions, and to raise your own exceptions when necessary.
Handling Exceptions
Try-except blocks are used in Python to handle exceptions. The code that might cause an exception is contained in the try block, and the code that handles the exception is contained in the except block.
A. Catching and Handling Exceptions
Example of Catching a Specific Exception
1try:
2 num1 = int(input("Enter a number: "))
3 num2 = int(input("Enter another number: "))
4 result = num1 / num2
5 print(result)
6except ZeroDivisionError:
7 print("Cannot divide by zero")
The above code uses a try-except block to catch a specific exception, ZeroDivisionError. If the user tries to divide by zero, the except block will be executed.
Example of Catching Multiple Exceptions
1try:
2 num = int(input("Enter a number: "))
3 fruits = ["apple", "banana", "cherry"]
4 fruit = fruits[num]
5 print(fruit)
6except (IndexError, ValueError):
7 print("Invalid input")
The above code uses a try-except block to catch multiple exceptions, IndexError and ValueError. If the user enters invalid input or an index that is out of range, the except block will be executed.
B. Raising Exceptions
The raise statement in Python can be used to manually raise an exception. When you want to handle particular errors or make your own exceptions, this can be helpful.
Example of Raising a Specific Exception
1def calculate_square_root(num):
2 if num < 0:
3 raise ValueError("Cannot calculate square root of a negative number")
4 return math.sqrt(num)
5
6calculate_square_root(-5)
The above code uses the raise statement to raise a ValueError if the input number is negative.
Example of Raising a User-Defined Exception
1class NegativeNumberError(Exception):
2 pass
3
4def calculate_square_root(num):
5 if num < 0:
6 raise NegativeNumberError("Cannot calculate square root of a negative number")
7 return math.sqrt(num)
8
9calculate_square_root(-5)
The above code raises a user-defined exception called NegativeNumberError if the input number is negative.
Common Built-in Exceptions
When particular errors take place during the execution of a program, Python raises a number of built-in exceptions. Some of the most common built-in exceptions are:
- SyntaxError: Raised when there is a syntax error in the code.
- TypeError: Raised when an operation or function is applied to an object of inappropriate type.
- NameError: Raised when a variable or function name is not defined.
- ValueError: Raised when a function or operation receives an argument of the correct type but with an inappropriate value.
- IndexError: Raised when an index is out of range.
- KeyError: Raised when a key is not found in a dictionary.
- ZeroDivisionError: Raised when trying to divide a number by zero.
- FileNotFoundError: Raised when trying to open a file that does not exist.
Best Practices for Errors and Exceptions in Python
A. Proper Handling of Errors and Exceptions
It’s crucial to provide users with informative error messages when handling errors and exceptions so they can comprehend the issue. It’s crucial to gracefully handle exceptions and prevent program crashes.
B. Best Practices for Maintaining Code Readability
Use evocative variable and function names, add comments to clarify complicated code, and adhere to a consistent coding style to maintain code readability.
C. Pitfalls to Avoid
Catching all exceptions with a broad except block is a common pitfall to avoid. It may be challenging to locate and correct particular errors as a result. Additionally, since raising exceptions without a need can lead to user confusion, it’s important to avoid doing so.
Conclusion
In this article, we covered Python exceptions and errors. First, we defined errors and exceptions and discussed why it is crucial to comprehend them. Next, we discussed the three categories of Python errors: runtime errors, logical errors, and syntax errors. The handling of exceptions with try-except blocks, the raise statement, and finally blocks was covered in detail after that. Additionally, Python’s common built-in exceptions and error handling best practices were covered.
For Python code to be robust and reliable, errors and exceptions must be handled correctly. It enables programs to continue operating even in the face of unforeseen circumstances and aids programmers in quickly finding and correcting errors. Programmers can make their programs easier to use and maintain by supplying clear error messages and gracefully handling exceptions.
Programmers will still need to have a solid grasp of errors and exceptions as programming languages develop and become more complex. It’s critical to stay current with error handling best practices and to keep picking up new tricks for handling errors and exceptions in Python.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are errors and exceptions in Python?
In Python, errors and exceptions refer to mistakes or unanticipated occurrences that happen while a program is running and cause it to crash or produce incorrect results.
What are the 3 types of errors in Python?
The 3 types of errors in Python are syntax errors, logical errors, and runtime errors.
What is an error in Python?
An error in Python is a mistake made by the programmer in their code that prevents the program from running.
What is error and exception?
Errors and exceptions are two ideas in programming that are related. Errors are mistakes programmers make in their code that stop the program from working, whereas exceptions are unanticipated events that happen while the program is running and stop it from working normally.
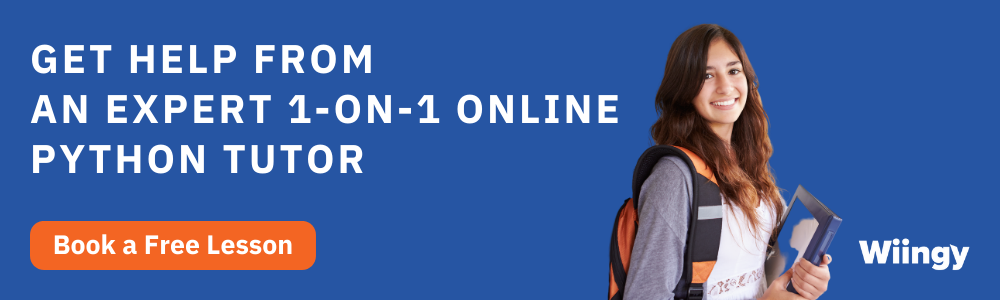
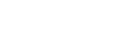
Jan 30, 2025
Was this helpful?