Python Tutorials
What are Python Variables?
Definition of Variables in Python
Variables are essential components of programming languages that allow programmers to store values in a named container. In Python, a variable is created when a value is assigned to it using the assignment operator “=”. The value can be of any data type, including numbers, strings, booleans, and objects. Once a variable is defined, it can be used throughout the program to refer to the stored value.
Importance of Understanding Variables in Python
It’s essential to comprehend variables if you want to understand Python programming. The fundamental units of any program, variables, are heavily utilized in Python coding. The ability to define and use variables is a requirement for creating programs that can carry out intricate operations and address real-world issues.
Overview of the Article
In this article, we will discuss variables in Python, including their definition, importance, and usage. We will cover the different types of variables, including integers, floating-point numbers, strings, and booleans. Additionally, we will discuss the rules for naming variables and best practices for using them in Python code. By the end of this article, you will have a solid understanding of variables in Python and be able to use them effectively in your own programs.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Creating and Initializing Variables in Python
Variable Naming Rules
In Python, variable names must follow specific rules to be valid. Variable names can only contain letters, numbers, and underscores, and they cannot start with a number. Variable names are case-sensitive, so “myVar” and “myvar” are two different variables. It’s also good practice to choose a descriptive name for your variable that reflects its purpose.
Data Types in Python
Python supports various data types, including integers, floating-point numbers, strings, and booleans. Integers are whole numbers, such as 1, 2, and 3. Floating-point numbers are decimal numbers, such as 3.14 or 0.5. Strings are a sequence of characters, such as “hello” or “world.” Booleans have two possible values, True and False, and are often used for logical operations.
How to Declare and Initialize Variables in Python
To declare a python variable , we use the assignment operator “=” and assign a value to the variable. For example, to create a variable called “age” and assign it the value 10, we would use the following code:
age = 10
We can also declare and initialize multiple variables in a single line, like this:
name, age, grade = "John", 10, "5th"
Examples of Initializing Variables
Here are some examples of initializing variables in Python:
# Integer variable
my_age = 12
# Floating-point variable
my_height = 5.6
# String variable
my_name = "Jane"
# Boolean variable
is_student = True
We can also perform operations on variables, such as arithmetic operations on integers and floating-point numbers. For example:
# Adding two integer variables
num1 = 5
num2 = 7
result = num1 + num2
print(result) # Output: 12
# Dividing two floating-point variables
num3 = 3.5
num4 = 2.0
result2 = num3 / num4
print(result2) # Output: 1.75
In summary, creating and initializing variables is an important aspect of programming in Python. By following the naming rules and understanding the different data types, you can create variables that store and manipulate various values in your programs.
Data Types of Python Variables
Numeric Data Types
- Integers
- Floats
- Complex Numbers
Boolean Data Type
Boolean data types represent a logical value that can be either True or False. For example:
# Boolean variable
is_student = True
is_teacher = False
Boolean data types are often used in conditional statements, such as if-else statements and while loops, to control the flow of a program.
String Data Type
String data types represent a sequence of characters. They can be enclosed in single quotes (‘…’) or double quotes (“…”). For example:
# String variable
my_name = "John"
Strings can also be concatenated using the + operator, and individual characters can be accessed using indexing.
List Data Type
List data types represent an ordered collection of items. They can contain elements of different data types, such as integers, strings, and even other lists. For example:
# List variable
my_list = [1, "apple", True, [2, 3, 4]]
Lists can be modified by adding or removing elements using various built-in methods.
Tuple Data Type
Tuple data types are similar to lists but are immutable, meaning that they cannot be modified after they are created. They are often used to store related values that should not be changed. For example:
# Tuple variable
my_tuple = (1, "apple", True)
Tuples can be accessed using indexing, and individual elements can be unpacked into separate variables.
Set Data Type
Set data types represent an unordered collection of unique elements. They can be used to perform set operations such as union, intersection, and difference. For example:
# Set variable
my_set = {1, 2, 3}
Sets can be modified by adding or removing elements using various built-in methods.
Dictionary Data Type
Dictionary data types represent a collection of key-value pairs. They are often used to store data that can be accessed using a specific key. For example:
# Dictionary variable
my_dict = {"name": "John", "age": 25, "is_student": True}
Dictionaries can be modified by adding or removing key-value pairs using various built-in methods. Values can be accessed using their corresponding keys.
Type Conversion and Casting
Implicit Type Conversion
Python automatically converts one data type to another when necessary. This is known as implicit type conversion. For example, if we try to add an integer and a float, Python will convert the integer to a float and perform the addition:
# Implicit type conversion
a = 10 # integer
b = 3.14 # float
c = a + b # integer converted to float
print(c) # Output: 13.14
Explicit Type Conversion
We can also convert one data type to another explicitly using casting. This is known as explicit type conversion. There are built-in functions in Python that can be used to perform type casting. For example:
# Explicit type conversion
a = 10 # integer
b = float(a) # integer converted to float
print(b) # Output: 10.0
We can also convert between different data types using the int(), float(), str(), list(), tuple(), set(), and dict() functions.
Examples of Type Conversion
# Integer to float conversion
a = 10
b = float(a)
print(b) # Output: 10.0
# Float to integer conversion
a = 3.14
b = int(a)
print(b) # Output: 3
# Integer to string conversion
a = 10
b = str(a)
print(b) # Output: '10'
# String to integer conversion
a = '100'
b = int(a)
print(b) # Output: 100
# List to tuple conversion
a = [1, 2, 3]
b = tuple(a)
print(b) # Output: (1, 2, 3)
# Tuple to list conversion
a = (1, 2, 3)
b = list(a)
print(b) # Output: [1, 2, 3]
Type conversion is often used to ensure that the data types of variables are compatible with each other in a program.
Single or Double Quotes?
String Literals in Python
A string literal is a sequence of characters enclosed in quotes. In Python, we can use either single quotes (‘…’) or double quotes (“…”) to create a string. For example:
# Using single quotes
a = 'Hello, World!'
print(a) # Output: Hello, World!
# Using double quotes
b = "Python is awesome!"
print(b) # Output: Python is awesome!
Choosing the Right Quote Type
The choice between using single or double quotes is mostly a matter of personal preference. However, there are some cases where one is more appropriate than the other.
If the string contains a single quote, we should use double quotes to enclose the string literal. Similarly, if the string contains double quotes, we should use single quotes to enclose the string literal. For example:
# Using double quotes when the string contains a single quote
a = "I'm learning Python"
print(a) # Output: I'm learning Python
# Using single quotes when the string contains double quotes
b = 'He said, "Python is easy to learn"'
print(b) # Output: He said, "Python is easy to learn"
Case-Sensitivity in Python Variable Names
Understanding Case-Sensitivity
Python is a case-sensitive language, which means that variable names are distinguished by their case. This means that the variable “my_variable” is different from “My_Variable” and “MY_VARIABLE”.
# Example of case-sensitivity
my_variable = 42
My_Variable = "Hello"
MY_VARIABLE = [1, 2, 3]
print(my_variable) # Output: 42
print(My_Variable) # Output: Hello
print(MY_VARIABLE) # Output: [1, 2, 3]
Conventions for Naming Variables in Python
While Python is case-sensitive, it is recommended to use lowercase letters for variable names. This makes the code easier to read and understand. If a variable name consists of multiple words, it is common to use underscores to separate the words. For example:
# Examples of variable names with underscores
first_name = "John"
last_name = "Doe"
age = 42
In addition to using lowercase letters and underscores, there are some naming conventions that are commonly used in Python. For example, variable names should be descriptive and indicate the purpose of the variable.
# Examples of descriptive variable names
num_students = 25
average_grade = 87.5
is_raining = True
It is also common to use all capital letters for constants, which are values that do not change during the execution of the program.
# Example of a constant variable
PI = 3.14159
By following these conventions, we can make our code more readable and easier to understand for ourselves and others who may be working with our code.
If the string contains both single and double quotes, we can use either triple single quotes or triple double quotes to enclose the string literal. For example:
# Using triple single quotes to enclose a string with both single and double quotes
c = '''She said, "I'm learning Python"'''
print(c) # Output: She said, "I'm learning Python"
# Using triple double quotes to enclose a string with both single and double quotes
d = """He said, 'Python is easy to learn'"""
print(d) # Output: He said, 'Python is easy to learn'
In general, it is best to choose the quote type that makes the code easier to read and understand.
Variable Scope in Python
Global Variables
A global variable is a variable that is defined outside of a function or block of code, which means it can be accessed from anywhere in the program. However, it is important to be careful when using global variables, as they can sometimes lead to unexpected behavior.
# Example of a global variable
x = 10
def my_function():
print(x)
my_function() # Output: 10
Local Variables
A local variable is a variable that is defined within a function or block of code, which means it can only be accessed within that function or block. Local variables have a limited scope, which means they are only available for use within the function or block of code where they are defined.
# Example of a local variable
def my_function():
y = 20
print(y)
my_function() # Output: 20
Scope Hierarchy
Python has a hierarchy of scopes, which determines the order in which variables are searched for within a program. The hierarchy is as follows: local scope, enclosing functions, global scope, and built-in scope. When a variable is referenced in a program, Python first looks for it in the local scope, then in any enclosing functions, then in the global scope, and finally in the built-in scope.
# Example of scope hierarchy
x = 10
def my_function():
x = 20
print(x)
my_function() # Output: 20
print(x) # Output: 10
In this example, the local variable “x” within the function “my_function” takes precedence over the global variable “x”.
Examples of Global and Local Variables
# Example of a global variable
x = 10
def my_function():
global x
x = 20
print(x)
my_function() # Output: 20
print(x) # Output: 20
In this example, the global keyword is used within the function “my_function” to indicate that we want to modify the global variable “x”. Without the global keyword, the function would create a new local variable with the same name as the global variable.
# Example of a local variable
def my_function():
x = 20
print(x)
my_function() # Output: 20
print(x) # NameError: name 'x' is not defined
In this example, the variable “x” is defined within the function “my_function” and is not accessible outside of the function. When we try to print the value of “x” outside of the function, we get a NameError because “x” is not defined in the global scope.
Outputting Variables
Using the print() Function to Output Variables
The print() function is a built-in function in Python that allows you to output variables and other data to the console. Here is an example of using the print() function to output a variable:
# Example of using the print() function to output a variable
x = 10
print(x) # Output: 10
You can also output multiple variables at once by separating them with commas:
# Example of outputting multiple variables with print()
x = 10
y = 20
print(x, y) # Output: 10 20
Formatting Output with f-strings
f-strings are a way of formatting strings in Python that allow you to embed variables directly into the string. To use f-strings, you begin the string with the letter “f” and enclose variables in curly braces. Here is an example:
# Example of using f-strings to format output
name = "Alice"
age = 12
print(f"My name is {name} and I am {age} years old.") # Output: "My name is Alice and I am 12 years old."
You can also perform calculations or manipulate variables within the curly braces:
# Example of using f-strings to manipulate variables in output
x = 10
y = 20
print(f"The sum of {x} and {y} is {x + y}.") # Output: "The sum of 10 and 20 is 30."
Best Practices for Using Variables in Python
Choosing Clear and Descriptive Variable Names
One of the most important best practices when using variables in Python is to choose clear and descriptive variable names. This makes your code more readable and understandable for both yourself and others who may be reading your code. Here are some tips for choosing variable names:
- Use meaningful names that describe what the variable represents.
- Use lowercase letters for variable names.
- Use underscores to separate words in variable names.
- Avoid using single-letter variable names, except in cases where the variable represents a common convention (e.g. “i” for loop indices).
Here is an example of choosing clear and descriptive variable names:
# Example of using clear and descriptive variable names
height_in_inches = 60
weight_in_pounds = 120
body_mass_index = weight_in_pounds / (height_in_inches ** 2) * 703
Using Constants
Constants are variables whose value is intended to remain constant throughout the program. In Python, constants are typically declared using all capital letters to distinguish them from regular variables. Here is an example of using a constant:
# Example of using a constant in Python
TAX_RATE = 0.08
subtotal = 100
total = subtotal * (1 + TAX_RATE)
Avoiding Variable Shadowing
Variable shadowing occurs when a variable declared within a certain scope has the same name as a variable declared in an outer scope. This can cause confusion and unexpected behavior in your code. To avoid variable shadowing, it’s best to use unique variable names and avoid reusing variable names in nested scopes. Here is an example of variable shadowing:
# Example of variable shadowing in Python
x = 10
def foo():
x = 5
print(x)
foo() # Output: 5
print(x) # Output: 10
Minimizing the Use of Global Variables
Global variables are variables that are declared outside of any function or class, and can be accessed from anywhere in the program. While global variables can be convenient, they can also make your code more difficult to understand and maintain. It’s best to use global variables sparingly, and instead pass variables as arguments to functions or create class instances to store data. Here is an example of minimizing the use of global variables:
# Example of minimizing the use of global variables in Python
def calculate_area(radius):
return 3.14 * radius ** 2
def main():
radius = 5
area = calculate_area(radius)
print(f"The area of a circle with radius {radius} is {area}.")
if __name__ == "__main__":
main()
In this example, the radius variable is passed as an argument to the calculate_area() function rather than being declared as a global variable. This makes the code more modular and easier to understand.
Debugging with Variables
In programming, debugging is the process of finding and fixing errors or bugs in the code. Variables are often a common source of errors in code. Here are some common variable-related errors and debugging tips to help you fix them:
Common Variable-Related Errors
- NameError: This error occurs when a variable is referenced before it is defined or if it is misspelled.
# Example
a = 10
print(b)
Output:
NameError: name 'b' is not defined
- TypeError: This error occurs when an operation is performed on a variable of the wrong data type.
Example:
a = 10
b = '5'
print(a + b)
Output:
TypeError: unsupported operand type(s) for +: 'int' and 'str'
- SyntaxError: This error occurs when the code is not written according to the correct syntax rules.
Example:
a = 10
b = 5
print(a + b
Output:
SyntaxError: unexpected EOF while parsing
Debugging Tips and Tricks
- Check variable names: Make sure that you have spelled the variable name correctly and that it has been defined before it is used.
- Check data types: Make sure that you are using variables of the correct data type. If necessary, use type conversion to convert the data type of a variable.
- Use print statements: Use print statements to help you debug your code. Print the value of variables to check if they have the expected value.
What are variables in Python?
Example:
a = 10
b = 5
print("The value of a is:", a)
print("The value of b is:", b)
print("The sum of a and b is:", a + b)
Output:
pythonCopy code
The value of a is: 10
The value of b is: 5
The sum of a and b is: 15
- Use a debugger: A debugger is a tool that allows you to step through your code line by line and see the values of variables at each step. This can be helpful in finding and fixing errors in your code.
By following these debugging tips and best practices, you can easily identify and fix variable-related errors in your code.
Conclusion
In this article, we learned about Python variables, including their definition, importance, and how to create and initialize them. We also explored different data types, type conversion, and variable scope. We discussed best practices for using variables in Python, and common variable-related errors and debugging tips.
Variables are an essential concept in programming and are used to store values that can be used later in the code. By following best practices for variable naming and scope, you can write clean and readable code. Understanding how to use variables correctly can help you write more efficient and effective programs.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs:
What are variables in Python?
Variables in Python are containers that hold values, such as numbers, strings, lists, or other data types. They are used to store and manipulate data within a program.
What is an example of a variable in Python?
An example of a variable in Python is “x”, which can hold a numerical value, such as “5”, or a string value, such as “Hello, World!”
What is a variable?
A variable is a symbolic name that represents a value or data item stored in memory that can be used and manipulated by a program.
What is an array in Python?
In Python, an array is a data structure that stores a fixed-size sequential collection of elements of the same type, such as integers or floats. It is commonly used for numerical computations and data analysis.
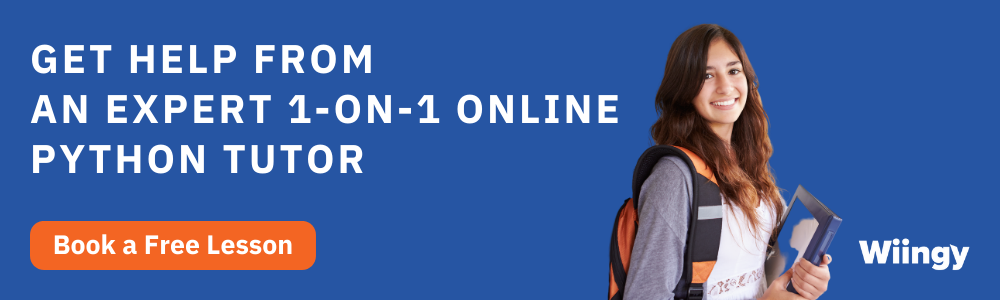
Written by
Rahul LathReviewed by
Arpit Rankwar