Python Tutorials
What are Division Operators in Python?
Division is one of several mathematical operations that programming languages can handle. One of the most frequently used mathematical operations in Python is division. To divide one integer by another and output the result of the division, utilize division operators.
The goal of this article is to explain the different ways to divide in Python and when to use each one. You will know more about Python’s division operators at the conclusion of this article and be able to use them in your programs.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Types of Division Operators in Python
Python offers float division and integer division as its two different division operators. While integer division yields an integer result, floating-point division always results in a floating-point value.
Float Division
Float division is the division of one number by another, resulting in a floating-point number. In Python, the float division operator is the forward slash (/). Here’s an example:
In this example, 7 is divided by 2 using the forward slash operator (/), which results in a floating-point number 3.5.
Integer Division (Floor Division)
Integer division, also known as floor division, is the division of one number by another, resulting in an integer number. In Python, the integer division operator is the double forward slash (//). Here’s an example:
In this example, 7 is divided by 2 using the double forward slash operator (//), which results in an integer number 3.
Floor Division with Negative Numbers
Floor division with negative numbers can be tricky because Python rounds the result towards negative infinity. Here’s an example:
In this example, -7 is divided by 2 using the double forward slash operator (//), which results in an integer number -4. This is because Python rounds towards negative infinity.
Floor Division Use Cases
When we need to divide integers and round to the nearest integer, floor division comes in handy. For instance, we may use floor division to determine how many groups can be created from a given number of objects.
Here’s an example:
In this example, we have 10 items and want to divide them into groups of 3. Using floor division, we can calculate that we can form 3 groups.
Alternative Approaches to Floor Division
In addition to the double forward slash operator (//), there are several alternative approaches to perform floor division in Python. Here are some examples:
- The math.floor() function – This function returns the largest integer less than or equal to a given number. Here’s an example:
- The math.ceil() function – This function returns the smallest integer greater than or equal to a given number. Here’s an example:
- The int() function – This function converts a floating-point number to an integer by rounding down to the nearest integer. Here’s an example:
- The round() function – This function rounds a floating-point number to the nearest integer. Here’s an example:
In the example above, the floating-point result of 3.5 is rounded to the nearest integer, which is 4.
Division Operation in Python 3.x
In Python 3.x, there is a change in the division operator. The forward slash (/) operator now always performs float division, and the double forward slash (//) operator always performs integer division. Here are some examples:
In this example, the forward slash (/) operator produces a floating-point number 3.5, while the double forward slash (//) operator produces an integer number 3.
Conclusion
In this article, we discussed the different types of division operators in Python and their use cases. We explained float division and integer division, provided examples of their use, and discussed floor division with negative numbers. We also covered alternative approaches to floor division and the changes in the division operation in Python 3.x. With this knowledge, you should now be able to use division operators in Python more effectively.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are division operators?
Division is a fundamental arithmetic operation used to distribute a quantity into equal parts. It is the process of splitting a larger number into smaller equal parts. It is one of the four basic arithmetic operations, alongside addition, subtraction, and multiplication. Division is typically denoted by the symbol “÷” or “/” and is the inverse of multiplication.
What are the 4 types of division?
The four types of division are short division, long division, partial quotient division, and chunking division.
Short division: This type of division is a simplified form of long division and is used to divide small numbers quickly. For example, 64 ÷ 8 = 8.
Long division: This type of division is a more complex method for dividing large numbers. It involves several steps and can be used for both whole-number and decimal division. For example, 120 ÷ 4 = 30.
Partial quotient division: This type of division involves breaking the dividend into smaller parts and dividing each part by the divisor. The quotients are then added together to obtain the final result. For example, 145 ÷ 5 = (100 ÷ 5) + (40 ÷ 5) + (5 ÷ 5) = 20 + 8 + 1 = 29.
Chunking division: This type of division involves dividing the dividend into manageable chunks and dividing each chunk by the divisor. The quotient is then multiplied by the divisor and subtracted from the chunk to get the remainder. This process is repeated until all the chunks have been divided. For example, 678 ÷ 6 = (600 ÷ 6) + (70 ÷ 6) + (8 ÷ 6) = 100 + 11 + 1.33 = 112.33.
What is the division operator in C++?
In C++, the division operator “/” is used to perform division of two numbers. It returns the quotient of the division operation. The division operator can be used with both integers and floating-point numbers. If both operands are integers, then the result of the division will be an integer. If either operand is a floating-point number, then the result of the division will be a floating-point number.
For example, consider the following C++ code snippet:
int a = 10, b = 2;
float c = 10.0, d = 3.0;
cout << a / b << endl; // Outputs 5 (integer division)
cout << c / d << endl; // Outputs 3.33333 (floating-point division)
In the above code, the first division operation uses integer division because both operands are integers. The second division operation uses floating-point division because at least one operand is a floating-point number.
What are division operators in Python?
In Python, there are two division operators: “/” for floating-point division and “//” for integer division. The division operator “/” always returns a floating-point number, while the “//” operator returns an integer. The behavior of the division operators depends on the types of the operands.
For example, consider the following Python code:
x = 10
y = 3
z = x / y # floating-point division
w = x // y # integer division
print(z) # Outputs 3.3333333333333335
print(w) # Outputs 3
In the above code, the division operator “/” performs floating-point division, and the “//” operator performs integer division. The result of the “//” operator is the floor of the division, which is the largest integer less than or equal to the result.
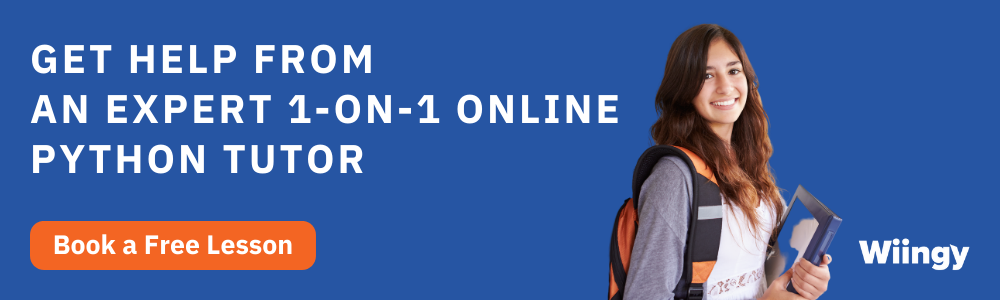
Written by by
Rahul LathReviewed by by
Arpit Rankwar