Python Tutorials
How to get input from console in Python?
Many programs in Python frequently need to accept input from the user. Input can be used to gather data, carry out calculations, or base decisions on user input. The most popular way to receive input in Python is to read it from the console, though there are other options. In this post, we’ll concentrate on utilizing Python’s terminal to take input.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Reading Input using input() function
There is a built-in function in Python called “input()” that lets users enter data from the console.The string parameter that is passed to the input() function is used to ask the user for input.
Here is an example of how to use the input() function to get user input:
In the above code, the input() function is used to prompt the user to enter their name. The input() function takes the string “Enter your name: ” as a parameter, which is used as a prompt for the user to enter their name. The user’s input is stored in the variable “name”. The print() function is then used to output a personalized greeting to the user.
Here is another example of how to use the input() function to get numeric input:
In the above code, the input() function is used to prompt the user to enter their age. The input() function takes the string “Enter your age: ” as a parameter, which is used as a prompt for the user to enter their age. The user’s input is stored in the variable “age” as an integer using the int() function. The print() function is then used to output a message that tells the user what their age will be next year.
Reading Input using command line arguments
Python supports taking input via command-line parameters. Values provided to a program when it is run from the command line are known as command-line arguments. Access to command-line arguments is made available by the sys module through the argv attribute.
Here is an example of how to use command line arguments to get user input:
In the above code, we import the sys module and access the command line arguments using the argv attribute. We assume that the first command line argument is the user’s name, so we store it in the variable “name”. The print() function is then used to output a personalized greeting to the user.
To run the program with command line arguments, you would enter the following command:
In this example, “program_name.py” is the name of the Python program, and “John” is the command line argument passed to the program.
Reading Input from a file
Reading input from a file is a common requirement in many Python programs. Python provides several ways to read input from a file, but the most common method is to use the open() function to open the file and read its contents.
Here is an example of how to read input from a file:
In the above code, we use the open() function to open the file “input.txt” in read mode (‘r’). The with statement ensures that the file is properly closed after it is read. The readlines() method is used to read the contents of the file and store them as a list of strings in the variable “lines”. Finally, we loop through the lines and print each line after removing any whitespace using the strip() method.
To use this code, you would need to create a file named “input.txt” in the same directory as the Python program and add some text to it.
Best Practices and Tips
If you want to avoid making a lot of mistakes when taking input in Python, you should follow some best practices and pointers.
- Verify user input at all times to prevent mistakes and security problems.
- To make it clear what input is needed, employ detailed prompt messages.
- To gently manage input-related failures, use error handling.
- Depending on the type of input you expect, use the right function or method to convert the input to the right data type.
- Use comments in your code to describe the range of input values and how they should be formatted.
Conclusion
This article went over all of the different ways to get input in Python, such as reading from files, the command line, and the console. We’ve also talked about the best ways to take input in Python, like making sure the user’s input is correct and using clear prompt messages.
Effective input handling is a crucial Python programming skill. We can prevent frequent mistakes and make sure our programs are reliable and safe by employing the right approach for gathering feedback and adhering to best practices and guidelines.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
How do you take inputs from console in Python?
To gather user input, we use a built-in function called input ().# input display.
Input_1 = input()
# output display.
print(input_1)
What is console input?
Users can interact with operating system system programs and other console applications through the Console, a window of the operating system. Text input from the standard input (typically the keyboard) or text display on the standard output (typically the computer screen) make up the interaction.
What is console input in Python?
Python console enables line-by-line execution of Python commands and scripts, comparable to Python Shell.
Is input () a command in Python?
Python input() Function
The input() function enables user input.
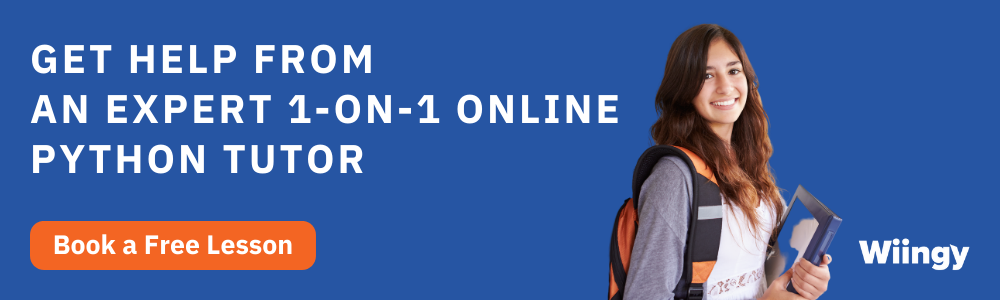
Written by by
Rahul LathReviewed by by
Arpit Rankwar