Python Tutorials
What are comments in Python?
Python comments provide descriptive language to code that isn’t run by the interpreter. Comments are often used to add details to the code, to describe its operation or purpose, or to leave notes for future developers who may work on the code.
The character “#” designates a comment in Python. Every character on the line after the “#” character is ignored by the interpreter. A single line of code or a number of lines of code can have comments added to them.
Here is an example of a single-line comment in Python:
# This is a single-line comment
And here is an example of a multi-line comment in Python:
"""
This is a multi-line
comment in Python.
It can span multiple lines
and is often used to provide
detailed explanations
"""
Python comments are crucial for a number of reasons. First, by supplying context and explanation, comments can aid in improving the readability of code. New programmers who are attempting to learn how to understand and write Python code may find this to be extremely beneficial.
Second, by making it simpler to comprehend the intent and operation of code, comments can aid in the prevention of errors. Developers are less likely to make errors when writing or altering code when they are aware of what it is intended to do.
Finally, comments provide notes for upcoming developers who may need to work on the code, making it easier to maintain. When code is documented with comments, it is simpler for others to comprehend how it functions and to make any necessary adjustments.
This article will discuss the many kinds of Python comments and how to successfully use them. We will also go over some common errors to avoid and give examples of the best practices for commenting code. You ought to have a firm grasp of how to utilize comments to make your Python code more comprehensible, error-free, and manageable by the end of this tutorial.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Single-Line Comments
Comments in Python Single-line comments in Python start with a ‘#’ character and continue until the end of the line. The ‘#’ character must be the first character on the line to indicate that it is a comment and should be ignored by the interpreter. Here is an example:
# This is a single-line comment
Single-line comments can be added to the end of a line of code or on a separate line by themselves.
Here are some examples of single-line python comments:
# Calculate the sum of two numbers
sum = num1 + num2
# Print the result to the console
print(sum)
In this example, the first comment explains the purpose of the code that follows, while the second comment explains what the code does.
# Get user input for their age
age = input("Enter your age: ")
# Convert the age to an integer
age = int(age)
# Check if the user is old enough to vote
if age >= 18:
print("You are old enough to vote!")
else:
print("Sorry, you are not old enough to vote.")
In this example, the comments provide additional information about what the code does at each step, making it easier to understand.
# This line of code does not actually do anything
# It is just here as an example of a comment
In this example, the comment is not providing any useful information about the code, but is instead just used as an example to demonstrate how comments work.
Single-line comments can be used to explain any part of the code that may be unclear, to provide additional information, or to make notes for future reference. It is important to use comments sparingly and only when necessary to avoid cluttering the code with unnecessary information.
Multiline Comments
Multiline comments in Python are enclosed within triple quotes (”’ or “””) and can span multiple lines of code. The interpreter ignores everything inside the triple quotes, allowing developers to add comments that span multiple lines of code. Here is an example:
"""
This article will explain
Python Comments in detail
with examples, for beginners
to learn with ease.
"""
Multiline comments can be used to provide more detailed explanations or to describe complex algorithms that may be difficult to understand from the code alone.
Here are some examples of multiline comments in Python:
"""
This program calculates the
area of a rectangle.
The user is prompted to enter the width and height of the
rectangle, which are then used
to calculate the area.
The area is then printed to
the console.
"""
width = int(input("Enter the width: "))
height = int(input("Enter the height: "))
area = width * height
print("The area of the rectangle is:", area)
In this example, the multiline comment provides a detailed explanation of what the code does, including information about how the user is prompted for input and how the area of the rectangle is calculated.
"""
This function calculates the
factorial of a given number.
The function takes a single
parameter, n, which is the
number to calculate the factorial
of.
The function returns the factorial
of n.
"""
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n-1)
In this example, the multiline comment provides a detailed explanation of how the factorial function works, including information about the input parameter and the return value.
Multiline comments are useful for documenting complex code, providing detailed explanations, and making notes for future reference. It is important to use multiline comments sparingly and only when necessary to avoid cluttering the code with unnecessary information.
Docstrings: Documenting Your Code using Python Comments
Python docstrings are used to explain the functionality of Python code and to describe it. They are usually inserted at the start of a module, function, or class and are enclosed in triple quotes. Docstrings differ from comments in that they may be accessed programmatically and are used to provide more structured documentation.
There are three main types of docstrings in Python:
- Module Docstrings– Module docstrings are placed at the start of the file and give an overview of the module. Often, they explain how the module works, how to use it, and any important notes or instructions. Here is an example:
"""
This module provides a set of functions for
working with strings in Python.
Functions include:
- reverse_string: reverses a given string
- uppercase_string: converts a given string to uppercase
- lowercase_string: converts a given string to lowercase
"""
- Function Docstrings– Function docstrings are given at the beginning of a function’s definition and explain what the function does. They often give details about the function’s purpose, the parameters it requires, and the results it produces. Here is an example:
def calculate_sum(num1, num2):
"""
Calculates the sum of two numbers.
Parameters:
- num1: an integer
- num2: an integer
Returns:
The sum of num1 and num2
"""
return num1 + num2
- Class Docstrings– Class docstrings are at the beginning of a class’s definition and give information about that class. Most of the time, they tell you what the class does, how it works, and any important notes or warnings. Here is an example:
class Car:
"""
A class representing a car.
Methods include:
- start_engine: starts the car's engine
- stop_engine: stops the car's engine
- drive: drives the car
"""
def start_engine(self):
pass
def stop_engine(self):
pass
def drive(self):
pass
Here are some examples of how docstrings can be used to document Python code:
"""
This program calculates the
area of a rectangle.
To use the program, run it and
follow the prompts to enter the
width and height of the rectangle.
The area of the rectangle will
then be printed to the console.
"""
def calculate_area():
"""
Calculates the area of a rectangle.
Prompts the user to enter the width and
height of the rectangle, and then calculates
the area using the formula: width * height.
Returns:
The area of the rectangle.
"""
width = int(input("Enter the width: "))
height = int(input("Enter the height: "))
area = width * height
return area
In this example, the module docstring provides an overview of the program and what it does, while the function docstring provides detailed information about how the calculate_area function works.
class Rectangle:
"""
A class representing a rectangle.
Attributes:
- width: the width of the rectangle
- height: the height of the rectangle
Methods:
- get_area: returns the area of the rectangle
- get_perimeter: returns the perimeter of the rectangle
"""
def __init__(self, width, height):
self.width = width
self.height = height
def get_area(self):
"""
Calculates the area of the rectangle.
Returns:
Difference between Docstrings and Multiline Comments
While both docstrings and multiline comments can be used to provide information about Python code, there are some important differences between them:
- Docstrings are structured and provide information that can be accessed programmatically, while multiline comments are unstructured and provide information that is only meant for human readers.
- Docstrings are enclosed within triple quotes and are typically placed at the beginning of a module, function, or class, while multiline comments can be placed anywhere in the code.
- Docstrings are used to document code, while multiline comments are used to explain or clarify specific sections of code.
Here are some examples of how both docstrings and multiline comments can be used in Python code
"""
This program calculates the
area of a rectangle.
To use the program, run it and
follow the prompts to enter the
width and height of the rectangle.
The area of the rectangle will
then be printed to the console.
"""
def calculate_area():
# Prompt the user to enter the width and height of the rectangle
width = int(input("Enter the width: "))
height = int(input("Enter the height: "))
# Calculate the area of the rectangle using the formula: width * height
area = width * height
# Return the area of the rectangle
return area
In this example, a module docstring is used to provide an overview of the program and what it does, while a combination of single-line and multiline comments are used to explain the specific sections of code.
class Rectangle:
"""
A class representing a rectangle.
Attributes:
- width: the width of the rectangle
- height: the height of the rectangle
Methods:
- get_area: returns the area of the rectangle
- get_perimeter: returns the perimeter of the rectangle
"""
def __init__(self, width, height):
self.width = width
self.height = height
def get_area(self):
"""
Calculates the area of the rectangle.
Returns:
The area of the rectangle.
"""
# Calculate the area of the rectangle using the formula: width * height
area = self.width * self.height
# Return the area of the rectangle
return area
In this example, a class docstring is used to provide information about the Rectangle class and its attributes and methods, while a function docstring is used to provide information about the get_area method. Multiline comments are also used to explain specific sections of code within the get_area method
Best Practices for Writing Comments in Python
It is important to include comments that provide an overview of the purpose and design of your code. This can help other developers understand your code and how it fits into the overall project. For example:
"""
This program reads a text file
containing a list of names and
prints the names in alphabetical order.
"""
In addition to providing an overview of your code, it is important to include comments that explain specific code blocks and variables. This can help other developers understand what the code does and how it works. For example:
# Create an empty list to store the names
names = []
# Open the file containing the names
with open('names.txt', 'r') as f:
# Read each line in the file and add the name to the list
for line in f:
names.append(line.strip())
# Sort the names in alphabetical order
names.sort()
# Print the sorted names
for name in names:
print(name)
It is important to strike a balance between over-commenting and under-commenting. Over-commenting can make the code difficult to read and understand, while under-commenting can make it difficult for other developers to understand the code. A good rule of thumb is to include comments that explain what the code does, but not how it does it. For example:
# Calculate the area of the rectangle using th formula: width * height
area = width * height
It is important to maintain consistency in your commenting style. This can help make the code easier to read and understand. Some common commenting styles include:
- Using single-line comments for short comments and inline comments
- Using multiline comments for longer comments and docstrings
- Using descriptive variable names that make it clear what the variable represents
By following these best practices, you can write comments that help make your Python code easier to read and understand for both yourself and other developers.
Tools for Generating Documentation from Your Comments
Sphinx is a well-liked tool for creating documentation from the comments and docstrings in your Python code. It works especially effectively for producing documentation for bigger projects. The documentation is produced by Sphinx using the reStructuredText markup language and can be altered using themes and extensions.
Doxygen is an extra tool for creating documentation from your code’s comments and docstrings. It can make output in many different formats, like HTML, LaTeX, and PDF, and it works with many programming languages, including Python. Similar to Javadoc, Doxygen’s unique syntax for comments is used.
Python comes with a tool called Pydoc that you can use to create documentation for your code. Pydoc creates HTML documentation that can be seen in a web browser by using the docstrings in your code.
Here is an example of using Sphinx to generate documentation for a Python module:
- First, install Sphinx using pip:
pip install sphinx
- Create a directory for your documentation and navigate to it:
mkdir docs
cd docs
- Initialize Sphinx in your documentation directory:
sphinx-quickstart
- Follow the prompts to configure your documentation project.
- Once you have configured your project, you can generate documentation by running:
make html
- Your documentation will be generated in the _build directory.
Here is an example of using Pydoc to generate documentation for a Python module:
- Add docstrings to your Python code.
- Generate documentation by running:
python -m pydoc -w mymodule.py
- This will generate an HTML file called mymodule.html that contains documentation for your module.
By using documentation generation tools like Sphinx, Doxygen, and Pydoc, you can create professional-looking documentation for your Python code that is easy to read and understand.
Commenting in Collaborative Environments
Guidelines for Commenting in Group Projects– When working on group projects, it’s important to establish guidelines for commenting to ensure that everyone can understand and contribute to the codebase. Here are some general guidelines to follow:
- Be descriptive: Comments should describe the purpose and behavior of the code they are associated with. Use clear and concise language to explain what the code is doing.
- Be consistent: Establish a consistent commenting style for the entire project. This makes the codebase easier to read and understand.
- Avoid redundant comments: Don’t repeat what the code is already saying. Instead, focus on explaining the purpose and intent behind the code.
- Comment your changes: When making changes to existing code, make sure to comment on what you changed and why. This helps other developers understand the thought process behind the change and makes it easier to review.
Using Version Control to Track Comments and Changes- Version control systems like Git can be used to track changes to code and comments over time. When working on group projects, it’s a good idea to use a version control system to track changes and ensure that everyone is working with the latest version of the code.
Here are some tips for using version control to track comments and changes:
- Use descriptive commit messages: When making changes to the codebase, use descriptive commit messages to explain what you changed and why. This makes it easier for other developers to understand the changes you made.
- Use branches: When working on new features or making significant changes to the codebase, create a new branch in the version control system. This allows you to work on the changes independently without affecting the main codebase.
- Review changes: Before merging changes into the main codebase, review the changes with other developers to ensure that they understand the changes and that they don’t introduce any new bugs or issues.
By following these guidelines and using version control to track changes and python comments, you can ensure that your group project is well-documented and easy to understand for all developers involved.
Conclusion
In this article, we discussed the importance of Python comments and explored the different types of comments available to developers. We looked at single-line comments, multiline comments, and docstrings, and provided examples of each. We also discussed best practices for writing Python comments, such as being descriptive, avoiding over-commenting or under-commenting, and maintaining consistency in your commenting style. Finally, we talked about using documentation generation tools and version control to track comments and changes in collaborative environments.
Comments are an essential part of any programming language, including Python. They provide a way for developers to explain their code, document its purpose and behavior, and help others understand what the code does. By following best practices for commenting and using documentation generation tools and version control, developers can ensure that their codebase is well-documented and easy to understand for everyone involved.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are the types of comment in Python?
There are three types of comments in Python:
Single-line comments: denoted by a hash symbol (#) and only affect the line they are on.
Multi-line comments: enclosed in triple quotes (”’ or “””) and can span across multiple lines.
Documentation comments: used to document modules, classes, functions, and methods and enclosed in triple quotes (”’ or “””).
How do I comment out multiple lines in Python?
To comment out multiple lines in Python, you can enclose the lines you want to comment with triple quotes (”’ or “””), like this:'''
This is a comment
This is another comment
This is a third comment
'''
What is comment function in Python?
There is no specific “comment function” in Python. Comments are used to provide additional information about the code and are denoted by a hash symbol (#) for single-line comments or triple quotes (”’ or “””) for multi-line comments.
What is the shortcut to comment in Python?
For different IDEs and Code Editors,
select Code | Comment with Line Comment.
Press Ctrl+/ or Ctrl + 1 for single line, Ctrl + 4 for multi-line (4 lines) etc
How do you check if a line is a comment in Python?
Python string has a nice method “startswith” to check if a string, in this case a line, starts with specific characters. For example, “#comment”. startswith(“#”) will return TRUE. If the line does not start with “#”, we execute the else block.
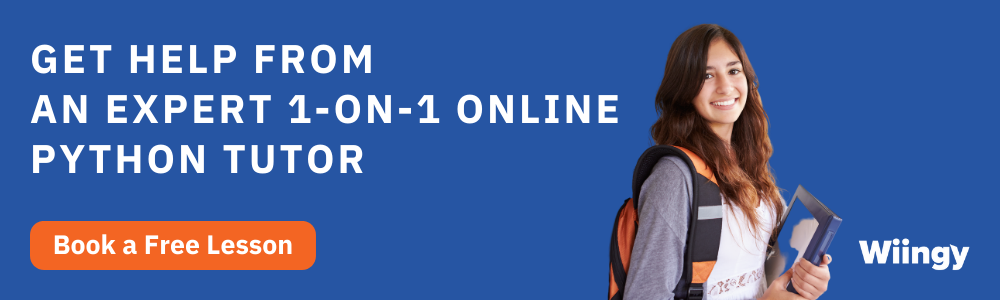
Written by
Rahul LathReviewed by
Arpit Rankwar