Python Tutorials
What are Python Strings?
Python strings are needed by programmers because they can represent and manipulate text data. Strings can be used to output program results, interact with files and databases, and store and display user input. Additionally, applications for artificial intelligence, machine learning, data analysis, and web development use strings.
It may also include special characters, numbers, and letters. Words, sentences, and paragraphs are all examples of text data that are represented by strings. Since strings in Python are immutable, they cannot be changed once they have been created. However, you can create a new string by combining or modifying existing strings.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Creating Strings in Python
Here’s how to create strings in python:
A. Single and double quotes:
You can create a string in Python by enclosing text in either single quotes (‘ ‘) or double quotes (” “). Both single and double quotes are interchangeable and can be used to represent a string.
Example:
# Using single quotes str1 = 'Hello, World!' # Using double quotes str2 = "Hello, World!"
B. Triple quotes:
Python also allows you to create multi-line strings using triple quotes (”’ ”’) or (“”” “””). Triple quotes are used when you want to create a string that spans multiple lines.
Example:
# Using triple quotes str3 = '''Python is a high-level programming language used for web development, data analysis, and machine learning.'''
C. Escape Characters:
Escape characters are special characters that are used to represent non-printable characters, such as newlines, tabs, and quotes, within a string. Escape characters are represented using a backslash () followed by a special character.
Example:
# Using escape characters str4 = "He said, \"I'm learning Python!\""
In the above example, the backslash before the double quote represents an escape character and allows us to include a double quote within the string.
String Operations:
A. Concatenation:
Concatenation is the process of combining two or more strings into a single string. In Python, you can concatenate strings using the + operator.
Example:
# Concatenation str1 = "Hello, " str2 = "World!" str3 = str1 + str2 print(str3) # Output: Hello, World!
B. Repetition:
Repetition is the process of repeating a string a certain number of times. In Python, you can repeat a string using the * operator.
Example:
# Repetition str1 = "Hello! " str2 = str1 * 3 print(str2) # Output: Hello! Hello! Hello!
C. Indexing:
Indexing is the process of accessing individual characters in a string. In Python, you can access a character in a string using its index, which starts from 0.
Example:
# Indexing str1 = "Python" print(str1[0]) # Output: P print(str1[3]) # Output: h
D. Slicing:
Slicing is the process of accessing a substring from a string. In Python, you can slice a string using the colon (:) operator.
Example:
# Slicing str1 = "Python" print(str1[0:3]) # Output: Pyt print(str1[1:]) # Output: ython
E. Length:
Length is the process of finding the number of characters in a string. In Python, you can find the length of a string using the len() function.
Example:
# Length str1 = "Python" print(len(str1)) # Output: 6
F. Changing case:
Changing case is the process of converting the case of a string. In Python, you can convert a string to uppercase or lowercase using the upper() and lower() methods, respectively.
Example:
# Changing case str1 = "Python" print(str1.upper()) # Output: PYTHON print(str1.lower()) # Output: python
G. Stripping:
Stripping is the process of removing leading and trailing whitespace from a string. In Python, you can strip a string using the strip() method.
Example:
# Stripping str1 = " Hello, World! " print(str1.strip()) # Output: Hello, World!
H. Replacement:
A substring in a string is replaced by another substring through the replacement process. In Python, you can replace a substring using the replace() method.
Example:
# Replacement str1 = "Hello, World!" str2 = str1.replace("World", "Python") print(str2) # Output: Hello, Python!
Splitting and Joining:
Splitting is the process of breaking a string into a list of substrings. In Python, you can split a string using the split() method.
Joining is the process of combining a list of substrings into a single string. In Python, you can join a list of substrings using the join() method.
Example:
# Splitting and Joining str1 = "Hello, World!" str2 = str1.split(", ") print(str2) # Output: ['Hello', 'World!'] str3 = "-".join(str2) print(str3) # Output: Hello-World!
String Formatting
A. Using placeholders:
String placeholders are used to insert values into a string. In Python, you can use placeholders by using the % operator.
Example:
# Using placeholders name = "John" age = 25 print("My name is %s and I am %d years old." % (name, age)) # Output: My name is John and I am 25 years old.
B. Using format method:
The format() method is an alternative to using placeholders. It allows you to insert values into a string by using curly braces {}.
Example:
# Using format method name = "John" age = 25 print("My name is {} and I am {} years old.".format(name, age)) # Output: My name is John and I am 25 years old.
C. Using f-strings:
f-strings (formatted string literals) are a new feature in Python 3.6 that allows you to embed expressions inside string literals. They are similar to using placeholders but are more concise and easier to read.
Example:
# Using f-strings name = "John" age = 25 print(f"My name is {name} and I am {age} years old.") # Output: My name is John and I am 25 years old.
By mastering these concepts and examples, you will be able to use strings effectively in Python.
Advanced String Operations
A. Regular Expressions:
Regular expressions (regex) are patterns used to match text in a string. They can be used for advanced text manipulation, such as finding and replacing text, searching for specific patterns, and validating input.
Example:
import re text = "The quick brown fox jumps over the lazy dog." pattern = r"fox" match = re.search(pattern, text) print(match.group()) # Output: fox
B. String comparison:
String comparison is the process of comparing two strings to determine if they are equal or not. In Python, you can compare strings using the == operator, which returns True if the strings are equal and False if they are not.
Example:
str1 = "hello" str2 = "world" if str1 == str2: print("Strings are equal.") else: print("Strings are not equal.") # Output: Strings are not equal
C. String interpolation:
String interpolation is the process of embedding values into a string. In Python, you can use f-strings (formatted string literals) for string interpolation.
Example:
name = "John" age = 25 print(f"My name is {name} and I am {age} years old.") # Output: My name is John and I am 25 years old
String Methods:
A. strip():
The strip() method removes whitespace from the beginning and end of a string.
Example:
text = " hello " print(text.strip()) # Output: "hello"
B. split():
The split() method splits a string into a list of substrings based on a delimiter.
Example:
text = "The quick brown fox" print(text.split()) # Output: ['The', 'quick', 'brown', 'fox']
C. join():
The join() method concatenates a list of strings into a single string.
Example:
words = ['The', 'quick', 'brown', 'fox'] print(" ".join(words)) # Output: 'The quick brown fox'
D. find():
The find() method returns the index of the first occurrence of a substring in a string.
Example:
text = "The quick brown fox" print(text.find("brown")) # Output: 10
E. replace():
The replace() method replaces all occurrences of a substring in a string with another substring.
Example:
text = "The quick brown fox" print(text.replace("brown", "red")) # Output: 'The quick red fox'
F. count():
The count() method returns the number of occurrences of a substring in a string.
Example:
text = "The quick brown fox" print(text.count("o")) # Output: 3
G. startswith() and endswith():
The startswith() and endswith() methods return True if a string starts or ends with a specified substring.
Example:
text = "The quick brown fox" print(text.startswith("The")) # Output: True print(text.endswith("fox")) # Output: True
H. isalpha(), isnumeric(), isalnum(), isspace():
The isalpha() method returns True if all characters in a string are alphabetic.
Example:
text = "The quick brown fox" print(text.isalpha()) # Output: False
The isnumeric() method returns True if all characters in a string are numeric.
Example:
text = "12345" print(text.isnumeric()) # Output: True
The isalnum() method returns True if all characters in a string are alphanumeric.
Example:
text = "The quick brown fox 123" print(text.isalnum()) # Output: False
The isspace() method returns True if all characters in a string are whitespace.
Example:
text = " " print(text.isspace()) # Output: True
I. capitalize(), title(), upper(), lower():
The capitalize() method capitalizes the first character of a string.
Example:
The title() method capitalizes the first character of each word in a string.
Example:
text = "the quick brown fox" print(text.title()) # Output: 'The Quick Brown Fox'
The upper() method converts all characters in a string to uppercase.
Example:
text = "the quick brown fox" print(text.upper()) # Output: 'THE QUICK BROWN FOX'
The lower() method converts all characters in a string to lowercase.
Example:
text = "THE QUICK BROWN FOX" print(text.lower()) # Output: 'the quick brown fox'
J. format_map():
The format_map() method formats a string using a dictionary.
Example:
person = {"name": "John", "age": 25} print("My name is {name} and I am {age} years old.".format_map(person)) # Output: 'My name is John and I am 25 years old.'
K. translate():
The translate() method returns a string where some specified characters are replaced with the character described in a dictionary, or in a mapping table.
Example:
text = "Hello, World!" table = text.maketrans("H", "J") print(text.translate(table)) # Output: 'Jello, World!'
Encoding and Decoding Strings
Python has a built-in encode() method that enables us to convert a string into a particular format, and a decode() method that decodes an encoded string back into its original format.
A. Unicode:
No matter the platform, program, or language, every character has a unique number thanks to the character encoding standard known as Unicode. It covers all scripts, characters, and symbols worldwide.
B. UTF-8:
The variable-length Unicode encoding UTF-8 makes use of 8-bit bytes. It is frequently used because it can represent any character in the Unicode standard and is backwards compatible with ASCII.
C. encode():
The encode() method converts a string into a specified encoding format.
Example:
text = "Hello, World!" encoded_text = text.encode(encoding="utf-8") print(encoded_text) # Output: b'Hello, World!'
D. decode():
The decode() method decodes a string from a specified encoding format.
Example:
encoded_text = b'Hello, World!' decoded_text = encoded_text.decode(encoding="utf-8") print(decoded_text) # Output: 'Hello, World!'
Regular Expressions:
A. re module:
Regular expressions are supported by Python’s re module.
B. Searching and Matching Patterns:
A search pattern is defined by a string of characters called a regular expression. They can be used for text manipulation, matching, and search. For instance, you can use regular expressions to search for every email address present in a text.
C. group():
The group() method returns the part of the string that matched the regular expression.
Example:
import re text = "The price of a stock is $100" pattern = r"\$(\d+)" match = re.search(pattern, text) print(match.group(1)) # Output: '100'
D. findall() and finditer():
The findall() method returns all non-overlapping matches of a regular expression in a string.
Example:
import re text = "The price of a stock is $100, but I bought it for $90" pattern = r"\$(\d+)" matches = re.findall(pattern, text) print(matches) # Output: ['100', '90']
The finditer() method returns an iterator of match objects of a regular expression in a string.
Example:
import re text = "The price of a stock is $100, but I bought it for $90" pattern = r"\$(\d+)" matches = re.finditer(pattern, text) for match in matches: print(match.group(1)) # Output: '100', '90'
E. sub():
The sub() method replaces all occurrences of a regular expression in a string with a new string.
Example:
import re text = "The price of a stock is $100, but I bought it for $90" pattern = r"\$(\d+)" new_text = re.sub(pattern, "X", text) print(new_text) # Output: 'The price of a stock is X, but I bought it for X'
String Formatting:
String formatting is also referred to as string interpolation. It involves adding a unique string or variable to predefined text.
A. Using format method with positional and named arguments
You can use the format() method to insert values into a string. You can use positional arguments or named arguments.
Example:
name = "John" age = 25 print("My name is {} and I am {} years old.".format(name, age)) # Output: 'My name is John and I am 25 years old.'
B. Using format specifiers for precision, alignment, and conversion:
You can use format specifiers to control the formatting of values in a string. You can specify precision, alignment, and conversion.
- Precision: Precision specifies the number of digits to be displayed after the decimal point in a floating-point number. You can specify precision by placing a dot (.) followed by the number of digits after the decimal point. For example:
x = 123.4567 print("{:.2f}".format(x)) # output: 123.46
In this example, the format specifier {:.2f} tells Python to display x with two digits after the decimal point.
- Alignment: Alignment specifies how the values in a string are aligned. You can specify alignment by placing a colon (:) followed by a symbol indicating the alignment. The symbol < means left-aligned, > means right-aligned, and ^ means centered. For example:
name = "Alice" age = 30 print("{:<10} is {:>5} years old.".format(name, age)) # output: "Alice is 30 years old."
In this example, the format specifier {:<10} means that the first value should be left-aligned with a width of 10 characters, while the specifier {:>5} means that the second value should be right-aligned with a width of 5 characters.
- Conversion: Conversion specifies the type of conversion to be applied to a value. You can specify conversion by placing a colon (:) followed by a letter indicating the type of conversion. The letter d means integer conversion, f means floating-point conversion, s means string conversion, and b means binary conversion. For example:
x = 42 print("{:b}".format(x)) # output: 101010
In this example, the format specifier {:b} tells Python to convert x to binary format.
In addition to these basic format specifiers, there are many other options you can use to control the formatting of strings in Python. The official Python documentation provides a comprehensive list of format specification options.
C. Using format strings with dictionaries:
You can use dictionaries to substitute values in a string. To do this, you use named placeholders in the string that correspond to keys in the dictionary. For example:
person = {'name': 'Alice', 'age': 30} print("{name} is {age} years old.".format(**person)) # output: "Alice is 30 years old."
In this example, the placeholders {name} and {age} correspond to the keys ‘name’ and ‘age’ in the dictionary person. The double asterisks (**person) are used to unpack the dictionary and pass its key-value pairs as named arguments to the format method.
D. Using template strings:
For formatting strings, template strings offer a less complex syntax that is comparable to the syntax used in other programming languages. To create a template string, you use placeholders enclosed in braces ({}) instead of percent signs (%) or format specifiers. For example:
from string import Template name = "Alice" age = 30 template = Template("$name is $age years old.") print(template.substitute(name=name, age=age)) # output: "Alice is 30 years old."
Conclusion
The fundamentals of Python strings, including string creation, string operations, string formatting, and advanced string operations, have been covered in this article. Additionally, we looked at some typical string methods.
Since the beginning of the language, Python strings have been used extensively and will continue to be so. We can anticipate new Python features and enhancements to the language’s string handling capabilities as its popularity continues to rise.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs:
What is strings in Python?
A string in Python is a sequence of characters enclosed within single, double or triple quotes. It is a datatype used to represent textual data.
What is string in Python with example?
A string in Python is a sequence of characters enclosed within quotes. For example:
# string with double quotes string1 = “Hello, World!” # string with single quotes string2 = ‘Python is fun’ # string with triple quotes string3 = ”’This is a multi-line string”’
How many types of strings are there in Python?
In Python, there are three types of strings:
Single quoted string
Double quoted string
Triple quoted string
What is {{ }} in Python?
Literal text is anything that is not enclosed in braces and is copied to the output in its original form. If you need to include a brace character in the literal text, it can be escaped by doubling: {{ and }} .
Other Useful Reads
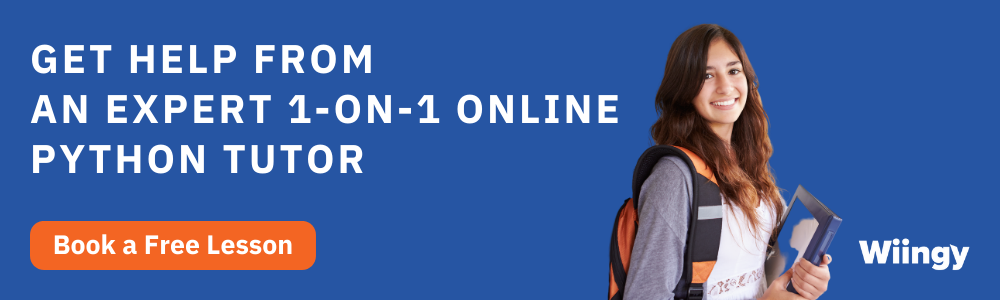
Written by by
Rahul LathReviewed by by
Arpit Rankwar