Python Tutorials
Taking input in python can be performed using various different functions available in their libraries. The built-in input() method in Python, its syntax and parameters, taking various types of input, handling input failures and exceptions, best practices for correct input processing, and hints and tips for effective input handling will all be covered in this article.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Using Python’s Built-in input() Function
The user can input data from the keyboard using Python’s input() function. The function returns the user-entered data as a string and is straightforward to use.
Here’s an example of using the input() function:
name = input("Enter your name: ")
print("Hello, " + name)
In this example, we use the input() function to ask the user to enter their name. The input() function takes an optional string prompt as an argument, which is displayed to the user before waiting for input. Once the user enters their name, we print a greeting that includes their name.
The input in python can also be used to accept input of different types, including strings and numbers. Here’s an example of accepting a number from the user:
age = int(input("Enter your age: "))
print("You are " + str(age) +
In this example, we use the input() function to accept the user’s age as an integer. We convert the user’s input to an integer using the int() function and then print out the user’s age as a string.
Syntax and Parameters of the input() Function
The syntax of the input() function is simple. The function takes an optional string prompt as an argument and returns the data entered by the user as a string. Here’s the syntax:
input([prompt])
The prompt parameter is optional and is used to display a message to the user before waiting for input. If the prompt parameter is not specified, the input() function will simply wait for the user to enter data.
The input() function can also be used to accept multiple inputs in python in a single line. Here’s an example:
age = int(input("Enter your age: "))
print("You are " + str(age) + " years old.")
In this example, we use the input() function to accept the user’s name and age in a single line. We use the split() method to separate the user’s input into two variables, name and age.
Accepting Different Types of Input in Python
Python allows users to accept different types of input, including numeric and string inputs. Here are some examples:
- Accepting a number as input:
num = int(input("Enter a number: "))
print("The square of " + str(num) + " is " + str(num*num))
In this example, we use the input() function to accept a number from the user. We convert the user’s input to an integer using the int() function and then calculate and print the square of the number.
- Accepting a string as input:
string = input("Enter a string: ")
print("The length of the string is " + str(len(string)))
In this example, we use the input() function to accept a string from the user. We use the len() function to calculate the length of the string and then print the result.
It is important to handle errors and exceptions when accepting user input. For example, if the user enters a string when a number is expected, a ValueError will occur. We will discuss how to handle these types of errors in the next section.
Handling Input Errors and Exceptions in Python
As mentioned earlier, it is important to handle errors and exceptions that may occur when accepting user input. In Python, we can use try-except blocks to handle exceptions. A try block contains the code that may raise an exception, while an except block contains the code to handle the exception if it occurs.
Let’s consider an example where the user is expected to enter a number, but instead enters a string:
try:
number = int(input("Enter a number: "))
print("The number is", number)
except ValueError:
print("Invalid input. Please enter a number.")
In the code above, we use the int() function to convert the user input to an integer. If the user enters a string that cannot be converted to an integer, a ValueError exception will occur. In the except block, we catch this exception and print an error message.
We can also handle multiple types of exceptions in the same try-except block. For example
try:
number = int(input("Enter a number: "))
result = 100 / number
print("The result is", result)
except ValueError:
print("Invalid input. Please enter a number.")
except ZeroDivisionError:
print("Cannot divide by zero.")
In the code above, we catch both ValueError and ZeroDivisionError exceptions. If the user enters a string that cannot be converted to an integer, the first except block will handle the exception. If the user enters the number zero, the second except block will handle the exception.
It is also possible to catch all types of exceptions using a single except block:
try:
number = int(input("Enter a number: "))
result = 100 / number
print("The result is", result)
except Exception as e:
print("An error occurred:", e)
In the code above, the except block catches any type of exception that may occur and prints an error message with the exception information.
Best Practices for Proper Input Handling in Python
When accepting user input in a Python program, it is important to follow best practices to ensure the input is handled properly. Here are some best practices to keep in mind:
- Input validation: Always validate user input to ensure it is in the expected format and within the expected range. This helps to prevent errors and exceptions.
- Meaningful prompts: Provide clear and meaningful prompts to the user so that they know what type of input is expected. This also helps to prevent errors and exceptions.
- Proper formatting: Format user input properly to ensure consistency and prevent errors. For example, use lowercase or uppercase consistently, and remove leading or trailing whitespace.
Let’s see how we can implement these best practices in a Python program. Consider the following example, where the user is asked to enter their age:
while True:
try:
age = int(input("Enter your age: "))
if age < 0 or age > 120:
print("Invalid age. Please enter a number between 0 and 120.")
else:
print("Your age is", age)
break
except ValueError:
print("Invalid input. Please enter a number.")
In the code above, we use a while loop to ensure that the user enters a valid age. We first check if the age is within the expected range, and if not, we print an error message and ask the user to try again. If the age is valid, we print a message with the age and break out of the loop. We catch any ValueError exceptions that might occur, which could happen if the user inputs a non-numeric value. In this case, we print an error message and prompt the user to enter a valid age again.
Handling exceptions in this way can improve the user experience by guiding them to enter valid input, and prevent the program from crashing due to unexpected input.
It’s important to note that a variety of other exceptions can happen when working with user input, depending on the sort of input anticipated and the program’s environment. These are a few instances of frequent exceptions that could happen:
A TypeError is thrown when an operation or function is applied to an object of the incorrect type.For instance, attempting to multiply an integer by a string will result in a TypeError.
When a name (variable, function, module, etc.) cannot be found, a NameError is raised. This can occur if a module or function name is spelled incorrectly, a variable is referred to before it has been defined, or both.
When an attribute of an object does not exist, an AttributeError is raised. If you try to access an attribute that hasn’t been declared or doesn’t fit the type of the object, this may happen.
To handle exceptions like these, you can use a try…except block. Here’s an example:
try:
x = int(input("Enter a number: "))
print("You entered:", x)
except ValueError:
print("Error: Invalid input. Please enter a number.")
In this code, we use a ‘try…except’ block to catch any ValueError exceptions that might occur when we try to convert the user’s input to an integer. If a ValueError occurs, we print an error message and prompt the user to enter a valid input again.
By handling exceptions in this way, we can make our programs more robust and user-friendly, and prevent them from crashing due to unexpected input.
Working with External Sources of Input in Python
Python programs can also accept input from external sources such as files and command line arguments.
- Reading from files:
Python provides several functions to read input from files, including the open() function. Here is an example of how to read input from a file:
with open('input.txt', 'r') as f:
data = f.read()
In the example above, we use the open() function to open a file called ‘input.txt’ in read mode. We then use a with statement to ensure that the file is closed properly when we are done with it. We read the contents of the file using the read() method and store the data in the data variable.
- Parsing command line arguments:
Python also provides a module called argparse to parse command line arguments. Here is an example:
import argparse
parser = argparse.ArgumentParser(description='Process some integers.')
parser.add_argument('integers', metavar='N', type=int, nargs='+',
help='an integer for the accumulator')
parser.add_argument('--sum', dest='accumulate', action='store_const',
const=sum, default=max,
help='sum the integers (default: find the max)')
args = parser.parse_args()
print(args.accumulate(args.integers))
In the example above, we create an ArgumentParser object and define two arguments: ‘integers’ and ‘sum’. The ‘integers’ argument accepts one or more integers and the ‘sum’ argument takes no input but will perform a summation on the input integers. We then use the parse_args() method to parse the command line arguments and store them in the args variable. We can then access the values of the arguments using the dot notation (args.integers, args.accumulate).
Common Mistakes to Avoid When Handling Input in Python
When using a Python program to get input from users, there are a few things to watch out for:
- Not checking user input: Always make sure user input is the right type and format. This will prevent the software from experiencing unforeseen errors.
- Not dealing with errors and exceptions: It’s very important to deal with errors and exceptions when getting user input. A ValueError will happen, for instance, if the user provides a string when a number is anticipated. To catch and properly handle errors, use try-except blocks.
- Not giving the user clear or meaningful cues: Always give the user clear or meaningful prompts to explain what input is expected. Hence, confusion and mistakes will decrease.
- Incorrect data types being used: Use the right data type when accepting numeric input (e.g., int or float). By doing so, unanticipated errors will be avoided, and the program’s calculations will be carried out as planned.
- Not cleaning up user input: Always clean up user input to prevent security flaws like SQL injection attacks. To sanitize input, use built-in functions like replace() and strip().
Tips and Tricks for Efficient Input Handling in Python
- Using list comprehensions-
List comprehensions are a powerful tool for processing and filtering input. Here is an example of how to use a list comprehension to filter out all negative numbers from a list:
numbers = [1, -2, 3, -4, 5]
positive_numbers = [x for x in numbers if x > 0]
print(positive_numbers)
- Using the map() function-
The map() function can be used to apply a function to each element of a list. Here is an example of how to use the map() function to convert a list of strings to numeric values:
# Convert list of strings to integers using map()
string_list = ['1', '2', '3', '4']
int_list = list(map(int, string_list))
print(int_list) # Output: [1, 2, 3, 4]
In this example, the map() function applies the int() function to each element of string_list, resulting in a new list of integers.
Conclusion
In conclusion, proper input handling is a critical aspect of Python programming. The built-in input() function is a powerful tool for accepting user input, and it is important to understand its syntax and parameters. Accepting different types of input and handling input errors and exceptions are also essential skills for any Python programmer.
By following best practices for proper input handling, such as input validation and meaningful prompts, you can create more robust and user-friendly programs. Additionally, working with external sources of input, such as reading from files and parsing command line arguments, can greatly expand the capabilities of your programs.
Finally, by using tips and tricks such as list comprehensions and the map() function, you can make your input handling code more efficient and elegant.
Overall, proper input handling is an important skill for any Python programmer to master, and by following the guidelines and examples presented in this article, you can improve the quality and functionality of your Python programs.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
How do you take input in Python?
You can also take input in Python using command line arguments. Command line arguments are passed to your Python script when it is run from the command line. You can access these arguments using the sys module. For example:
import sys
name = sys.argv[1]
What is input () in Python?
input() is a Python function that reads a line from the standard input in python and returns it as a string. The standard input can be a keyboard or another program’s output, depending on how the program is run.
How do you take no input in Python?
If you don’t need any input for your program, you can simply skip the input() function and proceed with your code. For example:print("Hello, world!")
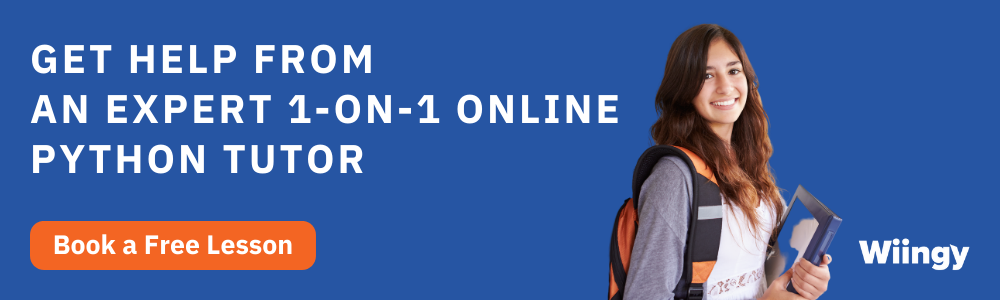
Written by by
Rahul LathReviewed by by
Arpit Rankwar